Getting Started with Go: Your First Steps in Writing, Running, and Building Programs

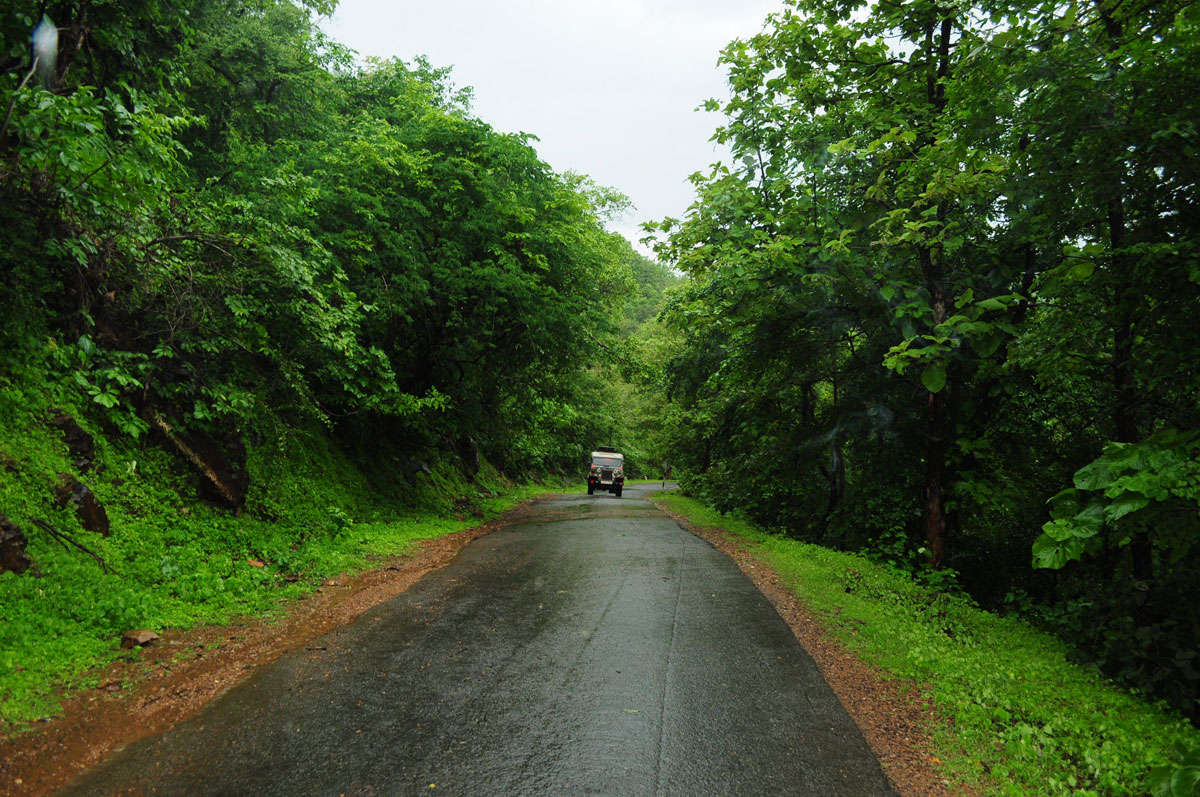
Welcome to the world of Go programming language, also known as Golang! If you are new to Go, this guide is perfect for you. We’ll walk you through creating a simple "Hello, World!" program, explain each line of code, and show you how to run and build your first Go application.
By the end of this tutorial, you’ll have a solid understanding of Go’s basic syntax, how to write code, and how to build an executable file. Let’s get started!
What is Go?
Go is an open-source programming language developed by Google. It is designed to be simple, efficient, and easy to learn. Go combines the performance of low-level languages like C with the simplicity of high-level languages like Python. It’s particularly well-suited for building web servers, cloud services, and command-line tools.
Prerequisites
Before we start coding, make sure you have Go installed on your machine. If you haven’t installed Go yet, check out our guide on installing and setting up Go.
Checking Your Go Installation
To confirm that Go is installed correctly, open your terminal (or Command Prompt on Windows) and run:
go version
You should see output like:
go version go1.x.x linux/amd64
If you see this, you’re good to go!
Writing Your First Go Program
Let’s dive into writing a simple "Hello, World!" program in Go.
Step 1: Create a New Go File
Open your terminal or code editor (VS Code, for example).
Create a new directory for your project:
mkdir hello-go cd hello-go
Create a new file named
main.go
:touch main.go
Step 2: Write the Code
Open main.go
in your editor and type the following code:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Let’s break this down line by line and understand each part.
Code Explanation
Line 1: package main
package main
In Go, every file begins with a package declaration.
A package is a way to group related code together. The
main
package is special in Go — it tells the Go compiler that this file is the entry point of the program.When you run a Go program, the
main
package and itsmain
function are executed first.
Line 3: import "fmt"
import "fmt"
The
import
keyword is used to include packages in your program.Here, we are importing the
fmt
package, which provides functions for formatted I/O (input and output).fmt
stands for "format," and it includes functions likePrintln
,Printf
, andScanln
for printing to the console.
Line 5: func main()
func main() {
This line defines a function named
main
.In Go, every executable program must have a
main
function. It is the entry point of the program and is automatically called when the program starts.The
func
keyword is used to declare a function.
Line 6: fmt.Println("Hello, World!")
fmt.Println("Hello, World!")
This line prints the text "Hello, World!" to the console.
fmt.Println
is a function from thefmt
package. ThePrintln
function stands for "print line," and it prints the text followed by a newline character.The string "Hello, World!" is enclosed in double quotes (
"
), indicating it is a string literal.
Line 7: Closing the Function
}
- This curly brace (
}
) indicates the end of themain
function.
Running Your Go Program
Now that we’ve written the code, let’s run it and see the output.
Step 1: Run the Program
In your terminal, navigate to the directory containing main.go
and type:
go run main.go
Output
Hello, World!
Explanation:
The
go run
command compiles and runs the program in one step. It’s perfect for quickly testing your code.You should see the text "Hello, World!" printed on the screen.
Building Your Go Program
While go run
is convenient, it’s mainly used for testing and development. When you’re ready to share your program or deploy it, you’ll want to build it into an executable file.
Step 1: Build the Executable
To build the program, use the go build
command:
go build main.go
Step 2: Run the Executable
After running go build
, you’ll find an executable file named main
(on Linux or macOS) or main.exe
(on Windows) in your directory. Run the executable using:
On Linux/macOS:
./main
On Windows:
main.exe
Output
Hello, World!
Explanation:
The
go build
command compiles the source code into a standalone executable file.This file can be distributed and run on any machine without requiring Go to be installed.
Understanding Go’s Workflow
To summarize the Go development process:
Write the code in a
.go
file.Run the code using
go run
for quick testing.Build the code using
go build
to create an executable file.Execute the built program directly to see the output.
Additional Tips for Beginners
1. Go File Naming Conventions
Go files usually have a
.go
extension.The main file is often named
main.go
, but you can use any name that follows Go’s naming conventions (lowercase, no spaces, use underscores or camelCase).
2. Formatting Your Code
Go has a built-in tool called gofmt
that automatically formats your code according to standard Go conventions. To format your code, simply run:
gofmt -w main.go
This helps maintain consistency and readability in your code.
3. Checking for Errors
Go provides a command to quickly check your code for syntax errors:
go vet main.go
If there are any issues, go vet
will point them out, making it easier to debug your program.
Conclusion
You’ve just written, run, and built your first Go program! 🎉 In this guide, we covered:
The basic structure of a Go program.
Line-by-line explanation of the "Hello, World!" code.
How to run and build a Go program.
Helpful tips for getting started with Go.
Go is a powerful language, and this is just the beginning. As you continue learning, you’ll discover Go’s unique features, such as its excellent concurrency model, robust standard library, and strong performance.
Stay tuned for more tutorials where we’ll explore Go’s data types, control structures, functions, and advanced features. Happy coding with Go!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
