Understanding Redux Architecture: The Story of State, Actions, and Reducers!

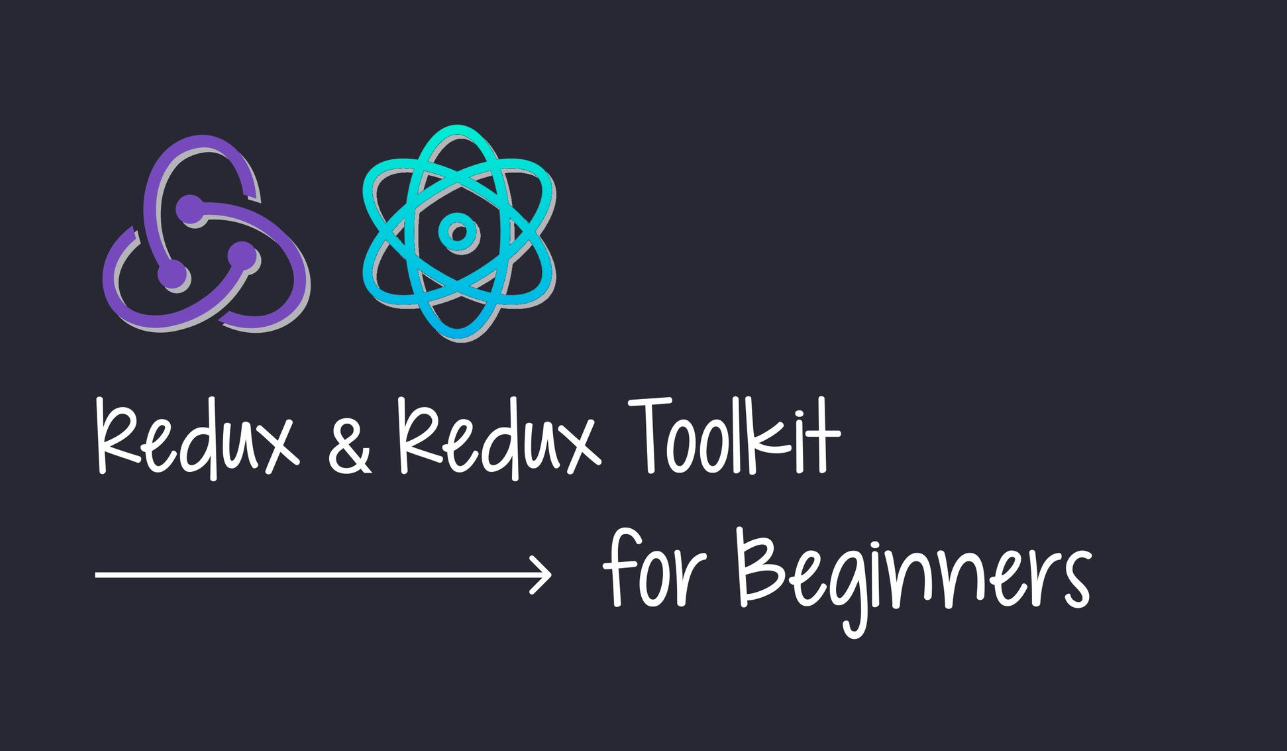
Hello, fellow coders! Today, we’re diving into the magical world of Redux—the ultimate sidekick for managing state in your React apps. If you've ever been overwhelmed by passing props all over the place, Redux is here to save you from the chaos! 🦸♂️
But before we jump in, let’s clarify something:
Redux isn’t a library for state management, it's a library for global state management.
This means it’s the guy you call in when your app has lots of data to handle, and you’re tired of your components shouting at each other for updates. Ready to meet the crew? Let’s go!
1. The Store: The Warehouse for All State
Imagine a giant warehouse where all your app's important data lives. That’s the store in Redux! It’s like the brain of your application, holding all the states you might need and making sure nothing’s lost in translation.
import { configureStore } from '@reduxjs/toolkit';
const store = configureStore({
reducer: {
// Add your reducers here
},
});
This store
object will hold all the state, and whenever a component needs data, it can come here and grab it. Think of it as a library where all your components can borrow books 📚, but they always get the latest edition!
2. Actions: The Messengers
Actions in Redux are like friendly messengers. They describe what happened without saying how to update the state. For example, imagine you’re running a bakery, and someone wants to buy bread:
Action: "Customer wants bread"
Store’s response: "Alright, here’s a fresh loaf 🍞."
An action is simply a JavaScript object with a type
property (to say what happened) and an optional payload
property (for extra data). Here’s an action example:
const incrementAction = {
type: 'counter/increment',
};
You’ll notice the type
property is a string like 'counter/increment'
. It's the identity tag of the action—Redux uses this to know which action is happening.
3. Reducers: The Change Makers
Reducers are the “doers” in Redux. They decide how to update the state based on the action received. If actions are the "what," reducers are the "how."
In Redux terms, a reducer is just a function that takes two things: the current state and an action. It processes the action and returns a new version of the state.
Let’s create a reducer for our bakery example:
const counterReducer = (state = { value: 0 }, action) => {
switch (action.type) {
case 'counter/increment':
return { ...state, value: state.value + 1 };
case 'counter/decrement':
return { ...state, value: state.value - 1 };
default:
return state;
}
};
🥳 Fun Fact: Reducers are pure functions. They don’t have side effects—they take in state and action, do their thing, and return fresh, updated state without changing the original. No drama, no fuss, just pure results.
4. Dispatching: How Actions Get Sent to the Store
When you click a button in your React app, you’ll want to dispatch an action. Dispatching is like shouting at the store: “Hey, we got some action here!” The store then grabs the action, sends it to the reducer, and boom—state updates!
In code, dispatching looks something like this:
import { useDispatch } from 'react-redux';
const dispatch = useDispatch();
dispatch({ type: 'counter/increment' });
5. Selectors: The Store’s Search Engine
In Redux, selectors are like Google Search for your store. They let you grab the specific slice of state you need without taking everything. This keeps your components neat and makes your app faster. ⚡
import { useSelector } from 'react-redux';
const count = useSelector((state) => state.counter.value);
Putting It All Together: The Redux Flow 🔄
Here's a quick breakdown of the Redux flow:
Click a Button → dispatch an action (e.g.,
{ type: 'counter/increment' }
)Store catches the action and hands it to the reducer.
Reducer processes it and returns a new state.
Store saves the new state and notifies components.
Component re-renders with the updated data.
It’s like a relay race 🏃, passing the baton (action) through each part of the Redux architecture until the state is updated and displayed.
Redux in a Nutshell
Redux might sound complex at first, but once you get the flow, it’s smooth sailing. Just remember:
Store holds all data.
Actions describe what happened.
Reducers decide how to change the state.
Dispatch sends the action to the store.
Selectors fetch specific parts of the state.
And that’s it! With this foundation, you’re ready to rock Redux in your own projects.
Final Words 🎉
Redux might seem like a lot, but trust me, once you get the hang of it, it’s a game-changer. No more passing props through a million components, and no more spaghetti state! 🍝 So go on, give Redux a try, and remember: you’ve got this!
Subscribe to my newsletter
Read articles from Abhishek Raut directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Raut
Abhishek Raut
🔭 I’m currently working on Full Stack Project 👯 I’m looking to collaborate on Animated Websites 🌱 I’m currently learning Data Science 💬 Ask me about React GSAP ⚡ Fun fact I am Working on Project and learning tech stack used in That