Automate EC2 Instance Stop/Start on Weekends Using AWS Lambda EventBridge and SNS

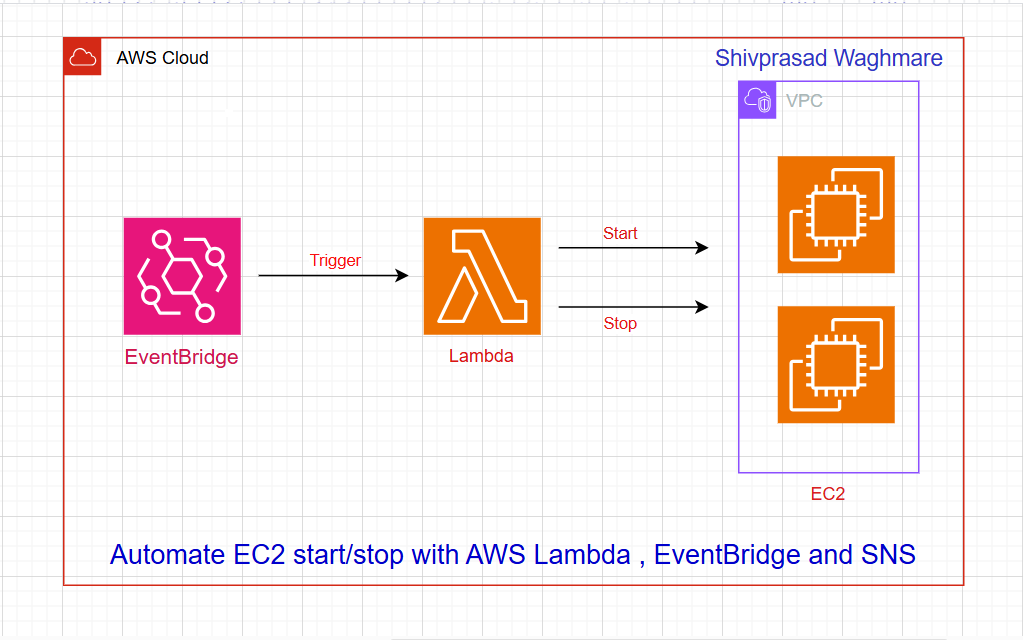
Introduction
In this tutorial, we will learn how to set up automatic scheduling to start EC2 instances on Monday mornings and stop them on Friday evenings. This will save costs by ensuring that the EC2 instances in lower environments (like development and staging) are only running during weekdays and SNS will notify through email.
Step 1: Create an SNS Topic
Go to the SNS Console:
Navigate to the SNS Console.
In the left-hand panel, click Topics and then click Create Topic.
Create the Topic:
Choose the Standard type.
Provide a name for the topic (e.g.,
EC2StartStopNotifications
).Click Create Topic.
Subscribe to the Topic:
After creating the topic, you’ll be directed to the topic details page.
Under the Subscriptions section, click Create subscription.
Copy paste the ARN (SNS topic you just created) and Choose Protocol (Email) and provide email address as a endpoint.
Click Create Subscription.
Confirm Subscription (for Email):
An Email will be sent to the provided address with a confirmation link.
Click the Confirm subscription link in your email.
Step 2: Create IAM Role for Lambda and SNS
Go to IAM Console:
Navigate to the IAM Console.
Select Roles in the left panel and click Create Role.
Select Trusted Entity:
- Choose Lambda as the trusted entity.
Attach Policies:
Attach the following policies to the role:
AmazonEC2FullAccess
(to allow the Lambda function to start and stop EC2 instances).AWSLambdaBasicExecutionRole
(for Lambda to write logs to CloudWatch).AmazonSNSFullAccess
(to send notifications)
Name and Create Role:
Name the role (
LambdaEC2StartStopRole
) and click Create Role.
Step 3: Configure 2 EC2 instances -
Configure 2 EC2 instances if not already created.
Step 4: Write the Lambda Function
Go to AWS Lambda and click Create function.
Choose Author from scratch, name it
EC2StartStopScheduler
, and select Python 3.11 as the runtime.Under Execution role, choose the role you created (
Lambda-EC2StartStopRole
).In the Function code section, copy and paste the following code:
import boto3 import os ec2 = boto3.client('ec2') sns = boto3.client('sns') def lambda_handler(event, context): action = event.get('action') instance_ids = os.environ['INSTANCE_IDS'].split(',') sns_topic_arn = os.environ['SNS_TOPIC_ARN'] try: if action == 'start': print(f"Starting instances: {instance_ids}") ec2.start_instances(InstanceIds=instance_ids) sns.publish( TopicArn=sns_topic_arn, Subject="EC2 Start Notification", Message=f"The following instances have been started: {', '.join(instance_ids)}" ) print("Instances started and notification sent.") return {"status": "started", "instances": instance_ids} elif action == 'stop': print(f"Stopping instances: {instance_ids}") ec2.stop_instances(InstanceIds=instance_ids) sns.publish( TopicArn=sns_topic_arn, Subject="EC2 Stop Notification", Message=f"The following instances have been stopped: {', '.join(instance_ids)}" ) print("Instances stopped and notification sent.") return {"status": "stopped", "instances": instance_ids} else: raise ValueError("Invalid action. Use 'start' or 'stop'.") except Exception as e: print(f"Error occurred: {e}") sns.publish( TopicArn=sns_topic_arn, Subject="EC2 Action Error", Message=f"An error occurred while processing EC2 instances: {str(e)}" ) raise e
Environment Variables: Add two environment variables:
INSTANCE_IDS: A comma-separated list of EC2 instance IDs
SNS_TOPIC_ARN: The ARN of your SNS topic from Step 1.
Change General Configuration → Timeout —> 1 min from 3 sec, so you won’t receive error while execution.
Test → Create test event and add payload as given
Save , Deploy and Test once the function, It should work, means either start or stop your instances.
Step 4: Set Up EventBridge Rules for Scheduling
Go to Amazon EventBridge and click Create rule.
Name it
EC2-stop-rule
.For Rule type, select Schedule and set it to:
Cron expression:
0 22 ? * FRI *
(This runs every Friday at 10:00 PM IST).
In the Target section:
Select Lambda function and choose
EC2StartStopScheduler
.
Select Lambda function and add Payload.
Create the schedule.
Create the Start Rule
Repeat the steps above to create another rule called
EC2-start-rule
Set the schedule:
Cron expression:
0 7 ? * MON *
(Runs every Monday at 7:00 AM IST).
Select Lambda function and add Payload..
Create the schedule.
Step 5: Test the Setup
To test, go to your Lambda function and configure a test event:
For stopping, create a test event with
{"action": "stop"}
.For starting, create a test event with
{"action": "start"}
.
Run each test, and check your email for SNS notifications to confirm the actions.
You must see an email like this @scheduled time!!!
Key Takeaways -
Cost Savings: Automating the process of starting and stopping EC2 instances during the weekend helps reduce costs for lower environments (dev, staging), as instances are only running during business hours.
Using Lambda for Automation: AWS Lambda is a cost-effective and scalable solution for automating infrastructure tasks such as starting and stopping EC2 instances.
SNS for Notifications: Integrating Amazon SNS with Lambda provides a simple way to send real-time notifications about your EC2 instances state changes (when they start or stop).
EventBridge for Scheduling: Amazon EventBridge (formerly CloudWatch Events) provides a robust and flexible way to schedule tasks like starting and stopping EC2 instances at specific times.
Scalability: The automation setup can be easily scaled for multiple instances and different environments by modifying Lambda code and EventBridge rules.
Reliability: Combining Lambda, SNS, and EventBridge ensures a reliable automation workflow for non-production environments, with minimal manual intervention required.
Subscribe to my newsletter
Read articles from Shivprasad Waghmare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shivprasad Waghmare
Shivprasad Waghmare
"Accidental AWS/DevOps Engineer | AWS Community Builder | 7+ Years of Experience | Exploring AWS, Kubernetes, Terraform, Docker, Jenkins | Passionate about automating cloud infrastructure | Let’s explore the world of DevOps together!"