Easy Integration of Scratchattach and Python: A Complete Guide

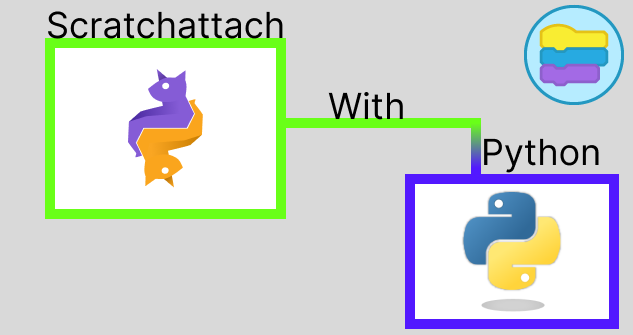
Introduction
Scratchattach is an API wrapper for Scratch. It allows you to do a large variety of things on the Scratch platformer all using Python. It can do so much for your project. But how do you use it? This post will show the full power of Scratchattach. You will even be able to make a full on stat-shower in the end…
What is Scratchattach?
Scratchattach is a Python module created by TimMcCool. It is an API designed to integrate with popular programming platform Scratch. It can do a variety of things, from setting cloud variables to deleting comments.
Now that we know what that is, get your computer out and lets get coding. 💻
How to use it
Scratchattach is a Python module, so you need to install it using pip
:
pip install -U scratchattach
If that doesn’t work, you can try this:
python -m pip install -U scratchattach
Once that installs, you can simply import it into your Python program.
import scratchattach as scratch3 # Imports the Scratchattach module
Next, you need a Scratch project. For now, you can name it whatever you like. The name is insignificant for all of this. Once you create it, look at the URL… it should look something like https://scratch.mit.edu/1234567890. Make note of the 1234567890 in the URL. This is the “project id.” You will need this. Once you have created the Scratch project, head back over to a Python file, import the “scratchattach” module, and enter this code to log you in:
import scratchattach as scratch3 # <--- this was already done
# New part:
username_var = "[username]" # Replace with your actual Scratch username.
password_var = "[password]" # Replace with your actual Scratch password.
# This connects scratchattach to your Scratch account.
session = scratch3.login(username_var, password_var)
This will log you into Scratch using the API. Don’t worry. Scratchattach doesn’t use this information maliciously. I’ve been using the API for years, and my accounts fine.
Now (below that code) we are going to connect this to that project we created. Remember that little number I told you to remember. We are going to need it. Enter this below the code we have above.
# ^ other code up above ^ #
# Replace [Project_ID] with your actual project ID
cloud = session.connect_cloud("[Project_ID]")
Once you have done that, save and run your program. If you did everything correctly, then you should see absolutely nothing. It should just stop. This means everything ran successfully. If you see an error saying The user corresponding to the connected login / session is not allowed to perform this action.
, this means you probably entered your information wrong. Also note if the project ID is wrong, it WILL NOT notify you.
Now you have connected to your project, but right now it isn’t doing anything. Go back to your project and create a new variable called “test var” and make it a cloud variable:
Click the big “OK” and now you have a Cloud variable.
Whoa, wait… what is a Cloud variable?
A cloud variable is a variable that stores numbers. These numbers are stored in a server somewhere, and that number is the same across devices. For example. If a user set the variable to two, then reloaded the page, the variable would say the value was two. However, if I set a normal variable to two, and then reloaded, then the variable is going to be reset to zero.
Changing cloud variables with Scratchattach
Below all the code we have so far, enter this line of code:
cloud.set_var("test var", "2")
Here, we are setting the variable “test vat” to the value of “2.” Save and run the program. Once it completes, check your Scratch project. You don’t need to reload. It should be set to two.
Now, there are also cloud listeners, to tell you when a cloud variable is changed. Here is the code (below the rest):
events = cloud.events()
@events.event
def on_set(activity):
print(f"{activity.var} was set to {activity.value}")
events.start() # <-- DON'T FORGET THIS
What this does, is listens (hints the name) to events, and when it senses that a cloud variable was changed, and then prints on the screen “[VARIABLE NAME] was set to [VARIABLE VALUE].” Try it. Click the “Set [test var] to [4567890] block” and see what happens…
Great! This works. Remember, cloud variables cannot be set to letters. You may be able to run this…
Annndd you might see this…
However, check your program. It won’t print anything. In fact, you can go to https://scratch.mit.edu/cloudmoniter/[YOUR PROJECT ID], and you will see the history of changing it to 4567890, but not anything with letters… annndd if you still don’t believe me. Share it, create/log in to an alternative account, and see the value… it will be 0 or 4567890 depending on what you set it to.
Creating a user-info program
Now, we are going to use scratchattach to get information about users the user of the program enters. Create a new program and import are module:
import scratchattach as s3
Now, we are going to start the loop:
while True:
username = input("Enter the username of the user: ") # Gets user input
username = username.lower()
Next, we are going to use scratchattach to get the user:
try:
user = s3.get_user(username) # <-- this is finding the user.
except:
print('We could not find the user you requested.')
Under the try:
section below the user = scratch3.get_user(username)
, we are going to print the stats:
# user = scratch3.get_user(username) is right above!
print(f"""
- Username: {user}
- UserID: {user.id}
- Join Date: {user.join_date}
- User Country: {user.country}
- Is On Scratch Team: {user.scratchteam}
- Followers: {user.follower_count()}
- Is new Scratcher: {user.is_new_scratcher()}
- Unread Messages: {user.message_count()}
----------------------ABOUT-ME----------------------
{user.about_me}
------------------WHAT-I-A-WORKING-ON---------------
{user.wiwo}
----------------------------------------------------
""")
Whooaaa. That was a big chunk of code. Let’s go over it:
- Username: {user}
: This confirms the username of the user. Rather then print your input, it prints out the Scratchattach result- UserID: {user.id}
: Prints there user ID… not quiiittee sure why this exists.- Join Date: {user.join_date}
: Prints when the user joined Scratch.- User Country: {user.country}
: This is the country that is registered on there account.- Is On Scratch Team: {user.scratchteam}
: This prints whether or not the user is part of the Scratch team. Most of them aren’t.- Followers: {user.follower_count()}
: This shows how many users follow them.- Is new Scratcher: {user.is_new_scratcher()}
: Whether or not the user is a new Scratcher.- Unread Messages: {user.message_count()}
: This is a creepy one, because you can only see it through Scratchattach… it shows how many unread Scratch messages they have.{user.about_me}
: What there “About me” page is.{user.wiwo}
: What there “What I am Working On” page is.
Together, the entire code is this:
import scratchattach as s3 # Import the scratchattach module.
# Do this forever!
while True:
username = input("Enter the username of the user: ") # Gets user input
username = username.lower() # Make the input lowercase.
try:
user = s3.get_user(username) # <-- this is finding the user.
# Print the stats.
print(f"""
- Username: {user}
- UserID: {user.id}
- Join Date: {user.join_date}
- User Country: {user.country}
- Is On Scratch Team: {user.scratchteam}
- Followers: {user.follower_count()}
- Is new Scratcher: {user.is_new_scratcher()}
- Unread Messages: {user.message_count()}
----------------------ABOUT-ME----------------------
{user.about_me}
------------------WHAT-I-A-WORKING-ON---------------
{user.wiwo}
----------------------------------------------------
""")
except: # In case they enter an invalid username... or just something is wrong
print('We could not find the user you requested.') # Print the error message.
Save and run the program. Enter a username like… oh, I don’t know… MilesWK?
Enter a name, and watch the stats appear… or an error message if you enter an invalid name. The program is NOT case-sensitive due to that username = username.lower
program.
Conclusion
We now know the basics of scratchattach! Way to go! 🥳. There is still a bunch of stuff I didn’t cover (because even I don’t fully know it all! 😀). Anyway, If you enjoyed this, check out my other posts. I want feedback on how I can make my posts better, so if you have suggestions, please post them in the comments below!
Thank you for reading this! See you all next time! 👋
— MilesWK
Links:
Subscribe to my newsletter
Read articles from MilesWK directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

MilesWK
MilesWK
Hi! I am MilesWK: a programmer and musician from planet Earth.*