Real World ML: Data Transformations
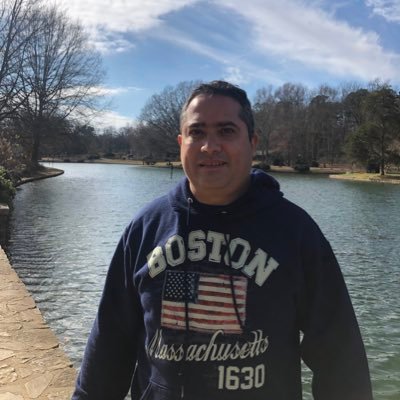
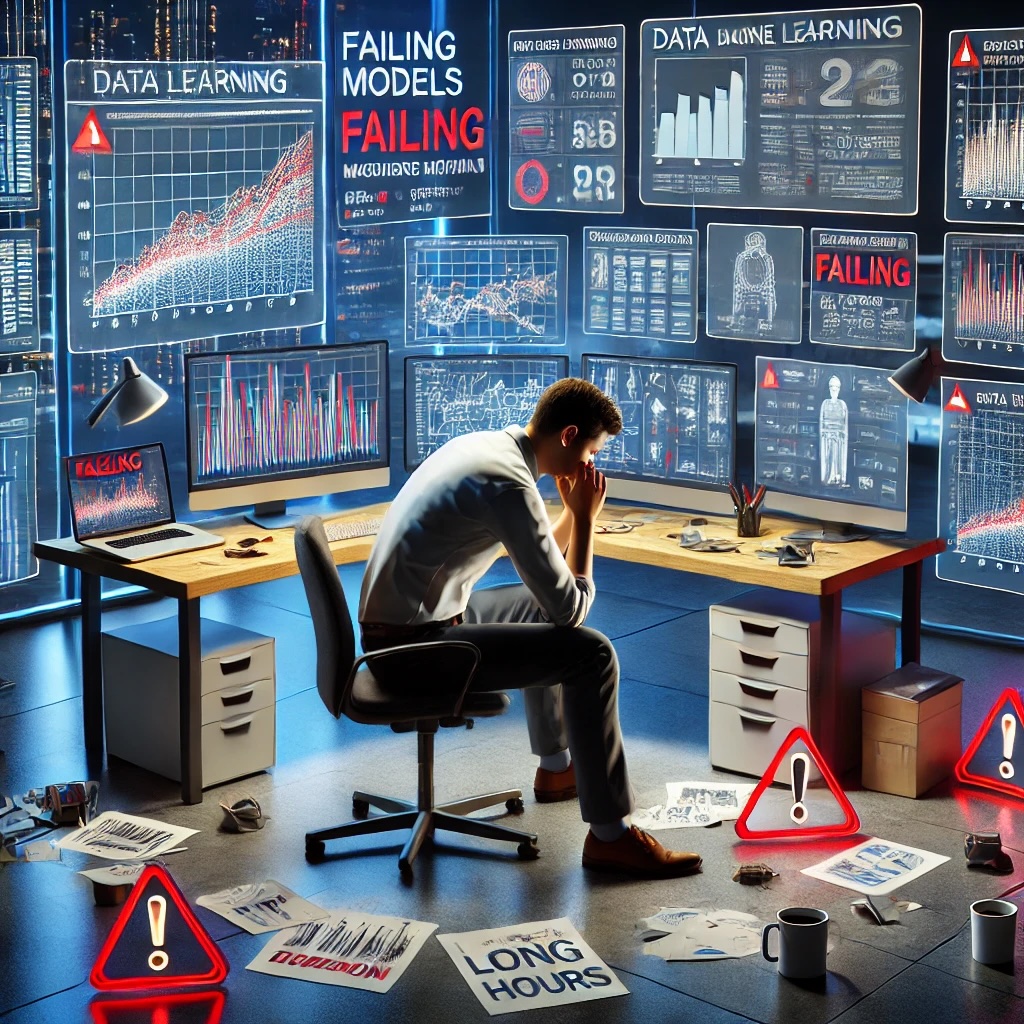
Imagine spending months building a machine learning model, only to watch it fail spectacularly in production. Your accuracy plummets from 95% in testing to 60% in the real world. Frustrating, isn't it?
Here's the twist: the problem likely isn't your sophisticated algorithm or carefully tuned hyperparameters. The silent killer? Your data distribution.
Most data scientists report that poor data quality is their biggest challenge, with skewed distributions being a primary culprit. This invisible enemy costs companies an average of 15-25% in lost model performance and weeks of wasted development time.
But there's hope.
By mastering data transformations – the art of reshaping your data's distribution – you can dramatically improve your model's performance.
In this guide, we'll explore proven techniques that top ML engineers use to transform raw, messy data into pristine inputs that machine learning models love.
You'll discover why seemingly perfect models fail and, more importantly, how to fix them using practical, code-first examples.
Understanding the Need for Data Transformations
Machine learning algorithms are like picky eaters.
They perform best when data is served in specific ways, typically following normal or uniform distributions.
Raw data, however, rarely comes in such a convenient form.
Instead, it often arrives skewed, with outliers, or showing irregular patterns that can confuse our models.
The Impact of Skewed Data
Skewed data can severely impact model performance in several ways:
It can make certain features dominate others unnecessarily.
It often leads to biased predictions favoring the majority of data points.
It can slow down model convergence during training.
It may cause models to miss important patterns in the minority of data points.
Types of Data Transformations
Linear Transformations
Linear transformations are the simplest form of data preprocessing.
They include operations like scaling and standardization.
While useful for many cases, they maintain the same relative relationships between data points.
This means they can't fix fundamental distribution problems.
Nonlinear Transformations
Logarithmic Transformation
The logarithmic transformation is one of the most popular nonlinear transformations:
It effectively compresses the range of large values.
It expands the range of small values.
It's particularly useful for right-skewed distributions.
It works well with data that follows exponential patterns.
Here's a simple Python implementation:
import numpy as np
# log1p adds 1 before taking log to handle zeros
def log_transform(data):
return np.log1p(data)
Sigmoid Transformation
The sigmoid transformation offers unique advantages:
It bounds all values between 0 and 1.
It handles extreme outliers effectively.
It maintains sensitivity in the middle range of values.
It's differentiable, making it suitable for gradient-based optimization.
def sigmoid_transform(data):
return 1 / (1 + np.exp(-data))
Polynomial Transformations
Polynomial transformations add flexibility to your preprocessing toolkit:
They can capture nonlinear relationships in the data.
Common forms include square root, square, and cube transformations.
They're particularly useful when dealing with power-law relationships.
They can help reveal hidden patterns in the data.
Advanced Transformation Techniques
If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
The Box-Cox Transformation
The Box-Cox transformation is a sophisticated method that automatically finds the optimal transformation parameter:
It uses maximum likelihood estimation to find the best transformation.
It helps stabilize variance across different magnitudes of data.
It can make data more normally distributed.
It's particularly useful when you're unsure which transformation to apply.
from scipy import stats
def box_cox_transform(data):
transformed_data, lambda_param = stats.boxcox(data)
return transformed_data, lambda_param
Histogram Equalization: The Bucketing Approach
When parametric transformations aren't sufficient, histogram equalization offers an alternative:
It divides data into buckets based on quantiles.
It ensures a more uniform distribution of values.
It's resistant to outliers.
It's particularly useful for image processing and similar applications.
Best Practices for Data Transformation
When to Transform Your Data
Consider transforming your data when:
You observe highly skewed distributions in your features.
Your model's performance varies significantly across different ranges of input values.
You notice slow convergence during model training.
You see evidence of heteroscedasticity in your residuals.
Transformation Pipeline Design
Follow these principles when designing your transformation pipeline:
Always fit transformations on training data only.
Apply the same transformations to validation and test sets.
Document your transformation choices and parameters.
Consider the interpretability of transformed features.
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import FunctionTransformer
def create_transformation_pipeline():
return Pipeline([
('log_transform', FunctionTransformer(np.log1p)),
('scaler', StandardScaler())
])
Common Pitfalls and How to Avoid Them
Data Leakage
Data leakage is a serious concern when applying transformations:
Never fit transformations on the entire dataset.
Always transform features independently of the target variable.
Keep training, validation, and test sets truly independent.
Document any assumptions made during transformation.
Interpretation Challenges
Transformed data can be harder to interpret:
Keep track of inverse transformations for interpretability.
Consider the business context when choosing transformations.
Document the reasoning behind each transformation choice.
Maintain clear communication with stakeholders about transformed features.
Real-world Applications
Financial Data Analysis
Financial data often requires careful transformation:
Returns data often needs log transformation.
Price data might benefit from differencing.
Volatility measures often require stabilization.
Trading volumes typically need normalization.
Conclusion
The success of your machine learning models often depends on how well you prepare your data.
By mastering these transformation techniques, you're better equipped to handle real-world data challenges.
Remember: there's no one-size-fits-all solution - the best transformation depends on your specific data and problem.
Keep experimenting, measuring results, and refining your approach.
PS: If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
Subscribe to my newsletter
Read articles from Juan Carlos Olamendy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
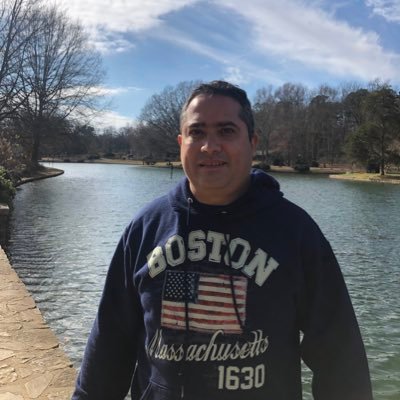
Juan Carlos Olamendy
Juan Carlos Olamendy
🤖 Talk about AI/ML · AI-preneur 🛠️ Build AI tools 🚀 Share my journey 𓀙 🔗 http://pixela.io