Introduction to LangChain: Part 1 of 10
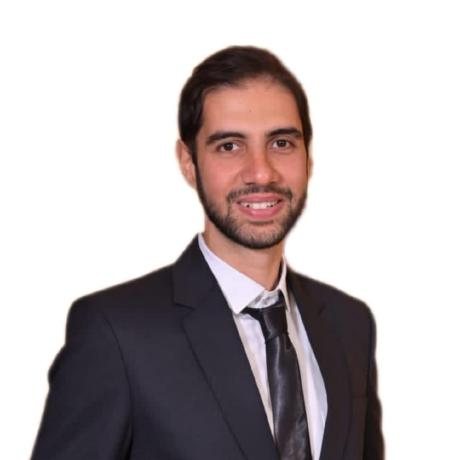
GenAI: GenAI is trained using lots of data from the web, social media, and other online sources. It creates content by looking at the data and finding patterns.
LLM: LLM as the name suggests are large language models which help to provide answers as per the content they are trained upon. GPT-4, Llama, Claude, etc. are a few of them to name.
AI: Encompasses all aspects of bringing intelligence to computers. It allows for the processing of images, text, and audio to generate results.
Machine Learning: A subset of AI where rules and answers are provided to train the ML model. Examples include supervised and unsupervised learning.
Deep Learning: A modern and advanced form of Machine Learning, utilizing multiple interconnected neurons to form a network.
Let’s get started with LangChain
LangChain is a powerful framework that enables developers to build applications with large language models (LLMs) and switch between different models seamlessly.
It’s a middleware to switch to any LLM or Vector DB. It sits between application and LLM, Vector DB, Embeddings, External Data to easily switch to any other LLM without affecting your code.
There are 6 Components of Langchain:
1. Model I/O: Core module. Handles prompt (input) and response (output) from the LLM.
2. Chains: It links prompts, models, tools, memory and retrival. It helps in ordered execution.
3. Tools: Helps to connec to various services/apis like Wikipedia, Google search, etc.
4. Retrival: It will bring context from Vector DB which has all the contents from various sources. Any data cannot be retrieved directly from pdf, jpg, etc. source. It has go to go though Load, Tranform, Embed, Store in Vector DB. So here when you send a prompt to LLM, it will fetch the data/context from Vector DB and send it to LLM.
5. Memory: Store and retrieve short term and long term memory.
6. Agents: Helps to co-ordinate multiple chains, tools, memory, retrival, etc. Then accomplish an action out of it after getting the response from LLM. Eg. personal assistants, chatbots, etc. Handle lot of abilities which LLM miss.
There are few common LangChain libraries to know before we jump to our first development:
langchain_core: Runtime responsible for executing chains, agents, tools, etc. It has Interfaces, Abstractions and LangChain Expression Language(LCEL) - Using these base abstractions LLM Providers create library Responsible for connecting to LLM. Eg. AWS Bedrock, OpenAI, Google Vertex AI, etc. It integrates in langchain core.
langchain_community (earlier), langchain_openai (New one): This is a community library which has all the LLM providers.
langchain: This also uses the core module and has agents, etc. eg. lang_chain.agnets
Now as you know about it, let’s start with installing LangChain libraries:
pip install langchain
pip install langchain-core
pip install langchain-openai # to use with openai API Key
pip install langchain-google-vertexai # to use with gemini api key
After the above steps are completed. You are now good to create your very first GenAI app using LangChain.
Let’s start with each block of code and understand it.
First, we are importing basic os packages and the Gemini model from Google and saving it in the model variable object.
import getpass
import os
os.environ["GOOGLE_API_KEY"] = getpass.getpass()
from langchain_google_vertexai import ChatVertexAI
model = ChatVertexAI(model="gemini-1.5-flash")
Now starts the interesting part. Here we are importing the HumanMessage and SystemMessage libraries to help LangChain understand the context well as instructed in the SystemMessage and answer what is given in the HumanMessage.
Once the below is created. We are ready to call our model and will get the response from it.
from langchain_core.messages import HumanMessage, SystemMessage
messages = [
SystemMessage(content="Translate the following from English into Italian"),
HumanMessage(content="hi!"),
]
model.invoke(messages)
This will give below output in your shell
AIMessage(content='ciao!', response_metadata={'token_usage': {'completion_tokens': 3, 'prompt_tokens': 20, 'total_tokens': 23}, 'model_name': 'gpt-4', 'system_fingerprint': None, 'finish_reason': 'stop', 'logprobs': None}, id='run-fc5d7c88-9615-48ab-a3c7-425232b562c5-0')
The above is quiet difficult to read and you would like to only read the string response.
from langchain_core.output_parsers import StrOutputParser
parser = StrOutputParser()
result = model.invoke(messages)
parser.invoke(result)
# Gives output as -> 'ciao!'
If you want to use the chain feature of langchain to pass one output to the other just as we use | in bash.
chain = model | parser
chain.invoke(messages)
# Gives output as -> 'ciao!'
Conclusion
This article provides an overview of GenAI, large language models (LLMs), AI, machine learning, and deep learning, with a focus on LangChain—a framework for building applications with LLMs. It explains the components of LangChain, such as Model I/O, Chains, Tools, Retrieval, Memory, and Agents, and introduces libraries like langchain_core and langchain_openai. The article then guides readers through installing LangChain and creating a simple application using the Google Gemini model. A code example demonstrates translating English text to Italian using LangChain and highlights the use of chaining to pass outputs efficiently.
In the next step we will go more deep and learn about Prompting techniques in LangChain.
Subscribe to my newsletter
Read articles from Godrej Wadia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
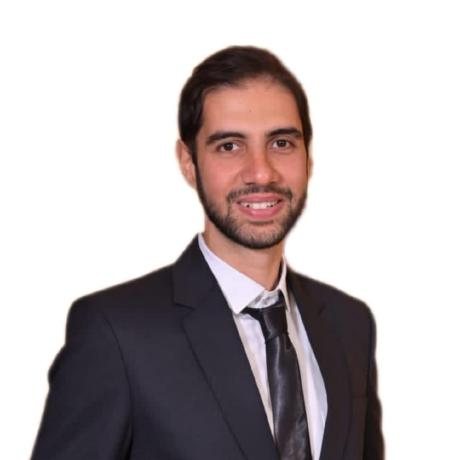
Godrej Wadia
Godrej Wadia
Senior DevOps engineer with 10 years of experience in cloud computing, automation, and CI/CD. Expert in Kubernetes, Docker, Azure, AWS and Jenkins with a track record of delivering scalable and reliable solutions. Proficient in PowerShell, Python, and Terraform with a passion for innovation and optimization.