Simplifying NLP with Stemming and Lemmatization Techniques
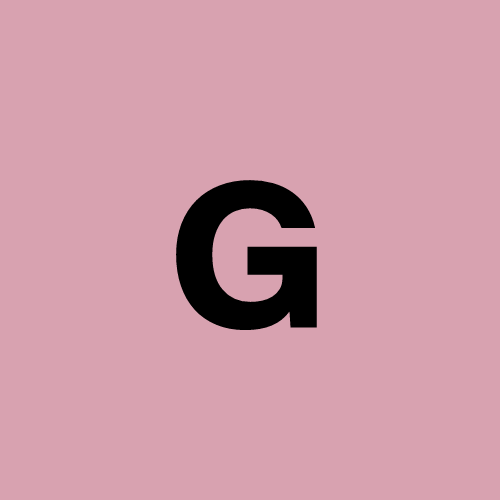
Stemming
Stemming is the process of simplifying a word to its basic form or root. This means removing any suffixes or prefixes to get to the core part of the word, which is called the lemma. For example, in stemming, words like "running," "runner," and "runs" would all be reduced to the root word "run." This helps in understanding and processing text because it groups similar words together based on their core meaning.
Example:
Word | Word Stem |
eating, eat, eaten | eat |
runs, running, runner | run |
Stemming is needed in Natural Language Processing (NLP) for several reasons:
Text Normalization: Stemming helps in normalizing text by reducing words to their base or root form. This is essential for ensuring that different forms of a word are treated as the same entity, which simplifies text processing.
Improved Search and Retrieval: By reducing words to their stems, stemming enhances search engines and information retrieval systems. It allows these systems to match queries with relevant documents more effectively, even if the exact word forms differ.
Dimensionality Reduction: In text analysis, stemming reduces the dimensionality of the data by decreasing the number of unique words. This makes it easier to analyze and model the data, improving computational efficiency.
Consistency in Text Analysis: Stemming ensures consistency in text analysis by grouping similar words together. This is particularly useful in tasks like sentiment analysis, topic modeling, and clustering, where variations of a word should be considered equivalent.
Language Processing Efficiency: By simplifying words, stemming reduces the complexity of language processing tasks, making algorithms faster and more efficient. This is crucial for handling large volumes of text data in real-time applications.
Different Types of Stemming
There are several stemming algorithms available, each with its own approach and complexity. The most common ones include:
Porter Stemmer: One of the oldest and most widely used stemming algorithms. It uses a series of rules to iteratively reduce words to their stems.
RegexpStemmerClass: This approach uses regular expressions to identify and remove suffixes from words. It provides a customizable way to define stemming rules based on specific patterns.
Snowball Stemmer: An improvement over the Porter Stemmer, offering more flexibility and support for multiple languages.
Practical Example of Stemming
Porter Stemmer
## Importing NLTK
import nltk
from nltk.stem import PorterStemmer
# Ensure you have the necessary resources
nltk.download('punkt')
# Initialize the Porter Stemmer
porter_stemmer = PorterStemmer()
# Sample words to stem
words = ["running", "runner", "runs", "easily", "fairly"]
# Perform stemming
stems = [porter_stemmer.stem(word) for word in words]
# Print the results
for word, stem in zip(words, stems):
print(f"Original: {word} -> Stemmed: {stem}")
Output:
Original: running -> Stemmed: run
Original: runner -> Stemmed: runner
Original: runs -> Stemmed: run
Original: easily -> Stemmed: easili
Original: fairly -> Stemmed: fairli
RegexpStemmerClass
from nltk.stem import RegexpStemmer
# Define a regular expression pattern for stemming
# This example removes common suffixes like 'ing', 'ly', 'ed', 'er', 's'
pattern = r'ing$|ly$|ed$|er$|s$'
# Create a RegexpStemmer object with the defined pattern
regexp_stemmer = RegexpStemmer(pattern)
# List of words to be stemmed
words = ['running', 'runner', 'runs', 'easily', 'fairly']
# Apply the stemmer to each word
stemmed_words = [regexp_stemmer.stem(word) for word in words]
# Output the results
for original, stemmed in zip(words, stemmed_words):
print(f'Original: {original} -> Stemmed: {stemmed}')
Output:
Original: running -> Stemmed: runn
Original: runner -> Stemmed: runn
Original: runs -> Stemmed: run
Original: easily -> Stemmed: easi
Original: fairly -> Stemmed: fair
Snowball Stemmer
from nltk.stem import SnowballStemmer
# Create a SnowballStemmer object for English
snowball_stemmer = SnowballStemmer("english")
# List of words to be stemmed
words = ['running', 'runner', 'runs', 'easily', 'fairly']
# Apply the stemmer to each word
stemmed_words = [snowball_stemmer.stem(word) for word in words]
# Output the results
for original, stemmed in zip(words, stemmed_words):
print(f'Original: {original} -> Stemmed: {stemmed}')
Output:
Original: running -> Stemmed: run
Original: runner -> Stemmed: runner
Original: runs -> Stemmed: run
Original: easily -> Stemmed: easili
Original: fairly -> Stemmed: fair
Disadvantages of Stemming
Stemming, while useful, has several disadvantages that can impact the accuracy and effectiveness of text processing tasks:
Over-stemming: This occurs when a stemming algorithm reduces a word to a root that is too short or not meaningful. For example, the word "university" might be stemmed to "univers," which is not a valid word. Over-stemming can lead to loss of meaning and context, making it difficult to accurately interpret the text.
Under-stemming: This happens when the stemming process does not reduce words to the same root, even though they are related. For instance, "data" and "datum" might not be stemmed to the same root, leading to inconsistencies in text analysis.
Lack of Context: Stemming algorithms typically do not consider the context in which a word is used. This can result in different words with similar stems being treated as equivalent, even when they have different meanings. For example, "better" and "bet" might be stemmed to the same root, despite having different meanings.
Language Limitations: Many stemming algorithms are designed for specific languages and may not work well with others. This can be a limitation in multilingual text processing tasks.
Lemmatization
Lemmatization is a crucial process in natural language processing (NLP) that involves reducing a word to its base or dictionary form, known as the lemma. Unlike stemming, which often simply cuts off prefixes or suffixes, lemmatization considers the context and the part of speech of a word to ensure that it is reduced to a meaningful base form. Here’s why lemmatization is important:
Context Awareness: Lemmatization takes into account the context and grammatical role of a word, ensuring that words are reduced to their correct base form. For example, "better" would be lemmatized to "good," preserving its meaning.
Accuracy: By using a more sophisticated approach that involves understanding the word's meaning and context, lemmatization provides more accurate results than stemming, especially in complex text analysis tasks.
Consistency: Lemmatization ensures that words with similar meanings are treated consistently, which is crucial for tasks like sentiment analysis and information retrieval.
Language Flexibility: Lemmatization can be adapted to work with multiple languages, making it a versatile tool for global applications.
Practical Example of Lemmatization
from nltk.stem import WordNetLemmatizer
import nltk
nltk.download('wordnet')
# Initialize the WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
# List of words to be lemmatized
words = ['running', 'runner', 'runs', 'easily', 'fairly', 'better']
# Apply the lemmatizer to each word
lemmatized_words = [lemmatizer.lemmatize(word) for word in words]
# Output the results
for original, lemmatized in zip(words, lemmatized_words):
print(f'Original: {original} -> Lemmatized: {lemmatized}')
Output:
Original: running -> Lemmatized: running
Original: runner -> Lemmatized: runner
Original: runs -> Lemmatized: run
Original: easily -> Lemmatized: easily
Original: fairly -> Lemmatized: fairly
Original: better -> Lemmatized: better
Usecases of Stemming and Lemmatization
Stemming and lemmatization are both used in natural language processing (NLP) to simplify and normalize text, but they serve slightly different purposes and are used in various applications:
Use Cases of Stemming:
Search Engines: Stemming helps search engines match user queries with relevant documents by reducing words to their base forms. This allows the search engine to retrieve documents containing different forms of a word.
Text Mining and Information Retrieval: In text mining, stemming reduces the dimensionality of the data by decreasing the number of unique words, making it easier to analyze and model the data.
Sentiment Analysis: Stemming can help in grouping similar words together, which is useful in sentiment analysis to ensure that variations of a word are considered equivalent.
Topic Modeling and Clustering: By reducing words to their stems, stemming ensures consistency in text analysis, which is crucial for tasks like topic modeling and clustering.
Real-time Text Processing: Stemming simplifies words, reducing the complexity of language processing tasks and making algorithms faster and more efficient, which is important for real-time applications.
Use Cases of Lemmatization:
Machine Translation: Lemmatization provides more accurate translations by considering the context and grammatical role of words, ensuring that words are translated to their correct base forms.
Information Retrieval: Lemmatization improves the accuracy of information retrieval systems by ensuring that words with similar meanings are treated consistently.
Text Analysis and NLP Applications: In complex text analysis tasks, lemmatization provides more precise results by understanding the word's meaning and context, which is crucial for applications like chatbots and virtual assistants.
Document Classification: Lemmatization helps in accurately classifying documents by reducing words to their dictionary forms, ensuring that similar words are treated as the same entity.
Multilingual Applications: Lemmatization can be adapted to work with multiple languages, making it a versatile tool for global applications where understanding the true meaning of words is essential.
Overall, stemming is often used for its speed and simplicity, while lemmatization is preferred for its accuracy and context-awareness in applications where understanding the true meaning of words is critical.
Part of Speech
In natural language processing (NLP), Part of Speech (POS) refers to the grammatical category of a word in a sentence. POS tagging is the process of labeling each word in a text with its corresponding part of speech, such as noun, verb, adjective, adverb, etc. This helps in understanding the structure and meaning of the text. Here's a simple breakdown:
Nouns (NN): These are words that represent people, places, things, or ideas. For example, "dog," "city," and "happiness" are nouns.
Verbs (VB): Verbs are action words that describe what the subject is doing. Examples include "run," "eat," and "think."
Adjectives (JJ): Adjectives describe or modify nouns, providing more information about them. For instance, "quick," "blue," and "happy" are adjectives.
Adverbs (RB): Adverbs modify verbs, adjectives, or other adverbs, often indicating how, when, where, or to what extent something happens. Examples are "quickly," "very," and "yesterday."
Pronouns (PRP): Pronouns are words that replace nouns to avoid repetition. Examples include "he," "she," "it," and "they."
Prepositions (IN): Prepositions show the relationship between a noun (or pronoun) and other words in a sentence. Examples are "in," "on," and "at."
Conjunctions (CC): Conjunctions connect words, phrases, or clauses. Examples include "and," "but," and "or."
Determiners (DT): Determiners introduce nouns and specify them in terms of definiteness, quantity, or possession. Examples are "the," "a," and "some."
POS tagging is essential in NLP because it provides the foundational structure needed for more complex text analysis tasks, such as parsing, sentiment analysis, and machine translation. By understanding the role each word plays in a sentence, NLP systems can better interpret and process human language.
import nltk
nltk.download('averaged_perceptron_tagger_eng')
from nltk import pos_tag
from nltk.tokenize import word_tokenize
# Sample sentence
sentence = "The quick brown fox jumps over the lazy dog."
# Tokenize the sentence into words
words = word_tokenize(sentence)
# Perform POS tagging
pos_tags = pos_tag(words)
# Output the results
for word, tag in pos_tags:
print(f'Word: {word} -> POS Tag: {tag}')
Output:
Word: The -> POS Tag: DT
Word: quick -> POS Tag: JJ
Word: brown -> POS Tag: NN
Word: fox -> POS Tag: NN
Word: jumps -> POS Tag: VBZ
Word: over -> POS Tag: IN
Word: the -> POS Tag: DT
Word: lazy -> POS Tag: JJ
Word: dog -> POS Tag: NN
Word: . -> POS Tag: .
Named Entity Tagging
Named Entity Tagging, often referred to as Named Entity Recognition (NER), is a process in natural language processing (NLP) that involves identifying and classifying key elements in text into predefined categories. These categories typically include:
Person Names: Identifying names of people, such as "Albert Einstein" or "Marie Curie."
Organizations: Recognizing names of organizations, such as "Google" or "United Nations."
Locations: Identifying geographical locations, such as "Paris" or "Mount Everest."
Dates and Times: Extracting date and time expressions, such as "January 1, 2020" or "3 PM."
Monetary Values: Recognizing expressions of currency, such as "$100" or "€50."
Percentages: Identifying percentage expressions, such as "50%" or "two-thirds."
NER is crucial for various applications, including information retrieval, question answering, and content recommendation systems. By tagging named entities, systems can better understand the context and meaning of the text, enabling more accurate and relevant responses or actions.
import nltk
nltk.download('maxent_ne_chunker_tab')
nltk.download('words')
from nltk import word_tokenize, pos_tag, ne_chunk
# Sample sentence
sentence = "Barack Obama was born in Honolulu and was the 44th President of the United States."
# Tokenize the sentence into words
words = word_tokenize(sentence)
# Perform POS tagging
pos_tags = pos_tag(words)
# Perform Named Entity Recognition
named_entities = ne_chunk(pos_tags)
# Output the results
print(named_entities)
(PERSON Barack/NNP) (PERSON Obama/NNP) was/VBD born/VBN in/IN (GPE Honolulu/NNP) and/CC was/VBD the/DT 44th/JJ President/NNP of/IN the/DT (GPE United/NNP States/NNPS)
Code:
Stemming: https://github.com/genvia-tech/generative_ai/tree/main/Stemming
Lemmatization: https://github.com/genvia-tech/generative_ai/tree/main/Lemmatization
Parts of Speech and Named entity tagging: https://github.com/genvia-tech/generative_ai/tree/main/PartOfSpeech
Stemming and lemmatization are key processes in natural language processing (NLP) for simplifying and normalizing text. Stemming reduces words to their root form, enhancing text normalization, search efficiency, and dimensionality reduction, while lemmatization considers context for more accurate word representation. Different stemming algorithms, like Porter, Snowball, and Regexp, offer varying levels of complexity. Part of Speech (POS) tagging categorizes words into grammatical categories to aid NLP tasks. Named Entity Recognition (NER) identifies and classifies entities in text, crucial for applications like information retrieval and content recommendation.
Subscribe to my newsletter
Read articles from genvia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
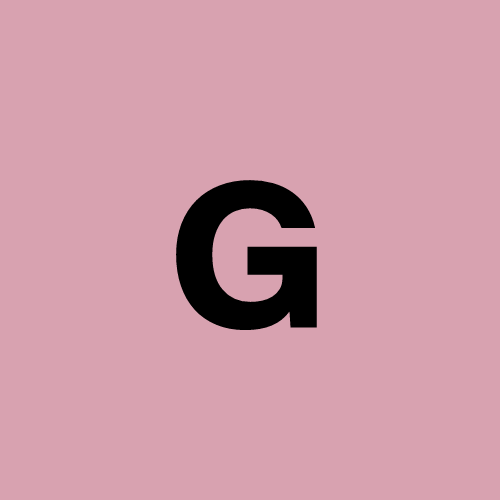