Day 35: Mastering ConfigMaps and Secrets in Kubernetes

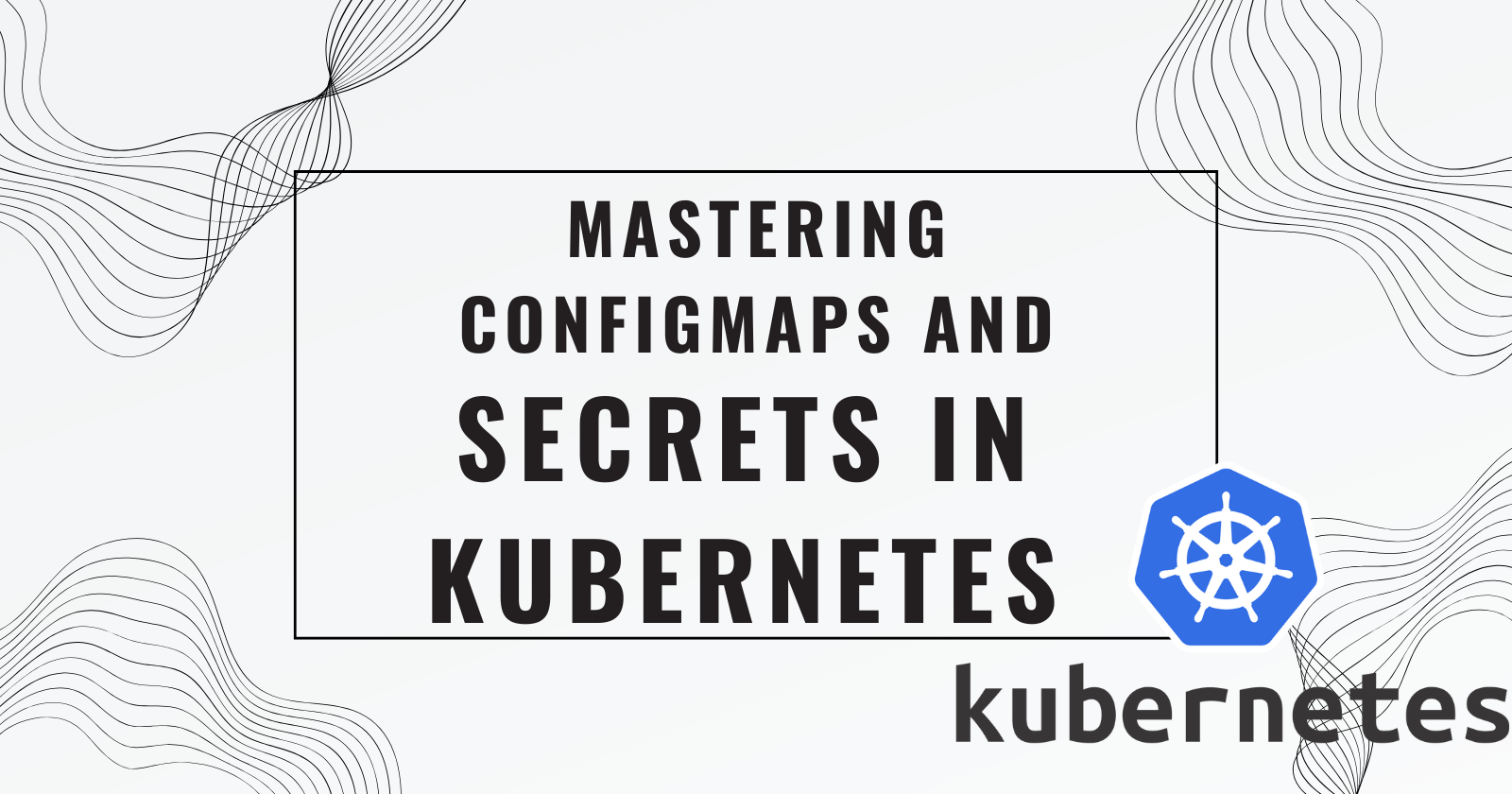
Yesterday was a big win! We took on Kubernetes Namespaces and Services, and now it’s time to dive into another essential part of Kubernetes: ConfigMaps and Secrets.
What Are ConfigMaps and Secrets in Kubernetes?
In Kubernetes, ConfigMaps and Secrets help manage and organize different types of data in our clusters:
ConfigMaps: Used to store general configuration data (like settings or URLs) as key-value pairs.
Secrets: Hold sensitive information (such as passwords) in an encrypted format, securing your data from unauthorized access.
Real-Life Analogy:
Imagine managing a spaceship (our Kubernetes cluster) with different compartments (containers). ConfigMaps are like a shared cabinet where compartments can access necessary instructions, while Secrets are like a safe where sensitive information is securely stored.
Real-Life Project Example: Connecting a Python Application to MySQL in Kubernetes
This project will demonstrate how to deploy a Python application in Kubernetes that connects to a MySQL database. We’ll use ConfigMaps for general settings and Secrets to securely manage MySQL credentials.
Objective
Build a Kubernetes-based Python application that connects to a MySQL database using ConfigMaps and Secrets for configuration management and security.
Prerequisites
Ensure you have:
A running Kubernetes cluster.
MySQL and Python installed locally to test the app.
kubectl
installed to manage Kubernetes resources.
Today’s Tasks: Hands-On with ConfigMaps and Secrets
Task 1: Set Up MySQL Deployment and Service
Create MySQL Deployment:
Create a file named
mysql-deployment.yml
to define a MySQL deployment and service in Kubernetes.apiVersion: apps/v1 kind: Deployment metadata: name: mysql spec: replicas: 1 selector: matchLabels: app: mysql template: metadata: labels: app: mysql spec: containers: - name: mysql image: mysql:5.7 env: - name: MYSQL_ROOT_PASSWORD valueFrom: secretKeyRef: name: mysql-secret key: password ports: - containerPort: 3306 --- apiVersion: v1 kind: Service metadata: name: mysql spec: selector: app: mysql ports: - protocol: TCP port: 3306 targetPort: 3306
Apply MySQL Deployment:
Use the command below to create the MySQL database in Kubernetes:
kubectl apply -f mysql-deployment.yml
Task 2: Create a Secret for MySQL Credentials
Define MySQL Secret:
Store the MySQL root password in a Kubernetes Secret. Create a file named
mysql-secret.yml
:apiVersion: v1 kind: Secret metadata: name: mysql-secret type: Opaque data: password: root@1234 # Replace "password" with your MySQL root password
You can generate the base64 string for your password with:
echo -n "yourpassword" | base64
Apply the Secret:
Run this command to create the Secret in your Kubernetes cluster:
kubectl apply -f mysql-secret.yml
Task 3: Set Up a ConfigMap for Database Connection Settings
Create ConfigMap:
Set up a ConfigMap to store general settings like the database name and host. Create a file called
mysql-configmap.yml
:apiVersion: v1 kind: ConfigMap metadata: name: mysql-config data: DB_HOST: mysql DB_NAME: mydatabase DB_USER: root
Apply ConfigMap:
Run this command to add the ConfigMap to your cluster:
kubectl apply -f mysql-configmap.yml
Task 4: Deploy the Python Application
Create a Python Script to Connect to MySQL:
Here’s a basic Python script (
app.py
) to connect to the MySQL database using environment variables:import os import mysql.connector from mysql.connector import Error def create_connection(): try: connection = mysql.connector.connect( host=os.getenv("DB_HOST"), user=os.getenv("DB_USER"), password=os.getenv("DB_PASSWORD"), database=os.getenv("DB_NAME") ) if connection.is_connected(): print("Connected to MySQL database") except Error as e: print(f"Error: {e}") return connection if __name__ == "__main__": create_connection()
Create a Deployment for the Python App:
Define a Kubernetes deployment (
python-app.yml
) to run the Python application:apiVersion: apps/v1 kind: Deployment metadata: name: python-app spec: replicas: 1 selector: matchLabels: app: python-app template: metadata: labels: app: python-app spec: containers: - name: python-app image: python:3.8 command: ["python", "/app/app.py"] env: - name: DB_HOST valueFrom: configMapKeyRef: name: mysql-config key: DB_HOST - name: DB_NAME valueFrom: configMapKeyRef: name: mysql-config key: DB_NAME - name: DB_USER valueFrom: configMapKeyRef: name: mysql-config key: DB_USER - name: DB_PASSWORD valueFrom: secretKeyRef: name: mysql-secret key: password volumeMounts: - name: app-volume mountPath: /app volumes: - name: app-volume configMap: name: python-app-config
Apply the Python App Deployment:
Use the command below to create the Python app deployment:
kubectl apply -f python-app.yml
Verify the Python App Deployment:
Check the logs to confirm the Python app is connecting to MySQL:
kubectl logs -l app=python-app
Wrapping Up
In this project, we used ConfigMaps to manage general database settings and Secrets to secure sensitive credentials. With this setup, our Python app can connect to MySQL safely and efficiently within Kubernetes.
Happy coding, and keep building securely!
Subscribe to my newsletter
Read articles from Dhruv Moradiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
