An Introduction to Different Types of Variables in Python - day 36

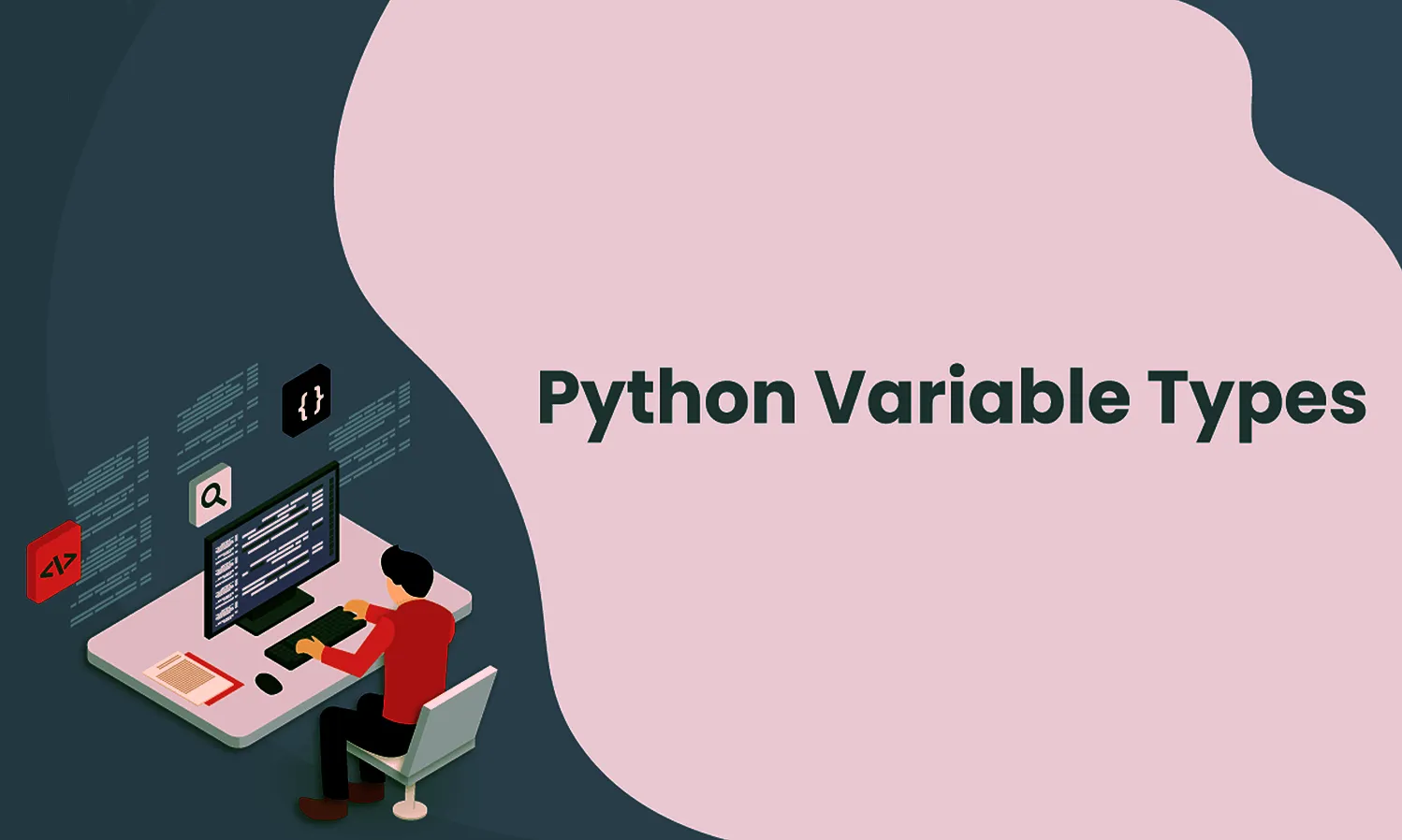
Introduction :
Welcome back to my Python journey! Previously, I started the OOPs concept in python in python.
Today, I dove into types of Variables, Let's explore what I learned!
Types of Variables:
Python support 3 types of variables
1. Instance Variables (Object Level Variables)
2. Static Variables (Class Level Variables)
3. Local variables (Method Level Variables)
Instance Variables:
If the value of a variable is varied from object to object, then such type of variables are called instance variables.
For every object a separate copy of instance variables will be created.
For every object a separate copy of instance variables will be created.
Where we can declare Instance variables:
1. Inside Constructor by using self variable
2. Inside Instance Method by using self variable
3. Outside of the class by using object reference variable
1. Inside Constructor by using self variable:
We can declare instance variables inside a constructor by using self keyword.
Once we creates object, automatically these variables will be added to the object
class Emp :
def __init__(self):
self.eno= 101
self.ename = "jithon"
self.sal = 20000
e= Emp()
print (e.__dict__)
2. Inside Instance Method by using self variable:
We can also declare instance variables inside instance method by using self variable.
If any instance variable declared inside instance method, that instance variable will be added once we call taht method
class Test:
def __init__(self):
self.a=10
self.b=20
def m1(self):
self.c=30
t=Test()
t.m1()
print(t.__dict__)
3. Outside of the class by using object reference variable:
We can also add instance variables outside of a class to a particular object.
class Test:
def __init__(self):
self.a=10
self.b=20
def m1(self):
self.c=30
t=Test()
t.m1()
t.d= 40
print (t.__dict__)
EXAMPLE :
class Emp :
''' this class take emp information like empid , empname & Bsal '''
def __init__ (self) : #uisng const
self. empid = 1000
self . empname= "Mr. Raja Kumar "
self. Bsal = 40000.50
def m1(self ) : #using instance method
self.TA = 2545.80
self.DA = 4000.10
eobj = Emp () # eobj is a referance type object
print ("doc string information", eobj. __doc__)
print ("variable with value in a dict format ", eobj. __dict__ )
eobj . m1 ()
print ("variable with value in a dict format ", eobj. __dict__ )
eobj . ESI = 400 #using referance object
eobj . EPF = 4000
print ("variable with value in a dict format ", eobj. __dict__ )
How to access Instance variables:
We can access instance variables with in the class by using self variable and outside of the class by using object reference.
class Test:
def __init__(self):
self.a=10
self.b=20
def display(self):
print(self.a)
print(self.b)
t=Test()
t.display()
print(t.a,t.b)
EXAMPLE:
class Emp :
''' this class take emp information like empid , empname & Bsal '''
def __init__ (self) : #uisng const
self. empid = 1000
self . empname= "Mr. Raja Kumar "
self. Bsal = 40000.50
def M1 (self) :
self. TA = 2000.10
self. DA = 4000.70
def Display (self) :
print ("address of self", id (self))
print ("Emp id=", self . empid)
print ("Emp Name=", self. empname)
print ("Emp Bsal =", self. Bsal)
print ("Emp TA =", self. TA )
print ("Emp DA =", self. DA )
print ("Emp ESI using referance object =", Eobj . ESI )
print ("Emp ESI using self variable =", self . ESI )
Eobj = Emp ()
Eobj. M1 ()
Eobj . ESI = 400
Eobj. Display ()
print ("address of obj =", id (Eobj))
print ("address of clas =", id (Emp))
print ("outside class ESI =", Eobj. ESI)
How to delete instance variable from the object:
1. Within a class we can delete instance variable as follows
del self.variableName
2. From outside of class we can delete instance variables as follows
del objectreference.variableName
class Test:
def __init__(self):
self.a=10
self.b=20
self.c=30
self.d=40
def m1(self):
del self.d
t=Test()
print(t.__dict__)
t.m1()
print(t.__dict__)
del t.c
print(t.__dict__)
Note:
The instance variables which are deleted from one object, will not be deleted from other objects.
class Test:
def __init__(self):
self.a=10
self.b=20
self.c=30
self.d=40
t1=Test()
t2=Test()
del t1.a
print(t1.__dict__)
print(t2.__dict__)
EXAMPLE:
class Emp :
''' this class take emp information like empid , empname & Bsal '''
def __init__ (self) : #uisng const
self. empid = 1000
self . empname= "Mr. Raja Kumar "
self. Bsal = 40000.50
def M1 (self) :
self. TA = 2000.10
self. DA = 4000.70
def Delete (self) :
del self . empname
del self . DA
del self. ESI
Eobj = Emp ()
Eobj. M1 ()
Eobj . ESI = 400
Eobj . EPF= 4000
Eobj. Delete ()
print (Eobj.__dict__ )
del Eobj . EPF
print (Eobj.__dict__ )
Modify / Update instance Variable :
If we change the values of instance variables of one object then those changes won't be reflected to the remaining objects, because for every object we are separate copy of instance variables are available.
class Test:
def __init__(self):
self.a=10
self.b=20
t1=Test()
t1.a=888
t1.b=999
t2=Test()
print('t1:',t1.a,t1.b)
print('t2:',t2.a,t2.b)
Challenges :
Understanding python databases.
Handling errors.
Resources :
Official Python Documentation: Types of Variables in python
W3Schools' Python Tutorial: Types of Variables in python
Scaler's Python Courses : Types of Variables in python
Goals for Tomorrow :
- Explore something more on Types of Variables in python.
Conclusion :
Day 36’s a success!
What are your favorite Python resources? Share in the comments below.
Connect with me :
GitHub: [ https://github.com/p-archana1 ]
LinkedIn : [ linkedin.com/in/archana-prusty-4aa0b827a ]
twitter : [ https://x.com/_archana77 ]
Join the conversation :
Share your own learning experiences or ask questions in the comments.
HAPPY LEARNING!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.