Introduction to Node.js

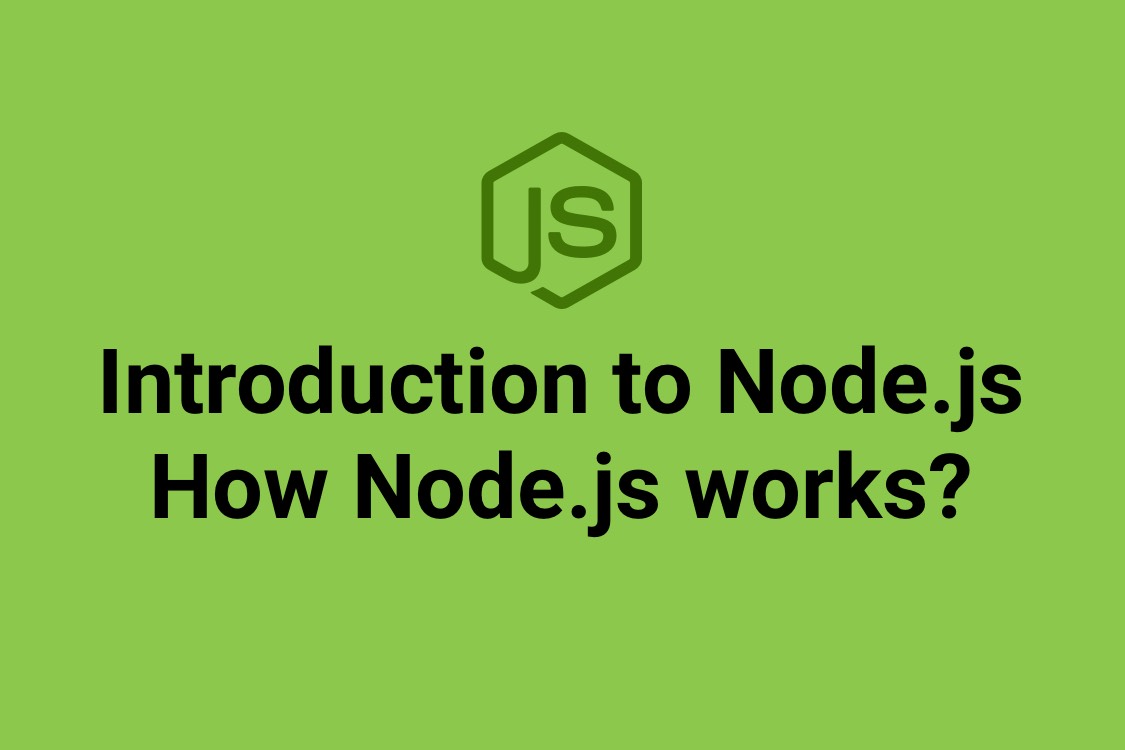
What is Node.js?
Node.js is a JavaScript runtime that allows developers to run JavaScript outside of the web browser. It is built on top of the V8 JavaScript engine, which is the same engine used by the Google Chrome web browser to execute JavaScript. With Node.js, developers can use JavaScript for server-side scripting, creating network applications, and building highly scalable programs that can handle a large number of concurrent connections.
Node.js vs. Browser JavaScript
The key differences between Node.js and browser-based JavaScript are:
Environment: Node.js runs in a server environment, while browser JavaScript runs in the web browser environment.
System Access: Node.js has direct access to system resources like the file system, network, and operating system, while browser JavaScript is limited to the DOM (Document Object Model) and CSS APIs provided by the browser.
Asynchronous I/O: Node.js uses an event-driven, non-blocking I/O model to handle asynchronous operations efficiently, while browser JavaScript is typically more synchronous and can block the main thread.
These differences make Node.js particularly well-suited for building scalable network applications and server-side services, as it can handle many concurrent connections efficiently without blocking the main thread.
Key Differences Between Node.js and Browsers: A Comprehensive Guide for Developers
• Node.js and web browsers are two distinct yet interconnected technologies in web development, both utilizing JavaScript as their primary programming language.
• Despite this commonality, they exhibit significant differences in their execution environments, available APIs, and target use.
• Node.js operates as a runtime environment for JavaScript outside the browser, enabling server-side programming and excelling in real-time applications due to its non-blocking I/O approach.
• In contrast, web browsers serve as platforms for accessing and viewing websites, interpreting JavaScript code embedded within HTML to facilitate user interactions and dynamic web pages.
• While Node.js employs a command-line interface for server-side development, browsers provide a graphical user interface for seamless user interaction with web content.
• Node.js and browsers differ in their module import mechanisms, supported module systems, and the availability of certain objects and APIs, such as document and window objects in browsers.
The Event Loop
The event loop is a core concept in Node.js that enables efficient asynchronous operations. The event loop continuously checks for new events (e.g., incoming network requests, file I/O operations) and processes them as they occur, without blocking the main thread. This model allows Node.js to be highly scalable and responsive, as it can handle multiple concurrent connections without slowing down.
npm (Node Package Manager)
npm is the default package manager for Node.js. It allows developers to easily install, manage, and share reusable JavaScript packages (modules) within their Node.js projects. The npm ecosystem is vast, with millions of publicly available packages that can be used to extend the functionality of Node.js applications, facilitating collaboration and code reuse.
ECMAScript 2015+ Support
Node.js fully supports the latest versions of the ECMAScript (ES) language specification, including ES6 (also known as ES2015) and beyond. This means that Node.js developers can take advantage of modern JavaScript features, such as classes, arrow functions, modules, and other syntactical and functional improvements, keeping their Node.js applications up-to-date with the latest web development practices.
TypeScript Support
In addition to native JavaScript, Node.js also supports TypeScript, a statically typed superset of JavaScript. TypeScript can be used in Node.js projects through transpilation (converting TypeScript to JavaScript) or native compilation (using the TypeScript compiler directly in Node.js). This allows for improved code maintainability and reduced runtime errors in Node.js applications.
Security Best Practices
When building Node.js applications, it's essential to follow security best practices to protect against common vulnerabilities, such as:
Validating user input to prevent injection attacks
Using HTTPS to encrypt communication
Keeping Node.js and dependencies up-to-date to address security fixes
Being cautious when using third-party libraries and modules
Adhering to these practices helps create secure server-side applications.
Debugging Node.js Applications
Node.js provides built-in debugging tools, such as the Node.js debugger, which can be used to step through code, set breakpoints, and inspect variables. Additionally, developers can use the Chrome DevTools to debug Node.js applications, taking advantage of the comprehensive debugging features available in the Chrome browser. Effective debugging is crucial for identifying and resolving issues in complex Node.js applications.
Profiling Node.js Apps
Profiling Node.js applications is essential for identifying and resolving performance bottlenecks. Node.js provides the Node.js Inspector tool, which can be used for in-depth profiling and performance analysis. Developers can also leverage external profiling tools, such as the Chrome DevTools profiler, to gain deeper insights into their Node.js applications. Understanding where time is spent in an application allows developers to optimize code for better performance.
Reading and Writing Files with Node.js
Node.js provides several ways to read and write files using the fs
(file system) module:
Reading Files
Using fs.readFile()
The fs.readFile()
function is the simplest way to read a file asynchronously. It takes the file path, an optional encoding (e.g., 'utf8'), and a callback function that receives the error (if any) and the file data.
const fs = require('fs');
fs.readFile('/path/to/file.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
Using fs.readFileSync()
The fs.readFileSync()
function is a synchronous version of fs.readFile()
. It blocks the event loop while reading the file, which can impact the overall performance of the application, especially for larger files or in high-concurrency scenarios.
const fs = require('fs');
try {
const data = fs.readFileSync('/path/to/file.txt', 'utf8');
console.log(data);
} catch (err) {
console.error(err);
}
Using fsPromises.readFile()
The fsPromises.readFile()
function provides a Promise-based API for reading files. This approach offers better error handling and more readable code, especially when combined with the async/await
syntax.
const fs = require('fs/promises');
async function example() {
try {
const data = await fs.readFile('/path/to/file.txt', { encoding: 'utf8' });
console.log(data);
} catch (err) {
console.error(err);
}
}
example();
Writing Files
Basic File Write
The fs.writeFile()
function is the most straightforward way to write content to a file. It takes the file path, the content to be written, an optional encoding, and a callback function to handle any errors.
const fs = require('fs');
const content = 'This is the content to be written to the file.';
fs.writeFile('example.txt', content, 'utf8', (error) => {
if (error) {
console.error('An error occurred while writing to the file:', error);
return;
}
console.log('File has been written successfully.');
});
Appending to a File
To append text to an existing file or create a new one, you can use the fs.appendFile()
function. This method adds the content to the end of the file, creating the file if it doesn't exist.
const fs = require('fs').promises;
async function appendToFile(filePath, content) {
try {
await fs.appendFile(filePath, content + '\n', { flag: 'a' });
console.log(`Content appended to ${filePath}`);
} catch (error) {
console.error(`Error appending to file: ${error.message}`);
}
}
Overwriting a File
To overwrite the contents of a file, you can use the fs.writeFile()
function. This will replace the entire contents of the file with the new data.
const fs = require('fs').promises;
async function overwriteFile(filePath, content) {
try {
await fs.writeFile(filePath, content, 'utf8');
console.log(`File ${filePath} overwritten successfully`);
} catch (error) {
console.error(`Error overwriting file: ${error.message}`);
}
}
By understanding these concepts and following best practices, you can effectively work with files in your Node.js applications.
Subscribe to my newsletter
Read articles from Muhammed Musa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Muhammed Musa
Muhammed Musa
From optimizing search results to building the future of the web - that's my journey. I'm Muhammed Musa, an SEO specialist with 5 years of experience, now venturing into the exciting realms of full-stack development and blockchain technology. I aim to blend my SEO expertise with cutting-edge web development and blockchain skills to create innovative, discoverable, decentralized solutions. I'm passionate about staying at the forefront of digital technology and eager to contribute to projects that push the boundaries of what's possible on the web.