Mastering Arithmetic, Relational, and Logical Operators

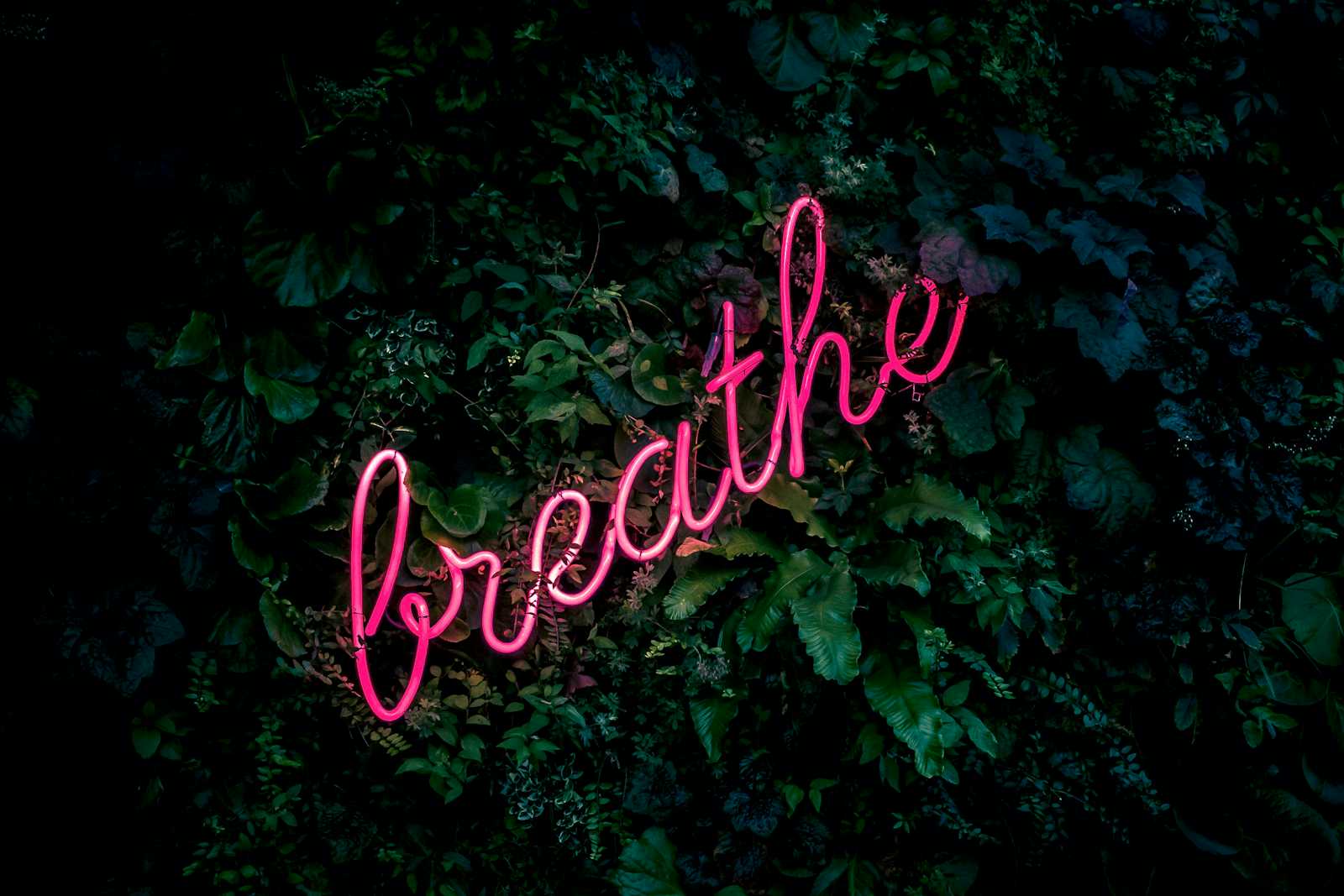
Operators are essential building blocks in any programming language, including Go. They allow you to perform various operations on values and variables. In this article, we’ll cover:
Arithmetic operators: For mathematical calculations
Relational operators: For comparing values
Logical operators: For combining multiple conditions
By the end of this article, you’ll understand how to use these operators in Go, with detailed explanations and code examples.
1. Arithmetic Operators
Arithmetic operators are used for basic mathematical calculations like addition, subtraction, multiplication, and division.
List of Arithmetic Operators
Operator | Description | Example |
+ | Addition | a + b |
- | Subtraction | a - b |
* | Multiplication | a * b |
/ | Division | a / b |
% | Modulus (Remainder) | a % b |
Example 1: Using Arithmetic Operators
package main
import "fmt"
func main() {
var a, b int = 10, 3
sum := a + b
difference := a - b
product := a * b
quotient := a / b
remainder := a % b
fmt.Println("Sum:", sum)
fmt.Println("Difference:", difference)
fmt.Println("Product:", product)
fmt.Println("Quotient:", quotient)
fmt.Println("Remainder:", remainder)
}
Code Explanation
Line 5: We declare two integer variables
a
andb
with values10
and3
.Line 7-11: We use arithmetic operators to calculate the sum, difference, product, quotient, and remainder of
a
andb
.Line 13-17: We print the results using
fmt.Println
.
Output
Sum: 13
Difference: 7
Product: 30
Quotient: 3
Remainder: 1
Key Points:
Division of integers results in an integer quotient (it discards the remainder).
The modulus operator
%
gives the remainder of integer division.
2. Relational Operators
Relational operators are used to compare two values. They return a boolean value (true
or false
) based on the comparison.
List of Relational Operators
Operator | Description | Example |
== | Equal to | a == b |
!= | Not equal to | a != b |
> | Greater than | a > b |
< | Less than | a < b |
>= | Greater than or equal to | a >= b |
<= | Less than or equal to | a <= b |
Example 2: Using Relational Operators
package main
import "fmt"
func main() {
var x, y int = 5, 10
fmt.Println("x == y:", x == y)
fmt.Println("x != y:", x != y)
fmt.Println("x > y:", x > y)
fmt.Println("x < y:", x < y)
fmt.Println("x >= y:", x >= y)
fmt.Println("x <= y:", x <= y)
}
Code Explanation
Line 5: We declare two integer variables
x
andy
with values5
and10
.Line 7-12: We use relational operators to compare
x
andy
and print the results.
Output
x == y: false
x != y: true
x > y: false
x < y: true
x >= y: false
x <= y: true
Key Points:
Relational operators return boolean values (
true
orfalse
).These comparisons are useful for decision-making in your code, such as in conditional statements.
3. Logical Operators
List of Logical Operators
Logical operators are used to combine multiple conditions or boolean expressions. They return true
or false
based on the result of the combined expressions.
List of Logical Operators
Example 3: Using Logical Operators
package main
import "fmt"
func main() {
var age int = 25
var hasID bool = true
// Check if age is greater than 18 AND has an ID
canVote := age > 18 && hasID
// Check if age is less than 18 OR does not have an ID
cannotVote := age < 18 || !hasID
fmt.Println("Can Vote:", canVote)
fmt.Println("Cannot Vote:", cannotVote)
}
Code Explanation
Line 5-6: We declare two variables:
age
(integer) andhasID
(boolean).Line 9: We use the
&&
(AND) operator to check if the person’s age is greater than18
and they have an ID.Line 11: We use the
||
(OR) operator to check if the person’s age is less than18
or they do not have an ID. We also use the!
(NOT) operator to negate thehasID
value.Line 13-14: We print the results of the logical expressions.
Output
Can Vote: true
Cannot Vote: false
Key Points:
The
&&
(AND) operator returnstrue
if both conditions are true.The
||
(OR) operator returnstrue
if at least one condition is true.The
!
(NOT) operator reverses the value of a boolean (true
becomesfalse
and vice versa).
Combining Operators
You can combine arithmetic, relational, and logical operators in a single expression for more complex conditions.
Example 4: Complex Expression
package main
import "fmt"
func main() {
var a, b, c int = 10, 20, 30
result := (a + b > c) && (b < c)
fmt.Println("Result of expression:", result)
}
Step-by-Step Analysis
Variable Declaration:
var a, b, c int = 10, 20, 30
a
is assigned the value10
.b
is assigned the value20
.c
is assigned the value30
.
Boolean Expression:
result := (a + b > c) && (b < c)
The expression
(a + b > c)
evaluates first:a + b
is10 + 20 = 30
.30 > 30
is false (because 30 is not greater than 30).
The expression
(b < c)
evaluates next:b
is20
andc
is30
.20 < 30
is true.
Logical AND (
&&
) Operation:The overall expression is
(a + b > c) && (b < c)
.Since
(a + b > c)
is false and(b < c)
is true, the result of the logical AND is false.- AND operation (
&&
) returnstrue
only if both operands aretrue
.
- AND operation (
Output
Result of expression: false
Key Points:
Go evaluates expressions from left to right, following operator precedence (arithmetic > relational > logical).
Parentheses can be used to control the order of evaluation and make the expression clearer.
Conclusion
In this article, we covered:
Arithmetic operators: Used for basic mathematical operations.
Relational operators: Used to compare values and return boolean results.
Logical operators: Used to combine multiple boolean expressions.
By mastering these operators, you gain the ability to create more complex and powerful expressions in your Go programs. This knowledge is fundamental to implementing logic, handling conditions, and making decisions in your code.
Happy coding with Go!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
