Understanding Map and Set Objects in JavaScript
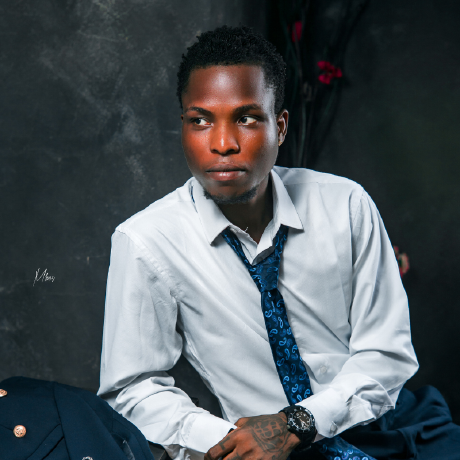
Have you ever felt overwhelmed by managing complex data structures in JavaScript? While arrays and objects are useful, they may not always be the most efficient solutions for your coding challenges. This is where Map and Set objects come into play—two powerful yet often overlooked features of modern JavaScript. 🚀
Understanding Map and Set objects can greatly enhance your ability to manage unique values, create key-value pairs with any data type, and improve your application's performance.
In this guide, we'll explore these versatile data structures, from basic operations to advanced implementations, and show you how they can streamline your code and solve common programming challenges.
Let's dive into the fundamentals of Map and Set objects, discover their unique capabilities, learn practical applications, and understand when to choose them over traditional arrays and objects.
Map Objects Fundamentals
The key differences between Maps and regular objects.
You'll find several crucial distinctions between Maps and regular JavaScript objects. Unlike objects, Maps allow any value as keys, including functions, objects, and primitives. Maps also maintain insertion order and provide built-in size tracking.
Feature | Map | Regular Object |
Key Types | Any value | Strings/Symbols only |
Order | Maintains insertion order | No guaranteed order |
Size | Built-in size property | Manual counting needed |
Iteration | Directly iterable | Requires Object methods |
Creating and initializing Map objects
You can create a Map using multiple approaches:
Empty Map:
new Map()
From an array of key-value pairs:
new Map([['key', 'value']])
From existing Map:
new Map(existingMap)
Basic Map methods for data manipulation
Your essential Map operations include:
set(key, value)
- Add or update entriesget(key)
- Retrieve valueshas(key)
- Check key existencedelete(key)
- Remove entriesclear()
- Remove all entries
Iterating through Map entries
You have several methods to iterate through Map data:
map.keys()
- Iterator for keysmap.values()
- Iterator for valuesmap.entries()
- Iterator for key-value pairsforEach()
- Loop through entries with callback
Now that you understand Map fundamentals, let's explore more advanced Map operations and their practical applications.
Advanced Map Operations
Chaining Map Methods
You can enhance your code efficiency by chaining multiple Map methods together. This technique allows you to perform several operations in a single line of code:
let userMap = new Map()
.set('name', 'John')
.set('age', 30)
.set('role', 'developer');
Converting Maps to Arrays and Objects
Transform your Maps into different data structures for enhanced flexibility:
Conversion Type | Method | Example |
Map to Array | Array.from() | Array.from(myMap) |
Map to Object | Object.fromEntries() | Object.fromEntries(myMap) |
Array to Map | new Map() | new Map([['key', 'value']]) |
Key conversion methods you can use:
Array.from()
for creating arrays of key-value pairsObject.fromEntries()
for converting to plain objectsmap.keys()
for extracting only the keysmap.values()
for getting just the values
Performance Benefits of Maps
Maps offer several performance advantages over regular objects:
Direct key-value lookups with O(1) complexity
Better memory usage for frequent additions/removals
Support for any type as keys (not just strings)
Built-in size tracking without manual calculation
Now that you understand advanced Map operations, let's explore Set objects and their unique characteristics.
Set Objects Explained
Unique Value Collections with Set
Set objects in JavaScript provide a powerful way to store unique values of any type. Unlike arrays, Sets automatically handle duplicate values by ignoring them, making your code more efficient and cleaner.
Creating and Populating Sets
You can create new Sets using multiple approaches:
// Empty Set
const mySet = new Set();
// Set from array
const numbersSet = new Set([1, 2, 3, 3]); // Contains 1, 2, 3
Essential Set Methods
Method | Description | Example |
add() | Adds a new value | mySet.add(value) |
delete() | Removes a value | mySet.delete(value) |
has() | Checks for value | mySet.has(value) |
clear() | Removes all values | mySet.clear() |
size | Returns set size | mySet.size |
Converting Arrays to Sets and Back
Transform between arrays and Sets seamlessly:
Array to Set:
new Set(array)
Set to Array:
Array.from(set)
or[...set]
Removing Duplicates Efficiently
Use Sets for the most efficient way to remove duplicates from arrays:
const arrayWithDuplicates = [1, 2, 2, 3, 3, 4];
const uniqueArray = [...new Set(arrayWithDuplicates)]; // [1, 2, 3, 4]
Now that you understand Sets and their fundamental operations, let's explore some practical applications where Sets truly shine in real-world scenarios.
Practical Applications
Using Maps for Data Caching
You can leverage Maps for efficient data caching in your JavaScript applications. Here's how to implement a simple cache system:
const dataCache = new Map();
function fetchData(key) {
if (dataCache.has(key)) {
return dataCache.get(key);
}
// Simulate data fetch
const data = expensiveOperation(key);
dataCache.set(key, data);
return data;
}
Set Operations in Real-World Scenarios
Sets excel at handling unique values in practical scenarios. Common applications include:
Removing duplicates from user input
Managing active user sessions
Tracking unique page visits
Implementing feature toggles
Maintaining unique tags or categories
Combining Maps and Sets Effectively
You can create powerful data structures by combining Maps and Sets. Here's a practical comparison of use cases:
Scenario | Implementation | Benefit |
User Preferences | Map<userId, Set> | Efficiently track unique preferences per user |
Event Tracking | Map<eventType, Set> | Manage unique event subscribers |
Cache Control | Map<resourceId, Set> | Track unique dependencies for cache invalidation |
For example, implementing a user preference system:
const userPreferences = new Map();
userPreferences.set('user123', new Set(['dark-mode', 'notifications-on']));
Now that you understand the practical applications, let's examine how these data structures perform in different scenarios.
Performance Considerations
Memory Usage Comparison
When working with data structures in JavaScript, memory consumption is crucial. Here's how Maps and Sets compare to traditional objects and arrays:
Data Structure | Memory Efficiency | Storage Type |
Map | High | Key-value pairs |
Set | Medium | Unique values |
Object | Low | Property-value pairs |
Array | Medium | Indexed values |
Operation Speed Benchmarks
You'll find significant performance differences in common operations:
Map operations:
get/set: O(1)
delete: O(1)
has: O(1)
Set operations:
add: O(1)
delete: O(1)
has: O(1)
Best Practices for Large Datasets
When handling large collections of data, consider these optimization techniques:
Pre-size your Maps and Sets when possible
Clear unused entries to free up memory
Use WeakMap for object references to prevent memory leaks
Batch operations instead of individual updates
When to Choose Maps and Sets
Choose Maps when you:
Need key-value pairs with any type of key
Frequently add/remove entries
Need to maintain insertion order
Want better performance for large datasets
Choose Sets when you:
Need to store unique values
Frequently check for value existence
Want automatic duplicate removal
Need fast lookup operations
Now that you understand the performance implications, you can make informed decisions about which data structure best suits your specific use case.
Mastering Map and Set objects opens up new possibilities for managing complex data structures in your JavaScript applications. These powerful built-in objects offer unique features that arrays and regular objects can't match - from maintaining insertion order and storing any type of key with Maps to automatically handling duplicate values with Sets.
As you continue developing your JavaScript skills, remember to choose the right data structure for your specific needs. Maps excel when you need key-value pairs with better performance and flexibility, while Sets are perfect for managing unique values in a collection. You'll write more efficient and maintainable applications by incorporating these modern JavaScript features into your code. Start experimenting with Maps and Sets in your next project to see their benefits firsthand.
Subscribe to my newsletter
Read articles from Stanley Owarieta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
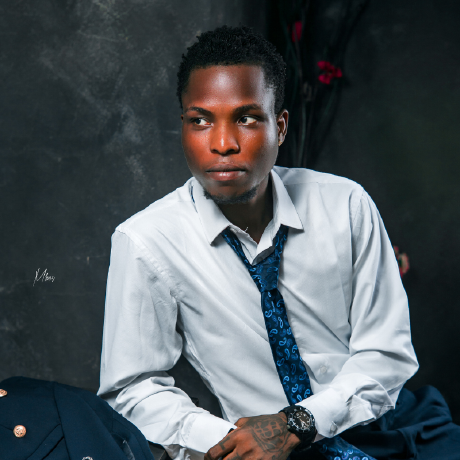
Stanley Owarieta
Stanley Owarieta
I help tech businesses and startups create engaging, SEO-driven content that attracts readers and converts them into customers. From in-depth blog posts to product reviews, I ensure every content is well-researched, easy to understand, and impactful. As a programming learner with HTML, CSS, and JavaScript knowledge, I bring a unique technical perspective to my writing. If you're looking for content that ranks and resonates, let's connect! 📩 Open to collaborations! Message me or email me at freelance@stanleyowarieta.com