Promise.allSettled

Table of contents
- Promise.allSettled: When You Care About Everyone's Story
- What is Promise.allSettled?
- Why Use Promise.allSettled?
- How to use Promise.allSettled !
- What’s Happening Here?
- Note: Customize the Logic as You Like! 🚀
- When to Use Promise.allSettled?
- But Why Not Just Use Promise.all?
- A Quick Joke to Lighten the Mood
- Final Thoughts
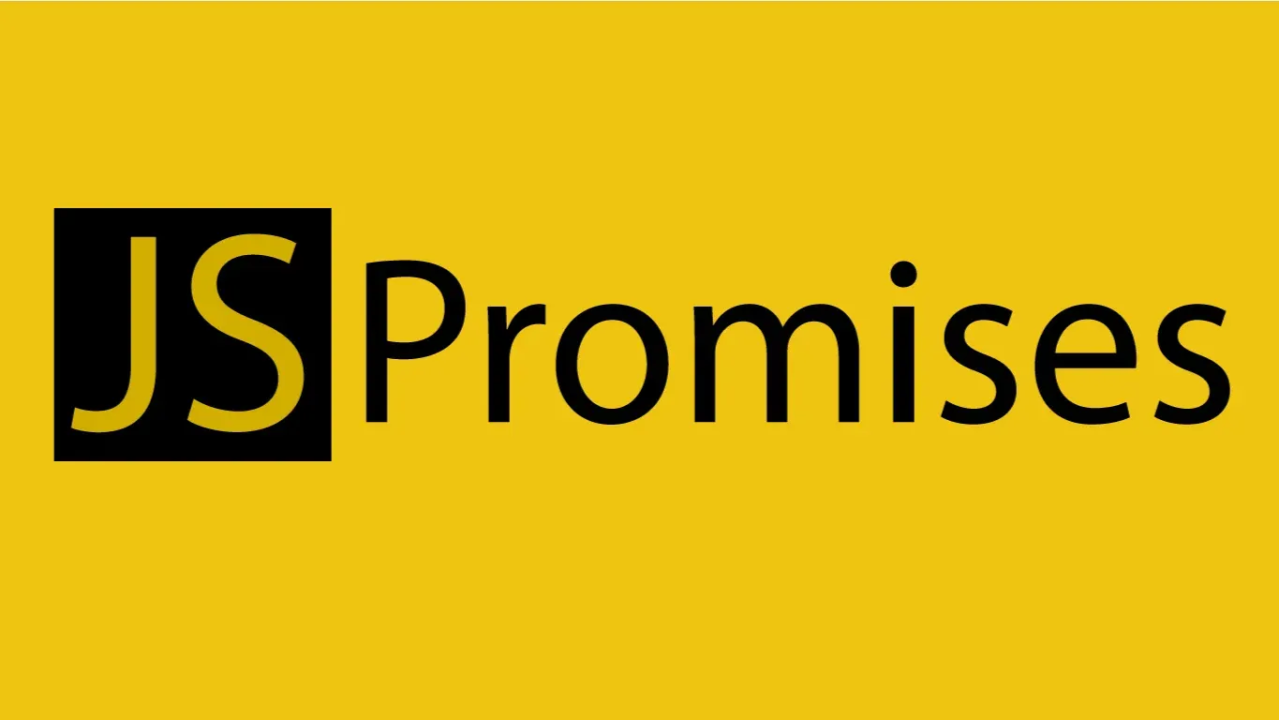
Promise.allSettled
: When You Care About Everyone's Story
Promises in JavaScript are like a group of friends—you’ve got the overachiever who gets everything done on time, the procrastinator who never finishes, and the drama queen who fails spectacularly. Sometimes, you only care about the ones who succeed (Promise.all
), or maybe you’re just looking for the fastest (Promise.race
). But what if you care about everyone's story, no matter how it ends? Enter: Promise.allSettled
.
In this blog, we’ll dive into what Promise.allSettled
does, why it’s your best friend for handling mixed outcomes, and how to use it with examples. And, of course, a bit of humor to keep things lively! 🎉
What is Promise.allSettled
?
Promise.allSettled
is like a therapist for promises—it listens to everyone and takes notes, regardless of whether they succeeded or failed.
Here’s how it works:
It takes an array of promises and waits for all of them to settle.
It returns an array of objects, each describing the outcome of a promise:
{ status: 'fulfilled', value: ... }
if the promise resolved successfully.{ status: 'rejected', reason: ... }
if the promise failed.
Unlike Promise.all
, it doesn’t stop or throw an error if one promise fails. Everyone gets a chance to be heard!
Why Use Promise.allSettled
?
Let’s say you’re fetching data from three APIs. One might succeed, another might fail, and the third might be slow but eventually succeed. You don’t want the whole operation to blow up just because one request failed, right? Promise.allSettled
ensures you get a complete picture.
How to use Promise.allSettled
!
Here’s how you can use Promise.allSettled
with both async/await
and .then()
.
Example 1: Using Promise.allSettled
with async/await
const fetchAllSettled = async function () {
const results = await Promise.allSettled([
fetch(`https://restcountries.com/v3.1/name/${'Australia'}`),
fetch(`https://restcountries.com/v3.1/name/${'Korea'}`),
fetch(`https://restcountries.com/v3.1/name/${'NonExistentCountry'}`), // This will fail
]);
results.forEach((result, index) => {
if (result.status === 'fulfilled') {
console.log(`Promise ${index + 1} fulfilled:`, result.value);
} else {
console.error(`Promise ${index + 1} rejected:`, result.reason);
}
});
return results;
};
fetchAllSettled();
What’s Happening Here?
We fire off three fetch requests.
Promise.allSettled
waits for all of them to settle, regardless of whether they succeed or fail.We loop through the results:
Log the successful ones.
Handle the failures gracefully without crashing the app.
Example 2: Using .then()
Promise.allSettled([
fetch(`https://restcountries.com/v3.1/name/${'Australia'}`),
fetch(`https://restcountries.com/v3.1/name/${'Korea'}`),
fetch(`https://restcountries.com/v3.1/name/${'NonExistentCountry'}`), // This will fail
])
.then((results) => {
results.forEach((result, index) => {
if (result.status === 'fulfilled') {
console.log(`Promise ${index + 1} fulfilled:`, result.value);
} else {
console.error(`Promise ${index + 1} rejected:`, result.reason);
}
});
});
Note: Customize the Logic as You Like! 🚀
Feel free to replace the if-else
statements in our examples with a ternary operator if you prefer a more concise approach. Here's a quick example:
results.forEach((result, index) => {
result.status === 'fulfilled'
? console.log(`Promise ${index + 1} fulfilled:`, result.value)
: console.error(`Promise ${index + 1} rejected:`, result.reason);
});
When to Use Promise.allSettled
?
Here are some real-world scenarios where Promise.allSettled
is a lifesaver:
Fetching from Multiple APIs:
- You want to show whatever data you can, even if some APIs fail.
Handling Mixed Outcomes:
- Maybe you’re uploading multiple files and some succeed while others fail. You don’t want to retry all just because a few failed.
Batch Processing:
- You’re running operations like reading files, sending notifications, or querying databases. Some tasks might succeed, others might not, but you want a report of everything.
But Why Not Just Use Promise.all
?
Great question! Here’s the difference:
Promise.all
: Stops and throws an error if any promise fails. It’s an all-or-nothing approach.Promise.allSettled
: Reports back on every promise, whether it succeeds or fails. Perfect when failure is an option.
A Quick Joke to Lighten the Mood
Why did the promise go to therapy? 🤔
Because it had too many unresolved issues. 😅
Final Thoughts
Promise.allSettled
is the friend you didn’t know you needed. It lets you handle multiple asynchronous operations with grace, ensuring that failures don’t bring down the whole operation. Whether you’re building resilient APIs, handling user uploads, or just want to know the full story of your asynchronous journey, this method has your back.
So, the next time you’ve got a group of promises and need a complete picture, let Promise.allSettled
do its thing. Happy coding, and may all your promises be settled peacefully! 🚀
That’s all for today! Got cool use cases for Promise.allSettled
? Drop them in the comments. Let’s learn together! 👩💻👨💻
Subscribe to my newsletter
Read articles from Oohnohassani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Oohnohassani
Oohnohassani
Hi 👋 I'm a developer with experience in building websites with beautiful designs and user friendly interfaces. I'm experienced in front-end development using languages such as Html5, Css3, TailwindCss, Javascript, Typescript, react.js & redux and NextJs 🚀