How to Use Python for Easy Image Transformation and Person Replacement
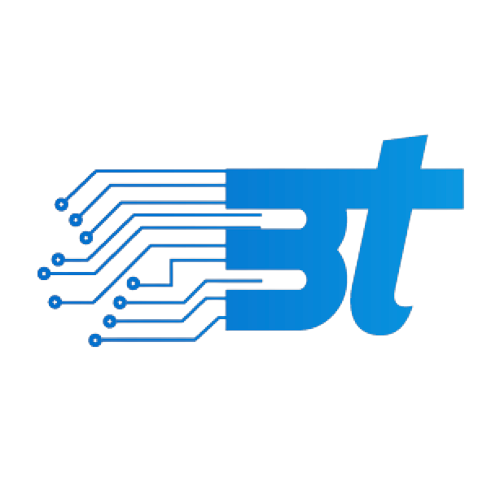
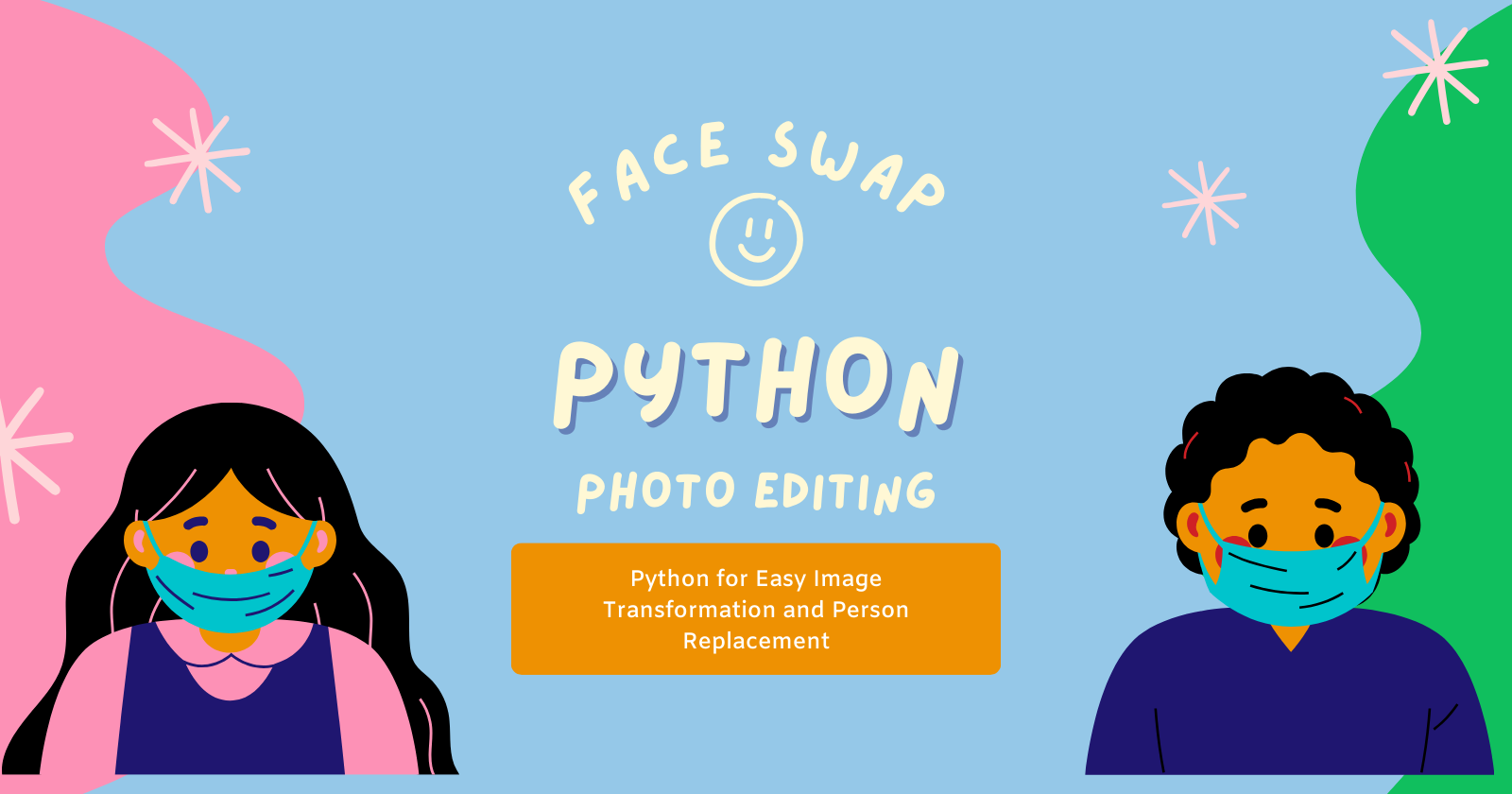
Replacing a person in an image with another image using Python is a common technique in photo editing, AI, and computer vision projects. This process usually involves detecting the subject in the image, removing or masking it, and then inserting the replacement image with proper blending and alignment. Let's dive into a simple guide on how to accomplish this with Python.
1. Setting Up the Environment
Before we begin, we need to install some essential libraries. We'll use:
OpenCV: for handling image processing.
NumPy: for numerical operations.
Mediapipe or YOLO: for detecting faces or bodies in the image.
You can install these libraries with the following commands:
pip install opencv-python mediapipe numpy
2. Detecting and Masking the Person in the Image
To replace a person in an image, we need to locate their position. We can use the Mediapipe library's face or pose detection for this.
Here’s a basic example using Mediapipe to detect the face or body in an image and create a mask:
import cv2
import mediapipe as mp
import numpy as np
# Load the image
img = cv2.imread("original_image.jpg")
# Initialize Mediapipe Face Detection
mp_face_detection = mp.solutions.face_detection
face_detection = mp_face_detection.FaceDetection(model_selection=1, min_detection_confidence=0.5)
# Convert the image to RGB
rgb_img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
results = face_detection.process(rgb_img)
# Create a mask of the detected area
mask = np.zeros(img.shape[:2], dtype=np.uint8)
if results.detections:
for detection in results.detections:
bbox = detection.location_data.relative_bounding_box
h, w, _ = img.shape
x, y, box_w, box_h = (int(bbox.xmin * w), int(bbox.ymin * h),
int(bbox.width * w), int(bbox.height * h))
# Draw the mask for the face
mask[y:y+box_h, x:x+box_w] = 255
# Invert mask to keep only background
inverse_mask = cv2.bitwise_not(mask)
background = cv2.bitwise_and(img, img, mask=inverse_mask)
Here, mask
isolates the detected face or person area. inverse_mask
will help in removing this area from the main image.
3. Inserting the Replacement Image
Now that we have isolated the area to replace, the next step is to insert the new image or person in this area. Load the new image, resize it to match the detected area, and overlay it onto the masked region.
# Load and resize the replacement image
replacement_img = cv2.imread("replacement_image.jpg")
replacement_img = cv2.resize(replacement_img, (box_w, box_h))
# Position the replacement image
positioned_replacement = background.copy()
positioned_replacement[y:y+box_h, x:x+box_w] = replacement_img
4. Blending and Fine-Tuning
To make the replacement seamless, blend the replacement image with the original image's edges. Use Gaussian Blur or OpenCV's seamlessClone function to blend the edges.
# Blend the images
center = (x + box_w // 2, y + box_h // 2)
output = cv2.seamlessClone(replacement_img, img, mask, center, cv2.NORMAL_CLONE)
This will blend the new image in place of the original person, creating a smoother look.
5. Saving the Final Image
Finally, save the resulting image:
cv2.imwrite("output_image.jpg", output)
Complete Code
Here’s the complete code to replace a person in an image:
import cv2
import mediapipe as mp
import numpy as np
# Load the original image
img = cv2.imread("original_image.jpg")
# Initialize Mediapipe Face Detection
mp_face_detection = mp.solutions.face_detection
face_detection = mp_face_detection.FaceDetection(model_selection=1, min_detection_confidence=0.5)
# Convert to RGB and process the face detection
rgb_img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
results = face_detection.process(rgb_img)
# Create a mask for the detected area
mask = np.zeros(img.shape[:2], dtype=np.uint8)
if results.detections:
for detection in results.detections:
bbox = detection.location_data.relative_bounding_box
h, w, _ = img.shape
x, y, box_w, box_h = (int(bbox.xmin * w), int(bbox.ymin * h),
int(bbox.width * w), int(bbox.height * h))
mask[y:y+box_h, x:x+box_w] = 255 # Apply mask on detected area
# Load and resize the replacement image
replacement_img = cv2.imread("replacement_image.jpg")
replacement_img = cv2.resize(replacement_img, (box_w, box_h))
# Place replacement image on masked area
positioned_replacement = cv2.bitwise_and(img, img, mask=cv2.bitwise_not(mask))
positioned_replacement[y:y+box_h, x:x+box_w] = replacement_img
# Blend using seamlessClone for smooth transition
center = (x + box_w // 2, y + box_h // 2)
output = cv2.seamlessClone(replacement_img, img, mask, center, cv2.NORMAL_CLONE)
# Save the final result
cv2.imwrite("output_image.jpg", output)
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
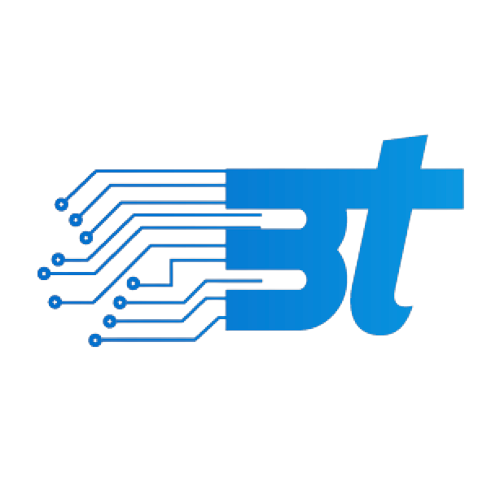
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.