π¦ Mastering JSON Manipulation in Python: A Comprehensive Guide
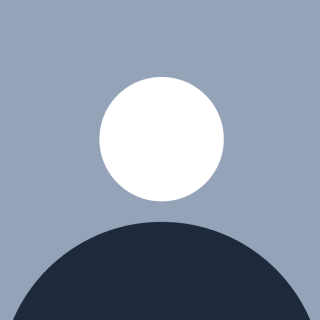
Hey Devs! π
If you've ever dealt with APIs, web development, or data interchange, you've likely encountered JSON (JavaScript Object Notation). It's everywhere! JSON is a lightweight format thatβs easy for both humans and machines to read and write. Today, I'll guide you through the manipulating JSON data in Python using Python's built-in json
library.
Letβs dive in! π
π What is JSON?
JSON (JavaScript Object Notation) is a popular format used to structure data, especially when transferring data between a server and a client. Here's what a typical JSON object looks like:
{
"name": "Krishna",
"age": 24,
"city": "Kolhapur"
}
Why Use JSON?
Lightweight & Easy to Read π
Language Independent π
Widely Used in APIs π
π§ Getting Started: Importing the JSON Library
Python provides a powerful built-in module called json
to work with JSON data. First, make sure you import it:
import json
π§© Parsing JSON: Convert JSON to Python Dictionary
Want to convert a JSON string into a Python dictionary? That's where json.loads()
comes in handy.
Example:
import json
# JSON string
json_data = '{"name": "Krishna", "age": 24, "city": "Kolhapur"}'
# Parse JSON string into a Python dictionary
data = json.loads(json_data)
print(data)
# Output: {'name': 'Krishna', 'age': 24, 'city': 'Kolhapur'}
π Whatβs Happening?
The json.loads()
function takes a JSON string and converts it into a Python dictionary. Now you can access the data just like a dictionary!
π Accessing JSON Data
Once you have your JSON parsed, accessing the data is a breeze!
print(data['name']) # Output: Krishna
print(data['age']) # Output: 24
βοΈ Modifying JSON Data
Need to make changes to your JSON data? No problem! You can easily add or update fields.
Example:
data['country'] = 'IND'
data['age'] = 25
print(data)
# Output: {'name': 'Krishna', 'age': 25, 'city': 'Kolhapur', 'country': 'IND'}
π Converting Python Dictionary Back to JSON String
After manipulating your data, you may want to convert it back to a JSON string. This is where json.dumps()
shines.
updated_json_data = json.dumps(data)
print(updated_json_data)
# Output: {"name": "Krishna", "age": 25, "city": "Kolhapur", "country": "IND"}
π Fun Fact:
json.dumps()
outputs a JSON string with double quotes, unlike Python dictionaries that use single quotes.
π Reading and Writing JSON Files
Python makes it super easy to read from and write to JSON files.
π Writing to a JSON File
data = {'name': 'Krishna', 'age': 24, 'city': 'Kolhapur'}
with open('output.json', 'w') as file:
json.dump(data, file)
print("Data saved to output.json")
π Reading from a JSON File
with open('output.json', 'r') as file:
loaded_data = json.load(file)
print(loaded_data)
# Output: {'name': 'Krishna', 'age': 24, 'city': 'Kolhapur'}
π load
vs loads
and dump
vs dumps
Understanding the differences between these methods can be tricky at first, so hereβs a quick guide:
Method | Purpose | Input Type | Output Type |
json.load | Read JSON data from a file | File object | Python dict |
json.loads | Parse JSON string to Python dict | JSON string | Python dict |
json.dump | Write Python dict to a JSON file | Python dict | JSON in file |
json.dumps | Convert Python dict to JSON string | Python dict | JSON string |
π Pro Tip:
Use
loads()
anddumps()
for strings.Use
load()
anddump()
for files.
π‘ Use Cases of JSON in Python
API Communication: Send and receive data in JSON format.
Configuration Files: Store settings in JSON format for easy access.
Data Serialization: Convert Python objects to JSON strings for storage or transmission.
π Conclusion
Mastering JSON manipulation in Python opens doors to seamless data handling, especially when dealing with APIs or web development. Now you can confidently parse, access, modify, and convert JSON data like a pro!
Got questions or want to share your thoughts? Drop them in the comments! Let's connect. π
π Keep Exploring
If you enjoyed this guide, consider following my blog for more exciting content on Python, DevOps, and everything tech! π
Happy coding! π¨βπ»π©βπ»
Subscribe to my newsletter
Read articles from Krishnat Ramchandra Hogale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Krishnat Ramchandra Hogale
Krishnat Ramchandra Hogale
Hi! Iβm Krishnat, a Senior IT Associate specializing in Performance Engineering at NTT DATA SERVICES. With experience in cloud technologies, DevOps, and automation testing, I focus on optimizing CI/CD pipelines and enhancing infrastructure management. Currently, I'm expanding my expertise in DevOps and AWS Solutions Architecture, aiming to implement robust, scalable solutions that streamline deployment and operational workflows.