How to Add or Remove Text Box in Word Documents with Python [Fast and Active]

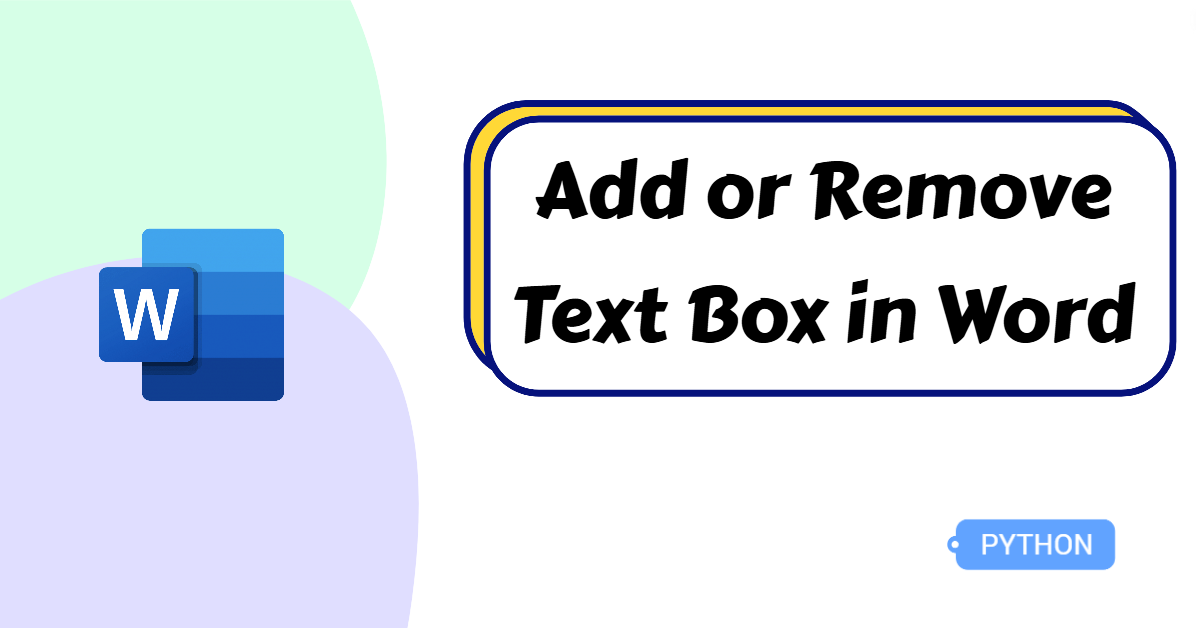
Inserting text boxes in Word documents is a popular way to emphasize key information or create callouts. While Microsoft Word offers built-in features for adding text boxes, manually repeating this task can become time-consuming, especially with larger documents. Luckily, Python can simplify this process, allowing you to efficiently add or remove text boxes with ease. In this article, we'll guide you through how to add or remove text boxes in Word with Python, helping you save time and streamline document editing and management.
Python Library to Add or Remove Text Box in Word
In this guide, we are going to use Spire.Doc for Python to demonstrate the whole process. It is one of the best MS Word alternatives, allowing users to add, remove, extract, and update text boxes quickly and easily.
You can install it with the pip command: pip install Spire.Doc
How to Add Text Box in Word with Python Easily
Spire.Doc(short for Spire.Doc for Python) offers the AppendTextBox() method to insert textboxes anywhere you like. It also provides several properties under the Format class that enable users to customize the position, border style, fill color, and so on. Let’s take a closer look at how it works.
Steps to add text boxes in Word documents:
Create a Document object.
Load a Word document from files using the Document.LoadFromFile() method.
Access a section, then a paragraph, and add a text box with the Sections.Paragraphs.AppendTextBox() method.
Configure properties under the Format class to customize the wrapping style, border style and fill color of the text box.
Insert text into the text box by calling the Paragraph.AppendText() method.
Set the alignment of the text using the Format.HorizontalAlignment property, and set the font style with properties under the CharacterFormat class.
Save the textbox-embedded Word document as a new file by calling the Document.SaveToFile() method.
The following code example demonstrates how to add a text box in the upper right corner of a Word document, complete with both images and text inside the text box:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
document = Document()
# Load a Word document
document.LoadFromFile("/input3.docx")
# Insert a textbox and set its wrapping style
textBox = document.Sections[0].Paragraphs[0].AppendTextBox(135, 300)
textBox.Format.TextWrappingStyle = TextWrappingStyle.Square
# Set the position of the textbox
textBox.Format.HorizontalOrigin = HorizontalOrigin.RightMarginArea
textBox.Format.HorizontalPosition = -145.0
textBox.Format.VerticalOrigin = VerticalOrigin.Page
textBox.Format.VerticalPosition = 120.0
# Set the border style and the fill color of the textbox
textBox.Format.LineColor = Color.get_DarkBlue()
textBox.Format.FillColor = Color.get_LightGray()
# Insert an image to the textbox as a paragraph
para = textBox.Body.AddParagraph()
picture = para.AppendPicture("C:/Users/Administrator/Desktop/Wikipedia_Logo.png")
# Set alignment for the paragraph
para.Format.HorizontalAlignment = HorizontalAlignment.Center
# Set the size of the inserted image
picture.Height = 90.0
picture.Width = 90.0
# Insert text to textbox as the second paragraph
textRange = para.AppendText("Wikipedia is a free encyclopedia, written collaboratively by the people who use it. "
+ "Since 2001, it has grown rapidly to become the world's largest reference website, "
+ "with 6.7 million articles in English attracting billions of views every month.")
# Set alignment for the paragraph
para.Format.HorizontalAlignment = HorizontalAlignment.Center
# Set the font of the text
textRange.CharacterFormat.FontName = "Times New Roman"
textRange.CharacterFormat.FontSize = 12.0
textRange.CharacterFormat.Italic = True
# Save the result file
document.SaveToFile("/AddTextBox.docx", FileFormat.Docx)
# Close the document
document.Close()
How to Remove Text Box from Word Document Quickly
Whether you want to clean up the Word document by deleting extra elements or to simplify the layout, removing text boxes from Word documents is an essential ability. While adding text boxes requires setting various styles and inserting content, removing them is much simpler. It only takes three straightforward steps: read the document, delete the text box, and save your changes. Let’s dive into the details of each step below.
Steps to remove text box from Word documents:
Create an object of the Document class and specify the file path to read Word documents using the Document.LoadFromFile() method.
Remove a certain text box with the TextBoxes.RemoveAt() method, and delete all text boxes by calling the TextBoxes.Clear() method.
Save the modified Word document using the Document.SaveToFile() method.
Below is an example of removing the first text box in the Word document:
from spire.doc import *
from spire.doc.common import *
# Create a Document object
document = Document()
# Load a Word document
document.LoadFromFile("C:/Users/Administrator/Desktop/TextBox.docx")
# Remove the first textbox
document.TextBoxes.RemoveAt(0)
# Remove all textboxes
# document.TextBoxes.Clear()
# Save the result document
document.SaveToFile("output/RemoveTextbox.docx", FileFormat.Docx)
To Wrap Up
This guide covers everything you need to know about adding or removing text boxes in Word documents using Python. By automating these tasks, Python helps save you time and effort, making your document editing process more efficient. We hope you found this guide helpful!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
