Mastering Constants and Zero Values for Cleaner Code

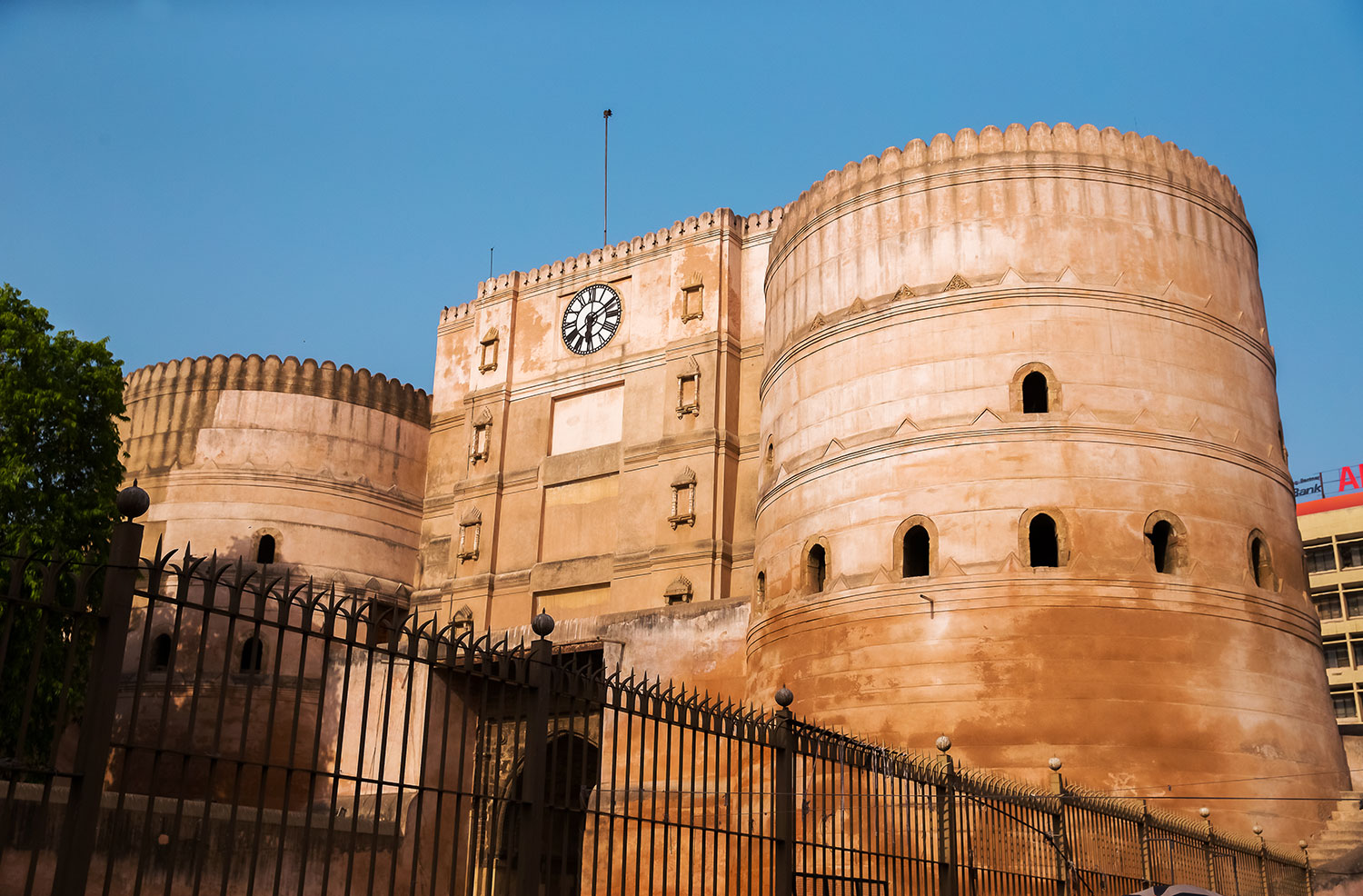
In Go, constants and zero values are important concepts that provide a strong foundation for writing robust, efficient, and predictable code. Understanding these concepts is crucial as they help prevent bugs and make your code easier to read and maintain. In this article, we’ll explore:
What constants are and how to use them
How Go handles default values with zero values
Detailed code examples with line-by-line explanations
Let’s dive in!
What is a Constant?
A constant in Go is a variable whose value cannot be changed once it has been assigned. Constants are declared using the const
keyword and are often used to represent fixed values that are known at compile time, such as mathematical constants, configuration settings, or fixed strings.
Why Use Constants?
Code Clarity: Constants make your code more readable and descriptive.
Prevents Errors: They protect against accidental changes since their values cannot be modified after declaration.
Performance: Using constants can be more efficient because their values are known at compile time.
Declaring Constants in Go
Constants are declared using the const
keyword, followed by the name, value, and optionally, the type.
Example 1: Declaring Basic Constants
package main
import "fmt"
func main() {
const pi = 3.14
const greeting string = "Hello, Go!"
fmt.Println("Value of pi:", pi)
fmt.Println(greeting)
}
Code Explanation
Line 1:
package main
- This declares the package. The
main
package is used as the entry point of the program.
- This declares the package. The
Line 3:
import "fmt"
- We import the
fmt
package, which provides functions for printing formatted output.
- We import the
Line 5-6: Declaring constants
We declare
pi
as a constant with a value of3.14
. The type is inferred asfloat64
.We also declare
greeting
with an explicit type ofstring
and a value of"Hello, Go!"
.
Line 8-9: Printing constants
- We use
fmt.Println
to print the values of the constants.
- We use
Output
Value of pi: 3.14
Hello, Go!
Key Points:
Constants are declared using the
const
keyword.They cannot be modified after declaration.
You can optionally specify the type, but Go can also infer it.
Rules for Constants
Compile-Time Value: The value of a constant must be known at compile time. This means you cannot use a value that is determined during program execution.
Supported Types: Constants can be of types like
int
,float64
,string
, andbool
.No Variables: Constants cannot be assigned a value from a variable, only from literals or other constants.
Example 2: Invalid Constant Declaration
package main
func main() {
const value = 5
const result = value * 2
// This line will cause an error because "userInput" is a variable.
var userInput = 10
const total = userInput + value
}
Output
# command-line-arguments
.\main.go:9:19: userInput + value (value of type int) is not constant
Explanation
- The above code will result in an error because
userInput
is a variable, and its value is not known at compile time. Constants must be assigned using literals or other constants, not variables.
Zero Values in Go
In Go, variables that are declared but not explicitly initialized are automatically assigned a zero value. The zero value is the default value for a variable’s type. This helps prevent unpredictable behavior caused by uninitialized variables.
Common Zero Values
Data Type | Zero Value |
int | 0 |
float64 | 0.0 |
string | "" (empty string) |
bool | false |
pointer , slice , map , chan , func , interface | nil |
Example 3: Understanding Zero Values
package main
import "fmt"
func main() {
var age int
var height float64
var name string
var isStudent bool
fmt.Println("Default age:", age)
fmt.Println("Default height:", height)
fmt.Println("Default name:", name)
fmt.Println("Default isStudent:", isStudent)
}
Code Explanation
Line 5-8: Declaring variables without initialization
- We declare variables
age
,height
,name
, andisStudent
without assigning any values.
- We declare variables
Line 10-13: Printing zero values
- We use
fmt.Println
to print the values of the variables. Since they are not initialized, they have their respective zero values.
- We use
Output
Default age: 0
Default height: 0
Default name:
Default isStudent: false
Key Points:
Go assigns zero values to uninitialized variables automatically.
This feature helps avoid errors caused by accessing uninitialized variables.
Zero values depend on the type of the variable.
Using Constants and Zero Values Together
Constants and zero values can be used together effectively to make your code more predictable.
Example 4: Constants and Zero Values in Action
package main
import "fmt"
func main() {
const maxScore = 100
var score int
fmt.Println("Initial score:", score)
fmt.Println("Maximum score:", maxScore)
// Updating score
score = 85
fmt.Println("Updated score:", score)
}
Code Explanation
Line 5: Declaring a constant
- We declare
maxScore
as a constant with a value of100
.
- We declare
Line 6: Declaring an uninitialized variable
- We declare
score
as an integer without initializing it, so it has a zero value of0
.
- We declare
Line 9: Printing the initial score
- This prints the zero value of
score
(0
).
- This prints the zero value of
Line 13: Updating the score
- We assign a new value (
85
) toscore
and print it.
- We assign a new value (
Output
Initial score: 0
Maximum score: 100
Updated score: 85
Key Points:
Using a constant for
maxScore
ensures that its value remains fixed throughout the program.The zero value for
score
provides a safe default before it is explicitly assigned.
Best Practices for Using Constants and Zero Values
Use Constants for Fixed Values: If a value should not change, use a constant to make your code more robust and readable.
Rely on Zero Values for Default Initialization: When declaring variables, you don’t always need to initialize them. Use the zero value as a sensible default.
Avoid Magic Numbers: Instead of using raw numbers in your code, use constants with descriptive names to make your code easier to understand.
Example 5: Using Constants for Code Clarity
package main
import "fmt"
func main() {
const taxRate = 0.05
var price float64 = 100
var total = price + (price * taxRate)
fmt.Printf("Price: $%.2f, Tax Rate: %.2f, Total: $%.2f\n", price, taxRate, total)
}
Code Explanation
We use a constant
taxRate
to represent the tax rate, making the code more readable.The total price is calculated using the constant, avoiding any confusion about what the
0.05
value represents.
Output
Price: $100.00, Tax Rate: 0.05, Total: $105.00
Conclusion
In this article, we covered:
How to declare and use constants in Go
The rules and benefits of using constants
The concept of zero values and how Go handles uninitialized variables
Best practices for using constants and zero values effectively
With a strong grasp of constants and zero values, you can write clearer, safer, and more efficient Go programs. In upcoming articles, we’ll dive into more advanced topics, including
Stay tuned with us for upcoming tutorials on Control Structures, Data Structures, Error Handling, etc.
Happy coding with Go!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
