Made a Spotify playlist using Terraform

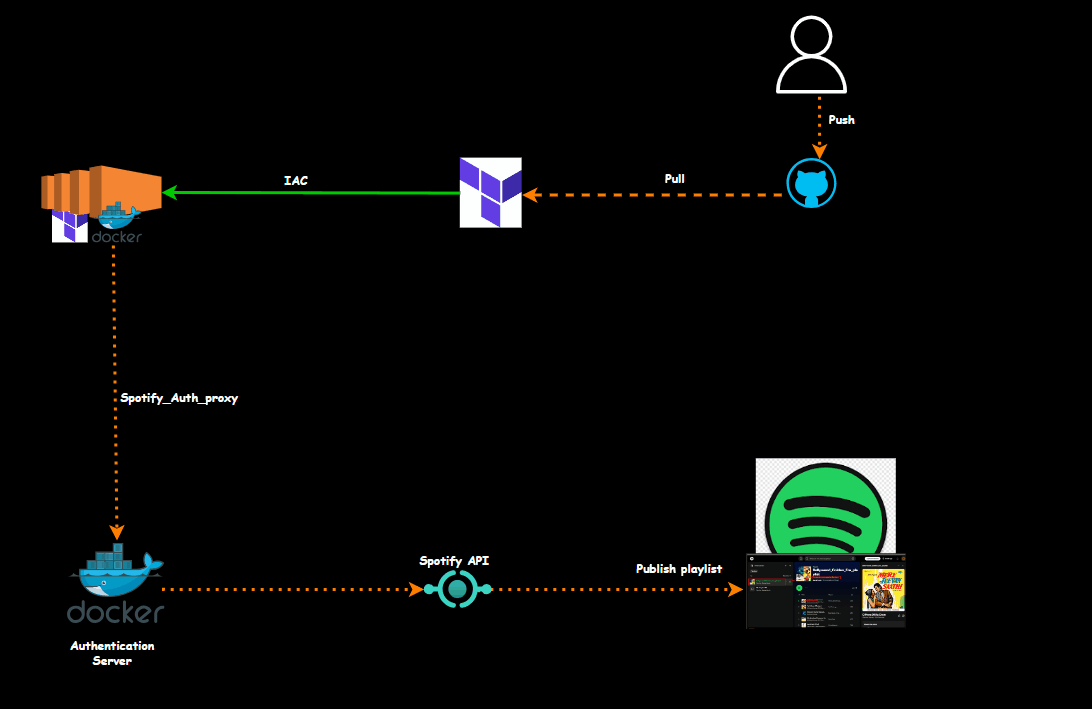
Hey everyone! I want to share a fun project I recently tackled: creating a Spotify playlist using Terraform. As an Infrastructure as Code (IaC) tool, Terraform typically handles cloud infrastructure tasks, but its flexibility allows for a lot of creative applications. So, why not use it to manage a Spotify playlist?
In this post, I’ll walk through the setup, challenges, and steps to automate the process of adding songs to a playlist using Terraform.
Prerequisites: To follow along, you’ll need:
A Spotify Developer Account with access to the API.
The Spotify provider for Terraform.
Terraform installed on your system.
Basic familiarity with Spotify's API and Terraform configurations.
Step 1: Set Up Spotify API Credentials
Create an App: Head to your Spotify Developer Dashboard and create a new app. Spotify will provide you with a
Client ID
andClient Secret
—keep these handy.Configure Permissions: You’ll need
playlist-modify-public
andplaylist-modify-private
permissions to create and manage playlists.Get Access Tokens: Use your credentials to request an access token. I used Postman for testing, but there are other tools as well. This token will enable Terraform to authenticate with Spotify’s API.
Step 2: Initialize the Spotify Provider in Terraform
Now, let’s configure Terraform to connect with the Spotify API.
provider "spotify" {
api_key = "YOUR API KEY"
}
With this setup, Terraform is ready to interact with Spotify.
Step 3: Create a Playlist
This code snippet will create a new playlist.
resource coderesource "spotify_playlist" "my_playlist" {
name = "Terraform Tunes"
tracks = ["track-1-URI","Track-2-URI"]
description = "A playlist created with Terraform"
public = true
}
After running terraform apply
, a new playlist named “Terraform Tunes” should appear in your Spotify account!
Step 4: Add Songs to Your Playlist
Let’s add some tracks! The Spotify provider accepts URIs for tracks, so you’ll need to grab these from Spotify.
Simply run terraform apply
again, and Terraform will add these songs to your playlist.
Step 5: Automate Updates
Terraform’s declarative nature allows us to maintain and update playlists as code. Need to add new tracks? Just update the list and reapply. Need to remove some? Remove the URIs, and Terraform will sync the playlist accordingly.
Challenges and Tips
API Rate Limits: Be mindful of Spotify’s API limits.
Token Expiry: Implementing a refresh token mechanism helps keep the playlist updates seamless.
Final Thoughts
Using Terraform for managing something as unconventional as a Spotify playlist was an eye-opener for me. It shows how flexible IaC tools can be beyond the traditional infrastructure domain. Whether you’re a Terraform enthusiast or a Spotify superfan, this project is a great way to blend both interests!
Feel free to ask questions or share your experiences in the comments. Happy playlisting!
Subscribe to my newsletter
Read articles from Parth Sikka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
