Modularity Magic: Structuring Your Code!
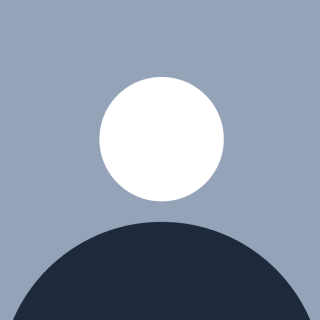
Table of contents
- What is Modularity?
- Understanding Modules in JavaScript
- ES6 Modules — Importing and Exporting
- Named Exports
- Default Exports
- Importing Modules
- Combining Named and Default Exports
- Flowchart: How Importing and Exporting Works
- Building with Modules
- Refactoring Code Using Modules
- Initial Code Without Modules
- Refactoring Into Modules
- Additional Tips for Modular Code
- Flowchart: Refactoring into Modules
- Challenge — Refactor Previous Code Using Modules
- Building Organized Code with Modules
- Next, let’s dive into Error Handling!
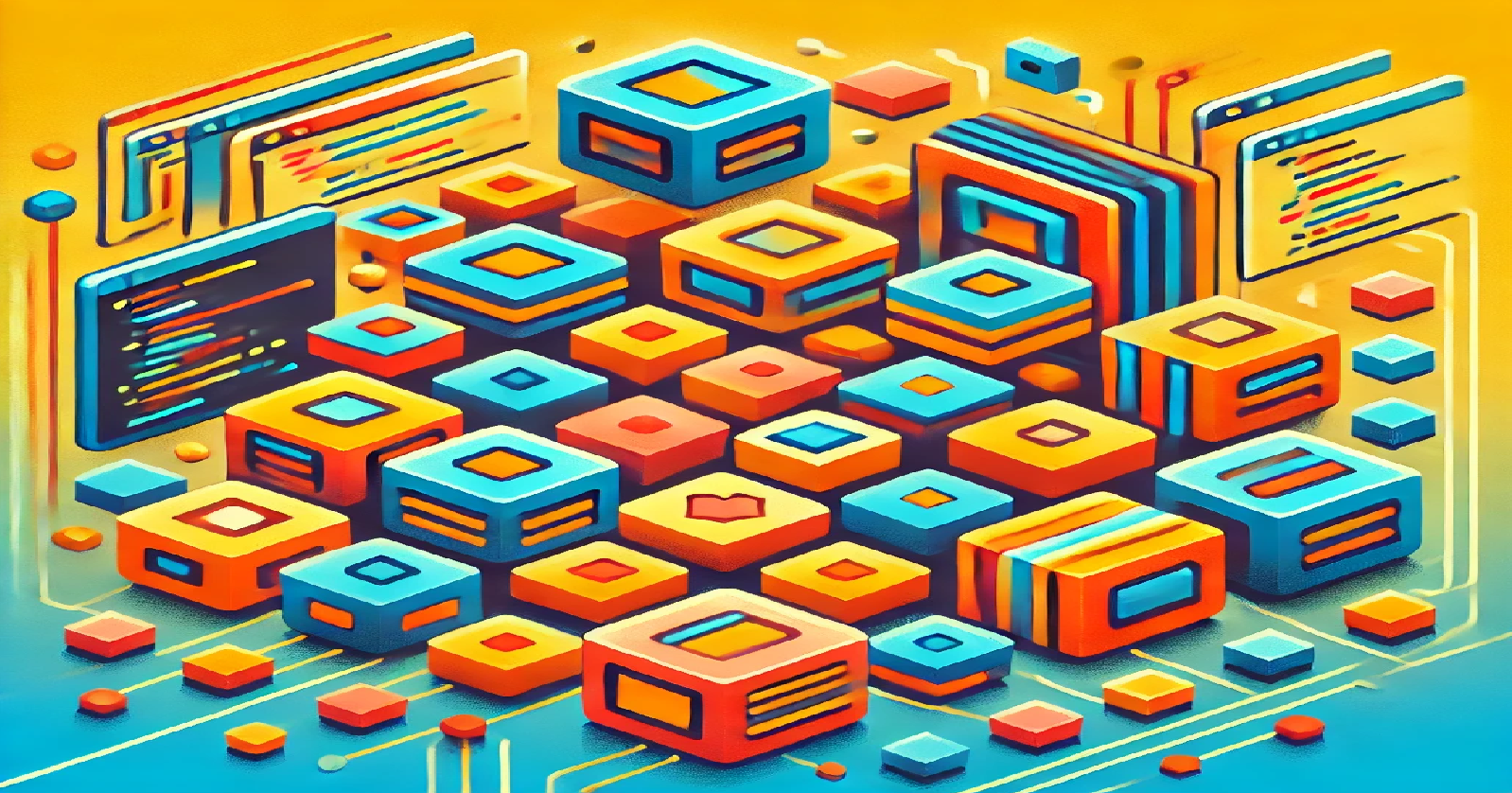
Imagine building a skyscraper brick by brick. Each brick is simple on its own, but when arranged with precision, they form a solid and impressive structure. In programming, modularity serves a similar purpose: it allows us to break down complex programs into smaller, reusable units of code called modules. These modules fit together, much like building blocks, to create more organized, maintainable, and scalable applications.
In this article, we’ll dive deep into the concept of modularity in JavaScript. We’ll explore how modules work, how they interact, and how the ES6 module system empowers developers to write cleaner, more maintainable code.
What is Modularity?
At its heart, modularity is about organizing your code into smaller, self-contained units that can be easily maintained and reused. Each module should ideally focus on a single responsibility, which makes your overall program:
Easier to maintain: You can update a module without worrying about affecting other parts of the code.
Easier to test: Since each module is independent, it’s easier to isolate and test individual parts of your program.
More reusable: You can use the same module in multiple applications or different parts of the same application.
More scalable: As your codebase grows, modular code helps manage the complexity.
Understanding Modules in JavaScript
In JavaScript, modules allow you to encapsulate code into different files, exporting the functionality from one module and importing it into another. Before ES6, developers used tools like CommonJS (Node.js) or AMD (browser-based) to handle modules. However, with the introduction of ES6 modules, JavaScript natively supports modular code, making it easier to structure your programs.
A module is just a file. Anything declared within that file is scoped to the module unless explicitly exported. Other parts of your application can import and use that functionality.
File Structure for Modular Code
Let’s visualize a typical folder structure for a modular JavaScript project:
/project
├── /src
│ ├── main.js
│ ├── utils.js
│ └── calculator.js
└── index.html
In this example:
main.js
is your entry point. This is where you’ll import the functionality from other modules.utils.js
andcalculator.js
contain individual functions or constants that can be reused across the application.
Each file (module) has its own responsibility. For example:
utils.js
might contain helper functions likeadd
and constants likePI
.calculator.js
could contain a specific function likesubtract
.
Flowchart: How Modules Interact in a File Structure
To better understand how modules interact, let’s look at the following flowchart that illustrates the relationship between main.js and the other modules:
[ utils.js ] --------> [ main.js ] <-------- [ calculator.js ]
| | |
[ Exports add() ] [ Imports add() ] [ Exports subtract() ]
[ Exports PI ] [ Imports subtract() ] [ Default Export ]
In this structure:
utils.js
exports functions likeadd
and constants likePI
.calculator.js
exports asubtract
function (in this case as a default export).main.js
imports both modules and uses their functionality to perform operations.
ES6 Modules — Importing and Exporting
ES6 modules introduced a built-in syntax for exporting and importing code across JavaScript files. This allows you to break your application into multiple files, each handling a specific part of the logic.
Exporting from a Module
There are two types of exports in JavaScript:
Named exports: Export multiple items by name, allowing you to import them selectively.
Default exports: Export a single value as the default, which can be imported without specifying a name.
Named Exports
Named exports allow you to export several values from a module.
Example:
// utils.js
export function add(a, b) {
return a + b;
}
export const PI = 3.14159;
Explanation:
- We export the
add
function and the constantPI
fromutils.js
. These can now be used in other parts of the application.
Default Exports
You can also export one default value from a module, typically used when the module’s primary responsibility is a single function or class.
Example:
// calculator.js
export default function subtract(a, b) {
return a - b;
}
Explanation:
- This exports the
subtract
function as the default export from thecalculator.js
module. This is useful when the module’s main functionality is based around this one function.
Importing Modules
To use the functionality exported from one module in another, you use the import
statement.
Importing Named Exports
When importing named exports, you need to specify the names of the functions, constants, or classes you want to import.
Example:
// main.js
import { add, PI } from './utils.js';
console.log(add(2, 3)); // Output: 5
console.log(PI); // Output: 3.14159
Explanation:
- We import the
add
function and the constantPI
fromutils.js
and use them inmain.js
.
Importing Default Exports
To import a default export, you can assign it a name without curly braces.
Example:
// main.js
import subtract from './calculator.js';
console.log(subtract(10, 5)); // Output: 5
Explanation:
- Here,
subtract
is imported fromcalculator.js
as the default export and can be used directly inmain.js
.
Combining Named and Default Exports
You can import both named exports and default exports from the same or different modules.
Example:
// main.js
import subtract from './calculator.js';
import { add, PI } from './utils.js';
console.log(add(5, 3)); // Output: 8
console.log(subtract(8, 3)); // Output: 5
console.log(PI); // Output: 3.14159
Flowchart: How Importing and Exporting Works
The following flowchart demonstrates how code is exported from one module and imported into another:
[ utils.js ] ----> [ main.js ] <---- [ calculator.js ]
| | |
[ Exports add() ] [ Imports add() ] [ Default Export ]
[ Exports PI ] [ Imports PI ] [ Imports subtract() ]
This shows the interaction between multiple files, where main.js
imports specific functionality from utils.js
and calculator.js
.
Building with Modules
In this part, we’ve explored how ES6 modules bring the power of modularity to JavaScript, allowing you to structure your code into manageable, reusable blocks. By using export and import statements, you can create clear boundaries between the different parts of your application, making it easier to maintain and scale.
In the next part, we’ll focus on refactoring code into modules and tackle a challenge that will put your modular skills to the test!
Refactoring Code Using Modules
Now that we’ve explored the basics of exporting and importing modules, it’s time to put modularity into practice. One of the greatest benefits of modular programming is the ability to refactor complex, monolithic codebases into smaller, more manageable pieces.
By separating concerns, you can break down a large script into different modules that handle specific tasks. This not only improves code readability but also enhances maintainability by isolating bugs or issues in specific modules.
Let’s take a simple example and refactor it into a modular structure.
Initial Code Without Modules
Here’s a non-modular script where all the functionality is in a single file:
// main.js (without modularity)
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a - b;
}
const PI = 3.14159;
console.log(add(5, 3)); // Output: 8
console.log(subtract(10, 2)); // Output: 8
console.log(PI); // Output: 3.14159
Problems:
All functions and constants are defined in a single file, making it harder to maintain as the project grows.
If you wanted to reuse
add
andsubtract
in another file, you’d have to copy-paste the code instead of importing it.
Refactoring Into Modules
Let’s refactor the code into separate modules for better structure and reusability.
Step 1: Create a utils.js
Module
Move the add
function and the PI
constant into a utils.js
module.
// utils.js
export function add(a, b) {
return a + b;
}
export const PI = 3.14159;
Step 2: Create a calculator.js
Module
Next, move the subtract
function into a separate module called calculator.js
.
// calculator.js
export default function subtract(a, b) {
return a - b;
}
Step 3: Refactor main.js
to Import These Modules
Now, we’ll import these modules into our main application file, main.js
.
// main.js
import { add, PI } from './utils.js';
import subtract from './calculator.js';
console.log(add(5, 3)); // Output: 8
console.log(subtract(10, 2)); // Output: 8
console.log(PI); // Output: 3.14159
Benefits:
Each function or constant is now self-contained in its own module, making it easier to manage and test.
main.js
is more focused on application logic, delegating specific tasks toutils.js
andcalculator.js
.The code is now modular, reusable, and scalable.
Additional Tips for Modular Code
Separation of Concerns: Ensure each module focuses on a single responsibility. This could be utility functions, data models, or specific UI components.
Reusability: Modules should be written with reusability in mind, especially when dealing with utility functions or shared components.
Avoid Circular Dependencies: Circular dependencies occur when two modules depend on each other, leading to potential bugs. To avoid this, ensure that modules are independent and serve clear, isolated purposes.
Flowchart: Refactoring into Modules
Let’s visualize how the refactoring from a single monolithic file into modular components looks:
Initial Monolithic Code
|
[ main.js ] (add(), subtract(), PI)
Refactor into Modules
|
[ main.js ] imports --> [ utils.js (add, PI) ]
[ calculator.js (subtract) ]
This shows how we’ve taken the monolithic script and divided it into three separate modules, each with a clear responsibility.
Challenge — Refactor Previous Code Using Modules
Now it’s your turn! Let’s refactor some existing code into modules. For this challenge, you’ll break down a simple application into multiple modules and restructure it for better maintainability.
Challenge Instructions:
Take an existing script you’ve written (or use the provided code below) and break it into three modules.
Each module should export its own functions or constants.
The main file should import the necessary modules and handle the application logic.
Provided Code (Non-modular):
// app.js
function multiply(a, b) {
return a * b;
}
function divide(a, b) {
return a / b;
}
function calculateCircleArea(radius) {
const PI = 3.14159;
return PI * radius * radius;
}
console.log(multiply(6, 7)); // Output: 42
console.log(divide(10, 2)); // Output: 5
console.log(calculateCircleArea(5)); // Output: 78.53975
Refactor the Code into Three Modules:
math.js: Contain
multiply
anddivide
.circle.js: Contain
calculateCircleArea
.app.js: Import functions from
math.js
andcircle.js
and handle application logic.
Example Solution:
Step 1: Create math.js
:
// math.js
export function multiply(a, b) {
return a * b;
}
export function divide(a, b) {
return a / b;
}
Step 2: Create circle.js
:
// circle.js
export function calculateCircleArea(radius) {
const PI = 3.14159;
return PI * radius * radius;
}
Step 3: Refactor app.js
:
// app.js
import { multiply, divide } from './math.js';
import { calculateCircleArea } from './circle.js';
console.log(multiply(6, 7)); // Output: 42
console.log(divide(10, 2)); // Output: 5
console.log(calculateCircleArea(5)); // Output: 78.53975
By following these steps, you’ll gain hands-on experience in refactoring code into modular components, which improves maintainability and reusability.
Building Organized Code with Modules
Modules are the building blocks of a well-structured codebase. By embracing modularity, you create a system where:
Complexity is reduced: Each module focuses on a specific task, making your code easier to understand and maintain.
Reusability is increased: Functions and constants can be shared across different parts of your application or even between projects.
Maintainability is enhanced: Changes to one module don’t affect the others, making it easier to isolate bugs and update features.
Key Takeaways:
ES6 modules allow you to split your code into smaller, more manageable files that can be imported and reused across your application.
Named and default exports provide flexibility in how you structure and share functionality between files.
Refactoring monolithic code into modules improves the overall organization and scalability of your codebase.
Next, let’s dive into Error Handling!
Now that we’ve mastered the art of modularity, it’s time to focus on the next important aspect of JavaScript development: error handling. In the upcoming article, we’ll learn how to manage errors gracefully, ensuring that your programs run smoothly even when things go wrong!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by