String programs in Java
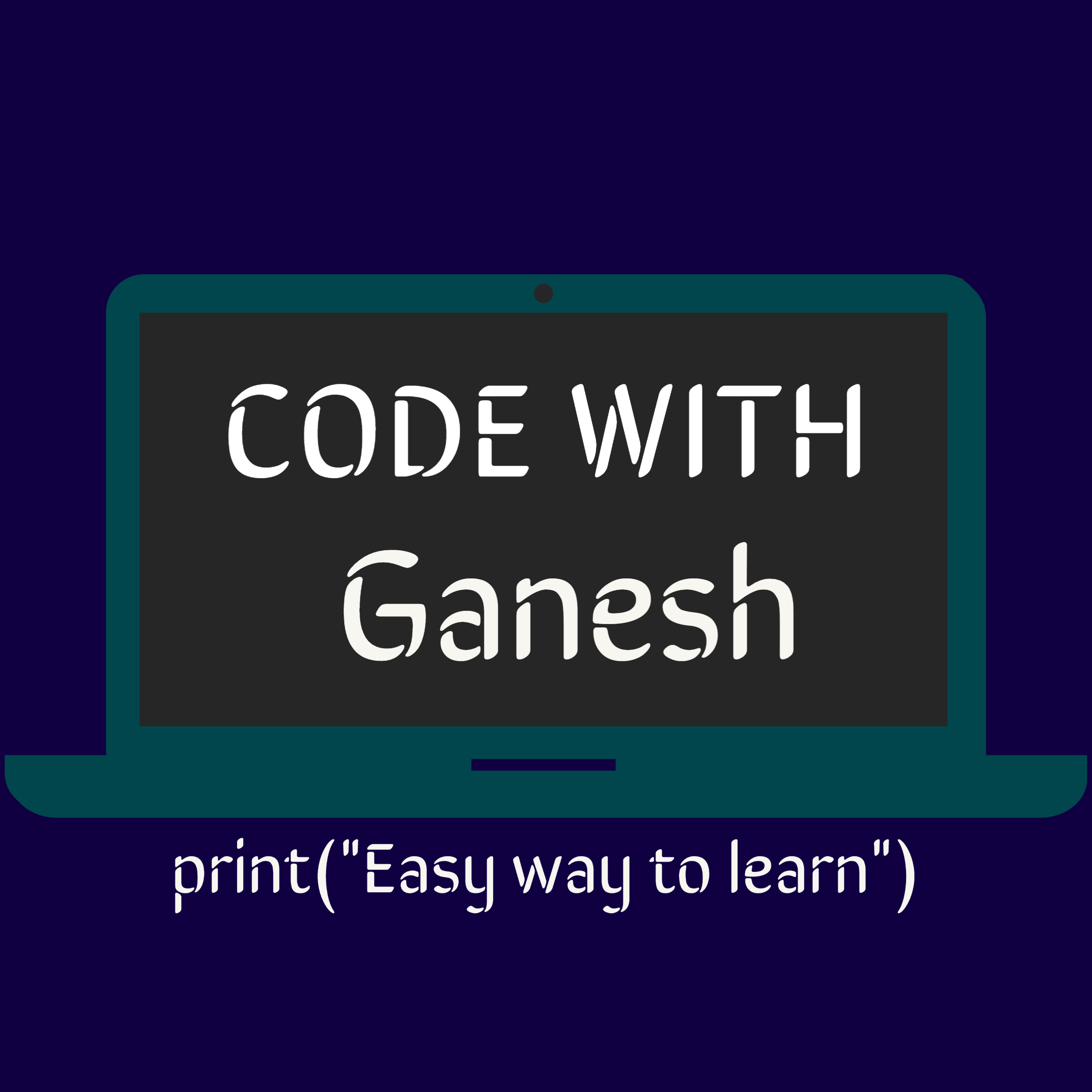
Comprehensive List of String Programming Questions
Basic Questions
Reverse a String
Check if a String is a Palindrome
Count the Number of Vowels and Consonants
Count the Occurrence of Each Character in a String
Find the First Non-Repeating Character in a String
Remove All Whitespaces from a String
Check if Two Strings are Anagrams
Intermediate Questions
Find the Longest Word in a Sentence
Count Occurrences of a Substring
Replace All Vowels in a String with a Specific Character
Check if a String Contains Only Digits
Check if a String is a Rotation of Another String
Convert a Sentence to Title Case
Find the Most Frequent Word in a Sentence
Advanced Questions
Implement Basic String Compression (e.g., "aaabb" becomes "a3b2")
Find the Longest Palindromic Substring
Find the Longest Common Prefix Among a Set of Strings
Implement a Basic Pattern Matching Algorithm (Substring Search)
Generate All Permutations of a String
Implement the KMP Algorithm for Pattern Matching
Find the Smallest Window in a String Containing All Characters of Another String
public class StringExample {
public static void main ( String[] args ) {
}
public static String reverse ( String string) {
// Null checking
if(string == null){
return string;
}
String reverse = "";
for ( int i = string.length () - 1; i >= 0 ; i-- ) {
reverse += string.charAt ( i );
}
return reverse;
}
//Count the vowels in the given string
public static int countVowels(String str) {
int count = 0;
for (int i = 0; i< str.length () -1 ; i++) {
if (str.charAt ( i ) == 'a' || str.charAt ( i ) == 'e' || str.charAt ( i ) == 'i' || str.charAt ( i ) == 'o' || str.charAt ( i ) == 'u') {
count++;
}
}
return count;
}
// 6. Count the occurrences of a character in the given string
public static int countOccurrences(String str, char ch) {
int count = 0;
for ( int i = 0; i < str.length () - 1; i++ ) {
if ( str.charAt ( i ) == ch ){
count++;
}
}
return count;
}
public static String expandString(String input) {
if (input == null || input.isEmpty()) {
return input;
}
String expanded = "";
for (int i = 0; i < input.length(); i++) {
char c = input.charAt(i);
int count = input.charAt(++i) - '0';
for (int j = 0; j < count; j++) {
expanded += c;
}
}
return expanded;
}
// 6. Write a program that compresses a string by replacing consecutive repeating characters with the character followed by the count. For example, "aaabbcddd" should become "a3b2c1d3". If the "compressed" string is longer than the original, return the original string.
public static String compressString(String string){
if(string == null){
return string;
}
String compressedString = "";
for ( int i = 0; i < string.length ( ); i++ ) {
char ch = string.charAt ( i );
int count = 1;
for ( int j = i+1; j < string.length (); j++ ) {
if(string.charAt ( j ) == ch){
count++;
i = j;
}else {
break;
}
}
compressedString += String.valueOf ( ch ) + count;
}
return compressedString;
}
public static String decodeString(String string){
if(string == null){
return string;
}
String decodedString = "";
for ( int i = 0; i < string.length (); i++ ) {
char character = string.charAt ( i );
int count = string.charAt ( ++i ) - '0';
for ( int j = 0; j < count; j++ ) {
decodedString += character;
}
}
return decodedString;
}
public static char findMostFrequentCharacter(String string){
if(string == null){
return 0;
}
int mostFreqCount = 0;
char mostFreqCharacter = '\0';
for ( int i = 0; i < string.length (); i++ ) {
int charCount = 0;
for ( int j = 0; j < string.length (); j++ ) {
if ( string.charAt ( i ) == string.charAt ( j ) ){
charCount++;
}
}
if(charCount > mostFreqCount){
mostFreqCount = charCount;
mostFreqCharacter = string.charAt ( i );
}
}
return mostFreqCharacter;
}
}
Subscribe to my newsletter
Read articles from Nethula Ganesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
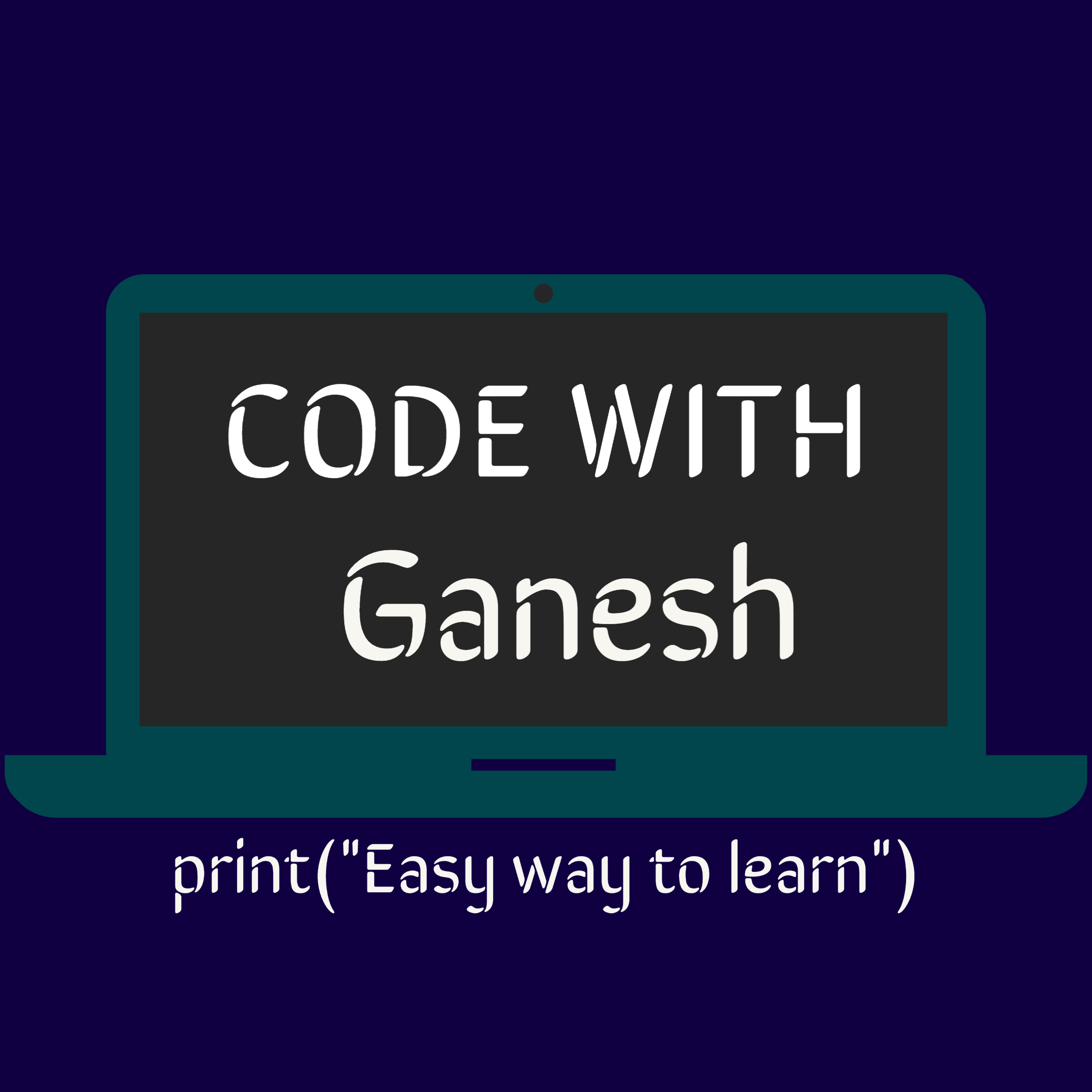