Resolving [Next.js] Error: Too Many Re-Renders
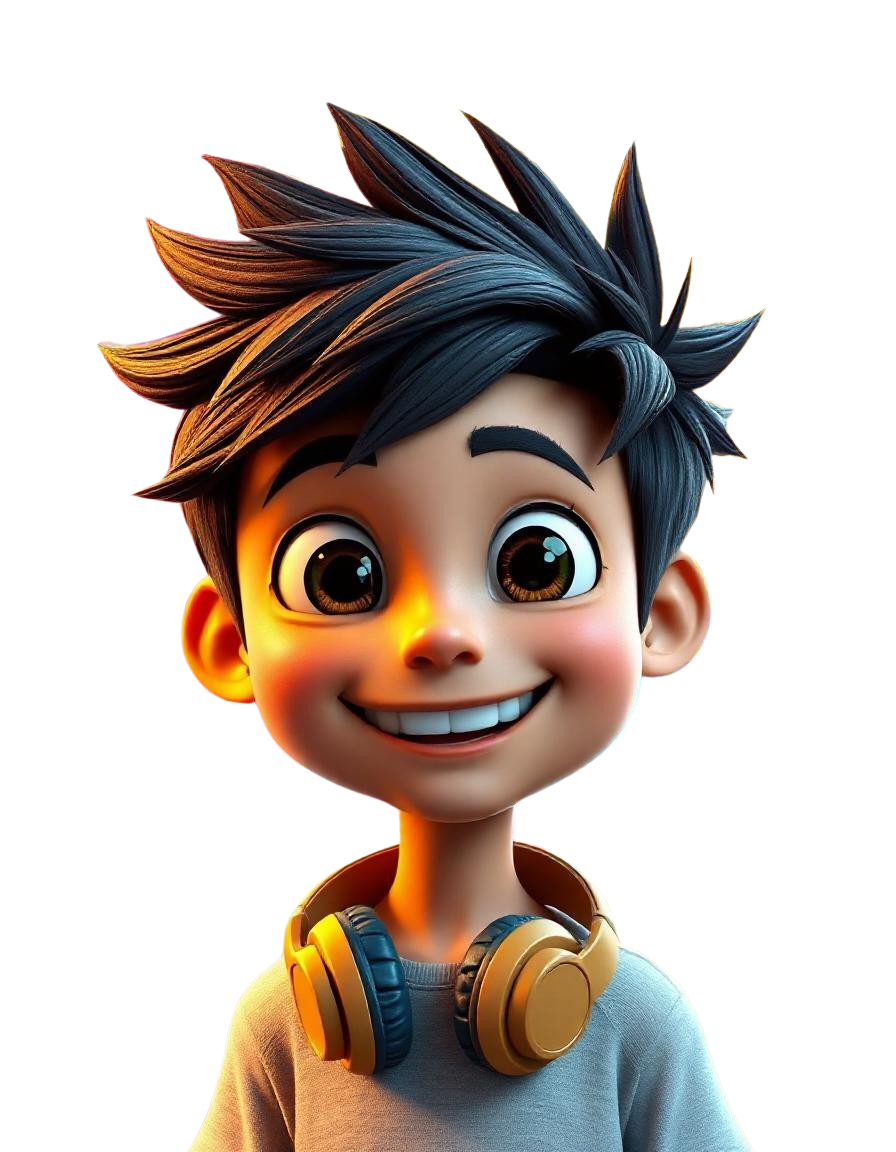
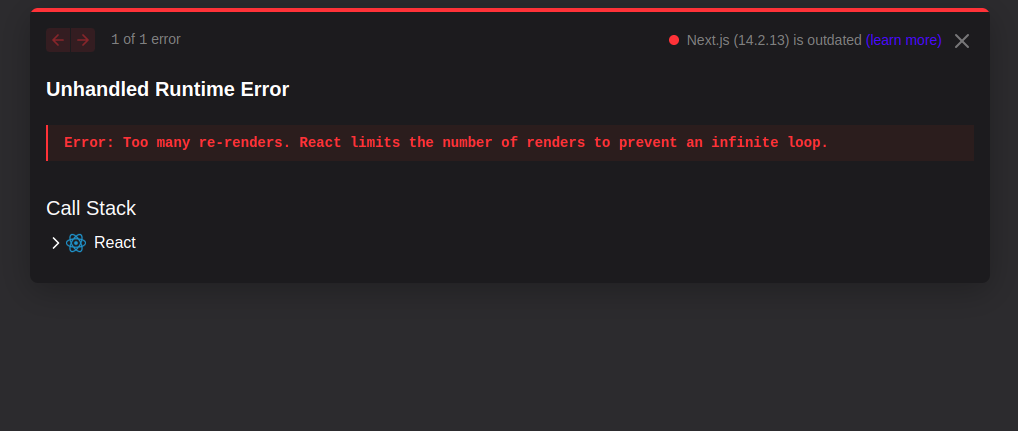
In today’s article, we will delve deeply into the common issue encountered in Next.js applications known as 'too many re-renders.' This problem often arises when a component is rendered repeatedly in a short period, which can lead to performance issues and a poor user experience. We will begin by exploring the various scenarios and coding practices that typically lead to this issue, such as improper use of state or effects. By understanding these root causes, we can then move on to discuss effective strategies and solutions to prevent excessive re-renders. These solutions will include optimizing state management, using memoization techniques, and ensuring that components only re-render when absolutely necessary. By the end of this article, you will have a comprehensive understanding of how to identify and resolve the 'too many re-renders' problem in your Next.js projects, leading to more efficient and smoother applications.
What causes the issue?
Setting State in Render
Misconfigured
useEffect
HookConditionally Setting State Inside Render
Using hooks(or state setters) directly in props
Solutions
If you're updating state directly in the render method or in a
useEffect
without dependencies, React will re-render infinitely. Make sure to only set state insideuseEffect
with proper dependencies.Example issue:
function MyComponent() { const [count, setCount] = useState(0); setCount(count + 1); // Causes infinite re-render return <div>{count}</div>; }
Fix: Move state updates to an event or
useEffect
:function MyComponent() { const [count, setCount] = useState(0); useEffect(() => { // Only run once on mount setCount((prevCount) => prevCount + 1); }, []); return <div>{count}</div>; }
If you're using
useEffect
to set state but forgot to provide dependencies, it will run on every render.Example issue:
useEffect(() => { setCount(count + 1); // No dependencies, causes infinite loop });
Fix: Add dependencies to the
useEffect
array to control when it runs:useEffect(() => { setCount(count + 1); }, [count]); // This will only re-run if count changes
If you have conditional logic that sets state based on certain conditions, make sure it doesn't lead to a state change that triggers another re-render immediately.
Example issue:
function MyComponent() { const [isReady, setIsReady] = useState(false); if (!isReady) setIsReady(true); // Causes infinite re-render return <div>Ready!</div>; }
Fix: Use
useEffect
to set initial state instead:useEffect(() => { setIsReady(true); }, []); // Runs only once on component mount
When you need to call a state-updating function in response to an event, wrap it in an anonymous arrow function or define it as a separate function to ensure it only runs when the event actually occurs.
Example issue:
<Input type="text" placeholder="First Name" onFocus={setError({ hasError:false, error:"" })} // cause an infinite re-render errorMessage={ taskError.error === "empty" ? "Task cannot be EMPTY" : "" } isInvalid={taskError.hasError} />
Fix: wrap the state-change in an arrow function so it only runs when the event is triggered
<Input type="text" placeholder="First Name" onFocus={() => setError({ hasError:false, error:"" })} // only calls when the focus event occurs errorMessage={ taskError.error === "empty" ? "Task cannot be EMPTY" : "" } isInvalid={taskError.hasError} />
Fix 2: You can also define a separate function and pass it as the event handler.
const handleFocus = () => setError({ hasError:false, error:"" }); <Input type="text" placeholder="First Name" onFocus={handleFocus} // Then pass it as a prop errorMessage={ taskError.error === "empty" ? "Task cannot be EMPTY" : "" } isInvalid={taskError.hasError} />
You've reached the conclusion of this article. I hope the information provided has been insightful and helpful in addressing any issues you may have encountered. By understanding how to properly handle state-updating functions in response to events, you can prevent common pitfalls like infinite re-renders. Remember, wrapping your state-change logic in an anonymous arrow function or defining it as a separate function ensures that your code runs efficiently and only when necessary. Thank you for reading, and I encourage you to apply these techniques in your projects to enhance performance and maintainability.
I will look forward to sharing more insights with you in future articles. Happy Coding 😊!!!
Subscribe to my newsletter
Read articles from Tharusha Nirmal Amarasooriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
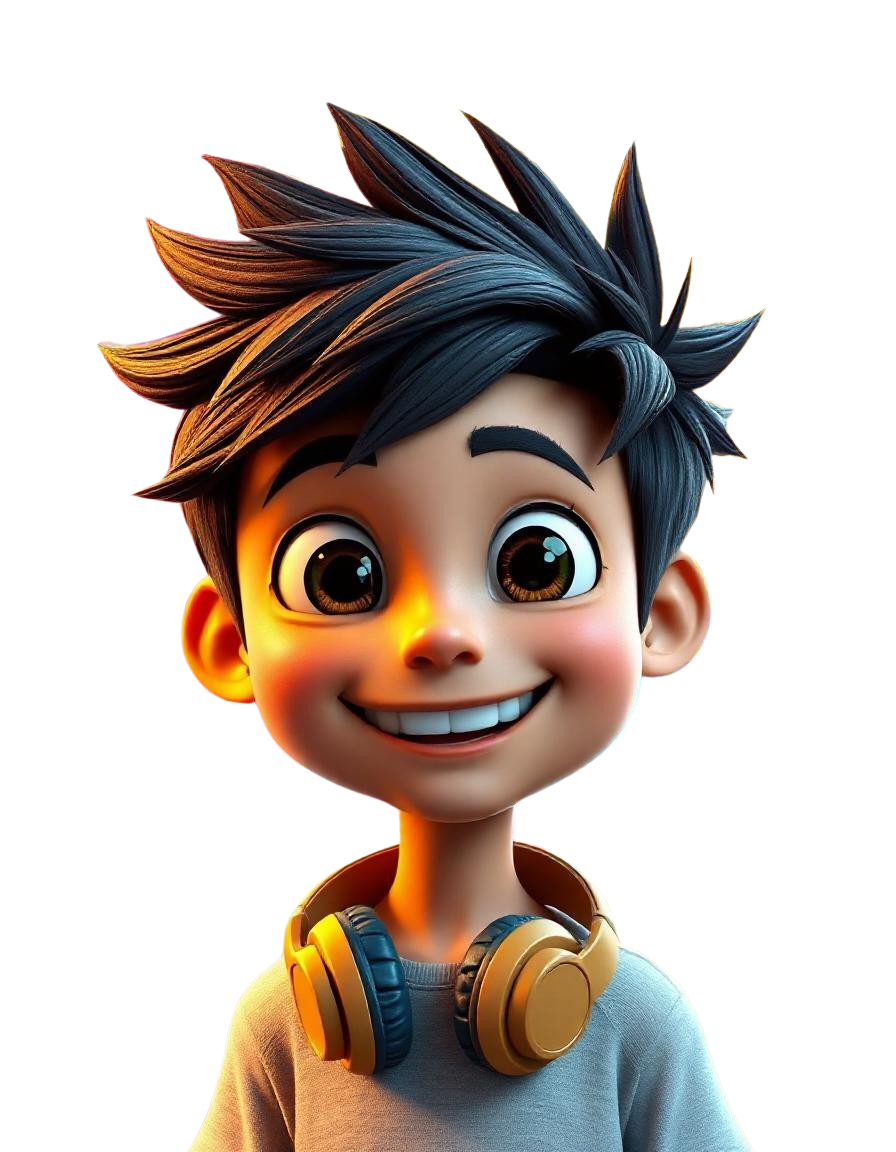
Tharusha Nirmal Amarasooriya
Tharusha Nirmal Amarasooriya
Student developer exploring Next.js, React, React Native & Tailwindcss. Solid in HTML & CSS. Passionate about building web/mobile apps. Always eager to learn🎯!