DOMination: Understanding the Document Object Model!
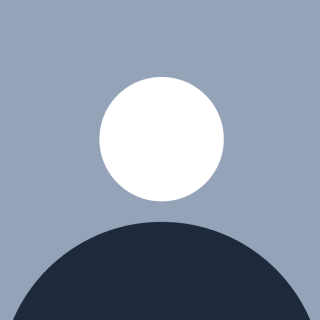
Table of contents
- What is the DOM? A Deep Dive into the Blueprint
- Basic DOM Manipulation Techniques
- Adding and Removing Elements
- Building the Foundations of DOM Manipulation
- Interacting with the DOM — Event Listeners
- Making the City Interactive — Adding Event Listeners
- Dynamically Adding and Removing Buildings
- Challenge Time - Build Your Interactive City Scene
- Mastering DOM Manipulation and Interactivity
- Join me for DOM Methods in-depth!
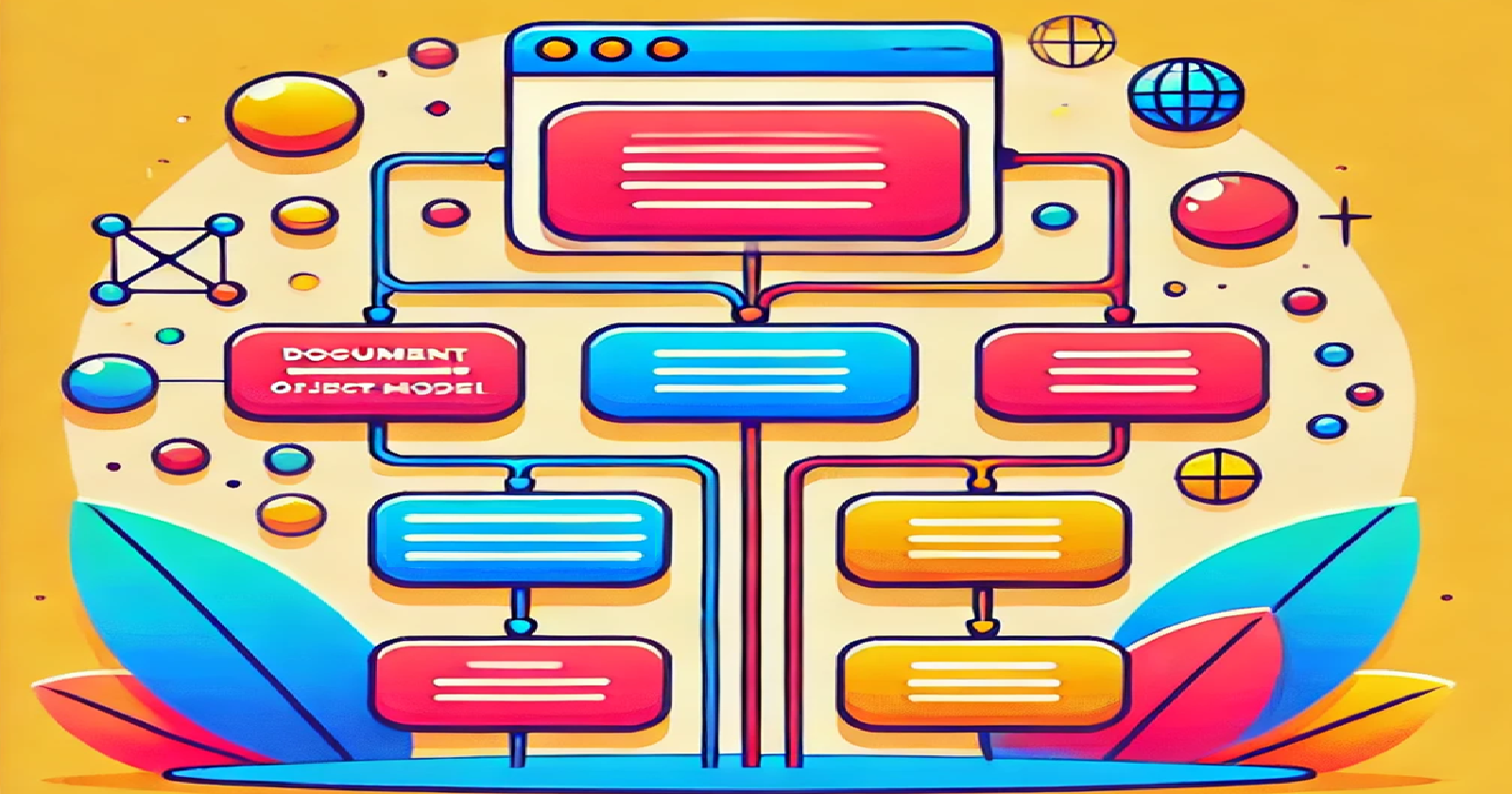
Imagine stepping into a vast city, where every building, street, and park is meticulously planned. You, as the city planner, have complete control over how the city is built, modified, and maintained. This city represents the Document Object Model (DOM) of your webpage. The DOM is the blueprint or the architectural plan of your web page, outlining how every piece of content is structured and how it can be manipulated.
But the DOM isn't just a static blueprint. It’s dynamic, meaning that with JavaScript, you can change the structure, content, and style of your webpage on the fly, turning a static page into a fully interactive and responsive web experience.
In this article, we’ll dive into what the DOM is, how it’s structured, and how you can manipulate it using JavaScript. By the end of this article, you’ll have the tools to start controlling and shaping your web page like a city builder.
What is the DOM? A Deep Dive into the Blueprint
The Document Object Model (DOM) is a tree-like structure that represents the HTML or XML content of your webpage. It’s called a tree because it starts with a single root (the document
) and branches out into smaller elements like <html>
, <body>
, <div>
, and so on.
In simple terms, the DOM is the interface between JavaScript and your webpage. It allows JavaScript to interact with the HTML content by representing every HTML element as an object. These objects can be accessed, modified, added, or removed via JavaScript.
Properties and Methods of the DOM
Every element in the DOM has properties and methods. These properties describe the characteristics of the element (such as its ID, class, or content), and methods are actions you can perform on the element (such as modifying its style, adding or removing it, or responding to user events).
For example:
Properties:
id
,className
,innerHTML
,style
,childNodes
.Methods:
getElementById()
,querySelector()
,appendChild()
,removeChild()
.
Accessing the DOM
JavaScript can access the DOM through the document
object. The document
object represents the entire webpage and provides several methods to interact with the various elements.
Common ways to access elements in the DOM:
getElementById(id)
: Selects an element by its ID.getElementsByClassName(className)
: Selects all elements with a given class.querySelector(selector)
: Selects the first element that matches a CSS selector.querySelectorAll(selector)
: Selects all elements that match a CSS selector.
Flowchart: The DOM Structure
Let’s visualize the DOM as a tree-like structure:
[Document]
|
[<html>]
|
[<head>] [<body>]
| |
[<title>] [<h1>] [<p>] [<div>]
| |
[Text] [<button>]
Each node in the tree represents an element on your webpage:
The root node is the
document
.The HTML element splits into two main branches:
<head>
(for metadata) and<body>
(for visible content).Inside
<body>
, you can have multiple children like<h1>
,<p>
,<div>
, and more, each representing different parts of the page.
Every part of your webpage, whether it’s text, buttons, or images, can be accessed and controlled via the DOM.
Basic DOM Manipulation Techniques
Now that we understand the structure and purpose of the DOM, let’s explore how to manipulate it using JavaScript. Manipulating the DOM means changing the structure, content, or style of the webpage after it has been loaded.
Step 1: Selecting Elements
The first step in manipulating the DOM is selecting the element you want to change. There are several ways to do this, depending on what you're targeting.
Example 1: Selecting an Element by ID
The most straightforward way to select an element is by using its id
:
let element = document.getElementById("myElement");
Explanation:
- Here,
getElementById("myElement")
returns the element with theid
ofmyElement
.
Example 2: Selecting Multiple Elements by Class
If you want to select multiple elements (like all buttons on a page), you can use getElementsByClassName()
:
let buttons = document.getElementsByClassName("btn");
Explanation:
getElementsByClassName("btn")
returns an array-like collection of all elements with the classbtn
.
Example 3: Selecting Elements with querySelector
You can also use CSS selectors to select elements with querySelector()
or querySelectorAll()
:
let firstButton = document.querySelector(".btn");
let allButtons = document.querySelectorAll(".btn");
Explanation:
querySelector()
selects the first matching element, whilequerySelectorAll()
selects all matching elements.
Step 2: Manipulating Elements
Once you’ve selected the element, you can manipulate its properties or modify its content.
Example: Changing the Text of an Element
let heading = document.getElementById("heading");
heading.innerHTML = "Welcome to the City Builder!";
Explanation:
innerHTML
allows you to change the content of an element. In this case, we changed the text inside theheading
element.
Example: Changing the Style of an Element
let building = document.getElementById("building");
building.style.backgroundColor = "blue";
building.style.width = "200px";
Explanation:
- The
style
property allows you to dynamically change the CSS styles of an element. Here, we changed thebackgroundColor
andwidth
of thebuilding
element.
Adding and Removing Elements
The DOM is dynamic, meaning you can add, remove, or even clone elements as the page is running. This allows you to build highly interactive web pages that respond to user input.
Example: Adding a New Element
let newBuilding = document.createElement("div");
newBuilding.className = "building";
newBuilding.innerHTML = "<p>New Office</p>";
document.body.appendChild(newBuilding);
Explanation:
createElement()
creates a newdiv
element.className
sets the class of the new element.innerHTML
inserts content inside the new element.appendChild()
adds the newly created element to the end of the<body>
.
Example: Removing an Element
let building = document.getElementById("building");
building.remove();
Explanation:
- The
remove()
method allows you to delete an element from the DOM.
Building the Foundations of DOM Manipulation
In this part, we’ve explored the basic structure of the DOM and learned how to manipulate elements by selecting, modifying, adding, and removing them using JavaScript. By thinking of the DOM as a dynamic blueprint, you now have the foundational skills to start building and modifying your webpage like a city builder.
In the next part, we’ll dive deeper into more advanced DOM manipulation techniques, event listeners, and how to create interactive web experiences.
Interacting with the DOM — Event Listeners
A powerful feature of the DOM is its ability to respond to user actions. Event listeners allow you to set up "listening stations" that detect when a user interacts with your webpage, such as clicking a button, hovering over an element, or typing in a text field. When an event occurs, you can execute a function to handle that interaction.
What are Event Listeners?
Event listeners listen for specific actions (events) on elements. These could be events like:
click: When a user clicks on an element.
mouseover: When the user hovers over an element.
input: When the user types into an input field.
keydown: When the user presses a key on the keyboard.
You attach an event listener to an element and provide a callback function that gets executed when the event occurs.
Example: Adding a Click Event Listener
Here’s how you can attach a click event listener to a button:
let button = document.getElementById("myButton");
button.addEventListener("click", function() {
alert("Button was clicked!");
});
Explanation:
We select the button using
getElementById("myButton")
.addEventListener()
is used to listen for the"click"
event. When the button is clicked, an alert box will display the message"Button was clicked!"
.
Event listeners are essential for creating interactive elements on your webpage. Let’s apply them to our city-building scenario.
Making the City Interactive — Adding Event Listeners
Now, let’s make our city more interactive by adding event listeners to the buildings. For example, when a building is clicked, we can change its color, and when hovered over, we can display additional information.
Example: Changing Building Colors on Click
Let’s make it so that clicking on a building changes its color to yellow:
let buildings = document.getElementsByClassName("building");
for (let i = 0; i < buildings.length; i++) {
buildings[i].addEventListener("click", function() {
this.style.backgroundColor = "yellow";
});
}
Explanation:
We select all buildings using
getElementsByClassName("building")
.For each building, we add a click event listener that changes its background color to yellow.
The keyword
this
refers to the building that was clicked.
Example: Displaying Information on Hover
We can also add a mouseover event listener to display information about a building when the user hovers over it:
let infoBox = document.getElementById("infoBox");
for (let i = 0; i < buildings.length; i++) {
buildings[i].addEventListener("mouseover", function() {
infoBox.innerHTML = "This is building number " + (i + 1);
});
}
Explanation:
We create an info box (
infoBox
) to display the building’s information.When the user hovers over a building, the
mouseover
event triggers a function that updates the text inside theinfoBox
to reflect which building the user is hovering over.
By using event listeners, you can create more dynamic and interactive elements on your webpage, giving users a richer experience.
Dynamically Adding and Removing Buildings
In many interactive applications, you’ll need to add or remove elements dynamically based on user input. Let’s expand on our city-building example by allowing users to add new buildings and remove existing ones.
Example: Adding a New Building
We can allow users to click a button that adds a new building to the city:
<button id="addBuildingBtn">Add Building</button>
<script>
let city = document.getElementById("city");
document.getElementById("addBuildingBtn").addEventListener("click", function() {
let newBuilding = document.createElement("div");
newBuilding.className = "building";
newBuilding.style.width = "100px";
newBuilding.style.height = "100px";
newBuilding.style.backgroundColor = "gray";
city.appendChild(newBuilding);
});
</script>
Explanation:
We create a button with the
id
ofaddBuildingBtn
that, when clicked, triggers a function to create a new building.Inside the function,
createElement()
is used to create a new<div>
representing the building. We style it and add it to the city by appending it to the city container usingappendChild()
.
Example: Removing a Building
You can also create functionality to remove buildings from the city:
<button id="removeBuildingBtn">Remove Last Building</button>
<script>
document.getElementById("removeBuildingBtn").addEventListener("click", function() {
let buildings = document.getElementsByClassName("building");
if (buildings.length > 0) {
let lastBuilding = buildings[buildings.length - 1];
lastBuilding.remove();
}
});
</script>
Explanation:
The button with
id="removeBuildingBtn"
is attached to a click event listener that removes the last building added to the city.The
remove()
method deletes the last element from the DOM.
Challenge Time - Build Your Interactive City Scene
Now that you have a solid understanding of DOM manipulation and event listeners, it’s time to build your Interactive City Scene.
Challenge Instructions:
Add event listeners to buildings, allowing users to click on them to change their color or display information.
Create dynamic controls to add new buildings or remove buildings.
Allow for additional user interactions, such as resizing buildings or changing their content on click.
Here’s a basic structure to get you started:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Interactive City</title>
<style>
.building {
width: 100px;
height: 100px;
background-color: gray;
margin: 10px;
}
</style>
</head>
<body>
<div id="city"></div>
<button id="addBuildingBtn">Add Building</button>
<button id="removeBuildingBtn">Remove Last Building</button>
<script>
let city = document.getElementById("city");
// Add building functionality
document.getElementById("addBuildingBtn").addEventListener("click", function() {
let newBuilding = document.createElement("div");
newBuilding.className = "building";
city.appendChild(newBuilding);
});
// Remove building functionality
document.getElementById("removeBuildingBtn").addEventListener("click", function() {
let buildings = document.getElementsByClassName("building");
if (buildings.length > 0) {
let lastBuilding = buildings[buildings.length - 1];
lastBuilding.remove();
}
});
</script>
</body>
</html>
Mastering DOM Manipulation and Interactivity
By now, you should have a strong understanding of how the Document Object Model (DOM) works and how you can manipulate it to create interactive web experiences. From selecting and modifying elements to adding event listeners and dynamically updating the page, DOM manipulation is one of the most powerful features of JavaScript.
Key Takeaways:
Event listeners allow your webpage to respond to user interactions like clicks, hovers, and keypresses.
Dynamic DOM manipulation allows you to add, remove, or update elements in response to user input.
By combining DOM manipulation with event listeners, you can create fully interactive, engaging web applications.
Join me for DOM Methods in-depth!
In the next article, we’ll dive even deeper into the DOM and explore more advanced DOM methods for traversing, manipulating, and optimizing the structure of your webpage. Get ready to master the full power of DOM manipulation in JavaScript!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by