Mastering Order Processing Systems: A Comprehensive Guide to Building Efficient Business Solutions with Python
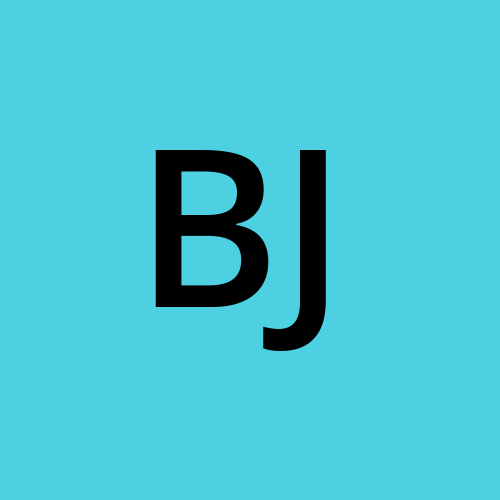
Part 6: Creating the User Interface - Introduction to Tkinter
Introduction
To make our order processing system user-friendly, we’ll build a graphical user interface (GUI) using Tkinter, Python's standard GUI toolkit. Tkinter allows us to create windows, dialogs, forms, buttons, and other UI components that make it easier for users to interact with our application. In this post, we’ll cover the basics of Tkinter, explore the essential components we’ll be using in our project, and set up the main application window to lay the foundation for the rest of the UI.
Basics of Tkinter
Tkinter is a built-in Python library for creating GUIs. Here are some key Tkinter concepts to understand before we dive into building the interface:
Widgets: Widgets are the elements of a graphical interface, such as buttons, labels, text fields, and frames. Tkinter provides a wide variety of widgets for different purposes.
Layouts: Tkinter provides three main layout managers for arranging widgets within windows:
.pack()
,.grid()
, and.place()
. We’ll primarily use.pack()
and.grid()
in our project.Event Handling: Tkinter uses an event-driven model, meaning that actions (like button clicks) trigger events handled by functions we define. This makes the interface interactive and responsive.
Overview of the UI Components Used in the Project
In our order processing system, the GUI will consist of various components designed to facilitate user interactions, such as logging in, placing orders, managing inventory, and accessing reports. Here are the primary UI components we’ll implement:
Login Screen: Allows users to enter their credentials and access the system based on their role (admin, manager, employee).
Order Management Screen: Lets users place orders, update order statuses, and view order details.
Inventory Management Screen: Displays current inventory levels and allows users to update stock.
Admin Screen: Provides admin users with additional options, such as approving new users or managing product data.
Analytics Screen: Displays sales and inventory analytics, such as sales summaries and low-stock alerts.
Setting Up the Main Application Window
The main application window serves as the container for all other UI components. Let’s go ahead and set up a simple Tkinter window that will be the entry point for our application.
Here’s how to create a basic Tkinter window:
Import Tkinter and initialize the main application window.
Configure window properties such as the title and dimensions.
Run the main event loop to display the window and listen for any user actions.
Below is a code snippet to set up the main application window for our order processing system:
import tkinter as tk
from tkinter import ttk
class OrderProcessingApp:
def __init__(self, master):
self.master = master
self.master.title("Order Processing System")
self.master.geometry("800x600") # Set the window size
self.master.configure(bg="light gray") # Background color
# Welcome label
self.welcome_label = ttk.Label(master, text="Welcome to the Order Processing System", font=("Arial", 16))
self.welcome_label.pack(pady=20)
# Instructions
self.instruction_label = ttk.Label(
master,
text="Please use the login screen to access the system features.",
font=("Arial", 12)
)
self.instruction_label.pack(pady=10)
# Button to initiate login (for now, it just prints a message)
self.login_button = ttk.Button(master, text="Go to Login", command=self.go_to_login)
self.login_button.pack(pady=30)
def go_to_login(self):
print("Login screen will be displayed here.") # Placeholder for login screen function
# Initialize Tkinter window
if __name__ == "__main__":
root = tk.Tk()
app = OrderProcessingApp(root)
root.mainloop()
Explanation of Code:
Initialize Tkinter Window: We initialize the main application window using
root =
tk.Tk
()
.Window Properties: We set the title of the window to "Order Processing System" and configure its dimensions to
800x600
.Welcome Label: A welcome label displays a message to the user, giving the application a friendly start.
Login Button: For now, the "Go to Login" button simply prints a message. Later, it will take the user to the login screen.
Main Event Loop:
root.mainloop()
keeps the window open and active until the user closes it.
Running the Code
To see this basic window in action, follow these steps:
Save the code in a file (e.g.,
main_
app.py
).Run the script in your terminal:
python main_app.py
You should see a window open with the title "Order Processing System," a welcome message, and a button that says "Go to Login."
Understanding Tkinter Widgets and Layouts in This Example
In this setup, we used three types of widgets:
Label Widget (
ttk.Label
): Displays static text in the window. We used labels to show welcome and instructional messages.Button Widget (
ttk.Button
): Represents a clickable button. We added a "Go to Login" button, which, when clicked, will eventually direct users to the login screen.Pack Layout Manager (
.pack()
): This layout manager arranges widgets in blocks before placing them in the parent widget. We used.pack()
with optional padding (pady
) to give each widget some spacing.
In the next parts of this series, we’ll expand upon this foundation by creating more sophisticated screens, such as a fully functional login screen, an order entry screen, and more.
Conclusion
In this post, we introduced Tkinter and created the main application window for our order processing system. We discussed the basics of Tkinter, including widgets, layouts, and event handling, and set up the foundation for the user interface. This window will serve as the entry point for the application, from which users can navigate to various features.
In the next part, we’ll create a secure login interface where users can authenticate with their credentials. Stay tuned as we continue building the GUI for our order processing system!
Link to My Source code : https://github.com/BryanSJamesDev/-Order-Processing-System-OPS-/tree/main
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
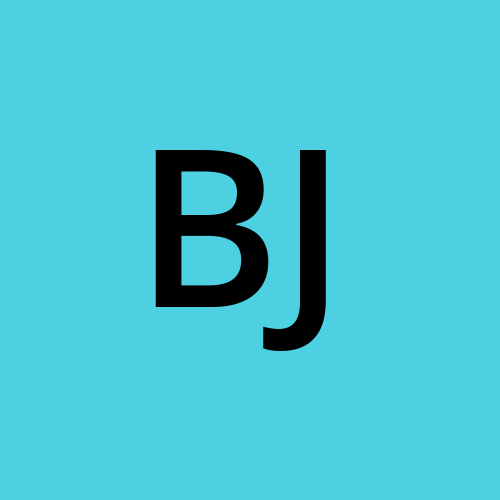