Mastering React Performance: Essential Tips for Writing Optimized and High-Performance Code

Table of contents
- 1. Use React.memo for Component Memoization
- 2. Use useCallback and useMemo to Cache Values and Functions
- 3. Lazy Loading Components with React.lazy and Suspense
- 4. Use Efficient State Management
- 5. Code-Splitting with Dynamic Imports
- 6. Avoid Inline Functions and Objects in JSX
- 7. Optimize Conditional Rendering
- 8. Use Proper Key Attributes in Lists
- 9. Optimize Rendering with shouldComponentUpdate (Class Components) or React.PureComponent
- 10. Minimize Re-Renders with React Profiler
- 11. Avoid Unnecessary DOM Manipulations
- 12. Optimize CSS and Assets
- 13. Limit API Calls and Use SWR or React Query for Data Fetching
- 14. Use Concurrent Features in React 18
- Example of Optimized Code:
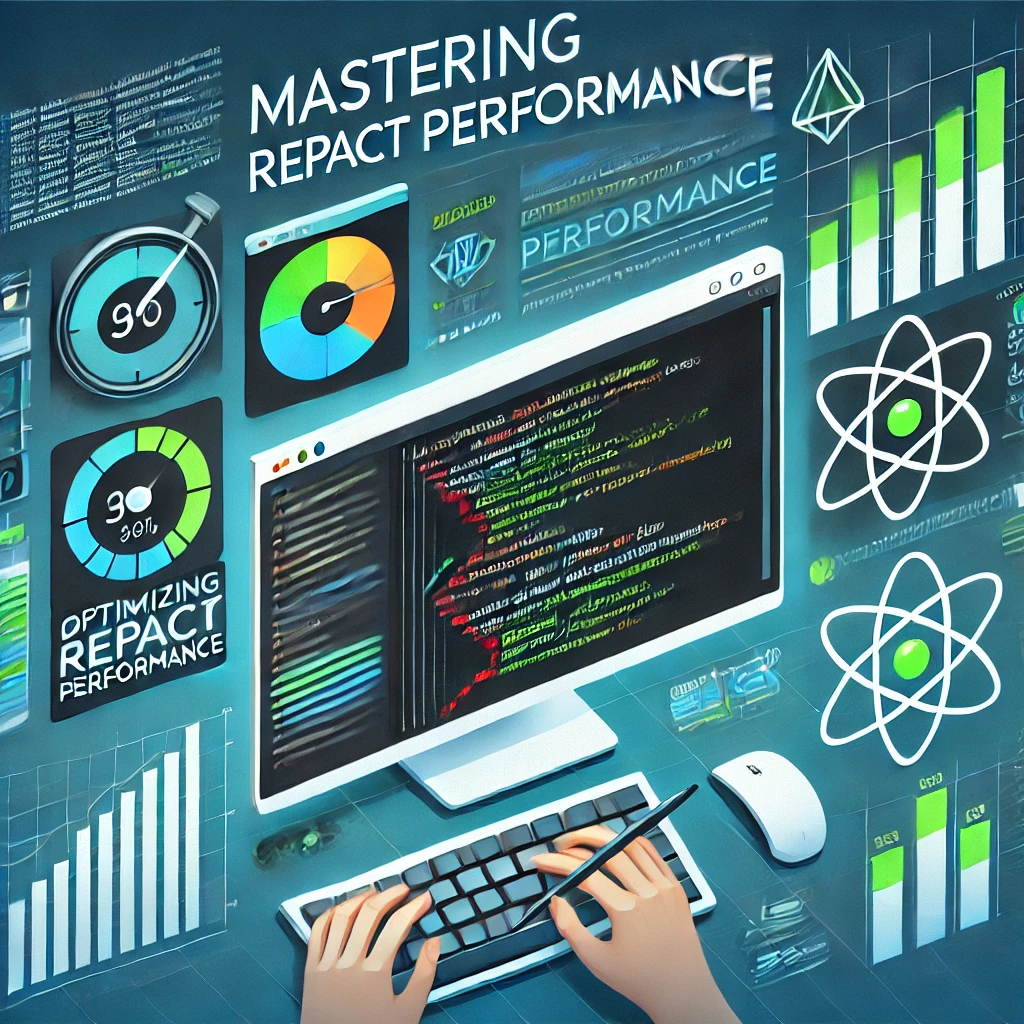
As a seasoned full-stack developer with a deep focus on creating high-performance, efficient applications, I've found that optimizing React code is key to delivering a smooth, responsive user experience. Whether you’re working on large-scale applications or single-page projects, React offers powerful features to streamline performance. In this article, I’ll guide you through some essential techniques for writing optimized, high-performance React code that not only improves user experience but also reduces load times and memory usage.
Writing optimized, high-performance React code involves several techniques that focus on reducing unnecessary renders, managing state effectively, and optimizing the way components interact. Here are some essential strategies:
1. Use React.memo
for Component Memoization
- Wrap functional components in
React.memo
to prevent re-renders when props haven't changed. This is particularly useful for components that receive the same props frequently.
const MyComponent = React.memo((props) => {
// component logic
});
2. Use useCallback
and useMemo
to Cache Values and Functions
useCallback
prevents functions from being recreated on every render.useMemo
caches computed values, reducing expensive calculations.
const cachedFunction = useCallback(() => {
// function logic
}, [dependencies]);
const memoizedValue = useMemo(() => {
return computeExpensiveValue(input);
}, [input]);
3. Lazy Loading Components with React.lazy
and Suspense
Load components only when they are needed to reduce initial load time.
Use
React.lazy
for lazy loading components and wrap them withSuspense
to handle loading states.
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
4. Use Efficient State Management
Minimize the number of states by grouping related data to avoid unnecessary renders.
Avoid lifting state up too high; use
context
only for global data, as it can trigger renders in all consuming components.Use libraries like
Redux Toolkit
orRecoil
for managing complex or global state efficiently.
5. Code-Splitting with Dynamic Imports
- Use dynamic imports to load only the necessary parts of your application. Libraries like
React Router
allow route-based code-splitting.
const Component = React.lazy(() => import('./MyComponent'));
6. Avoid Inline Functions and Objects in JSX
Avoid defining functions or objects directly in JSX, as they create new references on every render, causing child components to re-render unnecessarily.
Move functions and objects outside of the render method or use
useCallback
anduseMemo
.
// Instead of:
<MyComponent handleClick={() => doSomething()} />
// Use:
const handleClick = useCallback(() => doSomething(), []);
<MyComponent handleClick={handleClick} />
7. Optimize Conditional Rendering
- For conditional rendering, use short-circuiting (
&&
) instead of ternary operators when the component doesn’t have an alternate value.
// Prefer:
{isVisible && <Component />}
// Over:
{isVisible ? <Component /> : null}
8. Use Proper Key Attributes in Lists
- Always use unique, stable keys (like IDs) for list items. Avoid using array indexes as keys to prevent unnecessary re-renders when the list order changes.
9. Optimize Rendering with shouldComponentUpdate
(Class Components) or React.PureComponent
- For class components, use
shouldComponentUpdate
or extendReact.PureComponent
to automatically perform a shallow comparison of props and state.
10. Minimize Re-Renders with React Profiler
- Use the React DevTools Profiler to identify components with high render times or unnecessary re-renders. This can help pinpoint specific areas in the application that need optimization.
11. Avoid Unnecessary DOM Manipulations
- Avoid accessing or modifying the DOM directly. Instead, use React's state to reflect changes, as React handles DOM updates more efficiently.
12. Optimize CSS and Assets
Use CSS modules or styled-components to scope styles locally, which reduces the CSS file size.
Minimize the number of assets loaded initially by deferring non-essential assets.
13. Limit API Calls and Use SWR or React Query for Data Fetching
- Use data-fetching libraries like SWR or React Query to handle caching, synchronization, and re-fetching, which can prevent redundant API calls and improve data handling performance.
14. Use Concurrent Features in React 18
- Concurrent features (like
startTransition
,useDeferredValue
) help React prioritize important UI updates, enabling smoother interactions.
Example of Optimized Code:
import React, { useState, useCallback, useMemo, lazy, Suspense } from 'react';
// Lazy loading a heavy component
const HeavyComponent = lazy(() => import('./HeavyComponent'));
const MyComponent = ({ data }) => {
// Using memoized value
const calculatedValue = useMemo(() => calculateHeavyOperation(data), [data]);
// Using memoized callback
const handleClick = useCallback(() => {
console.log("Button clicked!");
}, []);
return (
<div>
<p>Calculated Value: {calculatedValue}</p>
<button onClick={handleClick}>Click Me</button>
<Suspense fallback={<div>Loading...</div>}>
<HeavyComponent />
</Suspense>
</div>
);
};
export default React.memo(MyComponent);
Optimizing React apps requires careful attention to how components are rendered, structured, and managed.
Optimizing React applications can feel like a complex task, but with these techniques, you can create faster more efficient applications that your users will love. By embracing memoization, state management best practices, lazy loading, and the other strategies discussed, you’ll be well on your way to mastering React performance. Remember, the best way to improve is to profile your app, identify areas for enhancement, and continuously test the impact of your changes.
Happy coding!
Subscribe to my newsletter
Read articles from Amar Jondhalekar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amar Jondhalekar
Amar Jondhalekar
👨💻 Amar Jondhalekar | Front End Web Developer at Cognizant | Content Creator | Founder at Campuslight With over 3 years of experience in web development, I specialize in HTML5, CSS3, JavaScript (ES6+), React.js, and Node.js. As a Full Stack Developer at Cognizant, I gained hands-on experience building dynamic, responsive applications focused on user-centric design and performance. I also founded Campuslight, where I create impactful digital solutions in the education sector, driven by a mission to make learning more accessible. Through my blogs, I share daily technical insights, coding tips, and career advice, aiming to inspire developers and impress recruiters alike. I’m dedicated to leveraging technology to create a more connected and accessible world. Let’s connect, collaborate, and make the web a better place for everyone!