Understanding Terraform Modules - A Complete Guide
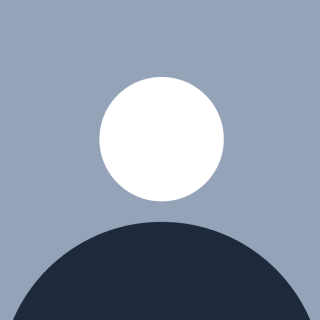
Introduction
Terraform modules are a fundamental concept that helps in organizing and maintaining Infrastructure as Code (IaC). This guide explains what modules are, why they're important, and how to implement them effectively.
Why Modules? - The Monolithic Problem
Let's understand the need for modules through an analogy:
The Monolithic Application Problem
Imagine a company with millions of lines of code in a single monolithic application. This creates several challenges:
Difficult Bug Resolution: New developers struggle to locate and fix bugs in a massive codebase
Lack of Ownership: Hard to determine who owns which part of the code
Maintenance Challenges: Upgrading and maintaining the application becomes complex
Testing Difficulties: Need to test the entire application even for small changes
Similar Challenges in Terraform
The same problems occur with Terraform when managing infrastructure:
One large Terraform project managing multiple resources
Difficult for new team members to understand
Hard to maintain and update
Testing becomes complex
Lack of code reusability across teams
Benefits of Terraform Modules
Modularity: Break down complex infrastructure into manageable components
Reusability: Share and reuse infrastructure code across teams
Simplified Collaboration: Better team ownership and maintenance
Version Control: Easier to manage versions of infrastructure components
Abstraction: Hide complex implementation details
Better Testing: Test individual modules independently
Enhanced Documentation: Document infrastructure components separately
Improved Scalability: Scale different components independently
Better Security: Manage access controls at module level
Practical Implementation
Basic Project Structure
project/
├── main.tf
├── variables.tf
├── outputs.tf
└── terraform.tfvars
Converting to Modular Structure
project/
├── main.tf
└── modules/
└── ec2_instance/
├── main.tf
├── variables.tf
└── outputs.tf
Module Implementation Example
- Create the module structure:
# modules/ec2_instance/main.tf
resource "aws_instance" "example" {
ami = var.ami_value
instance_type = var.instance_type_value
subnet_id = var.subnet_id_value
}
- Define module variables:
# modules/ec2_instance/variables.tf
variable "ami_value" {
description = "Value for the AMI"
}
variable "instance_type_value" {
description = "Value for instance type"
}
variable "subnet_id_value" {
description = "Value for the subnet ID"
}
- Use the module:
# main.tf
provider "aws" {
region = "us-east-1"
}
module "ec2_instance" {
source = "./modules/ec2_instance"
ami_value = "ami-xxxxx"
instance_type_value = "t2.micro"
subnet_id_value = "subnet-xxxxx"
}
Best Practices
Module Organization:
Keep modules focused on specific infrastructure components
Create separate repositories for frequently reused modules
Use clear naming conventions
Version Control:
Store modules in version control systems
Tag module versions for better dependency management
Document breaking changes between versions
Documentation:
Document input variables and their purposes
Include usage examples
Describe expected outputs
Document any dependencies or prerequisites
Testing:
Test modules independently
Include example configurations
Validate module behavior in different scenarios
Using Public vs Private Modules
Public Modules
Available through Terraform Registry
Community-maintained
Good for learning and reference
Use with caution in production environments
Private Modules
Organization-specific implementations
Stored in private repositories
Better control over security and compliance
Customized for organizational needs
Conclusion
Terraform modules are essential for maintaining clean, reusable, and manageable infrastructure code. They solve many of the same problems that microservices solve in application development. By properly implementing modules, teams can better manage their infrastructure code, improve collaboration, and ensure consistency across their organization.
Remember: Start with a simple structure and modularize as your infrastructure grows. Focus on creating reusable, well-documented modules that solve specific infrastructure needs.
Tags: #terraform #aws #devops #infrastructureascode #cloudcomputing
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by