Network Security in Android: A Comprehensive Guide [PART 4]
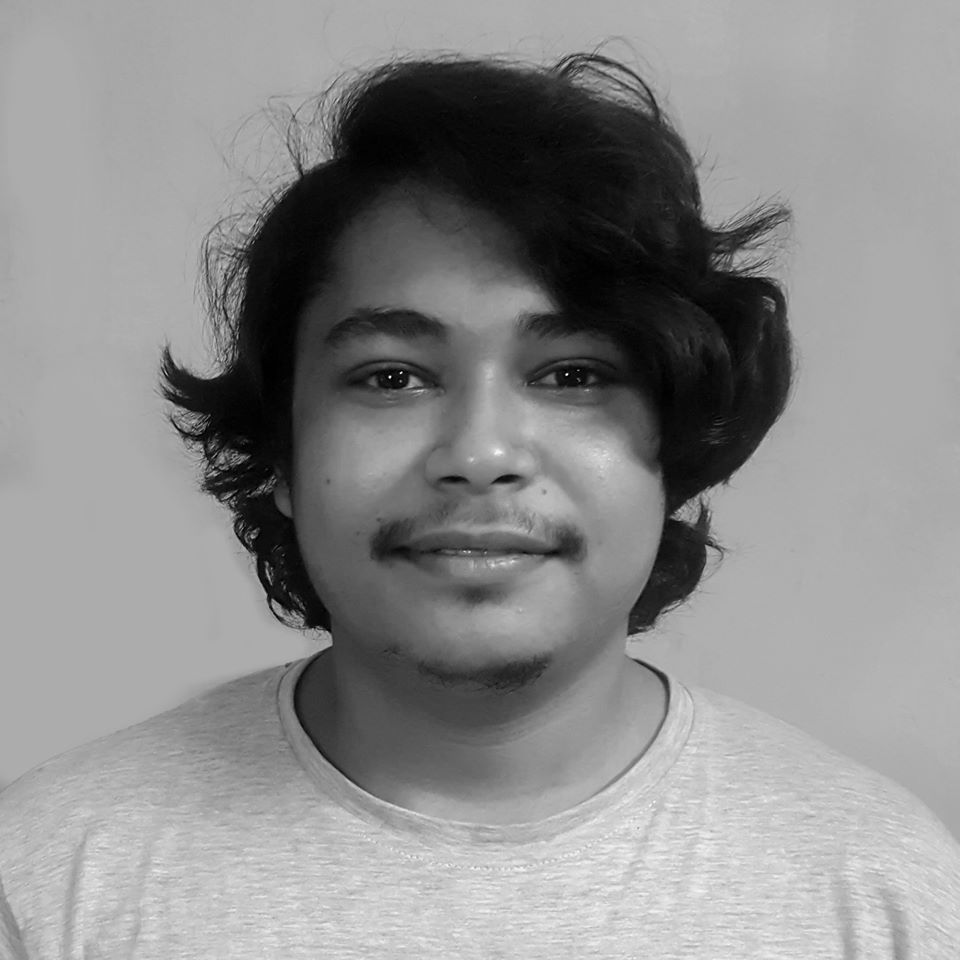
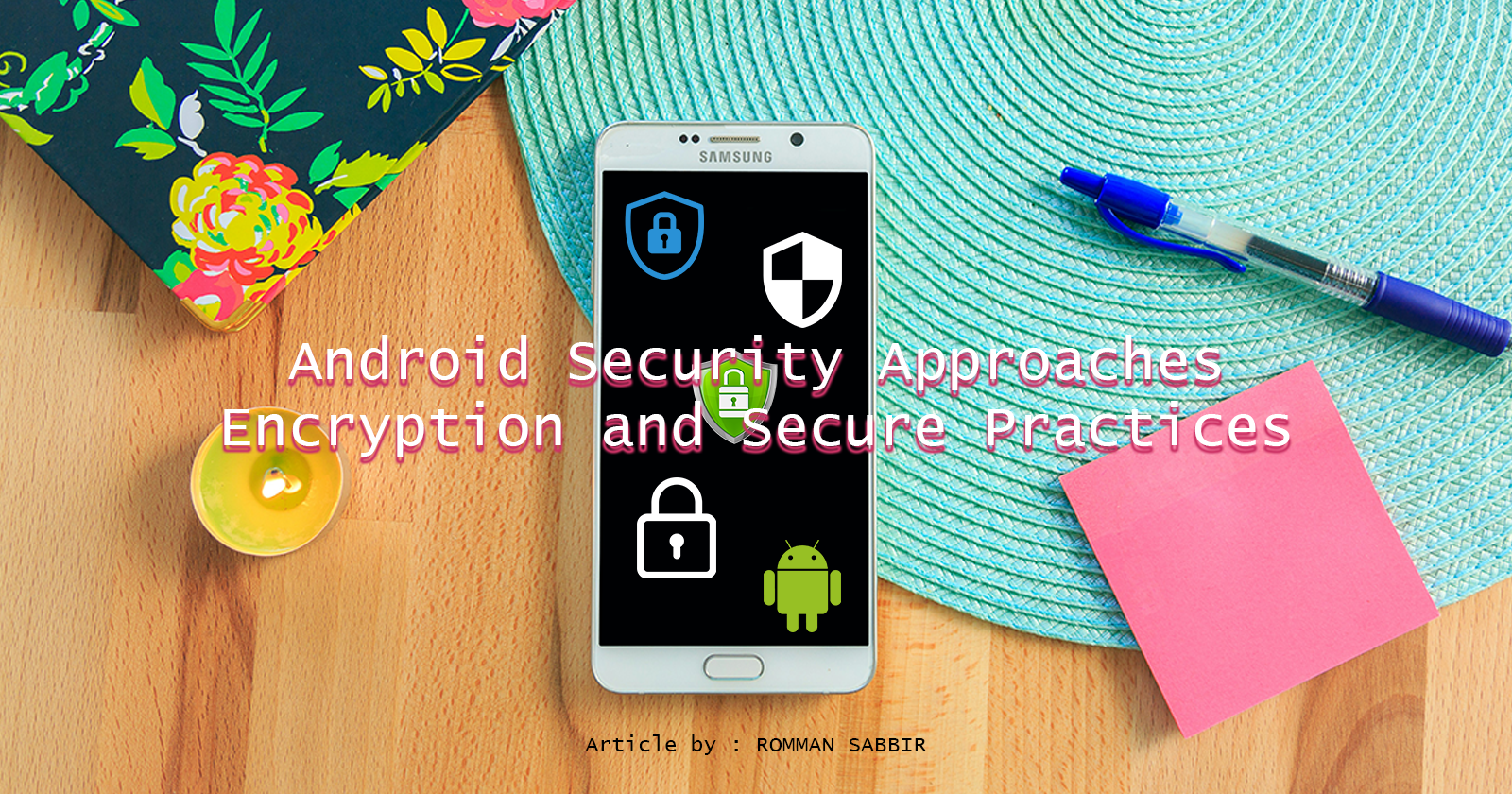
Network security is critical for protecting the communication between your Android app and remote servers. Without proper security measures, sensitive data like user credentials, personal information, or financial details can be intercepted, altered, or stolen. This article covers key strategies for securing network communications, including Transport Layer Security (TLS), Certificate Pinning, VPN Support, and Firewall & Intrusion Detection System (IDS) Integration. Each section includes explanations, real-life use cases, and Kotlin examples to help you implement these practices in your Android applications.
TLS (Transport Layer Security): Encrypted Communication Over the Network
TLS (formerly known as SSL) is a cryptographic protocol that ensures secure data transmission over the internet. It encrypts the data being transferred, preventing unauthorized access or tampering by third parties. TLS is a standard security protocol that every Android app should implement to secure communication between the client (app) and the server.
Use Case: Encrypted Data Transmission
When an Android app sends sensitive data, such as login credentials or user information, over the network, using TLS ensures that the data is encrypted and secure. This prevents attackers from reading or tampering with the information as it travels across the network.
Kotlin Example: Enforcing TLS/SSL for Network Requests
Android’s OkHttp library supports TLS out of the box. You can configure it to enforce secure communication by ensuring that the connection uses HTTPS (which runs on top of TLS).
- Add the OkHttp dependency to your
build.gradle
file:
implementation("com.squareup.okhttp3:okhttp:4.9.1")
- Configure OkHttp to make a secure request:
import okhttp3.OkHttpClient
import okhttp3.Request
import java.io.IOException
fun makeSecureRequest() {
val client = OkHttpClient()
val request = Request.Builder()
.url("https://yourserver.com/api")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
// Handle the secure response
}
}
In this example, OkHttp automatically negotiates the TLS handshake when connecting to the server via HTTPS. If the server doesn’t support TLS, the request will fail, ensuring that the communication is secure.
Real-Life Example: Secure API Communication
Most mobile apps that communicate with a backend API use TLS to encrypt data. For example, a health app that transmits medical records or a banking app transferring financial data must use TLS to ensure that sensitive information is not intercepted or altered during transit.
Certificate Pinning: Preventing Man-in-the-Middle Attacks
Certificate Pinning is a security technique that helps prevent man-in-the-middle (MITM) attacks by ensuring that the app communicates only with trusted servers using a specific SSL certificate. Instead of trusting any certificate issued by a valid certificate authority (CA), certificate pinning allows you to pin your app to a specific certificate or public key, ensuring that the communication is with a legitimate server and has not been tampered with.
Use Case: Securing Server Communication
An app that handles sensitive data, such as financial transactions, must ensure that it is communicating with the intended server. Certificate pinning prevents attackers from intercepting traffic and spoofing the server by using fraudulent certificates.
Kotlin Example: Implementing Certificate Pinning with OkHttp
First, get the public key or certificate that you want to pin. In this example, we’ll pin the public key of the server’s SSL certificate.
Configure OkHttp with certificate pinning:
import okhttp3.CertificatePinner
import okhttp3.OkHttpClient
import okhttp3.Request
fun setupCertificatePinning() {
val certificatePinner = CertificatePinner.Builder()
.add("yourserver.com", "sha256/AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA=")
.build()
val client = OkHttpClient.Builder()
.certificatePinner(certificatePinner)
.build()
val request = Request.Builder()
.url("https://yourserver.com/api")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
// Handle the response
}
}
In this example, CertificatePinner is used to enforce that only a specific certificate with the provided SHA-256 public key hash is accepted when connecting to the server.
Real-Life Example: Secure Banking App
A banking app that communicates with a remote server to handle user transactions should implement certificate pinning to ensure that the connection cannot be intercepted by an attacker using a fraudulent certificate. This adds an extra layer of security to the communication between the app and the server.
VPN Support: Secure Network Communication
VPNs (Virtual Private Networks) provide a secure and encrypted connection over a less secure network, such as the internet. VPNs are often used to protect sensitive data and prevent unauthorized access to network traffic. Implementing VPN support in your app or working with VPN providers can ensure that all network communication is encrypted and secure.
Use Case: Enhancing Security in Public Networks
Users who connect to your app over public Wi-Fi networks are vulnerable to attacks like packet sniffing or man-in-the-middle attacks. VPNs create a secure tunnel for communication, ensuring that data remains private even on insecure networks.
Kotlin Example: Launching a VPN Service in Android
Android provides built-in support for creating VPN connections via the VpnService class. Below is a basic example of how to start a VPN service in your Android app.
- Create a VPN Service by extending
VpnService
:
import android.app.Service
import android.content.Intent
import android.net.VpnService
class MyVpnService : VpnService() {
override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int {
// Setup VPN connection here
return Service.START_STICKY
}
override fun onDestroy() {
super.onDestroy()
// Clean up VPN connection
}
}
- Request VPN permissions in your
AndroidManifest.xml
:
<service android:name=".MyVpnService"
android:permission="android.permission.BIND_VPN_SERVICE">
<intent-filter>
<action android:name="android.net.VpnService" />
</intent-filter>
</service>
- Launch the VPN service from your app:
val vpnIntent = VpnService.prepare(this)
if (vpnIntent != null) {
startActivityForResult(vpnIntent, VPN_REQUEST_CODE)
} else {
// Already prepared, start VPN service directly
startService(Intent(this, MyVpnService::class.java))
}
In this example, VpnService is used to create a secure VPN connection. The VPN service can be configured to tunnel all network traffic securely.
Real-Life Example: Privacy-Focused Apps
Apps focused on privacy, like secure messaging or financial apps, can benefit from integrating VPN support to ensure that user data remains private, even when users are connected to insecure public networks.
Firewall & IDS Integration: Traffic Filtering and Intrusion Detection
A Firewall is a network security system that monitors and controls incoming and outgoing traffic based on predefined security rules. An Intrusion Detection System (IDS) helps detect potential malicious activities or policy violations within a network. Combining firewall rules and IDS can significantly enhance the security of your app by filtering out unwanted traffic and detecting suspicious network activities.
Use Case: Blocking Malicious Traffic
By using firewall rules, your app can restrict access to certain IP addresses or domains, preventing malicious traffic from reaching your app. Additionally, integrating an IDS can help detect and alert suspicious activities, such as DDoS attacks or unauthorized access attempts.
Firewall Example: Creating Traffic Filtering Rules
While Android does not provide built-in APIs for configuring firewalls directly, you can create custom network policies in your backend or use a third-party service to filter network traffic. Here is an example of creating firewall-like rules at the application level:
import okhttp3.Interceptor
import okhttp3.OkHttpClient
import okhttp3.Request
fun setupNetworkFiltering() {
val networkInterceptor = Interceptor { chain ->
val request: Request = chain.request()
// Example: Block requests to a specific domain
if (request.url.host == "malicious.com") {
throw IOException("Blocked by firewall")
}
return@Interceptor chain.proceed(request)
}
val client = OkHttpClient.Builder()
.addInterceptor(networkInterceptor)
.build()
}
This code snippet demonstrates how to block network requests to a specific domain at the app level using OkHttp’s Interceptor.
Real-Life Example: Securing APIs
An enterprise app that accesses sensitive data might have firewall rules in place to restrict access to only known IP addresses or domains. For example, an internal business app might restrict access to only trusted company networks, while blocking external traffic.
Conclusion
Network security is a critical component of any Android application, especially those that handle sensitive user data or perform financial transactions. TLS ensures encrypted communication between the app and the server, while Certificate Pinning prevents man-in-the-middle attacks. VPN Support can help secure network communication on public networks, and integrating Firewall & IDS provides an additional layer of protection by filtering traffic and detecting malicious activities.
By implementing these network security measures, you can ensure that your Android app remains secure and that sensitive user data is protected from potential threats.
That’s it for today. Happy coding…
Subscribe to my newsletter
Read articles from Romman Sabbir directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
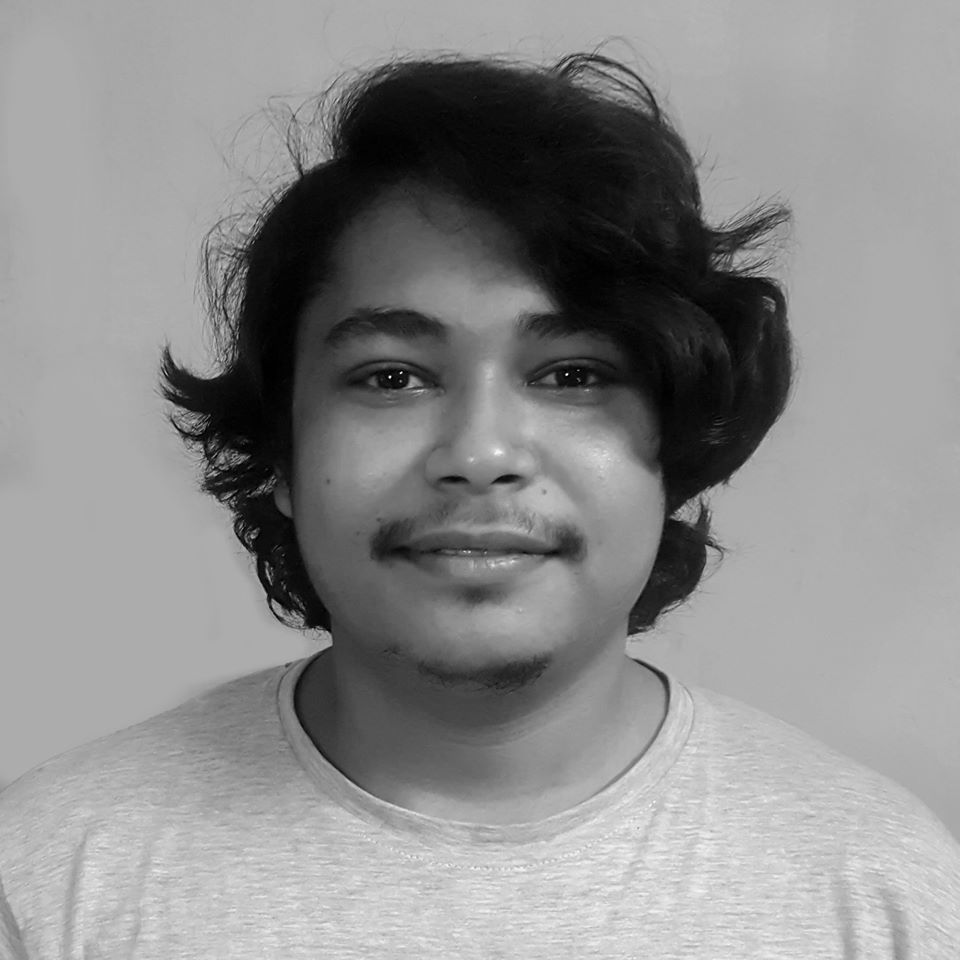
Romman Sabbir
Romman Sabbir
Senior Android Engineer from Bangladesh. Love to contribute in Open-Source. Indie Music Producer.