Mastering Spring Boot: The Ultimate Guide to Building Production-Ready Applications
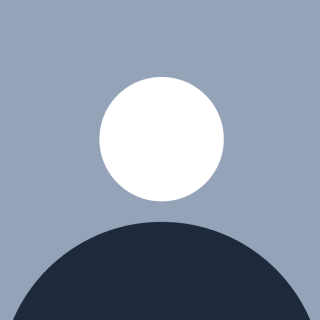
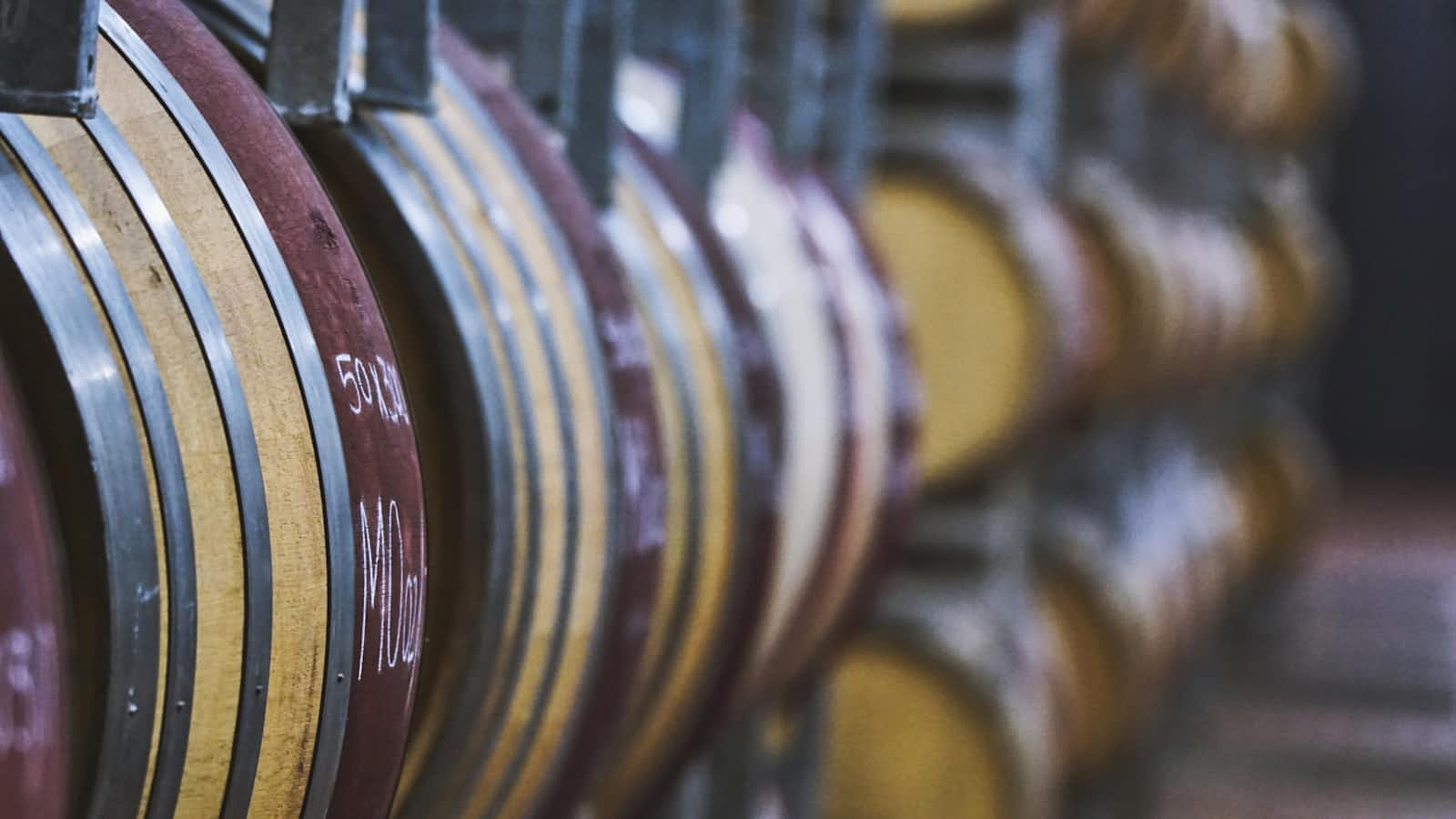
Spring Boot has become a go-to framework for building scalable, microservice-based Java applications due to its simplicity and productivity features. However, making an application "production-ready" requires a focus on stability, security, maintainability, and scalability. This guide will walk you through crucial areas to consider when preparing a Spring Boot application for production deployment.
We’ll cover best practices for project structure, configuration management, error handling, security implementation, logging setup, metrics and monitoring, and finally, deploying with Docker.
1. Project Structure Best Practices
A well-organized project structure simplifies maintenance and enables easier collaboration, especially in large applications.
Recommended Folder Structure
Organize the project by features and responsibilities to enhance readability:
controller
: Contains classes for handling HTTP requests and orchestrating responses.service
: Houses the business logic of the application.repository
: Manages data access, including CRUD operations with databases.config
: Stores configuration classes for external integrations and application setup.
Here's a sample structure:
src/main/java/com/example/appname
├── config
├── controller
├── model
├── repository
└── service
In a large project, it may be beneficial to further modularize each layer into feature-based packages, keeping related code together. This way, new developers can easily locate components and their dependencies.
Common Pitfall: Mixing Layers and Responsibilities
Mixing business logic within controllers or data access within services can lead to hard-to-maintain code. Ensure that each layer has a single responsibility to prevent tight coupling and to ease testing and debugging.
2. Configuration Management
Proper configuration management ensures that each environment (development, testing, production) has its own setup, without hard-coded values that may create security risks.
Application Properties and Profiles
Spring Boot enables you to manage environment-specific configurations via profiles. Store configurations in application.yml
or application.properties
, and use profile-specific files (e.g., application-dev.yml
, application-prod.yml
) to configure different environments.
Example:
# application-dev.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/dev_db
username: dev_user
password: dev_password
# application-prod.yml
spring:
datasource:
url: jdbc:mysql://prod-db-server:3306/prod_db
username: prod_user
password: prod_secure_password
Activate a profile by setting the --spring.profiles.active
flag, either in the application startup command or as an environment variable.
Troubleshooting Tip: Use Placeholders for Dynamic Configurations
When dealing with multiple environments, missing or incorrect values in environment variables can lead to application errors. Use @Value("${property:defaultValue}")
in Spring to provide fallbacks and avoid runtime issues if a property is missing.
Handling Sensitive Data Securely
Sensitive information like database credentials should never be committed in source control. Instead, consider these approaches:
Environment Variables: Use environment variables to inject sensitive data at runtime.
External Secret Management: Integrate a secret management tool such as Vault or AWS Secrets Manager to securely manage and retrieve secrets.
3. Error Handling
Consistent error handling improves user experience and simplifies debugging.
Global Exception Handling with @ControllerAdvice
Spring Boot provides @ControllerAdvice
and @ExceptionHandler
for centralized error handling. Define a global exception handler to catch exceptions across controllers and provide uniform responses to the client.
Example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<ErrorResponse> handleResourceNotFound(ResourceNotFoundException ex) {
ErrorResponse error = new ErrorResponse("Resource not found", ex.getMessage());
return new ResponseEntity<>(error, HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGeneralException(Exception ex) {
ErrorResponse error = new ErrorResponse("Internal Server Error", "An unexpected error occurred.");
return new ResponseEntity<>(error, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
The above setup allows you to return structured error responses with relevant HTTP status codes. Customize these messages to help users understand issues without exposing sensitive internal details.
Common Pitfall: Overusing Generic Exceptions
Using generic exceptions (like Exception
) without customization can obscure the actual issue and make debugging difficult. Instead, create specific exception classes (e.g., ProductNotFoundException
) and handle them appropriately.
4. Security Implementation
Securing your application is paramount, especially in production environments where data and resources are vulnerable to unauthorized access.
Using Spring Security
Spring Boot integrates well with Spring Security, making it easy to secure REST APIs and manage user access.
Basic Configuration Example:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable()
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.antMatchers("/admin/**").hasRole("ADMIN")
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
Implementing JWT Authentication
For stateless, token-based authentication, use JWT (JSON Web Tokens). This approach provides secure, sessionless authentication ideal for RESTful services. For detailed implementation, consider using Spring Security OAuth2 or custom JWT filters.
Troubleshooting Tip: Validate Tokens in Middleware or Filters
To improve security, validate tokens early in the request lifecycle (using filters) to block unauthorized access before reaching the controller layer.
Common Pitfall: Neglecting CSRF Protection in Non-Token-Based Authentication
If your application uses session-based authentication, ensure Cross-Site Request Forgery (CSRF) protection is enabled. Disable CSRF only when using JWT or other stateless approaches.
5. Logging Setup
Logging is essential for understanding application behavior in production, especially when troubleshooting or monitoring performance.
Configuring Logging Levels
In Spring Boot, configure logging levels in application.yml
or application.properties
:
logging:
level:
root: INFO
com.example: DEBUG
Set different log levels based on the environment—use DEBUG
for development and INFO
or higher for production to prevent performance issues and excessive log data.
Centralized Logging Solutions
For scalable applications, consider centralized logging with tools like the ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk. These tools aggregate logs from different instances and allow for advanced searching and analysis.
Troubleshooting Tip: Avoid Excessive Debug Logging in Production
Excessive debug-level logs can slow down applications and make logs harder to read. Use INFO
or higher for production environments to balance detail and performance.
6. Metrics and Monitoring
Monitoring provides real-time insights into your application’s health and performance, enabling proactive issue resolution.
Spring Boot Actuator for Application Monitoring
Spring Boot Actuator offers built-in endpoints for health, metrics, and application insights. Integrate Actuator endpoints with monitoring tools like Prometheus and Grafana to visualize metrics in dashboards.
Example Actuator Configuration:
management:
endpoints:
web:
exposure:
include: "health, metrics, prometheus"
With Actuator, you can monitor key metrics such as memory usage, request counts, and custom metrics. Health check endpoints are also helpful in load-balanced setups to route traffic only to healthy instances.
Common Pitfall: Ignoring Custom Metrics
Use custom metrics to monitor specific business logic. For example, track the number of successful orders in an e-commerce app to assess business performance alongside technical metrics.
7. Docker Deployment
Docker containers standardize application environments, making deployment consistent and reproducible across various platforms.
Writing a Dockerfile for Spring Boot
Define the Dockerfile to package your Spring Boot application into a container. Use multi-stage builds to minimize the final image size, which improves deployment speed and reduces storage costs.
Example Dockerfile:
# Stage 1: Build
FROM maven:3.8.4-openjdk-11 AS build
WORKDIR /app
COPY . .
RUN mvn clean package -DskipTests
# Stage 2: Run
FROM openjdk:11-jre-slim
COPY --from=build /app/target/app.jar /app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
Deploying with Docker Compose
For applications with multiple services (e.g., database, cache, message broker), use Docker Compose to manage multi-container setups:
Example docker-compose.yml
:
version: '3'
services:
app:
build: .
ports:
- "8080:8080"
environment:
SPRING_PROFILES_ACTIVE: "prod"
db:
image: postgres:13
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
ports:
- "5432:5432"
Using Docker Compose simplifies running your application locally in an environment similar to production. For larger deployments, tools like Kubernetes provide advanced orchestration capabilities.
8. Troubleshooting and Common Pitfalls
Preparing a Spring Boot application for production often involves addressing some common challenges:
Memory Leaks: Monitor for potential memory leaks with profiling tools like VisualVM or YourKit. Common sources include poorly managed caches, unclosed connections, or large collections.
Database Deadlocks: Deadlocks often occur due to conflicting row locks. Resolve them by optimizing query patterns and keeping transactions short.
Slow Boot Times: Identify boot time issues with Spring Boot's
applicationStartup
property, which provides insights into startup phases, allowing you to detect bottlenecks in initialization.
Real-World Case Study: E-Commerce Application Bottleneck
In an e-commerce application, frequent API timeouts were traced back to an unoptimized search function. Switching from SQL queries to an Elasticsearch-based indexing system reduced response times by 60%, improved concurrency, and eliminated slowdowns under high traffic.
Conclusion
Building a production-ready Spring Boot application requires going beyond development basics. Ensuring a robust structure, secure configuration management, standardized logging, centralized monitoring, and efficient deployment setup makes your application more resilient and maintainable.
By following these best practices, you can create a Spring Boot application prepared to handle real-world production demands. While each step can be implemented individually, integrating them together forms a holistic approach to reliability, security, and scalability.
Take these practices one step at a time, experimenting with tools and configurations in your development environment. For further exploration, consult Spring’s extensive documentation, or consider container orchestration with Kubernetes for managing complex deployments.
With these practices in place, your Spring Boot application will be production-ready, providing a solid foundation for future growth and scalability.
Subscribe to my newsletter
Read articles from Jaydeep Ravat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Jaydeep Ravat
Jaydeep Ravat
Java developer with 3 years experience. Committed to clean code, latest technologies, and sharing knowledge through blogging.