Understanding Terraform Provisioners: A Real-World Implementation Guide
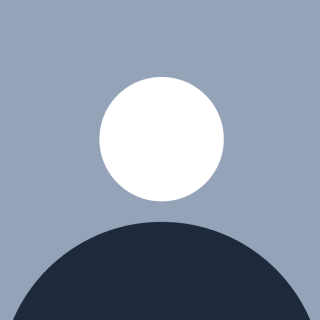
Introduction
Terraform Provisioners are powerful tools that enable you to execute scripts and commands on resources during creation or destruction. While Terraform excels at infrastructure provisioning, provisioners bridge the gap between infrastructure creation and configuration management.
What is a Provisioner?
A Terraform feature that lets you execute actions during resource creation/destruction
Helps automate post-deployment configuration
Enables "zero-touch automation" for infrastructure deployment
Reduces manual intervention in deployment processes
Types of Provisioners
1. Remote-exec Provisioner
Purpose: Execute commands on remote resources
Use Cases:
Installing software packages
Starting services
Running configuration scripts
Example:
provisioner "remote-exec" {
inline = [
"sudo apt-get update",
"sudo apt-get install -y python3-pip",
"pip install flask",
"python3 app.py"
]
}
2. File Provisioner
Purpose: Copy files from local machine to remote resources
Use Cases:
Deploying application files
Copying configuration files
Transferring scripts
Example:
provisioner "file" {
source = "app.py"
destination = "/home/ubuntu/app.py"
}
3. Local-exec Provisioner
Purpose: Execute commands on the Terraform running machine
Use Cases:
Creating local logs
Running local scripts
Triggering other tools
Progress tracking
Practical Implementation Example
Project Overview
Creating a complete deployment pipeline:
Infrastructure provisioning (VPC, Subnet, EC2)
Application deployment
Configuration management
Infrastructure Components
VPC with CIDR block
Public subnet
Internet Gateway
Route table
Security group
EC2 instance
Key pair for SSH access
Connection Block
connection {
type = "ssh"
user = "ubuntu"
private_key = file("~/.ssh/id_rsa")
host = self.public_ip
}
Best Practices
When to Use Provisioners
Simple configuration tasks
Initial setup requirements
Application deployment
When other tools (like user data) aren't suitable
When to Avoid Provisioners
Complex configuration management
Tasks better suited for specialized tools
When infrastructure changes don't require configuration updates
General Guidelines
Keep provisioner actions idempotent
Handle failure scenarios
Use appropriate timeout values
Consider security implications
Document provisioner usage
Common Challenges and Solutions
1. Connection Issues
Ensure security group rules are correct
Verify SSH key configuration
Check network connectivity
Allow sufficient time for instance initialization
2. Script Execution Problems
Use proper shebang lines
Handle script permissions
Consider environment variables
Implement error handling
3. File Transfer Issues
Verify file paths
Check file permissions
Ensure sufficient disk space
Handle large file transfers appropriately
Integration with CI/CD
Workflow Steps
Developers update application code
CI/CD pipeline triggers
Terraform creates/updates infrastructure
Provisioners deploy updated application
Automated testing validates deployment
Benefits
Reduced manual intervention
Consistent deployments
Faster development cycles
Better reliability
Conclusion
Terraform Provisioners provide a powerful way to bridge the gap between infrastructure provisioning and configuration management. While they should be used judiciously, they're invaluable for creating fully automated deployment pipelines.
Code Repository Structure
project/
├── main.tf
├── variables.tf
├── outputs.tf
├── app.py
└── README.md
Additional Resources
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by