Top Coding DSA Questions for Placements 2024
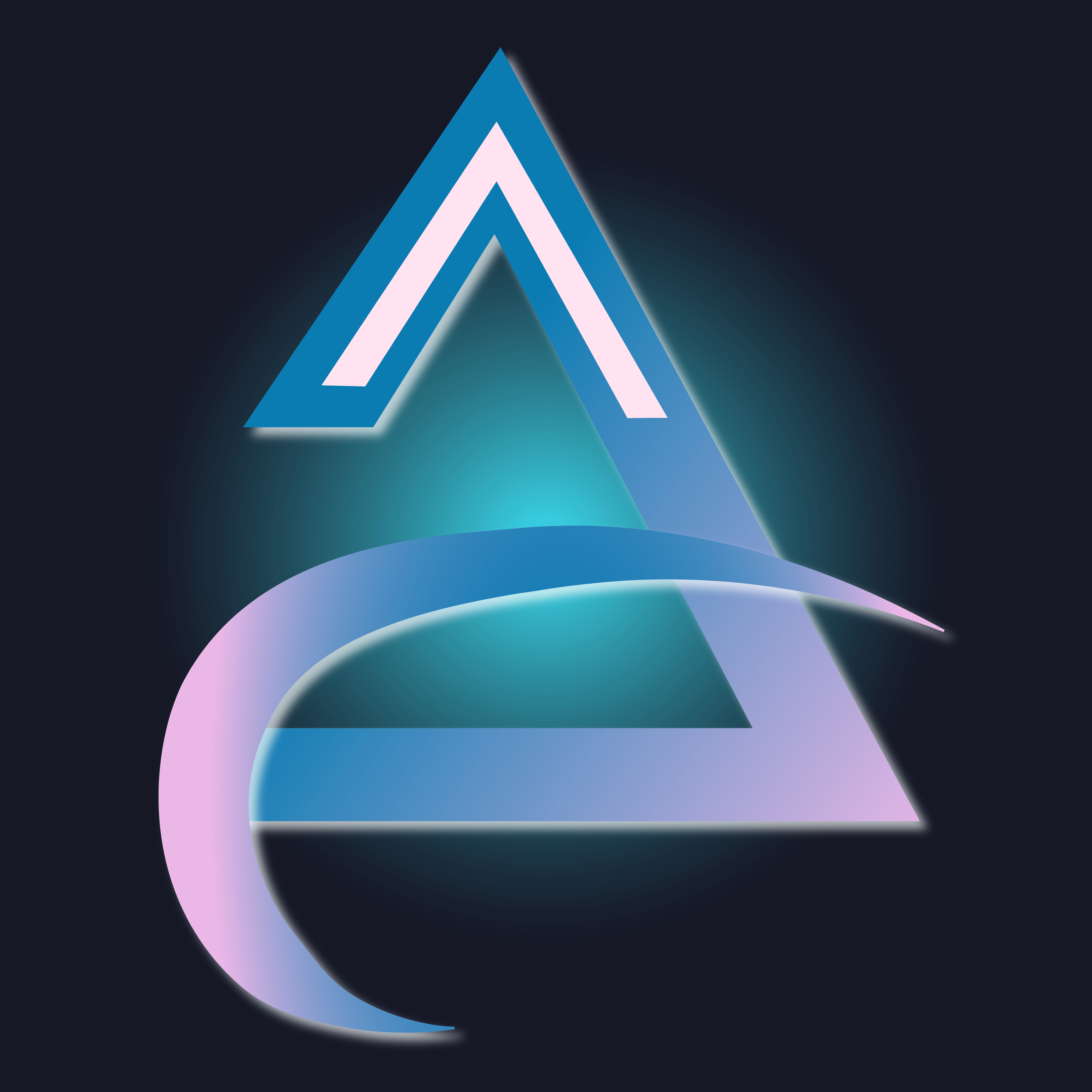
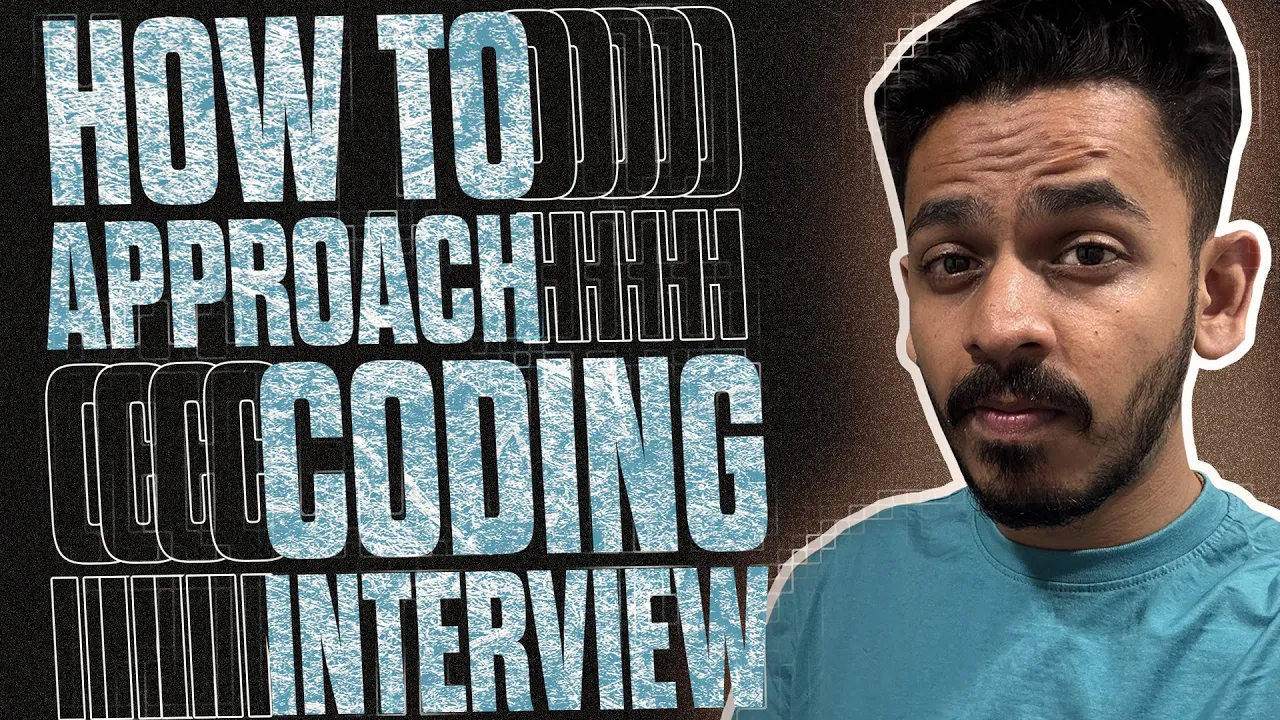
Welcome to our comprehensive resource guide for mastering Data Structures and Algorithms (DSA)! Whether you're a beginner or an experienced developer, this blog is designed to help you improve your coding skills and crack tough interviews.
In this resource guide, we've curated a list of essential DSA problems, including:
Dynamic Programming
Binary Search
Array and Matrix problems
Graph and Tree problems
Backtracking and Miscellaneous problems
Each problem comes with a detailed explanation, approach, and solution to help you understand the concepts and implement them efficiently.
What to Expect:
Expert-curated problems and solutions
Step-by-step explanations and approaches
Code snippets in popular programming languages
Regular updates with new problems and solutions
Interview-specific questions and tips
Who is this guide for?
Beginners looking to build a strong foundation in DSA
Experienced developers seeking to improve their coding skills
Interview candidates preparing for technical interviews
Coding enthusiasts who want to stay up-to-date with the latest DSA trends
The Questions given below are from the students who are placed for the year 2024:
Dynamic Programming:
DP Stock Question:
Problem Statement: Given an array of stock prices, find the maximum profit that can be achieved by buying and selling stocks.
Approach: Use dynamic programming to track the maximum profit at each step. Initialize two variables:
buy
andsell
. Iterate through the array, updatingbuy
andsell
based on whether it's better to buy or sell at each step.Good Subarray:
Problem Statement: Find the number of good subarrays in an array, where a good subarray is defined as one with a sum equal to 0.
Approach: Use a hashmap to store the cumulative sum of elements. Iterate through the array, updating the cumulative sum and checking if it exists in the hashmap.
LinkedList Intersection:
Problem Statement: Find the intersection point of two linked lists.
Approach: Use two pointers to traverse both linked lists. Calculate the difference in lengths and move the pointer of the longer list accordingly.
Fibonacci Series:
Problem Statement: Find the nth Fibonacci number using dynamic programming.
Approach: Create an array to store Fibonacci numbers. Initialize the first two elements and iterate through the array, updating each element as the sum of the previous two.
Subsequence Question:
Problem Statement: Find the longest common subsequence between two strings.
Approach: Create a 2D array to store lengths of common subsequences. Iterate through both strings, updating the array based on matches.
Binary Search:
Twisted Binary Search:
Problem Statement: Find an element in a twisted sorted array.
Approach: Modify the standard binary search algorithm to account for the twist.
Array:
Good Subarrays with Sum as 0:
Problem Statement: Find the number of good subarrays with a sum equal to 0.
Approach: Use a hashmap to store the cumulative sum of elements.
2D Array Sum:
Problem Statement: Find the maximum sum of a subarray in a 2D array.
Approach: Use Kadane's algorithm to find the maximum sum of subarrays in each row and column.
Second Largest Element:
Problem Statement: Find the second largest element in an array.
Approach: Iterate through the array, keeping track of the maximum and second maximum elements.
Kth Largest Element:
Problem Statement: Find the kth largest element in an array.
Approach: Use a priority queue or quickselect algorithm.
Matrix Problems:
Spiral Matrix Traversal:
Problem Statement: Traverse a matrix in a spiral order.
Approach: Use four pointers to keep track of the current boundaries.
Diagonal Traversal of a 2D Matrix:
Problem Statement: Traverse a matrix diagonally.
Approach: Use two pointers to keep track of the current diagonal
Reverse a String without Changing Special Characters:
Problem Statement: Reverse a string without changing the positions of special characters.
Approach: Use two pointers to swap characters.
Graph Problems:
Find Shortest Path between Two Nodes in a Binary Tree:
Problem Statement: Find the shortest path between two nodes in a binary tree.
Approach: Use DFS or BFS to find the lowest common ancestor.
Find Second Largest Element in an Array without Sorting:
Problem Statement: Find the second largest element in an array without sorting.
Approach: Iterate through the array, keeping track of the maximum and second maximum elements.
BackTracking:
Hanoi's Tower:
Problem Statement: Move a stack of disks from one rod to another, subject to certain rules.
Approach: Use recursive backtracking to move disks one by one.
Rat in a Maze:
Problem Statement: Find a path for a rat to escape a maze.
Approach: Use backtracking to explore possible paths.
Euler's Totient Function:
Problem Statement: Calculate the number of positive integers less than or equal to n that are relatively prime to n.
Approach: Use the formula φ(n) = n (1 - 1/p1) (1 - 1/p2) ... (1 - 1/pk), where p1, p2, ..., pk are prime factors of n.
Find Largest Prime Factor of a Number:
Problem Statement: Find the largest prime factor of a given number.
Approach: Use a loop to divide the number by increasing integers until it's no longer divisible.
Reverse Linked List in Groups of Size K:
Problem Statement: Reverse a linked list in groups of size k.
Approach: Use a recursive or iterative approach to reverse each group.
N Queen Problem | Return all Distinct Solutions to the N-Queens Puzzle:
Problem Statement: Place n queens on an nxn chessboard such that no two queens attack each other.
Approach: Use backtracking to explore possible placements.
Fractional Knapsack Problem:
Problem Statement: Maximize the value of items in a knapsack with limited capacity.
Approach: Use a greedy algorithm to select items with the highest value-to-weight ratio.
K-th Element of two Sorted Arrays:
Problem Statement: Find the kth element in the union of two sorted arrays.
Approach: Use binary search or a priority queue.
These problems cover a wide range of topics in data structures and algorithms. Practicing these problems will help you develop a strong foundation in coding and improve your chances of success in technical interviews.
Recommended Resources:
GeeksforGeeks
LeetCode
HackerRank
CodeForces
If you liked the resource, do not forget to like it and also share it among your friends, colleagues and juniors so that it will be useful for them too..
If you haven’t watched the video yet, do watch it now!!
Adios Amigos 👋👋
Subscribe to my newsletter
Read articles from Coding Adda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
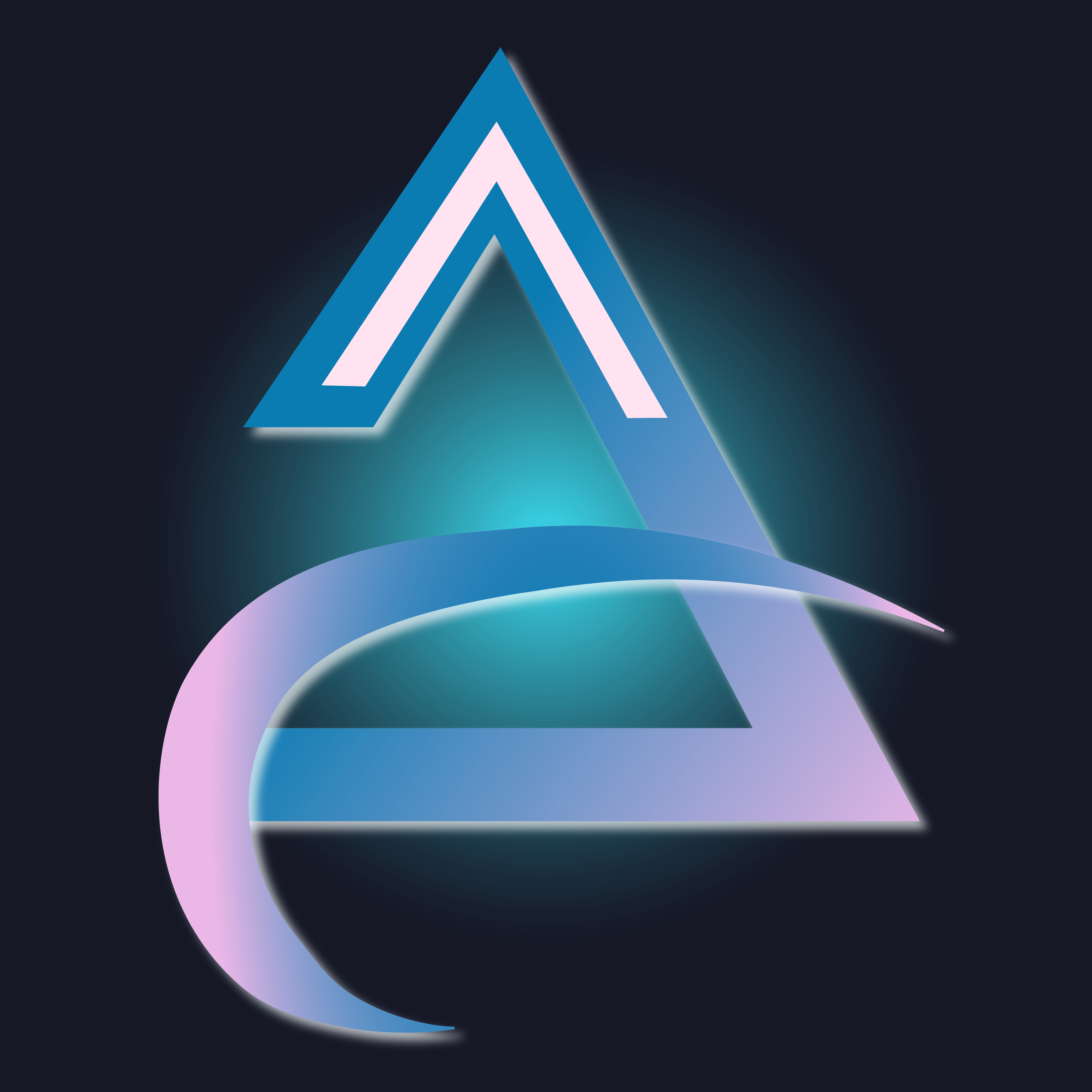
Coding Adda
Coding Adda
Hello Everyone, Presenting you CODING ADDA, We at Coding Adda will help you solve problems in Coding. We at Coding Adda try to clarify the concepts of Coding through short videos and real-life examples. Do support us in our journey. Like, Share and Subscribe to the Channel.