Patterns unravelled
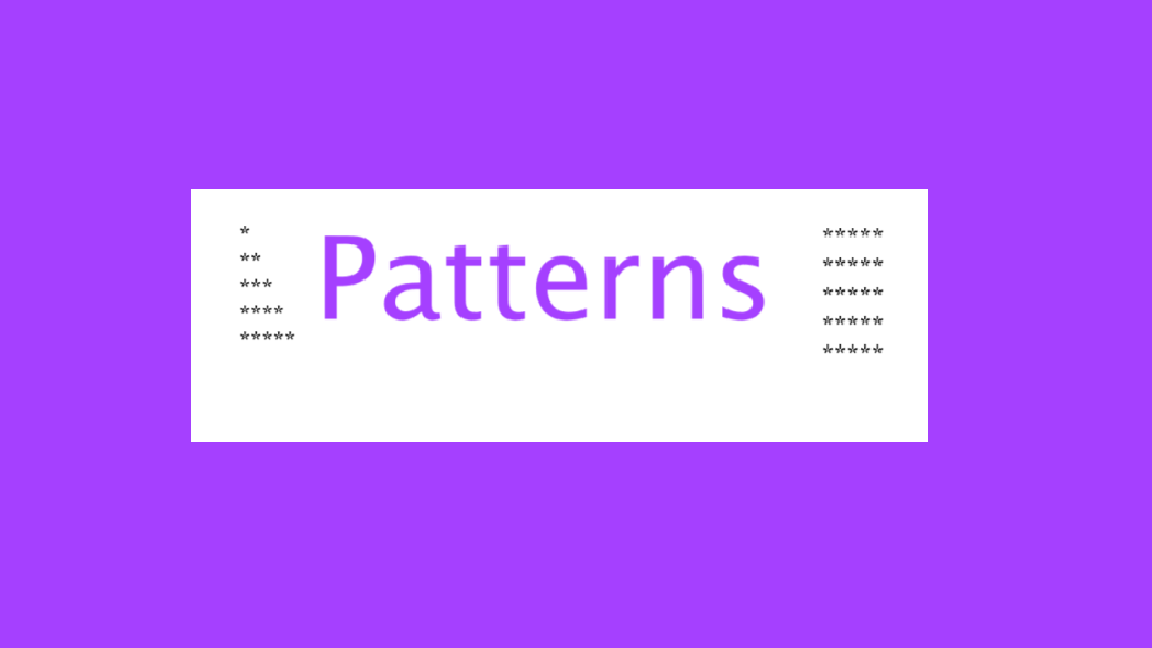
Code this pattern. This is a question I have never heard in any of my interviews, but it is the starting point of DSA. Why? The main reason is that it helps us better understand loops and conditions. Without this, we can't build optimal logic or understand DSA at all.
So, let's decode these patterns.
The way to start is to try to break it down into these simple steps.
Steps
Identify the Rows: Use an outer loop to determine the number of rows.
Identify the Columns: Use an inner loop to determine the number of columns and link it to the current row.
Print the Desired Output: Print the required characters or symbols within the inner loop.
Observe Symmetry: Look for any symmetry patterns in the rows and columns.
Now let's apply these steps to a few questions.
We will solve few questions using these steps only
Q1 -
// 1 Given an integer n. You need to recreate the pattern given below for any
// value of N. Let's say for N = 5, the pattern should look like as below:
// *****
// *****
// *****
// *****
// *****
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern1(num);
}
public static void pattern1(int num) {
// Outer loop to handle the number of rows
for (int i = 0; i < num; i++) {
// Inner loop to handle the number of columns (stars in each row)
for (int j = 0; j < num; j++) {
// Print a star ('*') in the current column
System.out.print("*");
}
// Move to the next line after printing all stars in a row
System.out.println();
}
}
Step 1 - Identify the Rows - 4
Step 2a - Identify the Columns - 4
Step 2b - Connection columns to rows
for 0th row - 4 stars
for 1th row - 4 stars
for 2th row - 4 stars
for 3th row - 4 stars
So, for the i-th row, there will be n stars (where n is the number of columns), placed in all the columns.
Step 3 - Print the Desired Output - System.out.print("*");
Step 4 - There is no symmetry to find here.
Q2 -
// 2 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// *
// **
// ***
// ****
// *****
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern2(num);
}
public static void pattern2(int num) {
// Outer loop to control the number of rows
for (int i = 0; i < num; i++) {
// Inner loop to print stars in each row
for (int j = 0; j <= i; j++) {
System.out.print("*"); // Print one star per iteration
}
// Move to the next line after each row is printed
System.out.println();
}
}
Step 1 - Identify the Rows - 4
Step 2a - Identify the Columns - 4
Step 2b - Connection columns to rows
for 0th row - 1 stars
for 1th row - 2 stars
for 2th row - 3 stars
for 3th row - 4 stars
So, for the i-th row, there will be i+1 stars placed in the first (i+1) columns. The number of stars increases by 1 as you move to the next row.
Step 3 - Print the Desired Output - System.out.print("*");
Step 4 - There is no symmetry to find here.
Q3 -
// 3 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// 1
// 12
// 123
// 1234
// 12345
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern3(num);
}
public static void pattern3(int num) {
// Outer loop to control the number of rows
for (int i = 0; i < num; i++) {
// Inner loop to print numbers in each row
for (int j = 0; j <= i; j++) {
System.out.print(j + 1); // Print the number (j+1) for each iteration
}
// Move to the next line after each row is printed
System.out.println();
}
}
Step 1 - Identify the Rows - 4
Step 2a - Identify the Columns - 4
Step 2b - Connection columns to rows
for 0th row - 1
for 1th row - 12
for 2th row - 123
for 3th row - 1234
So, for the i-th row, the connection will include the numbers from 1 to i+1.
Step 3 - Print the Desired Output - System.out.print("*");
Step 4 - There is no symmetry to find here.
Q4 -
// 4 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// 1
// 22
// 333
// 4444
// 55555
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern4(num);
}
public static void pattern4(int num) {
// Outer loop to control the number of rows
for (int i = 0; i < num; i++) {
// Inner loop to print the same number in each row
for (int j = 0; j <= i; j++) {
System.out.print(i + 1); // Print the number (i+1) repeatedly for each iteration
}
// Move to the next line after each row is printed
System.out.println();
}
}
}
Step 1 - Identify the Rows - 5
Step 2a - Identify the Columns - 5
Step 2b - Connection columns to rows
for 0th row - 1
for 1th row - 22
for 2th row - 333
for 3th row - 4444
for 4th row - 55555
So, for the i-th row, the pattern will include the numbers from 1 to i+1.
Step 3 - Print the Desired Output - System.out.print("*");
Step 4 - There is no symmetry to find here.
Q5 -
// 5 Given an integer n. You need to recreate the pattern given below for
// any vaalue of N. Let's say for N = 5, the pattern should look like as below:
// *****
// ****
// ***
// **
// *
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern5(num);
}
public static void pattern5(int num) {
// Outer loop controls the rows
for (int i = 0; i < num; i++) {
// Inner loop controls the number of stars in each row
for (int j = 0; j < num - i; j++) {
System.out.print("*"); // Print a star
}
// Move to the next line after printing the stars for the current row
System.out.println();
}
}
}
Step 1 - Identify the Rows - 5
Step 2a - Identify the Columns - 5
Step 2b - Connection columns to rows
for 0th row - 5 star
for 1th row - 4 star
for 2th row - 3 star
for 3th row - 2 star
for 4th row - 1 star
So, for the i-th row, the pattern will include the numbers from n-1 star.
Step 3 - Print the Desired Output - System.out.print("*");
Step 4 - There is no symmetry to find here.
Q6 -
// 6 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// 12345
// 1234
// 123
// 12
// 1
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern6(num);
}
public static void pattern6(int num) {
// Step 1: Outer loop to iterate over rows (from 0 to num-1)
for (int i = 0; i < num; i++) {
// Step 2: Inner loop to iterate over columns
// The number of columns decreases with each row (num - i)
for (int j = 0; j < num - i; j++) {
// Step 3: Print numbers from 1 to (num - i) in each row
// j + 1 gives numbers starting from 1 for each column in the row
System.out.print(j + 1);
}
// Step 4: After each row is printed, move to the next line
System.out.println();
}
}
}
Step 1 - Identify the Rows - 5
Step 2a - Identify the Columns - 5
Step 2b - Connection columns to rows
for 0th row - 12345
for 1th row - 1234
for 2th row - 123
for 3th row - 12
for 4th row - 1
So, for the i-th row, the pattern will include numbers starting from 1 to num - i
.
Step 3 - Print the Desired Output - System.out.print(j + 1);
Step 4 - There is no symmetry to find here.
Q7 -
// 7 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// *
// ***
// *****
// *******
// *********
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern7(num);
}
public static void pattern7(int num) {
// Outer loop for the number of rows (height of the triangle)
for (int i = 0; i < num; i++) {
// Inner loop for printing spaces
// It prints spaces to align the stars in the center
// The number of spaces decreases as i increases
for (int j = 0; j < num - i - 1; j++) {
System.out.print(" ");
}
// Inner loop for printing stars
// It prints 2*i + 1 stars for each row, forming a pyramid shape
for (int j = 0; j < 2 * i + 1; j++) {
System.out.print("*");
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - num
(number of rows is equal to the input num
)
Step 2a - Identify the Columns - Varies with each row
Step 2b - Connection columns to rows
For 0th row - 1 star (and 4 spaces before the star, assuming
num = 5
)For 1st row - 3 stars (and 3 spaces before the stars)
For 2nd row - 5 stars (and 2 spaces before the stars)
For 3rd row - 7 stars (and 1 space before the stars)
For 4th row - 9 stars (and no spaces before the stars)
So, for the i-th row:
The number of spaces =
num - i - 1
The number of stars =
2 * i + 1
Step 3 - Print the Desired Output
First, print the spaces:
System.out.print(" ");
Then, print the stars:
System.out.print("*");
Step 4 - There is no symmetry to find here.
Q8 -
// 8 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// *********
// *******
// *****
// ***
// *
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern8(num);
}
public static void pattern8(int num) {
// Outer loop for the number of rows (height of the inverted triangle)
for (int i = 0; i < num; i++) {
// Inner loop for printing spaces
// It prints increasing spaces as i increases
// This creates the right-aligned effect for the inverted triangle
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// Inner loop for printing stars
// The number of stars decreases with each row
// The formula (2 * num - (2 * i + 1)) calculates the number of stars to print on each row
for (int j = 0; j < 2 * num - (2 * i + 1); j++) {
System.out.print("*");
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - num
(number of rows is equal to the input num
)
Step 2a - Identify the Columns - Varies with each row
Step 2b - Connection columns to rows
For 0th row -
2 * num - 1
stars and 0 spaces before the stars.For 1st row -
2 * num - 3
stars and 1 space before the stars.For 2nd row -
2 * num - 5
stars and 2 spaces before the stars.For 3rd row -
2 * num - 7
stars and 3 spaces before the stars.For (num-1)th row - 1 star and (num-1) spaces before the star.
So, for the i-th row:
The number of spaces =
i
The number of stars =
2 * num - (2 * i + 1)
Step 3 - Print the Desired Output
First, print the spaces:
System.out.print(" ");
Then, print the stars:
System.out.print("*");
Step 4 - There is no symmetry to find here.
Q9 -
// 9 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// *
// ***
// *****
// *******
// *********
// *********
// *******
// *****
// ***
// *
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern9(num);
}
public static void pattern9(int n) {
// First half of the pattern (pyramid)
for (int i = 0; i < n; i++) {
// Inner loop to print spaces before the stars
for (int j = 0; j < n - i - 1; j++) {
System.out.print(" ");
}
// Inner loop to print stars
for (int j = 0; j < 2 * i + 1; j++) {
System.out.print("*");
}
// Move to the next line after each row
System.out.println();
}
// Second half of the pattern (inverted pyramid)
for (int i = n - 2; i >= 0; i--) {
// Inner loop to print spaces before the stars
for (int j = 0; j < n - i - 1; j++) {
System.out.print(" ");
}
// Inner loop to print stars
for (int j = 0; j < 2 * i + 1; j++) {
System.out.print("*");
}
// Move to the next line after each row
System.out.println();
}
}
// or
public static void pattern9(int num) {
pattern7(num);
pattern8(num);
}
}
Step 1 - Identify the Rows - The total number of rows is 2 * n - 1
, where n
is the input number.
Step 2a - Identify the Columns - The number of columns varies in each row depending on whether it's the upper or lower half of the pattern.
Step 2b - Connection columns to rows
First Half of the Pattern (Pyramid):
For the 0th row:
n - 1
spaces1
starFor the 1st row:
n - 2
spaces3
starsFor the 2nd row:
n - 3
spaces5
stars
Second Half of the Pattern (Inverted Pyramid):
For the
n-2
th row:1
space2 * (n - 2) + 1
starsFor the
n-3
th row:2
spaces2 * (n - 3) + 1
stars
Step 3 - Print the Desired Output
For each row in both halves, first print spaces:
System.out.print(" ");
Then, print stars:
System.out.print("*");
Step 4 - There is symmetry in the pattern.
This pattern forms a diamond shape. The first half creates an upward-pointing pyramid, and the second half forms a downward-pointing pyramid.
Q10 -
// 10 Given an integer n. You need to recreate the pattern given below for any value of N. Let's say for N = 5, the pattern should look like as below:
// *
// **
// ***
// ****
// *****
// ****
// ***
// **
// *
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern10(num);
}
public static void pattern10(int num) {
// Calling pattern2 to print the first part of the pattern
// (Assuming pattern2 is another method that prints a specific pattern)
pattern2(num);
// Outer loop to print the second part of the pattern (decreasing number of stars)
// It runs from i = 1 to i = num-1, reducing the number of stars with each iteration
for (int i = 1; i < num; i++) {
// Inner loop to print stars
// In each row, the number of stars printed is `num - i`, which decreases as i increases
for (int j = 0; j < num - i; j++) {
System.out.print("*");
}
// After printing the stars for the current row, move to the next line
System.out.println();
}
}
}
Step 1 - Identify the Rows - 4
Step 2a - Identify the Columns - 4
Step 2b - Connection columns to rows
First Part of the Pattern
for 0th row - 1 stars
for 1th row - 2 stars
for 2th row - 3 stars
for 3th row - 4 stars
Second Part of the Pattern
for 0th row - n - 1 stars
for 1th row - n -2 stars
for 2th row - n -3 stars
for 3th row - n -4 stars
Step 3 - Print the Desired Output -
For the second part of the pattern, the program first prints
num - i
stars in each row, wherei
increases from 1 tonum - 1
.The inner loop handles printing the stars:
System.out.print("*");
.After each row of stars,
System.out.println();
moves the cursor to the next line.
Step 4 - There is no symmetry to find here.
Q11 -
// 11 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// 1
// 0 1
// 1 0 1
// 0 1 0 1
// 1 0 1 0 1
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern11(num);
}
public static void pattern11(int num) {
// Outer loop for the number of rows
for (int i = 0; i < num; i++) {
// Determine the starting number (1 or 0) for the current row
int start = 1;
if (i % 2 == 0) {
start = 1; // If the row index is even, start with 1
} else {
start = 0; // If the row index is odd, start with 0
}
// Inner loop to print the pattern in the current row
for (int j = 0; j <= i; j++) {
System.out.print(start); // Print the current number (either 1 or 0)
// Flip the number for the next position
// If start is 1, it becomes 0; if start is 0, it becomes 1
start = 1 - start;
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - num
(number of rows is equal to the input num
)
Step 2a - Identify the Columns - Varies with each row
Step 2b - Connection columns to rows
For 0th row - 1
For 1st row - 01
For 2nd row - 101
For 3rd row - 0101
For 4th row - 10101
So, for the i-th row:
If the row index
i
is even, it starts with1
, followed by alternating numbers.If the row index
i
is odd, it starts with0
, followed by alternating numbers.
Step 3 - Print the Desired Output
Directly print each number with
System.out.print(start);
.Flip the number for the next column with
start = 1 - start;
Step 4 - There is no symmetry to find here.
Q12 -
// 12 Given an integer n. You need to recreate the pattern given below for any value of N. Let's say for N = 5, the pattern should look like as below:
// 1 1
// 12 21
// 123 321
// 12344321
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern12(num);
}
public static void pattern12(int num) {
// [num space num] n= 4
// [1, 6, 1] 0(row) 6 = 2*n - 2*i - 2 = 8-2
// [2, 4, 2] 1(row) 4 = 8- 2 * 1 - 2 = 8-2-2 = 4
// [3, 4, 3] 1(row) 4 = 8- 2 * 2 - 2 = 8-4-2 = 2
// Outer loop for the number of rows
for (int i = 0; i < num; i++) {
// First part of the row: increasing sequence of numbers from 1 up to (i + 1)
for (int j = 0; j <= i; j++) {
System.out.print(j + 1);
}
// Second part of the row: printing spaces in between the two number sequences
// The number of spaces decreases as the row index increases
// Formula for spaces: 2 * num - 2 * i - 2
for (int j = 0; j < 2 * num - 2 * i - 2; j++) {
System.out.print(" ");
}
// Third part of the row: decreasing sequence of numbers from (i + 1) down to 1
for (int j = i + 1; j > 0; j--) {
System.out.print(j);
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - num
(number of rows is equal to the input num
)
Step 2a - Identify the Columns
The pattern in each row consists of three parts:
An increasing sequence of numbers starting from
1
up toi + 1
.A sequence of spaces calculated based on the row number.
A decreasing sequence of numbers starting from
i + 1
down to1
.
Step 2b - Connection Columns to Rows
For an input num = 4
:
Row (i) | Increasing Sequence | Spaces (2 * num - 2 * i - 2) | Decreasing Sequence |
0 | 1 | 6 | 1 |
1 | 1 2 | 4 | 2 1 |
2 | 1 2 3 | 2 | 3 2 1 |
3 | 1 2 3 4 | 0 | 4 3 2 1 |
So, for the i-th row:
The increasing sequence starts from
1
and goes up toi + 1
.The number of spaces is calculated as
2 * num - 2 * i - 2
.The decreasing sequence starts from
i + 1
and goes down to1
.
Step 3 - Print the Desired Output
Print the increasing sequence for each row:
System.out.print(j + 1);
.Print the spaces based on the calculated count:
System.out.print(" ");
.Print the decreasing sequence:
System.out.print(j);
.
Step 4 - Symmetry
- This pattern is symmetric in structure due to the increasing and decreasing sequences around the spaces.
Q13 -
// 13 Given an integer n. You need to recreate the pattern given below for any value of N. Let's say for N = 5, the pattern should look like as below:
// 1
// 2 3
// 4 5 6
// 7 8 9 10
// 11 12 13 14 15
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern13(num);
}
public static void pattern13(int n) {
// Initialize the starting number
int num = 1;
// Outer loop for the number of rows
for (int i = 1; i <= n; i++) {
// Inner loop for printing numbers in each row
// Each row prints `i` numbers, starting from the current value of `num`
for (int j = 1; j <= i; j++) {
System.out.print(num + " "); // Print the current number followed by a space
num = num + 1; // Increment the number for the next position
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - number of row + 1
Step 2b - Connection columns to rows
for 0th row - 1
for 1th row - 2 3
for 2th row - 4 5 6
for 3th row - 7 8 9 10
for 4th row - 11 12 13 14 15
So, for the i-th row, the pattern will include the numbers from 1 to i+1.
Step 3 - Print the Desired Output - System.out.print(num ); This num
is a variable that increases after each i
loop.
Step 4 - There is no symmetry to find here.
Q14 -
// 14 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// A
// AB
// ABC
// ABCD
// ABCDE
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern14(num);
}
public static void pattern14(int n) {
// Outer loop for the number of rows
for (int i = 0; i < n; i++) {
// Inner loop for printing characters in each row
// Starts from 'A' and goes up to 'A' + i, so each row has an increasing sequence of letters
for (char ch = 'A'; ch <= 'A' + i; ch++) {
System.out.print(ch); // Print the current character
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - number of row + 1
Step 2b - Connection columns to rows
for 0th row - A
for 1th row - A B
for 2th row - A B C
for 3th row - A B C D
for 4th row - A B C D E
So, for the i-th row: The characters start from 'A' and continue up to
'A' + i
. Each new row adds one more character in alphabetical order.
Step 3 - Print the Desired Output - System.out.print(ch);
Step 4 - There is no symmetry to find here.
Q15 -
// 15 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// ABCDE
// ABCD
// ABC
// AB
// A
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern15(num);
}
public static void pattern15(int n) {
// Outer loop for the number of rows
for (int i = 0; i < n; i++) {
// Inner loop for printing characters in each row
// Starts from 'A' and prints characters up to 'A' + (n - i - 1)
// As `i` increases, the number of characters printed in each row decreases
for (char ch = 'A'; ch <= 'A' + (n - i - 1); ch++) {
System.out.print(ch); // Print the current character
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - n - row
Step 2b - Connection columns to rows
for 0th row - A B C D E
for 1th row - A B C D
for 2th row - A B C
for 3th row - A B
for 4th row - A
So, For each row, print characters from 'A' up to
'A' + (n - i - 1)
:System.out.print(ch);
.
Step 3 - Print the Desired Output - System.out.print(ch);
Step 4 - There is no symmetry to find here.
Q16 -
// 16 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// A
// BB
// CCC
// DDDD
// EEEEE
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern16(num);
}
public static void pattern16(int n) {
// Outer loop for the number of rows
for (int i = 0; i < n; i++) {
// Initialize the character 'v' as 'A' for each row
char v = 'A';
// Inner loop for printing characters in each row
// The character printed is always 'A' + i
for (char ch = 0; ch <= i; ch++) {
v = (char) ('A' + i); // Assign 'v' to 'A' + i
System.out.print(v); // Print the current character
}
// Move to the next line after printing each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - row + 1
Step 2b - Connection Columns to Rows
For an input n = 4
:
Row (i) | Characters Printed |
0 | A |
1 | B B |
2 | C C C |
3 | D D D D |
So, for the i-th row, the same letter 'A' + i
is printed i + 1
times.
Step 3 - Print the Desired Output
In each row, print the character
'A' + i
multiple times, based on the row index.Move to the next line after printing each row.
Step 4 - Symmetry - There is no symmetry to find here.
Q17 -
// 17 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// A
// ABA
// ABCBA
// ABCDCBA
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern17(num);
}
public static void pattern17(int n) {
// Outer loop for the number of rows
for (int i = 0; i < n; i++) {
// Print leading spaces before characters
for (int j = 0; j < n - i - 1; j++) {
System.out.print(" ");
}
// Print characters with a mirror pattern
char ch = 'A';
int breakpoint = (2 * i + 1) / 2; // Calculate the midpoint for the mirror effect
for (int j = 1; j <= 2 * i + 1; j++) {
System.out.print(ch);
if (j <= breakpoint) {
ch++; // Increase the character until the midpoint
} else {
ch--; // Decrease the character after the midpoint
}
}
// Print trailing spaces after characters
for (int j = 0; j < n - i - 1; j++) {
System.out.print(" ");
}
// Move to the next line after each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - 2 * row + 1
Step 2b - Connection Columns to Rows
For n = 4
, the pattern looks like:
Row (i) | Characters Printed |
0 | A |
1 | A B A |
2 | A B C B A |
3 | A B C D C B A |
Each row forms a sequence of characters that first increases and then decreases symmetrically.
Step 3 - Print the Desired Output
First, print spaces before characters.
Then, print the characters (starting with 'A' and increasing until the middle).
Then, print the characters in reverse order.
Finally, print spaces after characters.
Step 4 - Symmetry - There is no symmetry to find here.
Q18 -
// 18 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// E
// D E
// C D E
// B C D E
// A B C D E
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern18(num);
}
public static void pattern18(int n) {
// Outer loop for the number of rows
for (int i = 0; i < n; i++) {
// Start character is 'A' adjusted by (n - 1) - i
char ch = (char) ('A' + (n - 1) - i);
// Inner loop for printing characters in each row
for (int j = 0; j <= i; j++) {
System.out.print(ch + " "); // Print current character followed by a space
ch++; // Move to the next character in the alphabet
}
// Move to the next line after each row
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - row + 1
Step 2b - Connection Columns to Rows
For n = 4
:
Row (i) | Characters Printed |
0 | D |
1 | D E |
2 | D E F |
3 | D E F G |
Each row starts from the character 'A' + (n - 1) - i
and prints characters in increasing order.
Step 3 - Print the Desired Output
For each row, print the characters starting from
'A' + (n - 1) - i
and increase the character value in each iteration.Move to the next line after printing each row.
Step 4 - Symmetry - There is no symmetry
Q19 -
// 19 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// **********
// **** ****
// *** ***
// ** **
// * *
// * *
// ** **
// *** ***
// **** ****
// **********
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern19(num);
}
public static void pattern19(int n) {
// [star space star] n=5
// 0 [5,0,5] c
// 1[4,2,4] [n, 2i, n]
// 2 [3,4,3]
// Upper part of the pattern
for (int i = 0; i < n; i++) {
// Print stars for the left part
for (int j = i; j < n; j++) {
System.out.print("*");
}
// Print spaces in the middle
for (int j = 0; j < 2 * i; j++) {
System.out.print(" ");
}
// Print stars for the right part
for (int j = i; j < n; j++) {
System.out.print("*");
}
// Move to the next line
System.out.println();
}
// Lower part of the pattern (inverted)
for (int i = 0; i < n; i++) {
// Print stars for the left part
for (int j = 0; j <= i; j++) {
System.out.print("*");
}
// Print spaces in the middle
for (int j = i; j < 2 * n - i - 2; j++) {
System.out.print(" ");
}
// Print stars for the right part
for (int j = 0; j <= i; j++) {
System.out.print("*");
}
// Move to the next line
System.out.println();
}
}
}
Step 1 -Identify the Rows - n
Step 2a - Identify the Columns
the first part has
2 * i + 1
second part has
2 * n - 2 * i - 1
Step 2b - Connection columns to rows
For 0th row - 5 star 0 spaces 5 star
For 1st row - 4 stars 2 spaces 4 star
For 2nd row - 3 stars 4 spaces 3 star
For 3rd row - 2 stars 6 spaces 2 star
For 4th row - 1 stars 8 spaces 1 star
[Star Space Star] = [n 2 * row n]
Step 3 - Print the Desired Output
For each row, first print stars, then spaces, then stars again.
After the first part (top part of the hourglass), repeat the same for the second part.
Step 4 - Symmetry - There is no symmetry
Q20 -
// 20 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// * *
// ** **
// *** ***
// **** ****
// **********
// **** ****
// *** ***
// ** **
// * *
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern20(num);
}
public static void pattern20(int n) {
// Upper part of the pattern
for (int i = 0; i < n; i++) {
// Print stars for the left part
for (int j = 0; j <= i; j++) {
System.out.print("*");
}
// Print spaces in the middle
for (int j = i; j < 2 * n - i - 2; j++) {
System.out.print(" ");
}
// Print stars for the right part
for (int j = 0; j <= i; j++) {
System.out.print("*");
}
// Move to the next line
System.out.println();
}
// Lower part of the pattern (inverted)
for (int i = 1; i < n; i++) {
// Print stars for the left part
for (int j = i; j < n; j++) {
System.out.print("*");
}
// Print spaces in the middle
for (int j = 0; j < 2 * i; j++) {
System.out.print(" ");
}
// Print stars for the right part
for (int j = i; j < n; j++) {
System.out.print("*");
}
// Move to the next line
System.out.println();
}
}
}
Step 1 - Identify the Rows - n
Step 2a - Identify the Columns - n
Step 2b - Connection columns to rows
First section -
For 0th row - 1 star 8 spaces 1 star
For 1st row - 2 stars 6 spaces 2 star
For 2nd row - 3 stars 4 spaces 3 star
For 3rd row - 4 stars 6 spaces 4 star
For 4th row - 1 stars 8 spaces 1 star
[Star Space Star] = [i+1 2 * row i+1]
Second section -
For 0th row - 5 star 0 spaces 5 star
For 1st row - 4 stars 2 spaces 4 star
For 2nd row - 3 stars 4 spaces 3 star
For 3rd row - 2 stars 6 spaces 2 star
For 4th row - 1 stars 8 spaces 1 star
[Star Space Star] = [n 2 * row n]
Step 3 - Print the Desired Output
Print stars, then spaces, then stars, for each row.
The top part (first
n
rows) decreases stars and increases spaces.The bottom part (next
n-1
rows) does the opposite.
Step 4 - Symmetry - This pattern is symmetric both horizontally and vertically.
Q21 -
// 21 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// *****
// * *
// * *
// * *
// *****
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern21(num);
}
public static void pattern21(int n) {
// First way: Print the square border
for (int i = 0; i < 1; i++) {
// Print the full row of stars at the top
for (int j = 0; j < n; j++) {
System.out.print("*");
}
System.out.println();
}
// Print the middle part of the square with spaces between stars
for (int i = 1; i < n - 1; i++) {
// Print stars at the beginning and end of each row
for (int j = 0; j < 1; j++) {
System.out.print("*");
}
// Print spaces in the middle
for (int j = 0; j < n - 2; j++) {
System.out.print(" ");
}
// Print stars at the end
for (int j = 0; j < 1; j++) {
System.out.print("*");
}
System.out.println();
}
// Last row: Print the full row of stars at the bottom
for (int i = n - 1; i < n; i++) {
// Print the full row of stars at the bottom
for (int j = 0; j < n; j++) {
System.out.print("*");
}
System.out.println();
}
// Second way: Print square with border stars and spaces in the middle
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
// Print stars on the borders
if (i == 0 || j == 0 || i == n - 1 || j == n - 1) {
System.out.print("*");
} else {
System.out.print(" "); // Print spaces inside the border
}
}
System.out.println();
}
}
}
Step 1 -Identify the Rows - n
Step 2a - Identify the Columns - n
Step 2b - Connection columns to rows
For 0th row and n-1 row - n star
For rest row - 0th and n-1 column stars else spaces ]
Step 3 - Print the Desired Output
The first and last rows are completely filled with stars.
The middle rows have stars only at the borders, with spaces in between.
Step 4 - Symmetry - There is no symmetry
Q22 -
// 22 Given an integer n. You need to recreate the pattern given below for
// any value of N. Let's say for N = 5, the pattern should look like as below:
// 5 5 5 5 5 5 5 5 5
// 5 4 4 4 4 4 4 4 5
// 5 4 3 3 3 3 3 4 5
// 5 4 3 2 2 2 3 4 5
// 5 4 3 2 1 2 3 4 5
// 5 4 3 2 2 2 3 4 5
// 5 4 3 3 3 3 3 4 5
// 5 4 4 4 4 4 4 4 5
// 5 5 5 5 5 5 5 5 5
import java.util.Scanner;
public class Pattern {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number ");
int num = scanner.nextInt();
pattern22(num);
}
public static void pattern22(int n) {
// Outer loop for the rows
for (int i = 0; i < 2 * n - 1; i++) {
// Inner loop for the columns
for (int j = 0; j < 2 * n - 1; j++) {
// Calculate the minimum distance from each of the 4 borders
int top = i;
int bottom = j;
int right = (2 * n - 2) - j;
int left = (2 * n - 2) - i;
// Print the decreasing numbers, starting from 'n' at the borders
System.out.print((n - Math.min(Math.min(top, bottom), Math.min(left, right))) + " ");
}
// Move to the next row
System.out.println();
}
}
}
Step 1 -Identify the Rows - 2 * n - 1
Step 2a - Identify the Columns - 2 * n - 1
Step 2b - Connection columns to rows
For each cell, calculate the minimum distance from any of the four boundaries (top, bottom, left, right) and subtract it from
n
to get the value.For rest row - 0th and n-1 column stars else spaces ]
Step 3 - Print the Desired Output - Print the values for each cell and move to the next row.
Step 4 - Symmetry - There is no symmetry
So that's the final one.
In this blog, we've explored important patterns, explained the logic, and solved some questions to practice what we've learned. Patterns are not just visual designs; they help improve problem-solving skills and make coding more efficient. By learning these basic patterns, you're creating a solid foundation for tackling more complex problems in the future.
Subscribe to my newsletter
Read articles from Vaishnavi Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by