DOM Methods Deep Dive: Powering Up Your Web Pages!
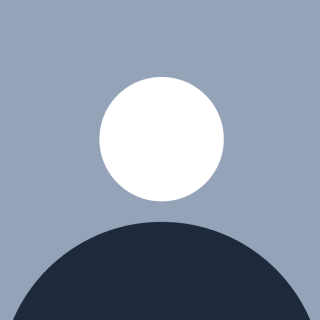
Table of contents
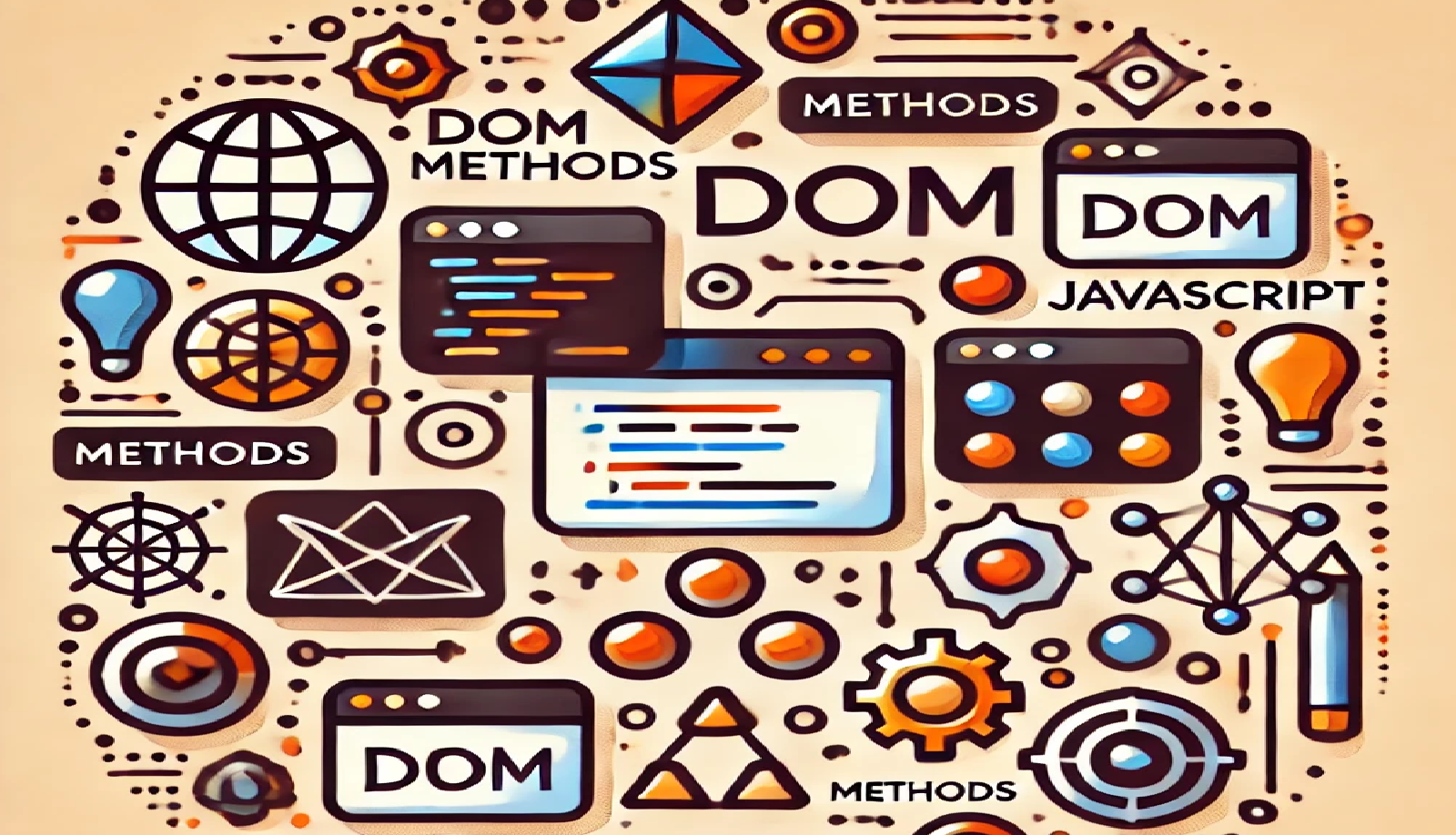
Imagine you’re a treasure hunter, and your mission is to search through a map, identify key landmarks, and collect hidden treasures. In the world of web development, the Document Object Model (DOM) is like that treasure map, and DOM methods are the tools that help you navigate it, finding elements, modifying them, and bringing interactivity to your webpage.
In this article, we’ll dive deep into the most important DOM methods that allow you to access and manipulate elements. These methods are the core tools every web developer needs to create dynamic, interactive web experiences.
Introduction to DOM Methods
DOM methods are built-in JavaScript functions that allow you to interact with the elements of your webpage. They enable you to select, modify, and create elements, making it possible to update the page dynamically based on user input or other actions.
Think of DOM methods as your treasure-hunting tools: some methods help you find specific elements quickly (like a treasure map), while others help you manipulate these elements once you’ve found them (like opening a treasure chest).
Common DOM Methods Explained
Let’s start by exploring the most commonly used DOM methods. These methods are the core of DOM manipulation, allowing you to access elements and their attributes efficiently.
Method 1: getElementById()
getElementById()
is one of the most commonly used methods for selecting elements. It allows you to find an element by its unique id
.
Example:
let heading = document.getElementById("pageTitle");
heading.innerHTML = "Welcome to the Treasure Hunt!";
Explanation:
getElementById("pageTitle")
returns the element with theid
of"pageTitle"
.We can then modify the
innerHTML
property to change the content of the heading.
Method 2: querySelector()
and querySelectorAll()
querySelector()
is a more versatile method that allows you to select the first element that matches a CSS selector, while querySelectorAll()
selects all matching elements.
Example:
let firstButton = document.querySelector(".treasureButton");
firstButton.style.backgroundColor = "gold";
let allButtons = document.querySelectorAll(".treasureButton");
for (let i = 0; i < allButtons.length; i++) {
allButtons[i].style.border = "2px solid black";
}
Explanation:
querySelector(".treasureButton")
selects the first element with the class"treasureButton"
.querySelectorAll(".treasureButton")
selects all elements with the class"treasureButton"
and returns a NodeList. We use a loop to apply a style change to each button.
Method 3: getElementsByClassName()
and getElementsByTagName()
These methods allow you to select multiple elements based on their class or tag name.
Example:
let treasureBoxes = document.getElementsByClassName("box");
for (let i = 0; i < treasureBoxes.length; i++) {
treasureBoxes[i].style.backgroundColor = "blue";
}
let paragraphs = document.getElementsByTagName("p");
for (let i = 0; i < paragraphs.length; i++) {
paragraphs[i].style.fontSize = "18px";
}
Explanation:
getElementsByClassName("box")
returns all elements with the class"box"
.getElementsByTagName("p")
returns all<p>
elements, allowing us to modify their styles.
Flowchart: How DOM Methods Work
Here’s a flowchart to help visualize how DOM methods find and manipulate elements in the DOM:
[Document] ---------> Methods (getElementById, querySelector)
| |
[Find Element] [Modify Element]
| |
[Return Element] ------------> [Change Attributes/Content]
The DOM methods first locate an element based on the provided criteria (ID, class, or tag name). Once the element is found, it can be modified by changing its properties, such as content, style, or attributes.
Event Listeners — Making It Interactive
Event listeners are what bring your webpage to life, allowing it to respond to user actions like clicks, mouse movements, or key presses. Once you’ve found an element using a DOM method, you can attach an event listener to it to make it interactive.
Adding Event Listeners
You can attach an event listener to an element using the addEventListener()
method. This allows you to run a specific function whenever the event occurs.
Example: Adding a Click Event Listener
let treasureChest = document.getElementById("treasureChest");
treasureChest.addEventListener("click", function() {
alert("You found the treasure!");
});
Explanation:
We select the treasure chest element using
getElementById("treasureChest")
.The
addEventListener()
method listens for a"click"
event. When the treasure chest is clicked, the function insideaddEventListener()
is executed, displaying an alert.
Example: Hovering Over an Element
You can also listen for hover events with the "mouseover"
event:
let treasureMap = document.getElementById("treasureMap");
treasureMap.addEventListener("mouseover", function() {
treasureMap.style.border = "5px solid gold";
});
Explanation:
- We listen for the
"mouseover"
event on the treasure map. When the user hovers over the element, it changes the border color.
Event listeners allow you to create a dynamic and interactive experience for users by making elements respond to actions like clicks, hovering, or typing.
Finding and Interacting with Your DOM Treasures
In this part, we explored the fundamental DOM methods that allow you to find and manipulate elements on your webpage. From using getElementById()
to select individual elements to querySelectorAll()
for more complex selections, these methods are the key tools in your treasure-hunting arsenal. We also introduced event listeners to bring interactivity to your page, making it respond dynamically to user input.
In the next part, we’ll dive deeper into more advanced DOM techniques and present a fun challenge: building your own Interactive Treasure Hunt Game!
Advanced DOM Methods
Now that we’ve covered the essential DOM methods, let’s dive into some advanced techniques that can take your web page manipulation to the next level. These methods will help you work more efficiently with elements and create dynamic user experiences.
Method 4: appendChild()
appendChild()
is used to add new elements to the DOM. You can use it to dynamically insert elements, such as adding new content or components to your webpage.
Example: Adding a new treasure box to the page:
let city = document.getElementById("city");
let newBox = document.createElement("div");
newBox.className = "box";
newBox.innerHTML = "<p>New Treasure Box</p>";
city.appendChild(newBox);
Explanation:
createElement()
is used to create a newdiv
element.The
className
property sets the class for the new box, andinnerHTML
adds content to it.appendChild()
adds the new box as a child of thecity
element.
Method 5: removeChild()
removeChild()
allows you to remove an element from the DOM. This is useful when you need to dynamically remove content based on user interactions.
Example: Removing a treasure box from the city:
let boxToRemove = document.getElementById("box1");
city.removeChild(boxToRemove);
Explanation:
- We select the
box1
element and then remove it from thecity
usingremoveChild()
.
Method 6: replaceChild()
Sometimes, instead of removing or appending elements, you may want to replace an existing element with a new one. This is where replaceChild()
comes in handy.
Example: Replacing an old treasure box with a new one:
let oldBox = document.getElementById("oldBox");
let newBox = document.createElement("div");
newBox.className = "box";
newBox.innerHTML = "<p>New Treasure!</p>";
city.replaceChild(newBox, oldBox);
Explanation:
replaceChild()
replaces theoldBox
with the newly creatednewBox
.
Method 7: cloneNode()
If you want to duplicate an element, you can use cloneNode()
. This method allows you to clone an element, with or without its child elements.
Example: Cloning a treasure box:
let treasureBox = document.getElementById("treasureBox");
let clonedBox = treasureBox.cloneNode(true); // true means clone with children
city.appendChild(clonedBox);
Explanation:
cloneNode(true)
creates a deep copy of thetreasureBox
, including its child elements.The cloned box is then appended to the city.
Challenge — Create an Interactive Treasure Hunt Game
Now that we’ve explored both basic and advanced DOM methods, it’s time to put your knowledge to the test with an interactive Treasure Hunt Game! In this challenge, users will search for hidden treasures by clicking on boxes. Some boxes will reveal treasure, while others will be empty.
Challenge Instructions:
Create multiple treasure boxes on the page, each represented by a clickable
<div>
element.When a user clicks on a box, it should either reveal a treasure (e.g., a message saying “You found treasure!”) or say “No treasure here, try again.”
Use event listeners to handle the user clicks and reveal the results.
Optionally, allow the user to remove or replace treasure boxes dynamically.
Here’s an example to get you started:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Treasure Hunt Game</title>
<style>
.box {
width: 100px;
height: 100px;
background-color: lightblue;
border: 2px solid black;
display: inline-block;
margin: 10px;
text-align: center;
line-height: 100px;
cursor: pointer;
}
</style>
</head>
<body>
<div id="city">
<div class="box" id="box1">Box 1</div>
<div class="box" id="box2">Box 2</div>
<div class="box" id="box3">Box 3</div>
<div class="box" id="box4">Box 4</div>
</div>
<script>
let treasureBox = document.getElementById("box3");
let boxes = document.getElementsByClassName("box");
for (let i = 0; i < boxes.length; i++) {
boxes[i].addEventListener("click", function() {
if (this === treasureBox) {
this.innerHTML = "Treasure Found!";
this.style.backgroundColor = "gold";
} else {
this.innerHTML = "No treasure here";
this.style.backgroundColor = "lightgray";
}
});
}
</script>
</body>
</html>
Explanation:
We create four clickable boxes, each represented by a
div
element.An event listener is attached to each box. When a box is clicked, it checks if it’s the treasure box (in this case,
box3
).If it’s the treasure box, the text changes to “Treasure Found!” and the background color turns gold. Otherwise, the box turns light gray and displays “No treasure here.”
You can enhance this game further by allowing the user to add or remove boxes dynamically, or even hide the treasure box in different locations each time the game starts.
Mastering DOM Methods
With the knowledge of both basic and advanced DOM methods, you are now equipped to manipulate web pages dynamically and create interactive experiences for users. Whether it’s selecting elements, modifying them, or listening to user interactions, DOM methods are your key tools for building engaging and responsive websites.
Key Takeaways:
Basic DOM methods like
getElementById()
andquerySelector()
help you locate elements quickly and efficiently.Advanced DOM methods like
appendChild()
,removeChild()
, andcloneNode()
give you more control over how elements are added, removed, or duplicated.Event listeners allow you to make elements interactive, responding to user actions like clicks or hovers.
By mastering these DOM methods, you’re well on your way to building complex and dynamic web applications.
Let’s explore Modularity next!
In the next article, we’ll dive into modularity in JavaScript—how to structure your code into reusable modules and make your programs more scalable. Get ready to explore a key principle for building maintainable codebases!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by