Variable Declaration and Types in Go: A Beginner’s Guide

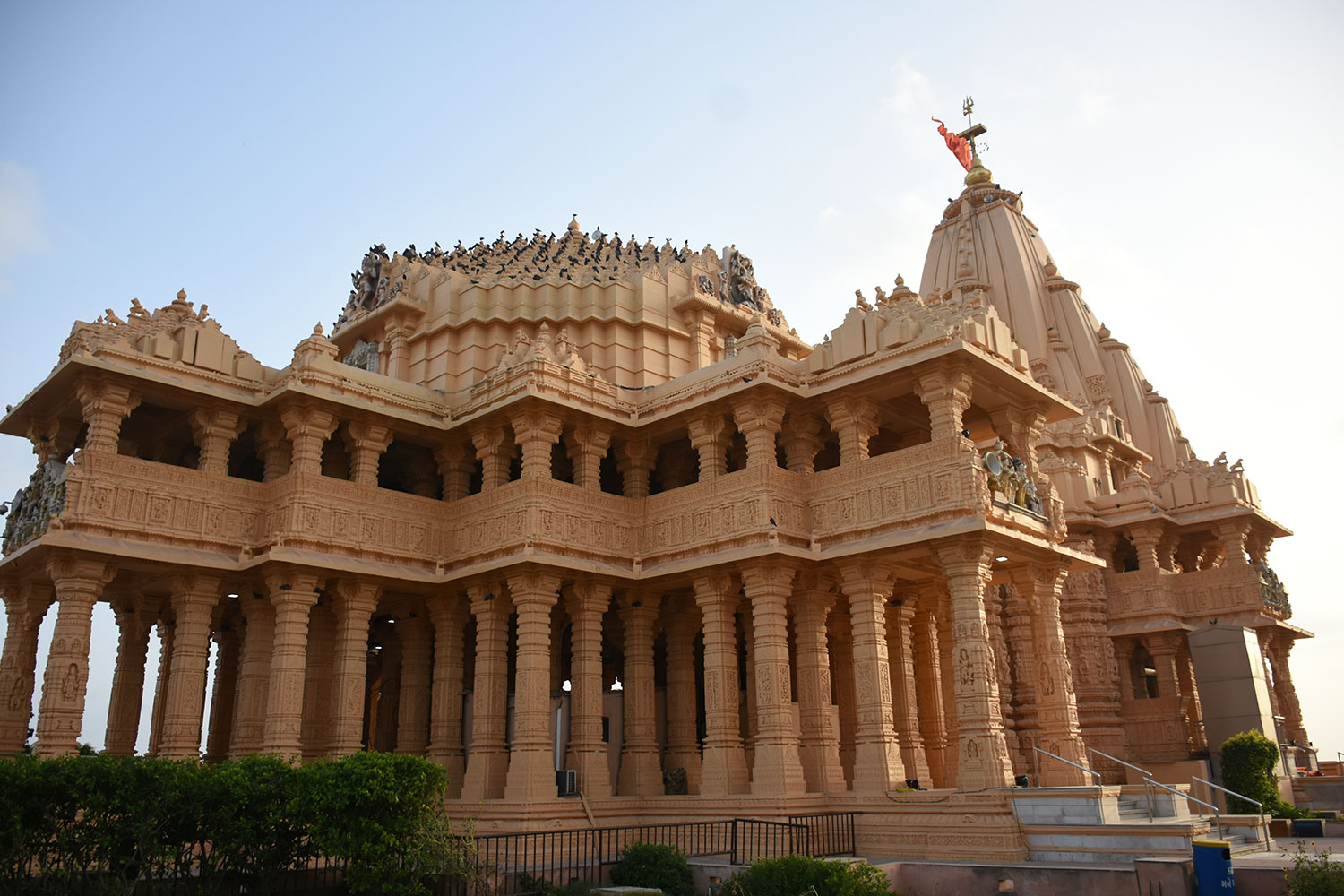
In Go, variables are a fundamental building block of programming. They are used to store data, which can be manipulated and used throughout your program. Understanding how to declare variables and work with different types is essential for any beginner. This article will introduce you to Go’s variable declaration syntax, types, and best practices, with line-by-line explanations of example code.
What is a Variable?
A variable is a named storage location that holds a value. The value of a variable can be changed throughout the program. In Go, variables have a type, which specifies what kind of data they can hold, such as integers, floating-point numbers, strings, or booleans.
Declaring Variables in Go
Go provides several ways to declare variables:
Using the
var
keywordShort variable declaration (using
:=
)Declaring multiple variables at once
Declaring constants
Let’s go through each of these in detail.
1. Variable Declaration Using the var
Keyword
The most basic way to declare a variable in Go is by using the var
keyword.
Example 1: Basic Declaration
package main
import "fmt"
func main() {
var message string
message = "Hello, Go!"
fmt.Println(message)
}
Code Explanation
Line 1:
package main
- This declares the package name. The
main
package is the starting point of the program.
- This declares the package name. The
Line 3:
import "fmt"
- We import the
fmt
package, which provides functions for formatted input and output, such asPrintln
.
- We import the
Line 5:
var message string
We declare a variable named
message
using thevar
keyword.The type of the variable is
string
, which means it can hold text (a sequence of characters).
Line 6:
message = "Hello, Go!"
- We assign the value
"Hello, Go!"
to the variablemessage
.
- We assign the value
Line 7:
fmt.Println(message)
- This prints the value of
message
to the console.
- This prints the value of
Output
Hello, Go!
Key Points:
The
var
keyword is used to declare a variable.You must specify the type of the variable (in this case,
string
).You can assign a value to the variable after declaring it.
2. Short Variable Declaration (Using :=
)
In Go, there is a shorthand for declaring and initializing a variable in a single line using :=
.
Example 2: Short Declaration
package main
import "fmt"
func main() {
message := "Hello, Go!"
number := 42
fmt.Println(message, number)
}
Code Explanation
Line 5:
message := "Hello, Go!"
We declare and initialize the
message
variable using:=
.The type is inferred from the assigned value. Here,
"Hello, Go!"
is a string, somessage
is a string.
Line 6:
number := 42
We declare and initialize the
number
variable.The type is inferred as
int
(integer) because42
is a whole number.
Line 7:
fmt.Println(message, number)
- This prints both variables to the console.
Output
Hello, Go! 42
Key Points:
The
:=
syntax can only be used inside functions.The type of the variable is inferred automatically.
This is a convenient way to declare variables when you know the initial value.
3. Declaring Multiple Variables
You can declare multiple variables in a single line or in a block using parentheses.
Example 3: Multiple Variable Declaration
package main
import "fmt"
func main() {
var name, city string
var age, score int
name = "Alice"
city = "New York"
age = 25
score = 90
fmt.Println(name, city, age, score)
}
Code Explanation
Line 5-6: Declaring multiple variables
We declare two string variables (
name
andcity
) and two integer variables (age
andscore
).This makes the code more concise and easier to read.
Line 7-10: Assigning values
- We assign values to the declared variables.
Line 11: Printing the values
- The
fmt.Println
function prints all the variables to the console.
- The
Output
Alice New York 25 90
Key Points:
You can declare multiple variables of the same type in one line.
This is useful when you have several variables of the same type.
4. Constants in Go
A constant is a variable whose value cannot be changed after it is declared. Constants are declared using the const
keyword.
Example 4: Declaring a Constant
package main
import "fmt"
func main() {
const pi = 3.14
const greeting = "Welcome to Go!"
fmt.Println(greeting, "The value of pi is:", pi)
}
Code Explanation
Line 5-6: Declaring constants
We declare
pi
andgreeting
as constants using theconst
keyword.The value of a constant cannot be changed once it is set.
Line 7: Printing the constants
- We print the constants to the console.
Output
Welcome to Go! The value of pi is: 3.14
Key Points:
Constants are useful for values that should not change throughout the program (e.g., mathematical constants).
Constants can hold any type of value (string, int, float, etc.).
Data Types in Go
Go is a statically typed language, meaning that each variable must have a specific type. Here are some common data types:
Basic Types:
int: Integer numbers (e.g., 42)
float64: Floating-point numbers (e.g., 3.14)
string: Text (e.g., "Hello")
bool: Boolean values (
true
orfalse
)
Composite Types:
array: A fixed-size list of elements.
slice: A dynamically-sized list of elements.
map: A collection of key-value pairs.
struct: A collection of fields.
Example 5: Different Data Types
package main
import "fmt"
func main() {
var age int = 30
var height float64 = 5.9
var isStudent bool = false
var name string = "John"
fmt.Printf("Name: %s, Age: %d, Height: %.1f, Student: %t\n", name, age, height, isStudent)
}
Code Explanation
Line 5-8: Declaring variables with types
- We explicitly specify the type of each variable (
int
,float64
,bool
,string
).
- We explicitly specify the type of each variable (
Line 10: Using
fmt.Printf
We use
fmt.Printf
for formatted output.%s
is a placeholder for strings,%d
for integers,%.1f
for floating-point numbers, and%t
for booleans.
Output
Name: John, Age: 30, Height: 5.9, Student: false
Conclusion
In this guide, we covered:
The basics of variable declaration in Go.
How to use the
var
keyword and the shorthand:=
syntax.Declaring multiple variables and constants.
An introduction to data types.
Understanding variables and data types is a crucial first step in mastering Go. With this foundation, you can start developing more complex programs and exploring Go’s powerful features.
Stay tuned for upcoming tutorials on Control Flow, Functions and Methods, Arrays, Slices, and Maps etc.
Happy coding with Go!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
