getting started with FastAPI: the beginner's guide to building high-performance apis with python

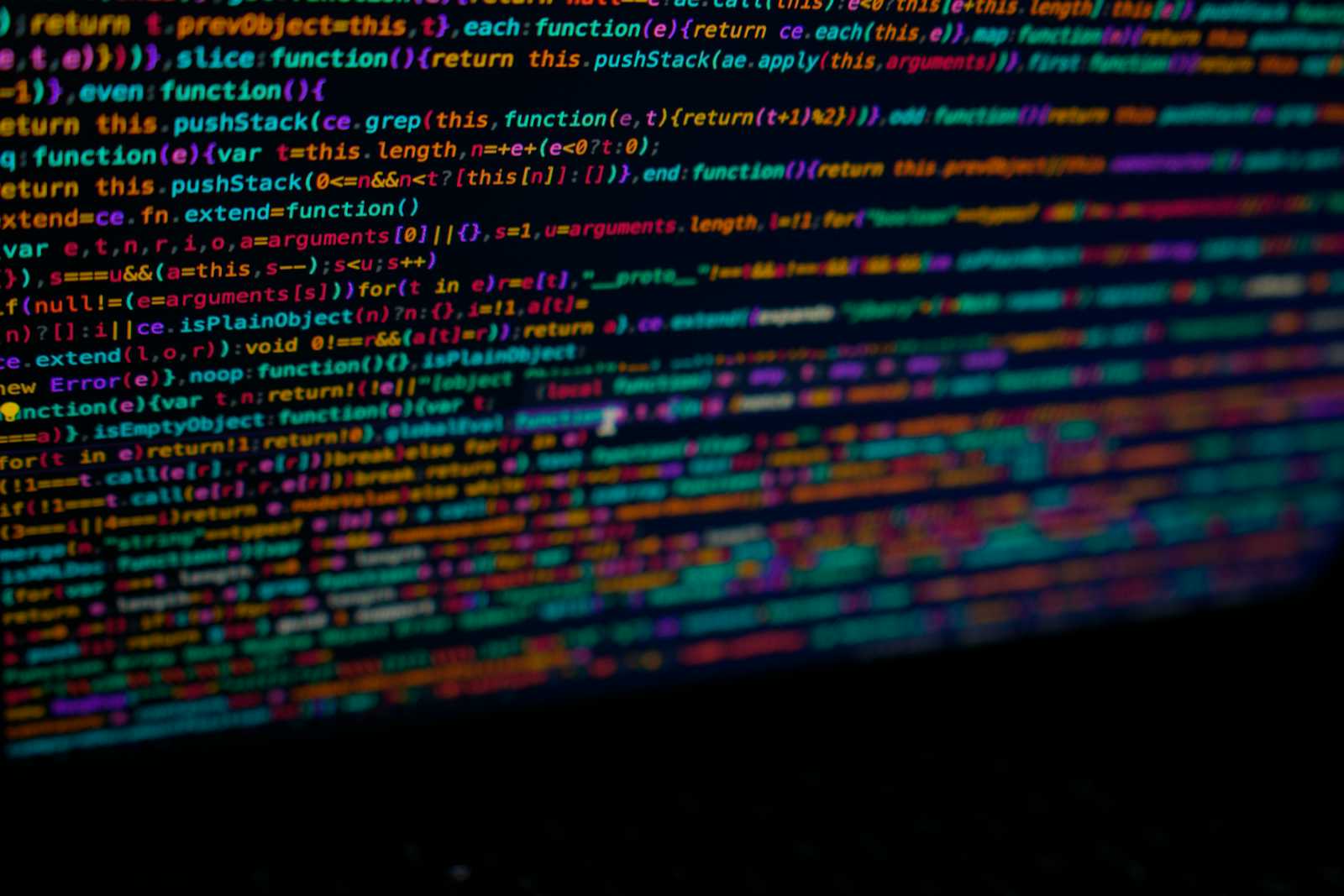
hello, i'm back with another article! today, let’s dive into understanding FastAPI—what it is, why it’s becoming so popular, and why you might want to use it for your python projects.
if you've worked with python backend frameworks, you've likely come across Django and Flask. and if you're a bit more into python, then you may have heard of FastAPI too. it’s quickly gaining traction, especially for building modern apis that require high performance and asynchronous processing.
officially, FastAPI is described as:
“a modern, fast (high-performance), web framework for building apis with python based on standard python type hints.”
let's break this down and see what makes it special.
what is a web framework, and why use it for apis?
a web framework provides the tools and libraries needed to build web applications, including apis, without starting from scratch. frameworks like Django, Flask, and FastAPI simplify the development process by managing much of the underlying code needed to build and deploy your application.
an API (Application Programming Interface) allows different applications to communicate with each other. for example, a mobile app might use an API to communicate with a server and access its database. FastAPI helps you create these APIs in a quick, clean, and efficient way.
why choose FastAPI?
if you're a python developer, here’s why learning it is a valuable skill:
easy to learn and use: fastapi is straightforward, with a syntax that’s easy to pick up, even if you're new to backend development.
high performance: FastAPI was designed to be fast—hence the name! it’s built on asynchronous calls, making it perfect for handling high loads and ensuring quick responses.
asynchronous capabilities: with the rise of generative ai, large language models (llms), and real-time applications, there's a greater need for asynchronous processing. FastAPI fully supports asynchronous programming, making it well-suited for projects in these fields.
automatic interactive api documentation: fastapi generates interactive api documentation automatically with swagger ui, making testing and working with your apis much easier.
getting started with fastapi
now that we understand what fastapi offers, let’s build our first api from scratch.
prerequisites
to follow along, ensure you have python installed on your system. you can check this by running:
python --version
if python is installed, you’ll see the version number. if not, download and install python using python installation guide.
step 1: installing fastapi and uvicorn
fastapi doesn’t work alone; it needs a server to run on. we’ll use uvicorn, a lightweight, fast server for python async frameworks.
install fastapi and uvicorn using the following command:
pip install "fastapi[standard]" uvicorn
step 2: creating your first api with fastapi
next, create a file named main.py
and add the following code:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def root():
return {"message": "hello world"}
understanding the code
FastAPI()
: initializes a fastapi app.@app.get("/")
: this is a decorator that tells fastapi to connect this function to the “/” endpoint. whenever a user accesses the root url (/
), this function will be triggered.async def root()
: this defines an asynchronous function that returns a simple json response{"message": "hello world"}
.
step 3: running your fastapi server
to launch the server, use the following command:
uvicorn main:app --reload
main
refers to the filename,app
is the fastapi instance we created, and--reload
automatically restarts the server when you make changes to the code.
testing the api
open your browser and go to http://127.0.0.1:8000.
you should see the following json response:
{"message": "hello world"}
interactive api documentation
one of fastapi’s standout features is its automatic interactive documentation, generated with swagger ui.
go to http://127.0.0.1:8000/docs.
you’ll see a detailed, interactive view of your api endpoints, where you can test your api directly from the browser.
fastapi also generates documentation at http://127.0.0.1:8000/redoc, using redoc.
adding a new endpoint
let’s expand our api with an additional endpoint to understand how easy it is to build with fastapi.
add the following code to main.py
:
@app.get("/greet/{name}")
async def greet(name: str):
return {"message": f"hello, {name}!"}
@app.get("/greet/{name}")
: defines a new endpoint that accepts aname
parameter.name: str
: type hints in fastapi ensure thatname
is a string, and it will raise an error if anything else is passed.
restart the server, then test this endpoint by visiting http://127.0.0.1:8000/greet/yourname.
closing note
fastapi is an excellent choice for building modern, high-performance apis in python. its asynchronous capabilities, combined with ease of use and automatic documentation, make it a powerful tool for developers, especially those working in fields like generative ai or machine learning.
whether you’re a beginner or an experienced python developer, learning fastapi can enhance your skills and help you create efficient, responsive backend services. happy coding, and don’t hesitate to share your own fastapi projects or tips!
you can check out the official fastapi documentation here if you want to learn more.
see ya :)
always eager to learn and connect with like-minded individuals. happy to connect with you all here!
Subscribe to my newsletter
Read articles from Vinayak Gavariya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinayak Gavariya
Vinayak Gavariya
Machine Learning Engineer