Flattening an array and little more.

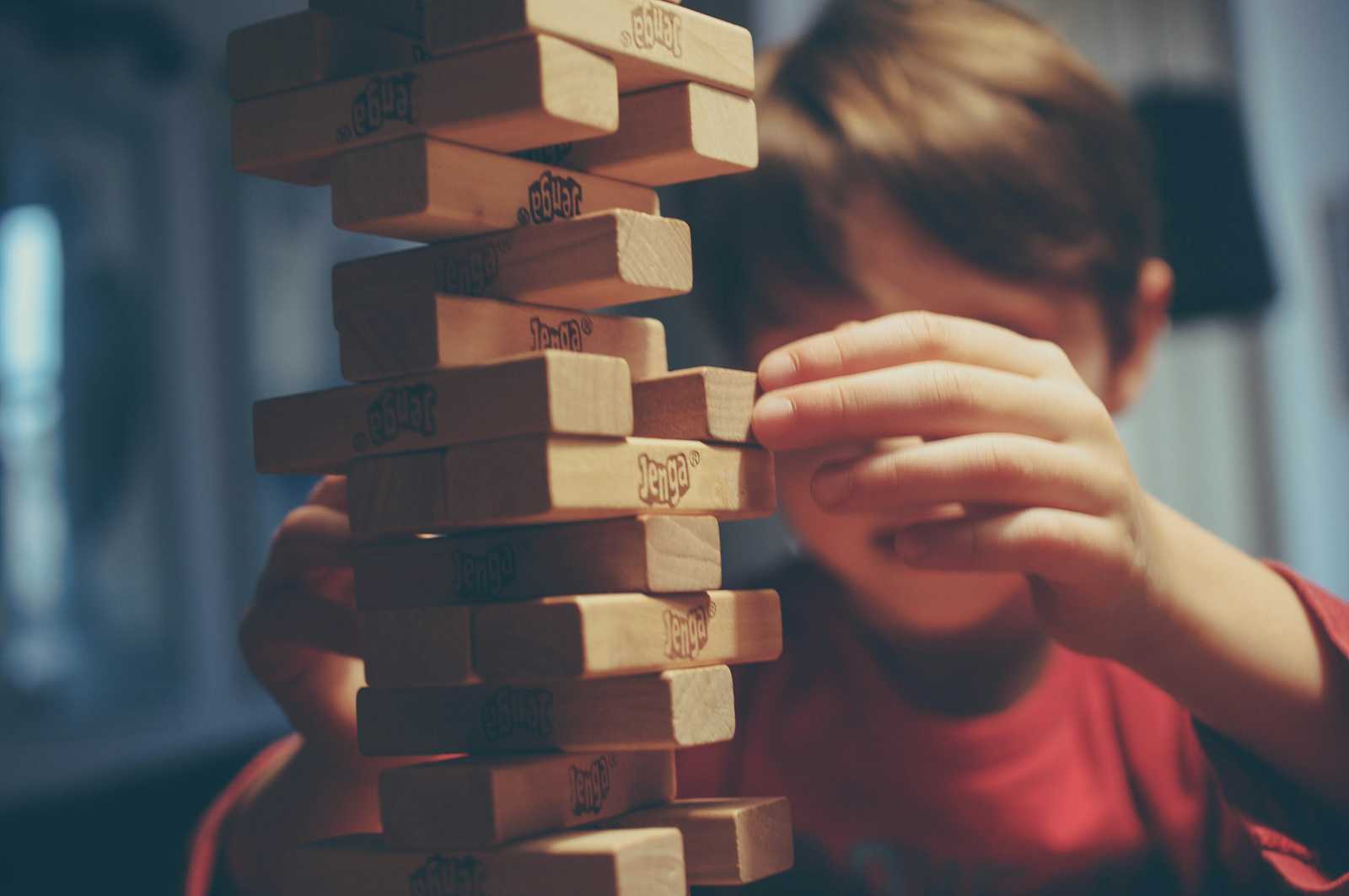
Interviewing coders requires smart and insightful questions to differentiate between those who truly understand coding concepts and those who rely solely on memorised answers. Below are a series of array manipulation questions designed to challenge candidates, complete with explanations and code examples.
I usually believe to put easy questions first and slowly increase the complexity of questions so that candidate feel confident and also warmed up.
Question 1: Basic Array Flattening
Input: [[1, 2], [3, [4, [5, 6]]], 7]
Output: [1, 2, 3, 4, 5, 6, 7]
function flattenArray(arr) {
return arr.reduce((acc, val) => {
return Array.isArray(val) ? acc.concat(flattenArray(val)) : acc.concat(val);
}, []);
}
// Example usage
let input = [[1, 2], [3, [4, [5, 6]]], 7];
console.log(flattenArray(input)); // Output: [1, 2, 3, 4, 5, 6, 7]
Question 2: Flattening While Tracking Nesting Levels
Input: [[1, 2], [3, [4, [5, 6]]], 7]
Output: ['1-1', '2-1', '3-1', '4-2', '5-3', '6-3', '7-0']
function flattenWithLevels(arr, level = 0) {
let result = [];
arr.forEach(item => {
if (Array.isArray(item)) {
result = result.concat(flattenWithLevels(item, level + 1));
} else {
result.push(`${item}-${level}`);
}
});
return result;
}
// Test the function
let input = [[1, 2], [3, [4, [5, 6]]], 7];
console.log(flattenWithLevels(input)); // Output: ['1-1', '2-1', '3-1', '4-2', '5-3', '6-3', '7-0']
Question 3: Reconstructing the Nested Array
Input: ['1-1', '2-1', '3-1', '4-2', '5-3', '6-3', '7-0']
Output: [[1, 2], [3, [4, [5, 6]]], 7]
function reconstructNestedArray(arr) {
let result = [];
let stack = [{ array: result, level: -1 }];
arr.forEach(item => {
let [value, level] = item.split('-').map(Number);
while (stack[stack.length - 1].level >= level) {
stack.pop();
}
let currentArray = stack[stack.length - 1].array;
let newArray = [];
currentArray.push(level > stack[stack.length - 1].level ? newArray : value);
if (level > stack[stack.length - 1].level) {
stack.push({ array: newArray, level: level });
}
});
return result;
}
// Test the function
let input = ['1-1', '2-1', '3-1', '4-2', '5-3', '6-3', '7-0'];
console.log(reconstructNestedArray(input)); // Output: [[1, 2], [3, [4, [5, 6]]], 7]
Final Thoughts
These questions test not only a candidate's ability to manipulate arrays but also their ability to think critically and adapt solutions as complexity increases. Candidates who solve these problems demonstrate a strong understanding of recursion, data structures, and logical problem-solving.
Happy coding and interviewing!
Subscribe to my newsletter
Read articles from Arsalan Adeeb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
