Unlocking React's Potential: Why Data Mutability Might Be Breaking Your App
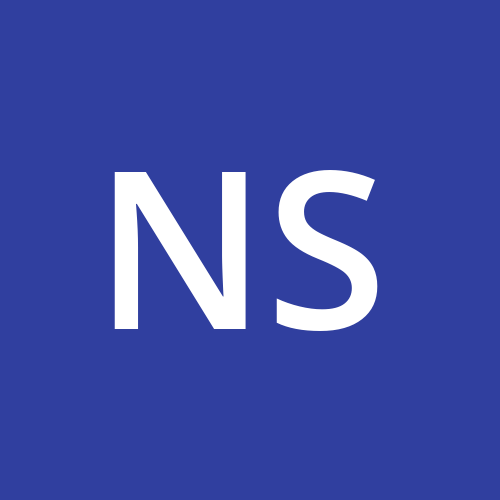
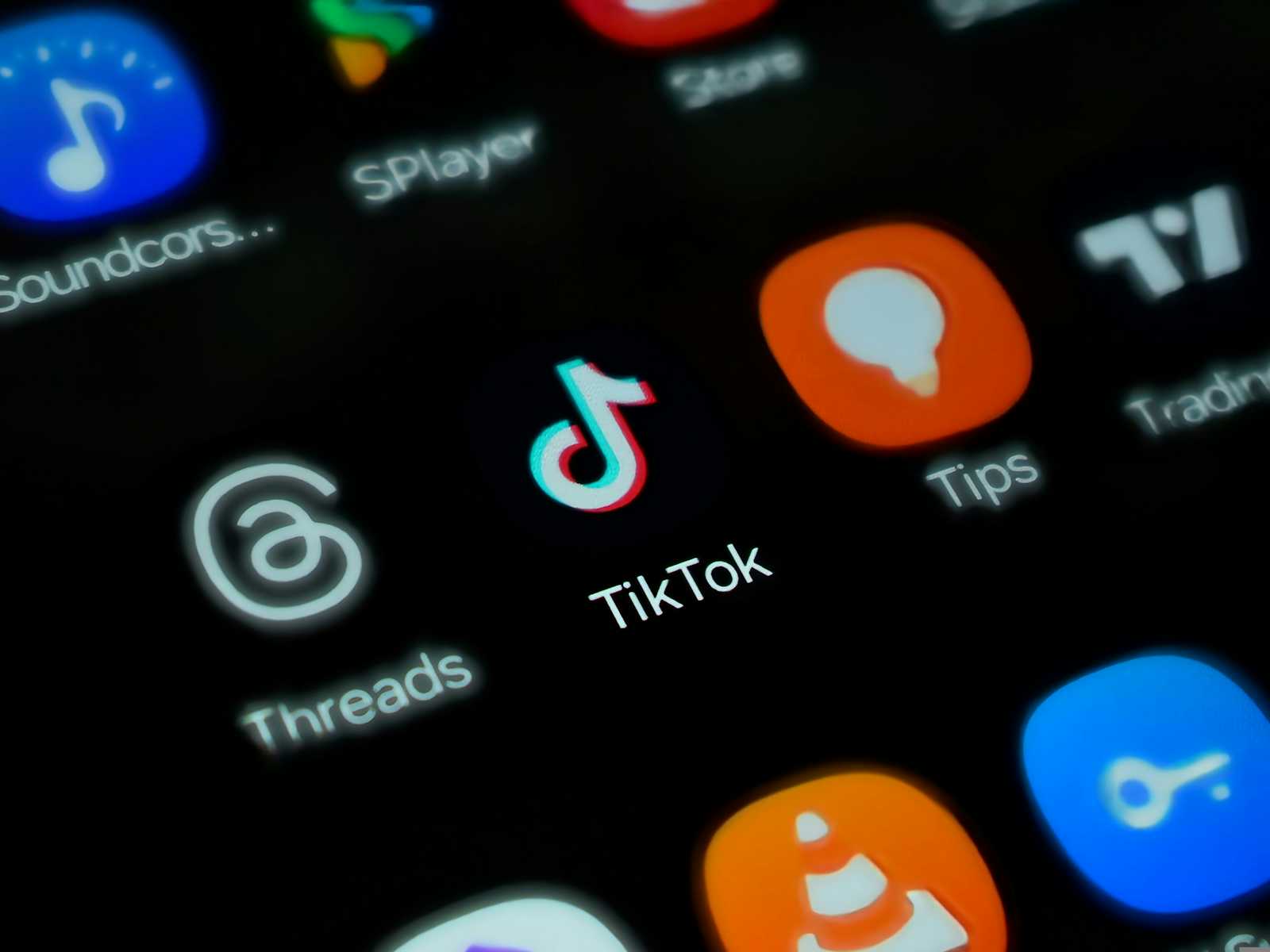
Last week, while working on some legacy React code, I stumbled upon a tricky bug: one component just wouldn’t re-render as expected. After a deep dive, I discovered the culprit—data mutation. The component wasn’t re-rendering because its state was being modified directly, preventing React from detecting the change. Here’s why this happens and how to avoid it.
How React Re-Rendering Works
React’s rendering relies heavily on immutability. When you update a component’s state, React compares the old and new state references. If the references are the same, React assumes nothing changed and skips the re-render. This is why mutating data directly doesn’t work.
For instance, say you have this code to add a new item to an array in state:
items.push(newItem); // Mutating existing array
setItems(items); // Same reference, so React doesn’t re-render
This fails because items
retains the same reference.
What is Mutable vs. Immutable Data?
At its core, mutable data can be modified directly. For example, if you have an array or object, you can add or change elements within it without creating a new instance.
Think of mutable data like editing a note on a sticky pad. When you cross out a word or add something new, you’re changing that same sticky note. It’s convenient, but sometimes others might not realize you've changed the note, which can cause confusion.
Immutable data, on the other hand, is like writing a fresh sticky note each time you make a change. You keep the old version, but the new note reflects the updates. This makes it easier to track changes and avoids accidental confusion because every change creates a clear, updated version.
In React, this approach of creating “new notes” (or new data) helps the app run more smoothly. React notices these changes right away, so your app stays fast and reliable.
Mutable Data Example
In this example, we directly modify an array inside the component state, which can lead to unexpected behavior because React may not detect the change.
import React, { useState } from 'react';
function MutableExample() {
const [items, setItems] = useState(['Item 1', 'Item 2']);
const addItem = () => {
// Mutating the array directly
items.push(`Item ${items.length + 1}`);
setItems(items); // React may not recognize this as a change
};
return (
<div>
<h3>Mutable Example</h3>
<button onClick={addItem}>Add Item</button>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
}
export default MutableExample;
Here, items.push()
modifies the existing array directly, and since React’s state relies on detecting changes by reference, this may not trigger a re-render. As a result, the UI may not update as expected.
Avoiding Mutation with Immutability
Instead, use immutability to ensure a new reference for each state update. By creating a new array or object, React recognizes a change and re-renders the component:
setItems([...items, newItem]); // New array, so React re-renders
This approach prevents unexpected bugs, ensuring consistent UI updates. Here’s another example with nested data where you’d want to avoid mutation:
// Incorrect: Directly modifying nested state
user.address.city = "New York";
setUser(user);
// Correct: Creating a new object for updated state
setUser({
...user,
address: {
...user.address,
city: "New York",
},
});
Caution: Why Mutation Causes Issues
Mutating data directly can cause various issues, from UI inconsistencies to performance problems. React is optimized for immutability, so following these principles helps maintain predictable and performant applications.
Key Takeaways
Don’t mutate state directly; use new objects or arrays.
Use spread operators or libraries like Immer to make complex updates easier and maintain immutability.
By embracing immutability, you ensure React recognizes every change and delivers a smoother, more reliable user experience.
Subscribe to my newsletter
Read articles from Nitesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
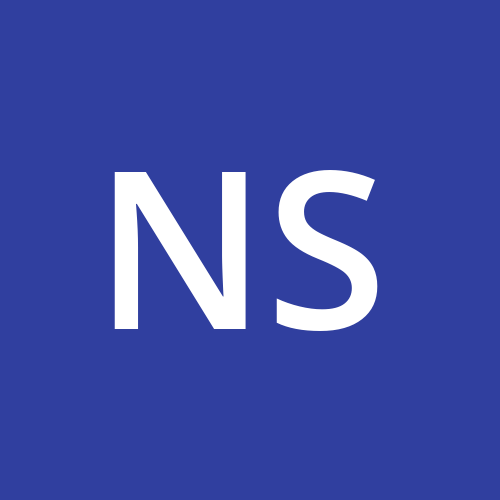
Nitesh Singh
Nitesh Singh
M365 Full Stack Developer - Experience in Azure, SharePoint SPFX, .NET, and React Full Stack Development, Driving Innovation and Digital Transformation Across Diverse Industries