Essential Tips for Securing Your Laravel 11 App from DDoS Attacks

Table of contents
- What Is a DDoS Attack?
- 1. Login Form Protection: Using Rate Limiting and CAPTCHA
- 2. Registration Form Protection: IP Blocking and Honeypots
- 3. API Endpoints: Throttle Requests and Require Tokens
- 4. Checkout and Payment Pages: Web Application Firewalls and Queueing
- 5. Interactive Forms (e.g. Feedback Forms): Adding Delays and Limiting Data Size
- 6. Monitor Activity with Real-Time Alerts
- Conclusion
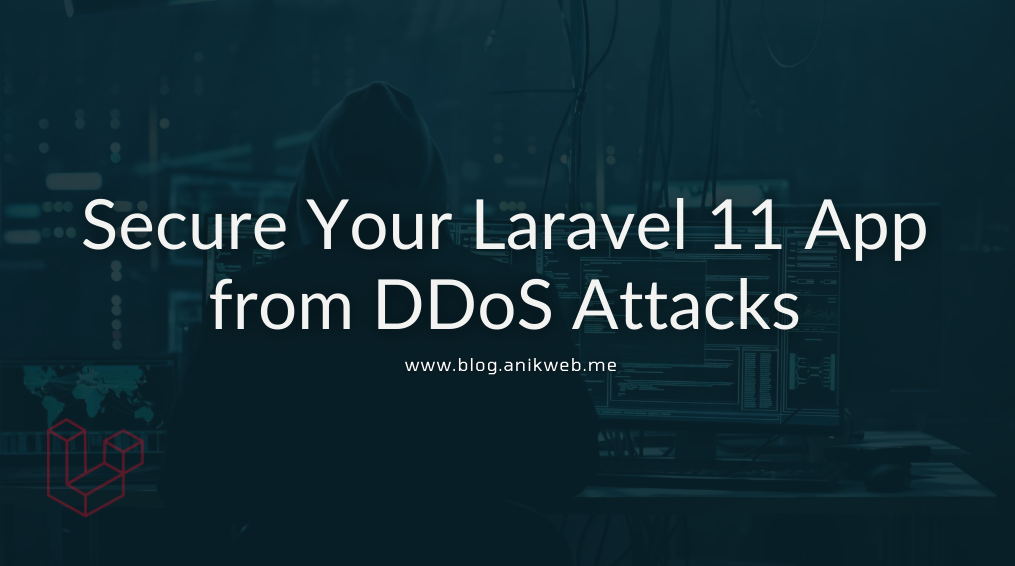
What Is a DDoS Attack?
A DDoS attack happens when lots of computers flood a server with too much traffic, aiming to slow it down or even knock it offline. It's like if hundreds of people tried to rush into a store all at once, causing a big jam that stops anyone from getting in normally. In a Laravel app, DDoS attacks might focus on pages like login, registration, and API endpoints, overwhelming them and possibly crashing the app.
To protect against these attacks, you'll want to make it tougher for bad traffic to hit these points without messing up the experience for real users. Let's explore some simple techniques you can use in Laravel.
1. Login Form Protection: Using Rate Limiting and CAPTCHA
The login form is one of the most at-risk areas because attackers might try to break into user accounts or flood the server with too many login attempts. Here's how you can keep it safe:
Step 1: Apply Rate Limiting
Laravel has a built-in feature to limit the number of requests that any user can make within a certain time frame. Adding rate limiting to the login route restricts the number of login attempts, helping prevent attacks.
Route::post('/login', [AuthController::class, 'login'])->middleware('throttle:5,1');
This line limits users to five login attempts per minute. If they exceed this limit, Laravel will return an error response, which slows down potential attackers.
Step 2: Add CAPTCHA Verification
CAPTCHA is a simple challenge (like clicking on images or typing a code) that humans can easily complete, but bots usually can't. By adding CAPTCHA to the login form, you make sure that only real users can get through.
- Google reCAPTCHA is a popular choice and it's free to use. Just register your site on the Google reCAPTCHA website, add the CAPTCHA keys to your
.env
file, and include the CAPTCHA code in your login form.
Example: If an attacker tries to overwhelm your login form with bot traffic, rate limiting will slow down their requests, and the CAPTCHA will stop automated bots from completing the form.
2. Registration Form Protection: IP Blocking and Honeypots
If you allow new users to register, this form can also become a target. Attackers may attempt to create fake accounts, which could drain resources or manipulate referral programs.
Step 1: Block Suspicious IPs and Apply Rate Limits
Just like in the login form, limit how often a single IP can submit the registration form.
Route::post('/register', [RegistrationController::class, 'register'])->middleware('throttle:3,60');
This limits each IP to three registrations per hour.
Step 2: Use a Honeypot Field
A honeypot is a hidden field in your form. Real users won’t fill it out since it’s hidden, but bots tend to fill in all form fields automatically. If this hidden field is filled out, you know it’s likely a bot.
To add a honeypot in Laravel, use the spatie/laravel-honeypot
package. Here’s how:
Run
composer require spatie/laravel-honeypot
to install the package.Add the
@honeypot
directive to your form:<form method="POST" action="/register"> @csrf @honeypot <!-- other form fields here --> </form>
Example: If a bot tries to create hundreds of fake accounts, rate limiting and the honeypot field together will make it harder for the bot to succeed without alerting you to its activity.
3. API Endpoints: Throttle Requests and Require Tokens
APIs are often used to serve data to your front-end or mobile app, but they’re also a common target for DDoS attacks. Here’s how to protect them:
Step 1: Apply Throttle Middleware
Limit how many times an API endpoint can be accessed in a short period. For example:
Route::middleware('throttle:10,1')->group(function () {
Route::get('/search', [SearchController::class, 'search']);
});
This limits users to 10 searches per minute.
Step 2: Require Token-Based Access
For sensitive or private API endpoints, make sure users provide an authentication token. You can use packages like Laravel Sanctum or Passport to set up token-based authentication. This way, users need to log in and get a token before they can use certain API features.
Example: If someone tries to attack your search API, they'll be limited to a certain number of requests. Plus, without the right tokens, they won't be able to access the endpoint at all.
4. Checkout and Payment Pages: Web Application Firewalls and Queueing
Checkout pages are super important for your app. If attackers target these pages, it could really hurt your revenue. Here's how you can keep these critical areas safe:
Step 1: Set Up a Web Application Firewall (WAF)
A WAF acts like a security guard between your server and the internet. It checks incoming requests and blocks anything that looks fishy. Services like Cloudflare and AWS WAF are great for defending against DDoS attacks.
Step 2: Queue Backend Processes
In Laravel, you can use queues to handle tasks in the background. For instance, when a user makes a purchase, you might want to send them a confirmation email. Instead of sending it immediately, you can queue it to be processed later, which helps reduce server load during busy times.
Example: If someone tries to overwhelm your checkout page with fake orders, the WAF can block those suspicious IPs. Meanwhile, queued processes help keep your server running smoothly for genuine customers.
5. Interactive Forms (e.g. Feedback Forms): Adding Delays and Limiting Data Size
Interactive forms are another target for bots, especially if they can submit text data (like feedback). Bots might try to spam this form with junk data, so here’s what you can do:
Step 1: Introduce Progressive Delays
Add a delay between submissions. If a single IP keeps submitting the form repeatedly, progressively increase the delay between allowed submissions.
$attempts = Cache::get("feedback_attempts_{$request->ip()}", 0);
if ($attempts > 3) {
sleep($attempts); // Progressive delay as attempts increase
}
Cache::put("feedback_attempts_{$request->ip()}", $attempts + 1, now()->addMinutes(10));
Step 2: Limit the Data Size for Each Field
Limit the length of data a user can submit in each field to avoid large payload attacks. Here’s an example of how to set limits in your controller:
$request->validate([
'feedback' => 'required|string|max:500',
'username' => 'required|string|max:100',
]);
Example: If bots try to flood your feedback form, adding delays and limiting the data size can slow them down and stop them from sending too much data.
6. Monitor Activity with Real-Time Alerts
Keeping an eye on your application's activity is a great way to catch attacks early. You can use Laravel's logging features or tools like Sentry or Papertrail to set up real-time alerts for any unusual traffic or patterns.
Example: If there's a sudden increase in traffic to your API endpoint, you'll get an alert. This means you can quickly respond by increasing rate limits or blocking specific IPs.
Conclusion
DDoS attacks can be tough to deal with, but you can make it harder for attackers to mess with your Laravel app by using simple protections like rate limiting, CAPTCHAs, and honeypots, along with improving backend processes with queues and WAFs. Start with these easy steps and add more security as needed.
These steps will help keep your app running smoothly and accessible to real users, even when it's under attack. Remember, the best defense is having multiple layers of protection across all entry points, from login and registration forms to API and checkout endpoints.
Subscribe to my newsletter
Read articles from Anik Kumar Nandi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anik Kumar Nandi
Anik Kumar Nandi
Hi there! I'm Anik Kumar Nandi, passionate programmer, strongly focus on backend technologies. I specialize in PHP and Laravel, and I'm always exploring new ways to enhance the robustness and efficiency of my code. In addition to PHP and Laravel, my skill set includes RESTful API development, JavaScript, jQuery, Bootstrap, Ajax, and WordPress customization. Also deeply interested in AWS. Feel free to check out my GitHub profile and my portfolio site to learn more about my work and projects. Let's connect and create something amazing together!