Sync vs Async: A Code-Off!

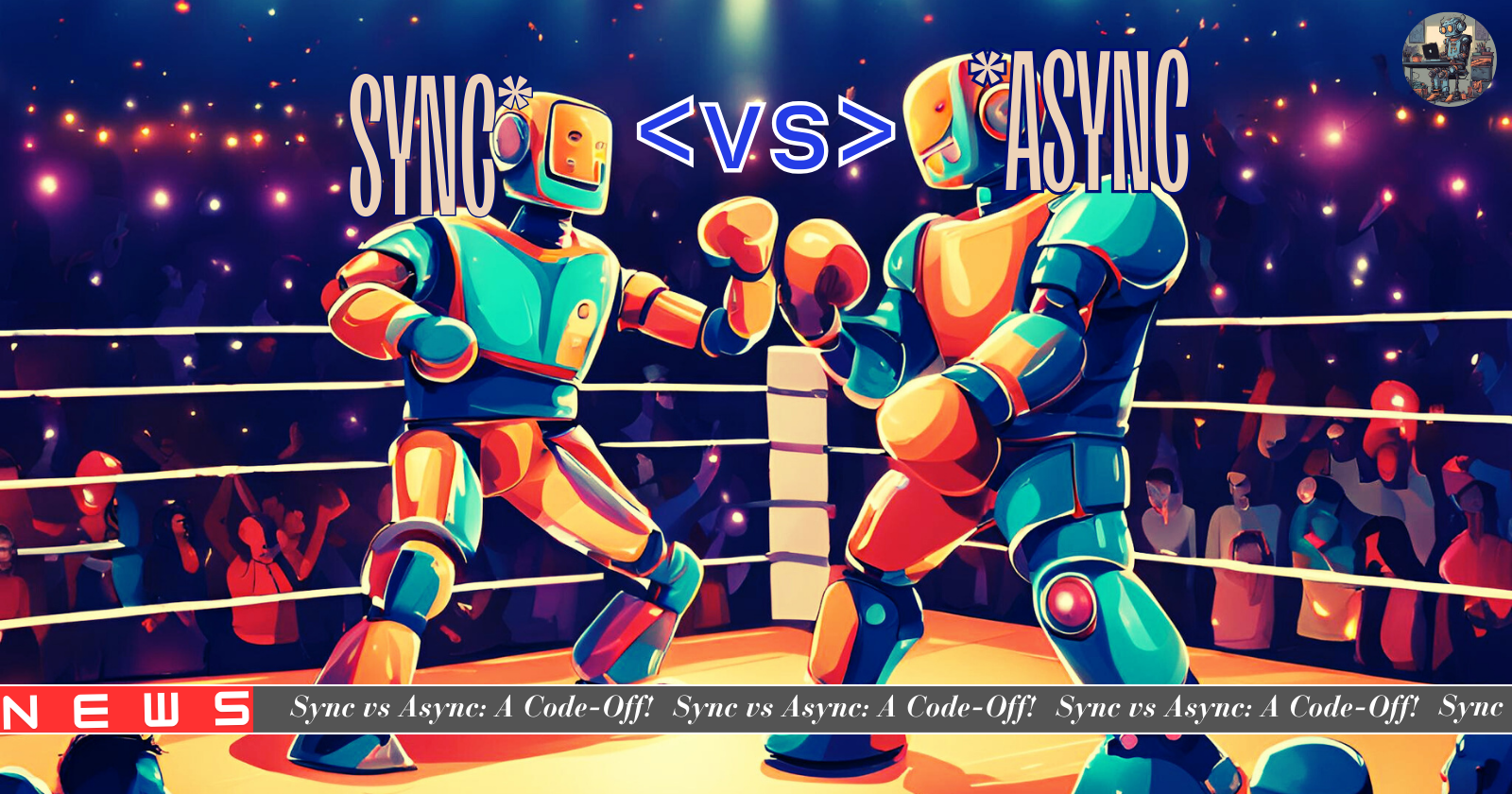
Since I already have some knowledge about basic HTML, CSS, and JavaScript, I decided to begin with Asynchronous and Synchronous JavaScript .Let me share what I’ve learnt!
Synchronous Code
Synchronous code is JavaScript that executes line by line in the order it appears. Let’s look at an example:
console.log("wazzupppp!");
function greet() {
console.log("Hello, World!");
}
greet();
console.log("done!");
Here’s what happens step by step:
"wazzupppp!" is logged to the console.
The compiler reads the function
greet()
but doesn’t execute it yet.When
greet()
is called, it goes back to the function definition and executes it, logging "Hello, World!".Finally, "done!" is printed.
This linear flow of execution, where one task starts only after the previous one completes, defines synchronous code.
Asynchronous Code
Now, let’s talk about asynchronous code. Unlike synchronous code, asynchronous tasks allow the program to move on to the next line while waiting for a previous task to complete. It sounds chaotic, but it’s incredibly efficient. Here’s a simple analogy:
Real-World Example
Imagine you have two tasks to complete before your mom gets home:
Microwave dinner.
Take clothes out of the washing machine.
Option 1 (Synchronous):
You could wait for the microwave to finish, then take the clothes out. This would take around 15 minutes.
Option 2 (Asynchronous):
Start the microwave and, while it's running, take out the clothes. By the time you’re done, dinner is ready. This takes only about 10 minutes.
While the time difference might be small in this example, imagine performing large-scale tasks. Asynchronous execution prevents delays and makes better use of time.
I/O-Bound and CPU-Bound Tasks
Here’s a quick side note on the types of tasks:
I/O-Bound Tasks: Rely on external operations like reading files or fetching data over a network.
CPU-Bound Tasks: Involve heavy computations and depend on the system’s processing power like running a loop from 0 to 100000000.
Synchronous File Reading with readFileSync
The readFileSync
method from Node.js reads files synchronously. This means the program stops and waits for the file to be fully read before proceeding.
Note: I don’t know Node.js yet, so if you're like me and have no idea about it, don’t PANIC. Just focus on grasping the concepts without stressing over the syntax or language too much.
const fs = require('fs');
const data = fs.readFileSync('example.txt', 'utf8');
console.log('File contents:', data);
console.log('This line runs after the file is read.');
What happens here?
The program halts at
fs.readFileSync
until the file is completely read.Once the file is read, its contents are logged.
Finally, the last line logs "This line runs after the file is read."
This behavior ensures the file is ready before moving on, but it can be problematic for large files or while running CPU-Bound tasks, potentially freezing the program.
Asynchronous File Reading with readFile
Now, let’s see how readFile
works asynchronously. This method reads files without blocking the execution of your program.
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
console.log('This line runs *before* the file is read.');
How does it work?
fs.readFile
starts reading the file in the background.Meanwhile, the program continues and logs "This line runs before the file is read."
Once the file is ready, the callback function logs "File contents".
This non-blocking behavior ensures your application remains responsive. Imagine running a web server—you wouldn’t want users to wait while you load a file in the background, right? Asynchronous methods allow your server to handle other tasks in the meantime. making it easier to handle CPU-Bound tasks.
Synchronous vs. Asynchronous: A Quick Comparison
Feature | Synchronous (readFileSync ) | Asynchronous (readFile ) |
Execution | Blocks execution until task completes. | Executes other tasks while waiting. |
Performance | Slower for large tasks. | Fast and responsive. |
Use Case | Small tasks, initialization. | Heavy or I/O-bound tasks. |
Error Handling | try...catch . | Handles errors in the callback. |
Summary….
Synchronous: Runs line by line, waits for each task.
Asynchronous: Runs tasks simultaneously, doesn’t wait.
I/O-Bound and CPU-Bound Tasks:
I/O-Bound Tasks: Dependent on the speed of external operations (e.g., file reading or network communication).
CPU-Bound Tasks: Dependent on the system's processing power (e.g., heavy computations).
File Reading Methods in Node.js:
readFileSync
(Synchronous): Blocks execution until the file is fully read.readFile
(Asynchronous): Allows other tasks to execute while the file is being read in the background.
Comparison between Synchronous and Asynchronous:
Synchronous = blocking the execution,
Asynchronous = non-blocking.
"Stay tuned for more reads through my web development journey. I hope to see you in the next post too! That's all from me for now... see ya! ✋"
The knowledge shared in this blog is based on my learnings from “Complete Web development + Devops Cohort” by Harkirat Singh. You can check out the course here:go to Harkirat's course website.
Subscribe to my newsletter
Read articles from Hitesh Venugopalan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hitesh Venugopalan
Hitesh Venugopalan
Hey there! I'm a college student majoring in Computer Science, and this blog is my way of documenting my journey from having scattered bits of programming knowledge to building meaningful projects. Join me as I dive into the world of web development (for now), and watch as I explore other exciting paths in tech along the way. I invite you to follow along, learn with me, and share your insights or guidance when I need it. Whether you’re a beginner or a seasoned developer, there’s something here for everyone as we voyage through the ever-evolving world of coding. P.S. I may not still be a beginner when you stumble across this blog, but you’ll get to see where it all began and how far I've come!