JavaScript Array Methods

Table of contents
- 20 JavaScript Array Methods You Need to Know
- 1. concat() β
- 2. slice() π°
- 3. at() πββοΈ
- 4. join() π΄
- 5. reverse() π
- 6. sort() π’
- 7. fill() ποΈ
- 8. forEach() π
- 9. map() π
- 10. flat() ποΈ
- 11. flatMap() π
- 12. reduce() β
- 13. filter() π§Ή
- 14. some() π
- 15. every() βοΈ
- 16. includes() β
- 17. find() π
- 18. findIndex() π
- 19. Array.from() π
- 20. splice() βοΈ
- Summary of Array Methods π
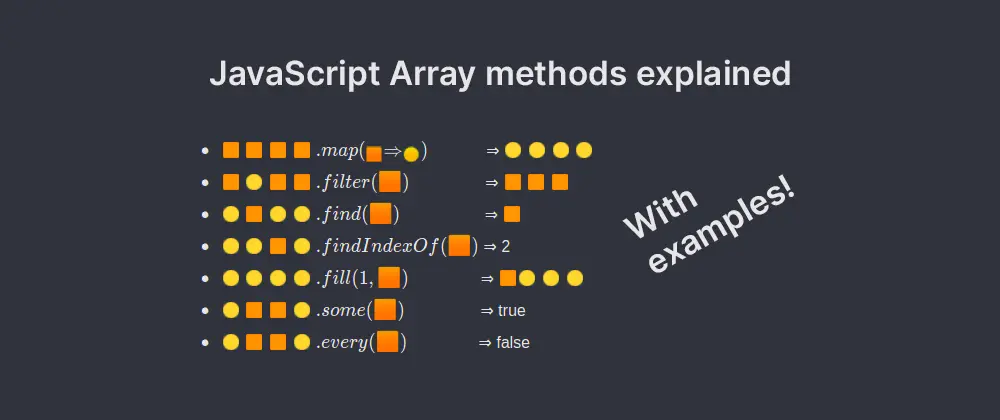
20 JavaScript Array Methods You Need to Know
JavaScript is a powerful language, and one of the key reasons for its versatility is the Array object. With array methods, we can perform a ton of operations on arraysβlike transforming data, flattening arrays, and checking conditionsβall without needing loops or counters. π
In this post, I'll walk you through 20 of the most commonly used array methods. Whether you're new to JavaScript or just need a refresher, these methods will help make your code cleaner and more efficient. Ready? Let's dive in! π
1. concat() β
What it does:
concat()
combines two or more arrays into a new array.
Returned Value:
A new array containing all the elements from the combined arrays.
const fruits = ['apple π', 'banana π'];
const moreFruits = ['mango π₯', 'pineapple π', 'kiwi π₯'];
const combined = fruits.concat(moreFruits);
console.log(combined); // ['apple π', 'banana π', 'mango π₯', 'pineapple π', 'kiwi π₯']
2. slice() π°
What it does:
slice()
returns a shallow copy of a portion of an array, without modifying the original array.
Returned Value:
A new array with the sliced elements.
const fruits = ['apple π', 'banana π', 'mango π₯', 'pineapple π'];
const slicedFruits = fruits.slice(1, 3);
console.log(slicedFruits); // ['banana π', 'mango π₯']
3. at() πββοΈ
What it does:
at()
returns an element at a specific index, supporting negative indices to count from the end.
Returned Value:
The element at the specified index.
const fruits = ['apple π', 'banana π', 'mango π₯', 'kiwi π₯'];
console.log(fruits.at(1)); // 'banana π'
console.log(fruits.at(-1)); // 'kiwi π₯'
4. join() π΄
What it does:
join()
joins all the elements of an array into a single string, separated by a specified delimiter.
Returned Value:
A string with all array elements joined.
const fruits = ['apple π', 'banana π', 'mango π₯'];
const fruitString = fruits.join(', ');
console.log(fruitString); // 'apple π, banana π, mango π₯'
5. reverse() π
What it does:
reverse()
reverses the order of elements in an array in place.
Returned Value:
The reversed array.
const animals = ['Lion π¦', 'Koala π¨', 'Kangaroo π¦'];
const reversedAnimals = animals.reverse();
console.log(reversedAnimals); // ['Kangaroo π¦', 'Koala π¨', 'Lion π¦']
6. sort() π’
What it does:
sort()
sorts the elements of an array in place (modifies the original array).
Returned Value:
The sorted array.
const animals = ['Lion π¦', 'Koala π¨', 'Kangaroo π¦', 'Giraffe π¦'];
const sortedAnimals = animals.sort();
console.log(sortedAnimals); // ['Giraffe π¦', 'Kangaroo π¦', 'Koala π¨', 'Lion π¦']
7. fill() ποΈ
What it does:
fill()
replaces all or part of an array with a specific value.
Returned Value:
The modified array.
const animals = ['Lion π¦', 'Koala π¨', 'Kangaroo π¦', 'Giraffe π¦'];
animals.fill('Elephant π', 1, 3);
console.log(animals); // ['Lion π¦', 'Elephant π', 'Elephant π', 'Giraffe π¦']
8. forEach() π
What it does:
forEach()
executes a provided function on each element, but does not return anything.
Returned Value:
undefined
.
const fruits = ['apple π', 'banana π', 'mango π₯'];
fruits.forEach(fruit => console.log(fruit + ' is tasty'));
9. map() π
What it does:
map()
allows you to transform each element in an array according to a given function.
Returned Value:
A new array with the transformed elements.
const fruits = ['apple π', 'banana π', 'mango π₯'];
const fruitLengths = fruits.map(fruit => fruit.length);
console.log(fruitLengths); // [5, 6, 5]
10. flat() ποΈ
What it does:
flat()
flattens an array, meaning it removes nested arrays.
Returned Value:
A new flattened array.
const nestedArr = [1, [2, 3], 4, [5, 6]];
const flattened = nestedArr.flat();
console.log(flattened); // [1, 2, 3, 4, 5, 6]
11. flatMap() π
What it does:
flatMap()
maps each element to a new array and then flattens the result.
Returned Value:
A new flattened array.
const numbers = [1, [2, 3], 4, [5, 6]];
const flattenedNumbers = numbers.flatMap(num => num);
console.log(flattenedNumbers); // [1, 2, 3, 4, 5, 6]
12. reduce() β
What it does:
reduce()
applies a function to each element in the array to reduce the array to a single value.
Returned Value:
A single value (could be a number, string, object, etc.).
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((total, num) => total + num, 0);
console.log(sum); // 10
13. filter() π§Ή
What it does:
filter()
checks each element and returns a new array with only those elements that pass a test.
Returned Value:
A new array with the elements that satisfy the condition.
const ages = [12, 5, 8, 21];
const adults = ages.filter(age => age >= 18);
console.log(adults); // [21]
14. some() π
What it does:
some()
checks if at least one element in the array satisfies a condition.
Returned Value:
true
or false
.
const ages = [15, 22, 34, 11];
const hasAdult = ages.some(age => age >= 18);
console.log(hasAdult); // true
15. every() βοΈ
What it does:
every()
checks if all elements in the array satisfy a condition.
Returned Value:
true
or false
.
const ages = [15, 22, 34, 11];
const allAdults = ages.every(age => age >= 18);
console.log(allAdults); // false
16. includes() β
What it does:
includes()
checks if an array contains a specific element.
Returned Value:
true
or false
.
const fruits = ['apple π', 'banana π', 'mango π₯'];
console.log(fruits.includes('banana π')); // true
17. find() π
What it does:
find()
searches for and returns the first element that satisfies a condition.
Returned Value:
The first element that passes the condition, or undefined
if no match is found.
const fruits = ['apple π', 'banana π', 'mango π₯'];
const foundFruit = fruits.find(fruit => fruit.startsWith('m'));
console
.log(foundFruit); // 'mango π₯'
18. findIndex() π
What it does:
findIndex()
returns the index of the first element that satisfies a condition.
Returned Value:
The index of the element, or -1
if no match is found.
const fruits = ['apple π', 'banana π', 'mango π₯'];
const index = fruits.findIndex(fruit => fruit.startsWith('b'));
console.log(index); // 1
19. Array.from() π
What it does:
Array.from()
creates a new array from an iterable (like a string or set).
Returned Value:
A new array.
const str = 'hello';
const arrayFromStr = Array.from(str);
console.log(arrayFromStr); // ['h', 'e', 'l', 'l', 'o']
20. splice() βοΈ
What it does:
splice()
adds/removes elements from an array at a specified index.
Returned Value:
The removed elements.
const animals = ['Lion π¦', 'Koala π¨', 'Kangaroo π¦', 'Giraffe π¦'];
const removedAnimals = animals.splice(1, 2, 'Elephant π');
console.log(animals); // ['Lion π¦', 'Elephant π', 'Giraffe π¦']
console.log(removedAnimals); // ['Koala π¨', 'Kangaroo π¦']
Summary of Array Methods π
Method | What It Does | Returns |
concat() | Combines two or more arrays | New combined array |
slice() | Returns a shallow copy of part of the array | New array with sliced elements |
at() | Accesses elements with positive or negative indices | Element at the specified index |
join() | Joins all elements into a string | String with joined elements |
reverse() | Reverses the array in place | Reversed array |
sort() | Sorts the elements in place | Sorted array |
fill() | Replaces elements with a specified value | Modified array |
forEach() | Executes a function on each element | undefined |
map() | Transforms each element | New array with transformed elements |
flat() | Flattens the array | Flattened array |
flatMap() | Maps and flattens the result | New flattened array |
reduce() | Reduces the array to a single value | Single output value |
filter() | Filters out elements based on a condition | New array with filtered elements |
some() | Checks if any element satisfies a condition | true or false |
every() | Checks if all elements satisfy a condition | true or false |
includes() | Checks if an array contains a specific value | true or false |
find() | Finds the first matching element | First matching element or undefined |
findIndex() | Finds the index of the first matching element | Index or -1 |
Array.from() | Creates a new array from an iterable | New array |
splice() | Adds/removes elements from the array | Removed elements |
With these 20 JavaScript array methods, youβll be able to tackle a wide variety of array manipulation tasks. π Whether you're transforming, filtering, searching, or flattening, there's a method for nearly every operation you need. Happy coding! π
Subscribe to my newsletter
Read articles from Oohnohassani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Oohnohassani
Oohnohassani
Hi π I'm a developer with experience in building websites with beautiful designs and user friendly interfaces. I'm experienced in front-end development using languages such as Html5, Css3, TailwindCss, Javascript, Typescript, react.js & redux and NextJs π