Promise.race

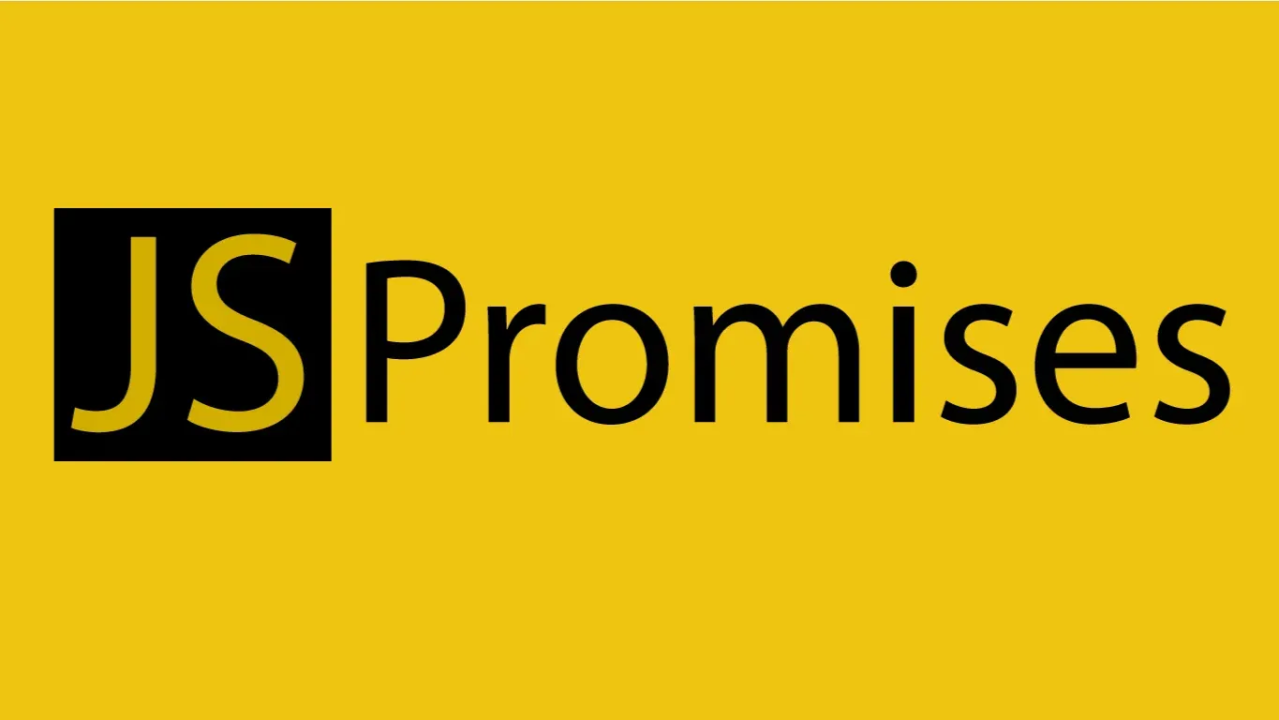
Promise.race
: Let’s See Who Wins the Asynchronous Race! 🏁
Promises in JavaScript are like marathon runners. They all start at the same time, but some are faster, some slower, and some just... don’t finish (we’ll get to that). But what if you only care about the fastest runner? That’s where Promise.race
comes in!
In this blog, we’ll break down Promise.race
, how it works, and why it’s perfect for situations where speed is everything. Plus, we’ll have fun with examples using both async/await
and good old .then()
.
What is Promise.race
?
Think of Promise.race
like a 100-meter dash. You throw a bunch of promises on the track, and the one that finishes first—whether it resolves successfully or crashes and burns—determines the result of the race.
Quick breakdown:
Promise.race
takes an array of promises.It resolves or rejects as soon as the first promise settles.
Doesn’t care if the others are still running—they’re ignored once the race has a winner.
Why Use Promise.race
?
Let’s say you’re fetching data from three different servers, but you only care about the one that responds the fastest. Maybe one is in Australia, one in Korea, and one in Somalia (and we all know network speeds can vary!).
Instead of waiting for all of them like a helicopter parent (looking at you, Promise.all
), Promise.race
lets you take the first result and move on with your life. 🏃♀️
How to use Promise.race
?
Here’s how you can use Promise.race
in both async/await
style and .then()
style.
Example 1: Using Promise.race
with async/await
const racePromises = async function () {
try {
const fastestResponse = await Promise.race([
fetch(`https://restcountries.com/v3.1/name/${'Australia'}`),
fetch(`https://restcountries.com/v3.1/name/${'Korea'}`),
fetch(`https://restcountries.com/v3.1/name/${'Somalia'}`),
]);
const data = await fastestResponse.json();
console.log('Fastest country data:', data);
return data;
} catch (error) {
console.error('The race failed:', error);
}
};
racePromises();
What’s Happening Here?
Promise.race
starts the race between three fetch requests.As soon as the fastest request resolves, the race is over.
We grab the winner’s response, parse the JSON, and log the data.
If something goes wrong (e.g., all requests fail), we catch the error.
Example 2: Using Promise.race
with .then()
Promise.race([
fetch(`https://restcountries.com/v3.1/name/${'Australia'}`),
fetch(`https://restcountries.com/v3.1/name/${'Korea'}`),
fetch(`https://restcountries.com/v3.1/name/${'Somalia'}`),
])
.then((res) => res.json())
.then((data) => console.log('Fastest country data:', data))
.catch((error) => console.error('The race failed:', error));
Same concept, just with chaining. Easy peasy!
When to Use Promise.race
Here are a few scenarios where Promise.race
shines:
Fastest Response Wins:
- Fetching from multiple servers and using the one that responds first.
Timeout Handling:
- Want to avoid waiting forever for a slow request? You can race a
fetch
call against a custom timeout promise!
- Want to avoid waiting forever for a slow request? You can race a
const timeout = new Promise((_, reject) =>
setTimeout(() => reject(new Error('Request timed out!')), 5000)
);
Promise.race([
fetch('https://restcountries.com/v3.1/name/Australia'),
timeout,
])
.then((res) => res.json())
.then((data) => console.log('Data:', data))
.catch((error) => console.error('Error:', error));
If the fetch doesn’t complete within 5 seconds, the timeout promise wins the race, and you get a timeout error.
The Catch (Pun Intended) 🐟
Here’s the thing: Promise.race
doesn’t guarantee a happy ending. If the first promise to settle is a rejection, the race resolves with an error. Think of it as betting on a racehorse that trips over its own hooves 🏇
A Quick Joke to Keep It Light
Why don’t promises ever get married? 🤔
Because they reject too often. 😅
Final Thoughts
Promise.race
is your go-to tool when speed is the name of the game. Whether you’re fetching data from multiple sources, implementing timeouts, or just want to see which server responds fastest, it’s a powerful method to have in your JavaScript toolkit.
Just remember: with great speed comes great responsibility. Handle errors wisely, and your code will be the Usain Bolt of asynchronous operations. 🚀
That’s it for today! Let me know your thoughts in the comments or share your coolest Promise.race
use cases. Happy coding, and may the fastest promise win! 🏆
Subscribe to my newsletter
Read articles from Hassani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hassani
Hassani
Hi 👋 I'm a developer with experience in building websites with beautiful designs and user friendly interfaces. I'm experienced in front-end development using languages such as Html5, Css3, TailwindCss, Javascript, Typescript, react.js & redux and NextJs 🚀