Welcome to My C++ Notes: A Beginner's Guide!
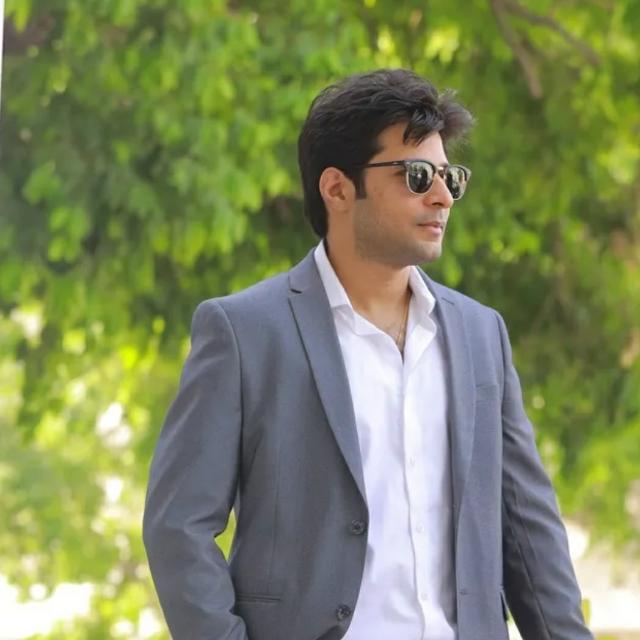
1 min read
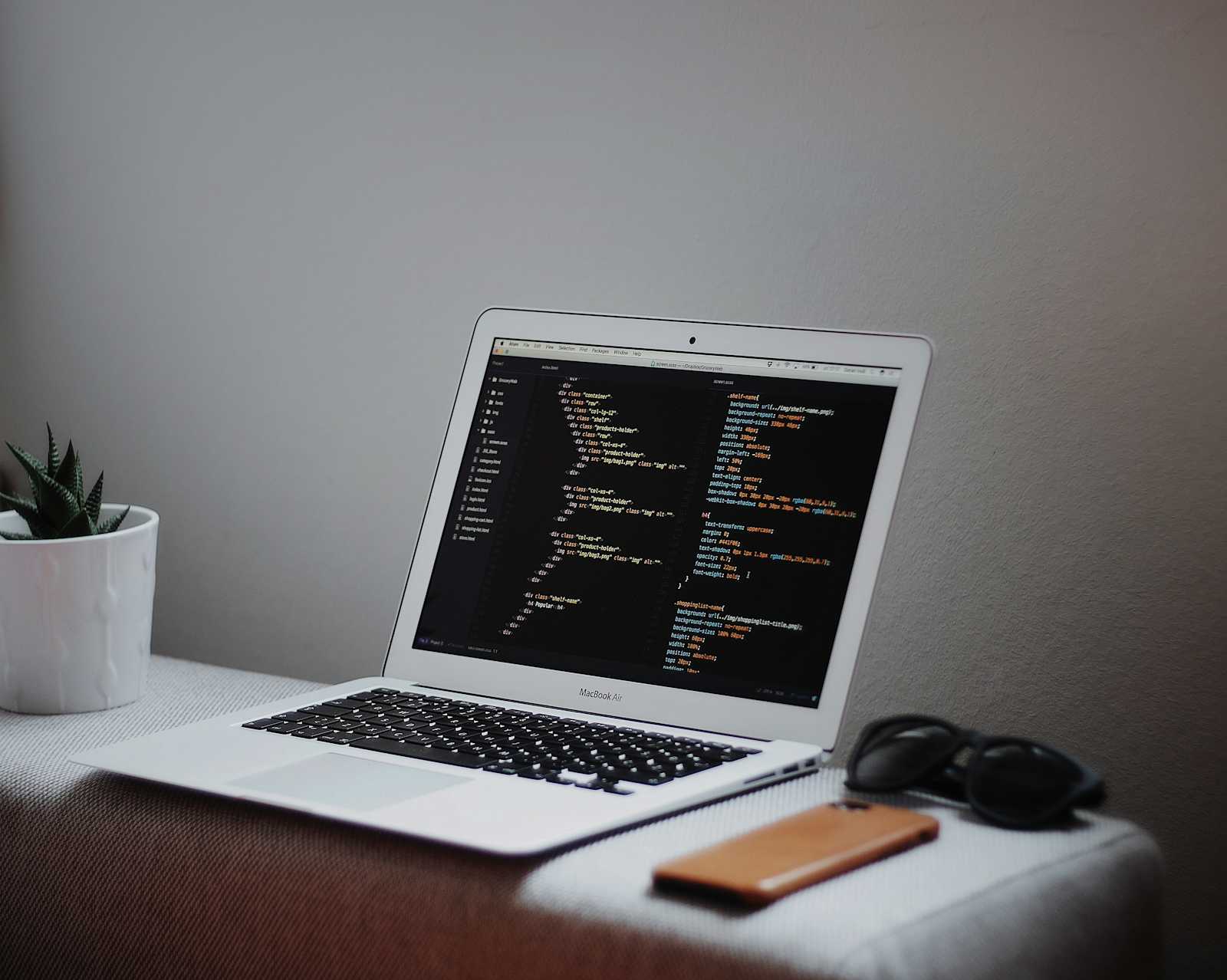
Exercise 1: Find the Maximum Value in a Vector
std::vector<int> vec = {10, 20, 5, 30, 15};
auto max_iter = std::max_element(vec.begin(), vec.end());
if (max_iter != vec.end()) {
std::cout << "The maximum value is " << *max_iter << "\n";
} else {
std::cout << "The vector is empty.\n";
}
Exercise 2: Remove the last element from the vector
To retrieve and remove the last element of a std::vector
in C++, you can use a combination of the back()
and pop_back()
member functions. Alternatively using Iterators, you can use end and erase although this approach is less efficient for removing than pop_back();
int lastElement = vec.back(); // Get the last element 15
vec.pop_back(); // // Remove 15
auto it = vec.end() - 1;
int lastElement = *it;
myVector.erase(it);
0
Subscribe to my newsletter
Read articles from Siddharth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
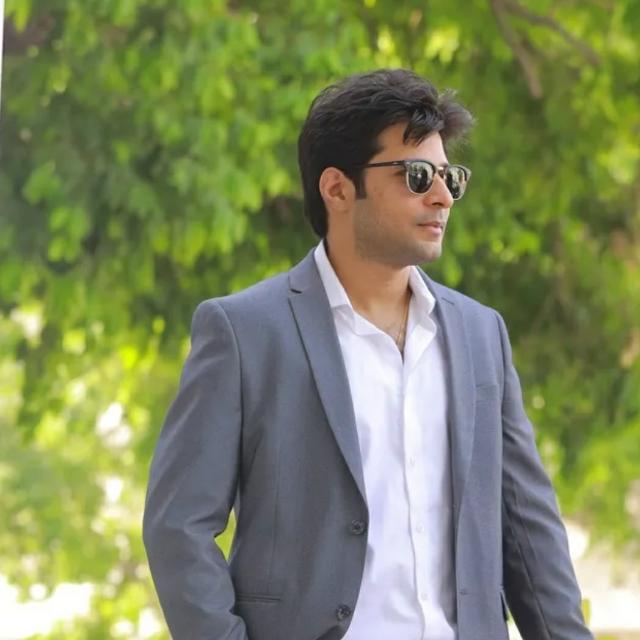
Siddharth
Siddharth
I am Quantitative Developer, Rust enthusiast and passionate about Algo Trading.