Setting Up a Simple REST API with Node.js and Express

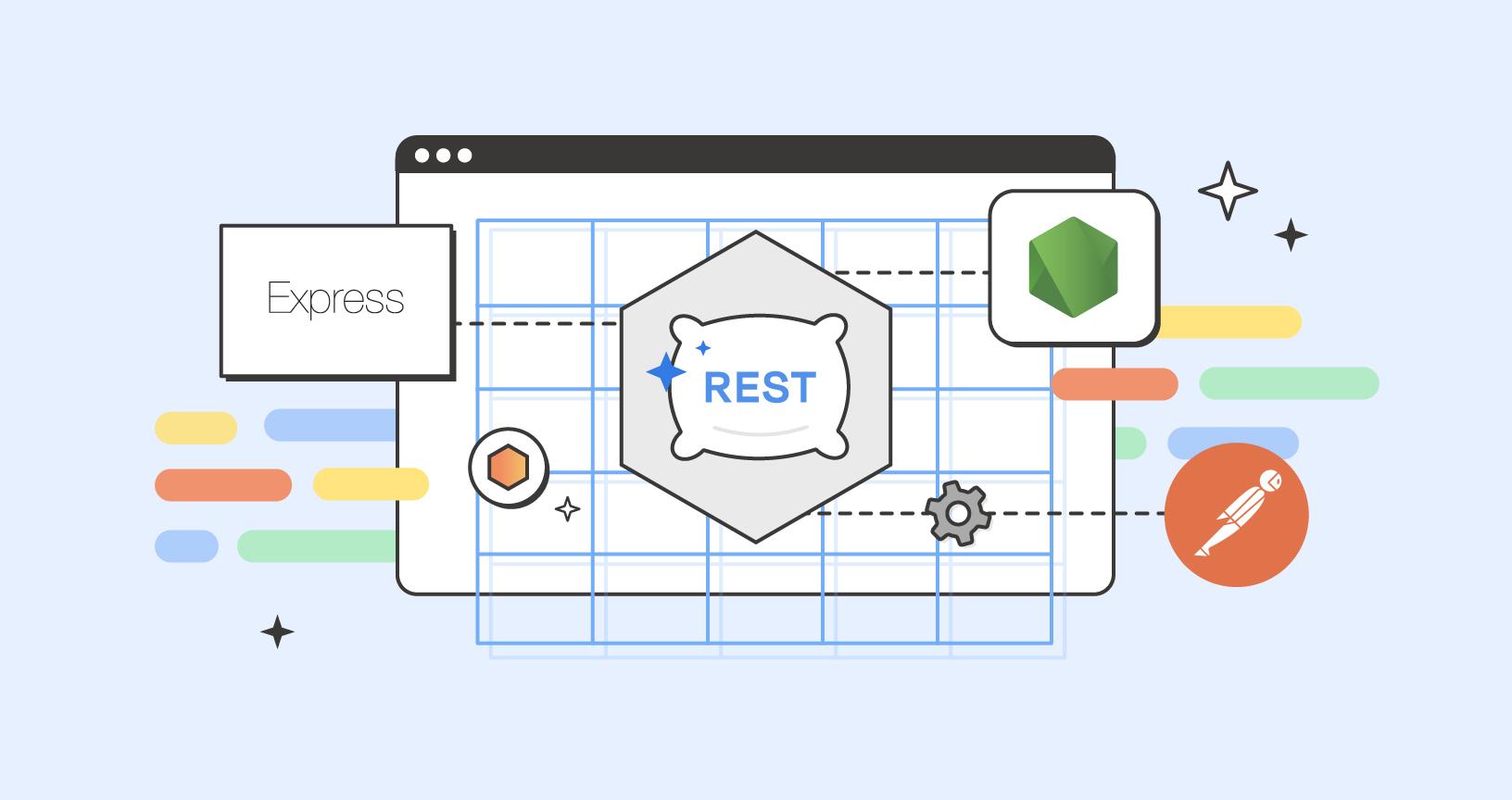
Introduction:
In today’s web development world, REST APIs (Representational State Transfer Application Programming Interfaces) have become essential for building web and mobile applications. They allow different systems to communicate with each other seamlessly. In this article, I’ll walk you through the steps to set up a simple REST API using Node.js and Express.
What is a REST API?
A REST API allows users to interact with your application using HTTP methods (GET, POST, PUT, DELETE). These methods correspond to actions like retrieving, adding, updating, or deleting data. REST APIs typically return data in a format like JSON, making them ideal for use in modern applications.
Tools You Will Need:
Node.js: JavaScript runtime for running server-side code.
Express: A minimal web framework for building APIs and web applications.
Postman or cURL: Tools for testing your API.
Step 1: Setting Up the Environment
Install Node.js: First, make sure you have Node.js installed. You can download it from Node.js Official Website.
Initialize a Node.js Project: Open your terminal, create a new folder for your project, and run the following commands:
mkdir rest-api
cd rest-api
npm init -y
This will initialize a new Node.js project and create a package.json
file.
- Install Express: In your project directory, run:
npm install express
This will install the Express framework.
Step 2: Setting Up the Express Server
- Create a Simple Express Server: In your project directory, create a file called
app.js
. This file will contain the code for your server.
const express = require('express');
const app = express();
const PORT = 3000;
app.use(express.json()); // Middleware to parse incoming JSON requests
app.get('/', (req, res) => {
res.send('Welcome to my REST API!');
});
app.listen(PORT, () => {
console.log(Server running on http://localhost:${PORT});
});
- Start Your Server: In the terminal, run the following command to start your server:
node app.js
You should see the message “Server running on http://localhost:3000”. Open your browser and navigate to http://localhost:3000
, and you should see “Welcome to my REST API!”.
Step 3: Defining API Endpoints
Now, let’s define some basic CRUD operations (Create, Read, Update, Delete) using HTTP methods:
- GET Request – Fetch Data: Add this route to
app.js
to return a list of items:
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
];
app.get('/items', (req, res) => {
res.json(items);
});
- POST Request – Add a New Item: To allow adding new items, add a
POST
route:
app.post('/items', (req, res) => {
const newItem = {
id: items.length + 1,
name: req.body.name,
};
items.push(newItem);
res.status(201).json(newItem);
});
- PUT Request – Update an Existing Item: You can update an item using its
id
with the following code:
app.put('/items/:id', (req, res) => {
const id = parseInt(req.params.id);
const item = items.find(i => i.id === id);
if (item) {
item.name = req.body.name;
res.json(item);
} else {
res.status(404).json({ message: 'Item not found' });
}
});
- DELETE Request – Delete an Item: Finally, add a route to delete an item based on its
id
:
app.delete('/items/:id', (req, res) => {
const id = parseInt(req.params.id);
const index = items.findIndex(i => i.id === id);
if (index !== -1) {
items.splice(index, 1);
res.status(204).send(); // No content response
} else {
res.status(404).json({ message: 'Item not found' });
}
});
Step 4: Testing the API
You can use Postman or cURL to test your API. For instance:
To get all items, send a
GET
request tohttp://localhost:3000/items
.To add a new item, send a
POST
request tohttp://localhost:3000/items
with a JSON body like:
{
"name": "New Item"
}
- To update an item, send a
PUT
request tohttp://localhost:3000/items/1
with a JSON body like:
{
"name": "Updated Item"
}
- To delete an item, send a
DELETE
request tohttp://localhost:3000/items/1
.
Step 5: Handling Errors and Improvements
To make your API more robust, you can add error handling, validate user inputs, and structure your code better by separating routes into different files.
For example, use the middleware to handle errors:
app.use((err, req, res, next) => {
res.status(500).json({ message: err.message });
});
Conclusion:
With just a few lines of code, you’ve created a simple REST API using Node.js and Express! You can now build more complex APIs by adding authentication, connecting to databases, and handling different types of requests.
Feel free to experiment with more features, such as connecting your API to a database (e.g., MongoDB or PostgreSQL), adding user authentication, or creating more complex routes.
Happy coding!
Subscribe to my newsletter
Read articles from Likhith SP directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Likhith SP
Likhith SP
My mission is to help beginners in technology by creating easy-to-follow guides that break down complicated operations, installations, and emerging technologies into bite-sized steps.