Promise.any

Table of contents
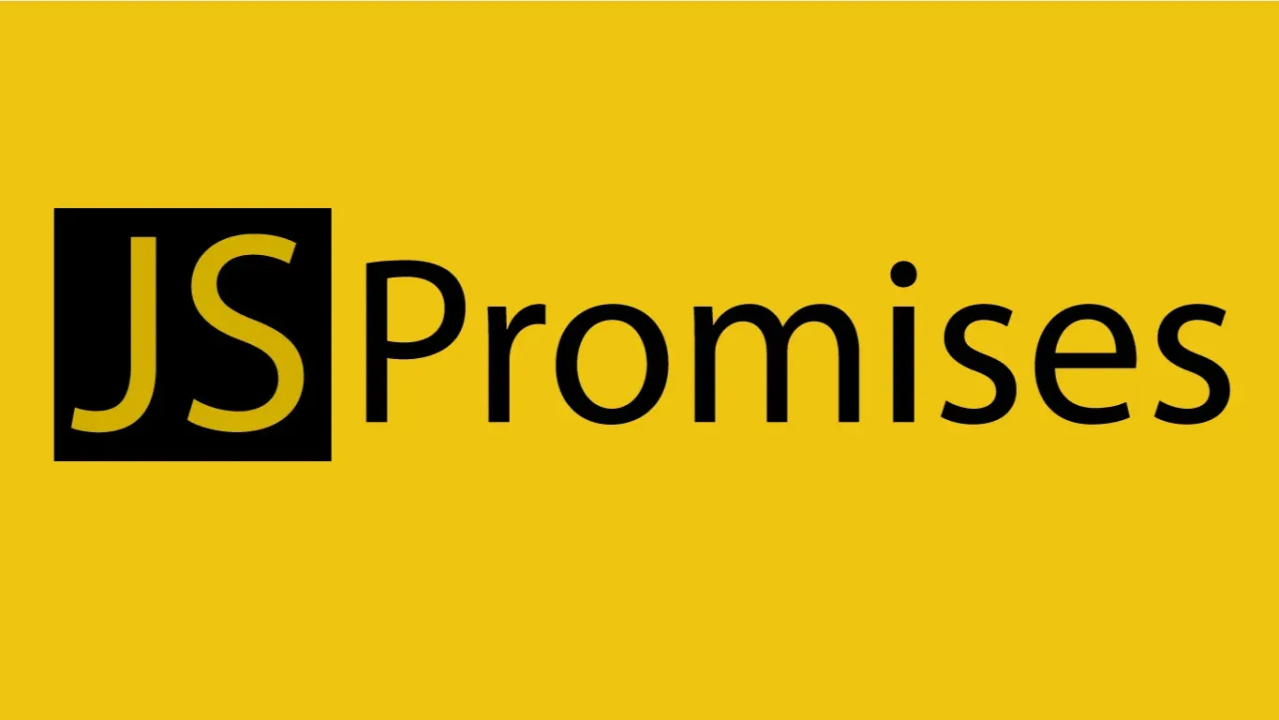
Promise.any
: The Race of the Fittest (First to Resolve Wins)
Promises, promises, promises! JavaScript has a few different ways to handle them, but what if you only care about the first promise to succeed?
Enter: Promise.any
—a superhero for when you're okay with failures, as long as one promise makes it across the finish line!
In this blog, we’re going to dive into what Promise.any
is, how it works, and when you’d want to use it. Don’t worry, we’ll keep it light, fun, and packed with examples so you can add this to your developer toolkit!
What is Promise.any
?
Think of Promise.any
as a race between promises. You set off a bunch of promises, and you don’t care if some fail along the way. You only care about the first promise that resolves (not rejects). Once that happens, the race is over, and you get the result of that promise.
Here’s how it works:
Promise.any
takes an array of promises.It resolves as soon as one promise is fulfilled.
If all promises are rejected, it returns an aggregate error with all the rejection reasons.
It’s like entering a race, but you’re not judging everyone. You just want to see who finishes first. 🏁
Why Use Promise.any
?
Let’s say you’re trying to fetch data from multiple sources, and you’re okay with getting the data from whichever source responds first, even if others fail or are slow. This is where Promise.any
comes in handy.
For example, you could be trying to fetch data from three servers: one in the U.S., one in Korea, and one in Africa. The first one to respond gives you the data, and you move on with your life. You don’t need to wait for the other servers!
How to use Promise.any
?
Let’s take a look at how Promise.any
works in both async/await
and the classic .then()
style.
Example 1: Using Promise.any
with async/await
const fetchData = async function () {
try {
const data = await Promise.any([
fetch(`https://restcountries.com/v3.1/name/${'Australia'}`),
fetch(`https://restcountries.com/v3.1/name/${'Korea'}`),
fetch(`https://restcountries.com/v3.1/name/${'Somalia'}`),
]);
const result = await data.json();
console.log('First to resolve:', result);
return result;
} catch (error) {
console.error('All promises were rejected:', error);
}
};
fetchData();
What’s Happening Here?
We fire off three
fetch
requests simultaneously.Promise.any
waits for the first one to resolve. If that happens, we immediately get the result.If all promises reject,
catch
catches the rejection and tells us what went wrong.
Example 2: Using .then()
Promise.any([
fetch(`https://restcountries.com/v3.1/name/${'Australia'}`),
fetch(`https://restcountries.com/v3.1/name/${'Korea'}`),
fetch(`https://restcountries.com/v3.1/name/${'Somalia'}`),
])
.then((data) => data.json())
.then((result) => {
console.log('First to resolve:', result);
})
.catch((error) => {
console.error('All promises were rejected:', error);
});
When to Use Promise.any
?
Here are some scenarios where Promise.any
is perfect:
Fastest API Response Wins:
- You’re hitting multiple servers or APIs and only care about the first successful response.
Handling Multiple Fallbacks:
- You want to provide users with the first available source, even if others are slow or unavailable.
Timeouts and Retries:
- Use
Promise.any
with a timeout promise to get the result of whichever action happens first—either success or timeout.
- Use
Difference Between Promise.any
and Promise.race
Promise.any
: Resolves when the first successful promise resolves. If all promises fail, it rejects with anAggregateError
.Promise.race
: Resolves or rejects as soon as any promise settles (either resolves or rejects). The first promise to settle wins, regardless of whether it's fulfilled or rejected.
In short:
Promise.any
cares only about success.Promise.race
cares about the first settlement (success or failure).
The Catch: All Promises Must Not Reject
If all promises reject, Promise.any
will throw an AggregateError
containing all the rejection reasons. So, don’t forget to handle errors, or you’ll get an uncaught rejection!
Quick Joke for the Road
Why did the promise break up with the async function? 🤔
Because it felt like it was always getting rejected. 😅
Final Thoughts
Promise.any
is a powerful tool when you only care about the first promise that resolves. It’s like a race, where only the winner gets the gold, and the losers are left behind. Perfect for scenarios where speed matters, but you’re okay with some potential failures along the way.
Just make sure to handle those rejection cases gracefully, and you’ll have a smooth race every time!
That’s all for today! Got any fun use cases for Promise.any
? Share them in the comments, and let’s learn together. Happy coding! 🏆
Subscribe to my newsletter
Read articles from Oohnohassani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Oohnohassani
Oohnohassani
Hi 👋 I'm a developer with experience in building websites with beautiful designs and user friendly interfaces. I'm experienced in front-end development using languages such as Html5, Css3, TailwindCss, Javascript, Typescript, react.js & redux and NextJs 🚀