Mastering Advanced ReactJS Techniques

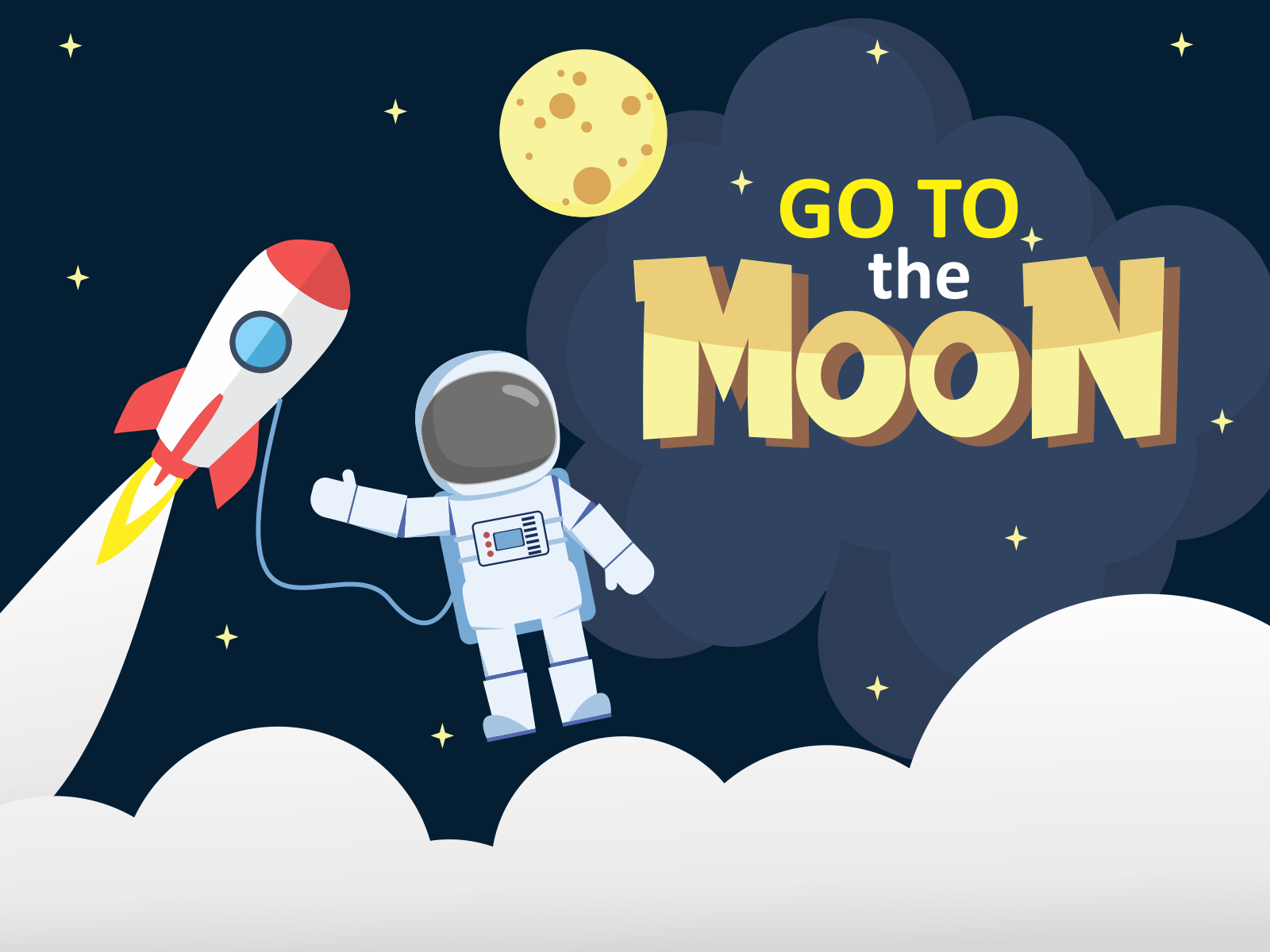
Here's what I’d recommend as a learning path to develop those areas. Each step has practical aspects and real-world examples so you can master these advanced concepts.
1. Refining Component Architecture
Key Concept: Building reusable, isolated components makes your app modular and maintainable.
Learning Steps:
Study Design Patterns: Explore patterns like Compound Components, Controlled Components, Render Props, and Higher-Order Components (HOCs). Each pattern is useful for creating flexible, reusable UI components.
Context and State Management: Master Context API and consider libraries like Zustand or Redux if your app grows larger. Context API can help you share state across components effectively, without passing props down too many layers.
Example: Build a Form Component that takes different field types as child components using the Compound Component pattern, allowing each field to manage its validation independently.
2. Advanced Real-Time Integrations
Key Concept: Real-time data updates keep your app interactive and engaging, especially for live dashboards, chats, or collaborative tools.
Learning Steps:
WebSockets & Libraries: Start with native WebSocket APIs for a solid base, then move on to
socket.io
or Ably for more features like automatic reconnection and room-based messaging.Optimized State Syncing: Experiment with state syncing techniques to handle real-time data. Learn to manage UI and data consistency, especially with conflict resolution when users interact with the same data in real time.
Example: Build a live chat or notifications system where each message or notification triggers an update on all active clients.
3. In-depth TypeScript Knowledge
Key Concept: TypeScript makes your code safer and more predictable, especially in complex or large-scale projects.
Learning Steps:
Generics & Utility Types: Practice with TypeScript generics, which allow you to create flexible and reusable type-safe components and functions.
Advanced Types: Learn about mapped types, conditional types, and how they can help you create versatile, reusable interfaces that adapt based on input types.
Example: Build a generic data-fetching hook that dynamically infers response data types based on the endpoint, ensuring type safety throughout your app.
4. State Management and Complex Data Handling
Key Concept: Efficient state management helps you avoid unnecessary re-renders and boosts performance, especially in large datasets or highly interactive apps.
Learning Steps:
State Libraries: Experiment with Zustand, Recoil, or React Query to manage local and server-side state seamlessly. These libraries can simplify data fetching, caching, and syncing with backend data sources.
Memoization Techniques: Use
React.memo
,useMemo
, anduseCallback
effectively to optimize component re-renders when working with large datasets or complex UIs.Example: Create a dashboard component that uses React Query to fetch and cache data, memoizes computed values, and only re-renders necessary components.
Final Project to Put It All Together
Goal: Build an advanced real-time dashboard that uses efficient data-fetching, component patterns, TypeScript for safety, and real-time updates.
Features:
Infinite scrolling or pagination with complex datasets
A component architecture that leverages patterns (Compound Components, Context) for modularity
Real-time updates with WebSockets
TypeScript for static type checking and reusability
This roadmap will equip you with the advanced knowledge you need to tackle a range of frontend challenges confidently! Let me know if you'd like more details on any of these points, and I'll be happy to dive deeper.
Subscribe to my newsletter
Read articles from kietHT directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

kietHT
kietHT
I am a developer who is highly interested in TypeScript. My tech stack has been full-stack TS such as Angular, React with TypeScript and NodeJS.