Understanding Code Smells in PHP and Laravel: A Beginner's Guide

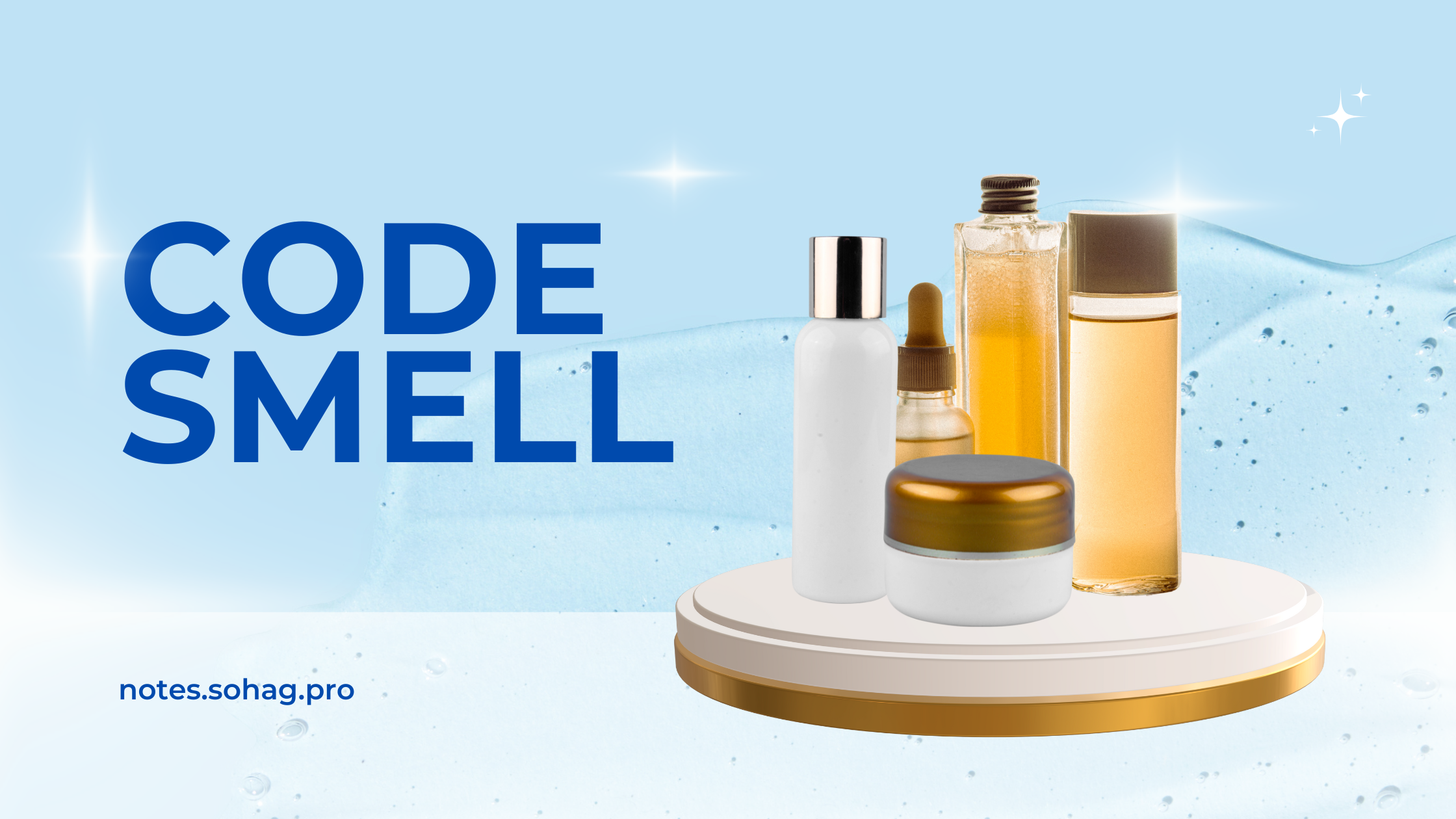
Code Smells in PHP and Laravel
Ever walked into a room and immediately noticed something was off? Maybe it was a faint burning smell from the kitchen or the sound of a washing machine that didn't quite seem right. These warning signs in our daily lives are similar to what developers call "code smells" in programming—hints that something in our code needs attention.
What Are Code Smells?
Code smells are warning signs in our code that suggest deeper problems. They're not bugs—the code might work perfectly fine—but they indicate areas where your code could be cleaner, more efficient, or easier to maintain.
Think of it like a cluttered garage. Everything works and you can still park your car, but finding your tools takes longer than it should, and adding new items becomes increasingly difficult. That's exactly how code with "smells" feels to work with.
Common Code Smells in PHP and Laravel (And How to Fix Them)
1. The Long Method Smell
This is like trying to read an instruction manual with no chapters or sections—it's overwhelming and hard to follow.
Before (Smelly Code):
public function processOrder($orderId)
{
$order = Order::find($orderId);
// Validate order
if (!$order) {
throw new Exception('Order not found');
}
// Check inventory
foreach ($order->items as $item) {
$product = Product::find($item->product_id);
if ($product->stock < $item->quantity) {
throw new Exception('Insufficient stock');
}
}
// Update inventory
foreach ($order->items as $item) {
$product = Product::find($item->product_id);
$product->stock -= $item->quantity;
$product->save();
}
// Process payment
$payment = new Payment();
$payment->amount = $order->total;
$payment->order_id = $order->id;
$payment->process();
// Send email
Mail::to($order->user->email)->send(new OrderConfirmation($order));
// Update order status
$order->status = 'processed';
$order->save();
}
After (Clean Code):
public function processOrder($orderId)
{
$order = $this->findOrder($orderId);
$this->validateInventory($order);
$this->updateInventory($order);
$this->processPayment($order);
$this->sendConfirmation($order);
$this->updateOrderStatus($order);
}
private function findOrder($orderId)
{
$order = Order::findOrFail($orderId);
return $order;
}
private function validateInventory(Order $order)
{
foreach ($order->items as $item) {
if ($item->product->stock < $item->quantity) {
throw new Exception('Insufficient stock');
}
}
}
// Additional methods...
2. The Duplicate Code Smell
Imagine having the same house key copied five times—if you need to change the lock, you'll have to replace all five keys. That's the problem with code duplication.
Before (Smelly Code):
public function calculateTotalPrice($items)
{
$total = 0;
foreach ($items as $item) {
$price = $item->price;
$tax = $price * 0.2;
$shipping = $price > 100 ? 0 : 10;
$total += $price + $tax + $shipping;
}
return $total;
}
public function calculateDiscountedPrice($items)
{
$total = 0;
foreach ($items as $item) {
$price = $item->price;
$tax = $price * 0.2;
$shipping = $price > 100 ? 0 : 10;
$discount = $price * 0.1;
$total += $price + $tax + $shipping - $discount;
}
return $total;
}
After (Clean Code):
private function calculateBasePrice($item)
{
$price = $item->price;
$tax = $price * 0.2;
$shipping = $price > 100 ? 0 : 10;
return $price + $tax + $shipping;
}
public function calculateTotalPrice($items)
{
return collect($items)->sum(function ($item) {
return $this->calculateBasePrice($item);
});
}
public function calculateDiscountedPrice($items)
{
return collect($items)->sum(function ($item) {
$basePrice = $this->calculateBasePrice($item);
return $basePrice - ($item->price * 0.1);
});
}
3. The God Object Smell
This is like having one kitchen drawer that holds everything from utensils to receipts to batteries. In Laravel, it often manifests as a model that knows too much and does too much.
Before (Smelly Code):
class User extends Model
{
public function processOrder($items)
{
// Order processing logic
}
public function calculateTaxes()
{
// Tax calculation logic
}
public function sendWelcomeEmail()
{
// Email logic
}
public function generateInvoice()
{
// Invoice generation logic
}
public function updateShippingAddress($address)
{
// Address update logic
}
}
After (Clean Code):
class User extends Model
{
public function orders()
{
return $this->hasMany(Order::class);
}
}
class OrderProcessor
{
public function process(User $user, array $items)
{
// Order processing logic
}
}
class TaxCalculator
{
public function calculate(User $user)
{
// Tax calculation logic
}
}
class UserMailer
{
public function sendWelcome(User $user)
{
// Email logic
}
}
Why It Matters
Addressing code smells isn't just about being pedantic—it has real, practical benefits:
Reduced Bug Risk: Cleaner code means fewer hiding places for bugs. When each piece of code has a single, clear responsibility, problems are easier to spot and fix.
Easier Maintenance: Think of clean code like a well-organized toolbox. When you need to fix something, you know exactly where to look.
Better Team Collaboration: New team members can understand and work with clean code more quickly, reducing onboarding time and friction.
Lower Technical Debt: By addressing code smells early, you prevent small issues from snowballing into major refactoring projects.
Laravel-Specific Tips
Use Laravel's built-in tools to fight code smells:
Leverage Service Providers for dependency injection
Use Form Requests for validation logic
Implement Jobs for complex processing
Utilize Events and Listeners for decoupling
Follow Laravel's conventions:
// Instead of this:
public function get_user_posts($user_id)
// Do this:
public function getUserPosts($userId)
Key Takeaways
Code smells are warning signs, not errors. They indicate areas where your code could be improved.
Regular refactoring is like regular house cleaning—it's easier to maintain cleanliness than to deal with accumulated mess.
Use Laravel's built-in features and conventions to write cleaner code from the start.
When in doubt, follow the Single Responsibility Principle: each class and method should do one thing and do it well.
Getting Started
Begin your code cleanup journey by:
Reviewing one piece of code at a time
Looking for the smells we've discussed
Making small, incremental improvements
Running tests after each change
Committing improvements regularly
Remember, perfect code doesn't exist, but better code always does. Start small, be consistent, and gradually build better coding habits. Your future self (and your teammates) will thank you!
Subscribe to my newsletter
Read articles from Sohag Hasan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sohag Hasan
Sohag Hasan
WhoAmI => notes.sohag.pro/author