Understanding Dockerfile, Docker Images, and Containers

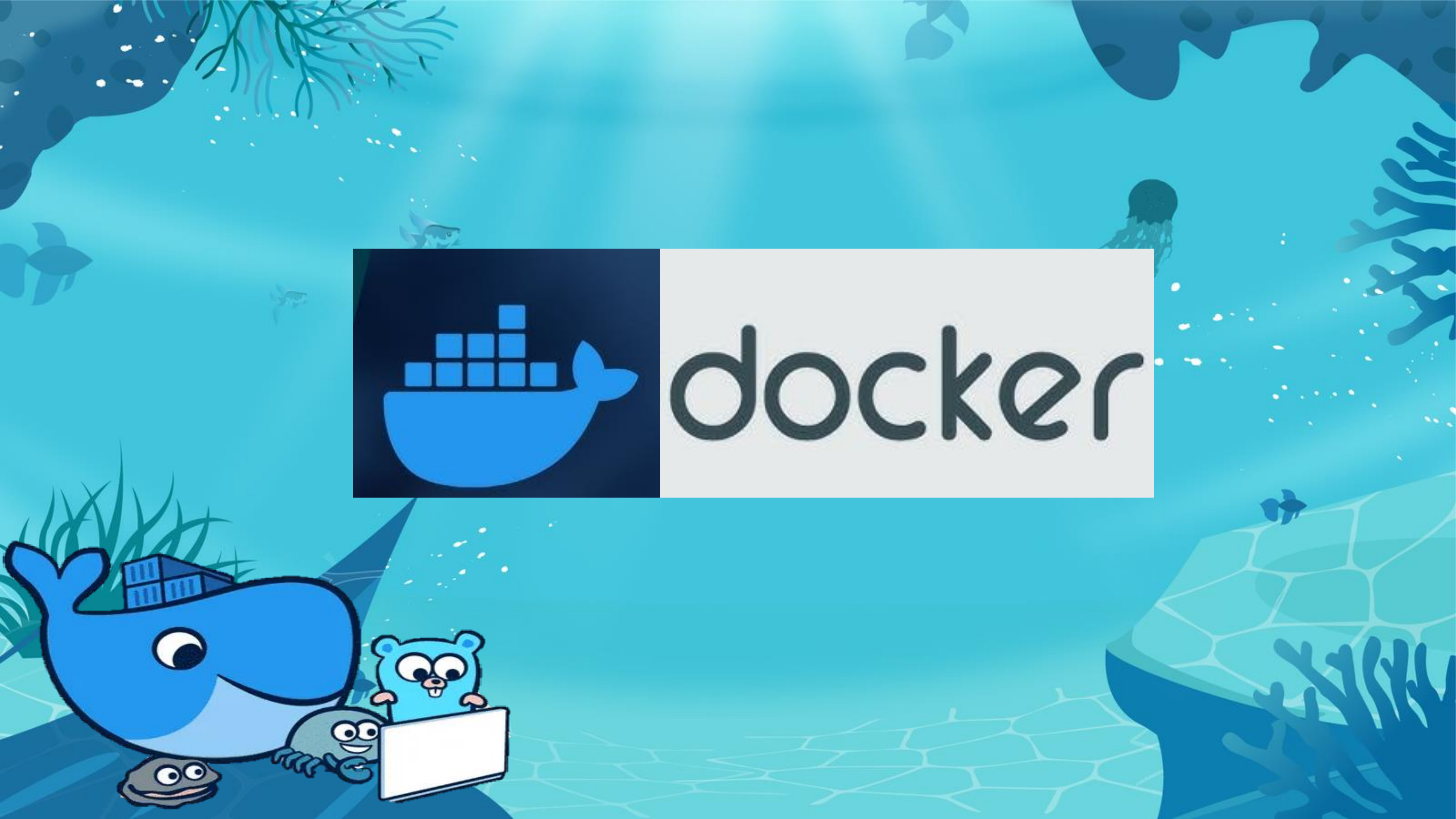
Dockerfile: The Blueprint for Docker Images
A Dockerfile is a simple text file that has a set of instructions for Docker to build a Docker image. You can think of it like a blueprint that tells Docker how to set up your application step by step. It tells Docker which image to use, what software to install, what files to copy, and what commands to run when the container starts.
1. FROM
The FROM command is the first line in every Dockerfile. It tells Docker which image to use as the base. This can be a full operating system image like Ubuntu or a simple runtime like Python or Node.js. This image is the foundation for your app.
Example:
FROM python:3.8-slim
This command tells Docker to use a Python 3.8 image with a smaller operating system version (slim). This helps make the final Docker image smaller in size.
2. WORKDIR
The WORKDIR command sets the directory where Docker should work inside the container. Any commands after WORKDIR will run from this directory. If the directory doesn’t exist, Docker will create it automatically.
Example:
WORKDIR /app
This sets the working directory to /app in the container. Any file copies or commands will be executed inside this /app folder.
3. COPY / ADD
The COPY and ADD commands are used to copy files from your local system to the Docker container. COPY is simpler and recommended because it only copies files. ADD has extra features like extracting .tar
files or downloading files from URLs.
Example:
COPY . /app
This copies all files from your current directory on your local machine to the /app folder in the container.
4. RUN
The RUN command is used to execute commands inside the container while Docker is building the image. You can use it to install software or run setup scripts. Every RUN command adds a new layer to the image, so it’s better to combine commands where possible.
Example:
RUN pip install --no-cache-dir -r requirements.txt
This installs Python dependencies listed in the requirements.txt file. The --no-cache-dir
flag is used to avoid storing unnecessary files and reduce image size.
5. EXPOSE
The EXPOSE command tells Docker that the container will listen on a specific network port. This doesn’t actually expose the port to the host machine, but it tells Docker and other containers that this port is in use.
Example:
EXPOSE 5000
This exposes port 5000 inside the container, typically used for applications like web servers.
6. ENV
The ENV command is used to set environment variables inside the container. These variables can be used to configure your app’s behavior while it’s running.
Example:
ENV APP_ENV=production
This sets the APP_ENV environment variable to production, which your app can use to run in production mode.
7. CMD
The CMD command tells Docker what to do when the container starts. This is the default command that runs when the container starts. If you pass a command while running the container, it will override the CMD command.
Example:
CMD ["python", "app.py"]
This runs the python app.py command when the container starts, which is common for Python applications.
8. ENTRYPOINT
The ENTRYPOINT command is similar to CMD, but it sets the main command for the container to run. It is harder to override compared to CMD. You can use ENTRYPOINT and CMD together to set default arguments.
Example:
ENTRYPOINT ["python"]
CMD ["app.py"]
Here, ENTRYPOINT sets python as the command, and CMD provides the default argument app.py. So, when the container starts, it will run python app.py.
9. VOLUME
The VOLUME command creates a mount point inside the container where you can store data. This is useful if you want data to persist even if the container is stopped or removed. For example, it can be used for databases or log files.
Example:
VOLUME /data
This creates a volume at /data, where you can store data that should remain even if the container is destroyed.
10. USER
The USER command sets the user the container should run as. By default, Docker containers run as the root user, but it's safer to run containers as a non-root user.
Example:
USER appuser
This makes the container run as appuser instead of the default root user, improving security.
11. ARG
The ARG command defines build-time variables you can pass during the build process. These are not available after the image is built, but they help customize the build.
Example:
ARG VERSION=1.0
This defines a build-time argument VERSION to set dynamic version numbers or other parameters during build.
12. LABEL
The LABEL command lets you add metadata to the Docker image. This can include version info, authorship, or other details.
Example:
LABEL version="1.0" maintainer="you@example.com"
This adds version information and maintainer’s email to the image metadata, which helps you manage and track versions.
13. STOPSIGNAL
The STOPSIGNAL command tells Docker which signal to send when stopping the container. By default, Docker sends the SIGTERM signal, but you can change it if your app needs another signal.
Example:
STOPSIGNAL SIGTERM
This sets SIGTERM as the stop signal for the container, asking Docker to gracefully stop it.
14. HEALTHCHECK
The HEALTHCHECK command defines a command that Docker uses to check the health of the container. This is useful for monitoring the container's status, and tools like Kubernetes can restart the container if it’s unhealthy.
Example:
HEALTHCHECK CMD curl --fail http://localhost:8080 || exit 1
This checks if the container’s web service is running by sending a request to localhost:8080. If it fails, it exits with an error, marking the container as unhealthy.
By using these commands in your Dockerfile, you can create efficient and customized images that run your apps in a consistent environment. Docker helps make it easy to deploy apps anywhere, and learning Dockerfiles is an important step to mastering Docker.
What is a Docker Image?
A Docker Image is like a template for creating containers. It’s a snapshot that includes everything needed to run an application: code, libraries, dependencies, configuration files, and even the runtime (like Python or Node.js). Docker images are built from the instructions written in the Dockerfile.
Think of it as a read-only blueprint that contains everything your app needs to run.
Key Points about Docker Images:
Immutable: Once an image is created, it cannot be changed. Any changes are made by creating a new image.
Layered: Docker images are built in layers. Each layer is created by a command in the Dockerfile. Docker reuses these layers, saving space and improving efficiency.
Portable: You can push Docker images to Docker Hub, making it easy to share and run your app on any system with Docker installed.
Built from Dockerfile: Docker images are created using the instructions in the Dockerfile.
What is a Docker Container?
A Docker Container is a running instance of a Docker image. When you run an image, it becomes a container. You can think of a container as an instance of an image, like a lightweight virtual machine.
Containers are isolated from each other and from the host system, making them perfect for running apps in a controlled and predictable environment.
Key Points about Docker Containers:
Ephemeral: Containers are temporary. They are created when you run an image and destroyed when you stop or remove them, but data in volumes or external storage is preserved.
Isolated: Containers run in separate environments, so they don't interfere with each other or the host.
Fast Startup: Containers start quickly because they don’t need to boot a full operating system like virtual machines.
Stateful or Stateless: Containers can store data in volumes (stateful) or lose data when stopped (stateless).
Relationship Between Docker Images and Docker Containers
Images are Templates: A Docker image is a static, read-only file that contains everything your app needs. It’s like a blueprint.
Containers are Running Instances: When you run an image with
docker run
, Docker creates a container based on that image. The container is the live, running version of the image.Containers Use Images to Run: Containers use the image to get their files and configuration, then run based on those instructions.
Images are Reusable: You can reuse an image to create as many containers as needed.
Subscribe to my newsletter
Read articles from Saifulla Syed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
