Modern ESLint Configuration in React: Managing Prop Types and Unused Variables Warnings
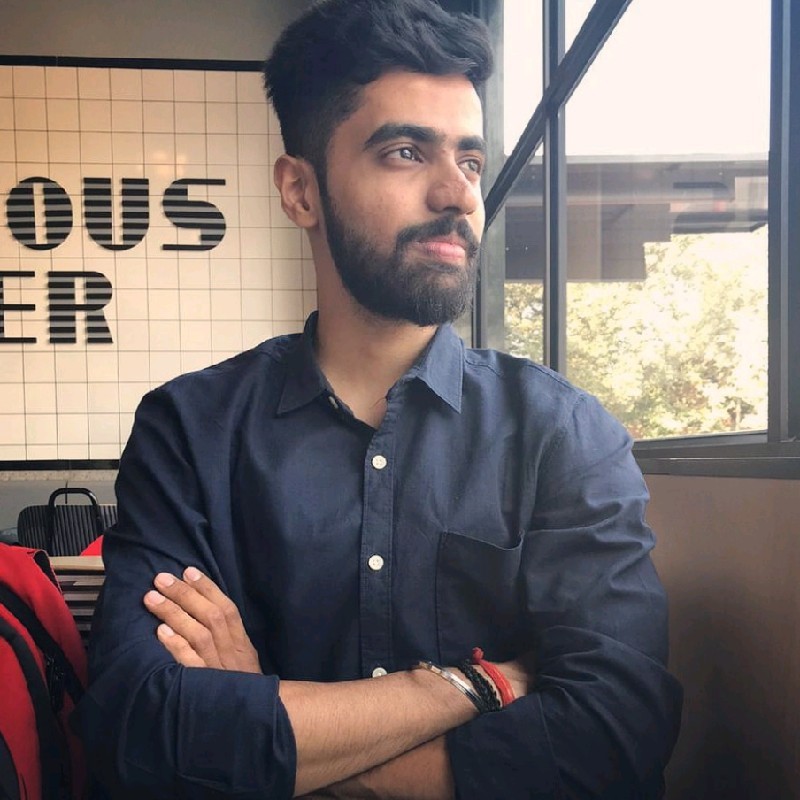
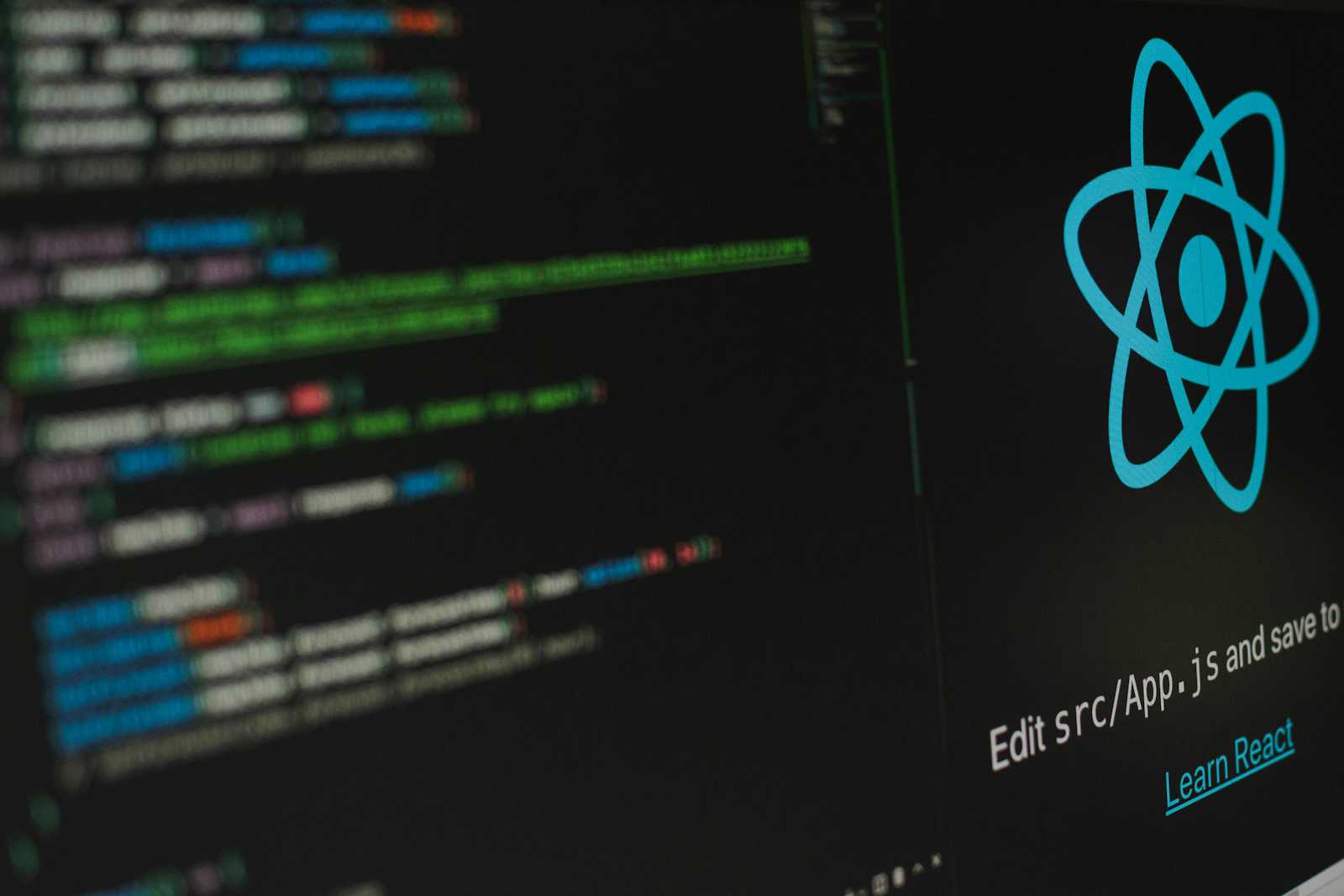
When working on React projects, ESLint is an invaluable tool for enforcing code quality. But sometimes, the default rules may not align with the specific needs of your project. In this article, we’ll explore how to configure ESLint for React applications to disable prop-types
checks (especially if using TypeScript) and manage no-unused-vars
warnings effectively.
Setting Up ESLint for React
To start, let’s ensure your ESLint setup is ready. Typically, a modern React project includes a file named eslint.config.js
. Here’s an example setup:
import js from '@eslint/js';
import globals from 'globals';
import react from 'eslint-plugin-react';
import reactHooks from 'eslint-plugin-react-hooks';
import reactRefresh from 'eslint-plugin-react-refresh';
export default [
{ ignores: ['dist'] },
{
files: ['**/*.{js,jsx}'],
languageOptions: {
ecmaVersion: 2020,
globals: globals.browser,
parserOptions: {
ecmaVersion: 'latest',
ecmaFeatures: { jsx: true },
sourceType: 'module',
},
},
settings: { react: { version: '18.3' } },
plugins: {
react,
'react-hooks': reactHooks,
'react-refresh': reactRefresh,
},
rules: {
...js.configs.recommended.rules,
...react.configs.recommended.rules,
...react.configs['jsx-runtime'].rules,
...reactHooks.configs.recommended.rules,
'react/jsx-no-target-blank': 'off',
'react-refresh/only-export-components': [
'warn',
{ allowConstantExport: true },
],
},
},
];
Updating ESLint Rules
To adjust prop-types
and no-unused-vars
rules:
Disable Prop-Types Rule: If your project doesn’t use
prop-types
, add'react/prop-types': 'off'
to therules
section.Customize No-Unused-Vars Rule: ESLint’s
no-unused-vars
rule flags unused variables, which can be helpful but sometimes too restrictive. Customize it as needed:'no-unused-vars': ['warn', { vars: 'all', args: 'none' }],
Here’s the updated eslint.config.js
:
coderules: {
...js.configs.recommended.rules,
...react.configs.recommended.rules,
...react.configs['jsx-runtime'].rules,
...reactHooks.configs.recommended.rules,
'react/jsx-no-target-blank': 'off',
'react-refresh/only-export-components': [
'warn',
{ allowConstantExport: true },
],
'react/prop-types': 'off', // Disable prop-types validation
'no-unused-vars': ['warn', { vars: 'all', args: 'none' }], // Customize no-unused-vars
}
Final Thoughts
With these updates, your ESLint setup should be better aligned with your project’s requirements. Fine-tuning ESLint can reduce noise in your codebase, focusing your team on the warnings that truly matter.
Subscribe to my newsletter
Read articles from Shikhar Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
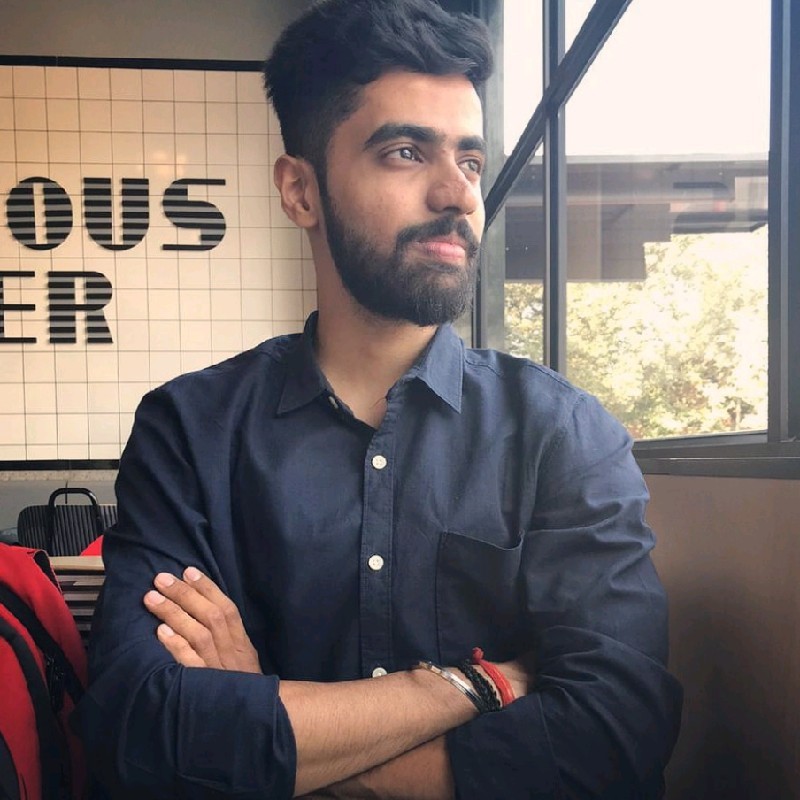