2024 Beginner's Guide to Learning Go (Golang)

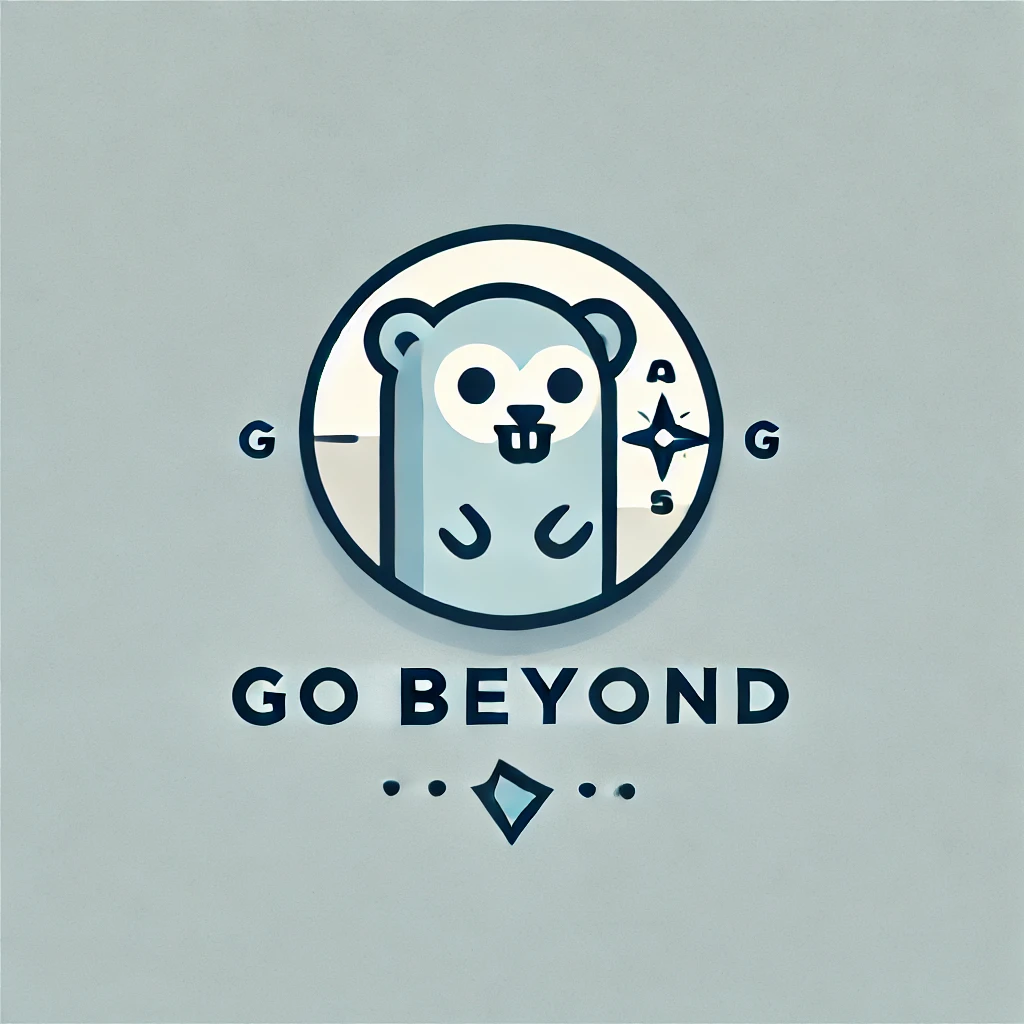
A Journey into Modern Programming Excellence
Welcome, fellow developers and coding enthusiasts! Whether you're a veteran programmer exploring new horizons or taking your first steps into the programming world, you're about to embark on an exciting journey with one of the most transformative programming languages of our time.
Table of Contents
Introduction: Why Go Matters in 2024 {#introduction}
In today's tech landscape, where complexity often reigns supreme, Go stands as a beacon of clarity and efficiency. But what makes Go particularly relevant in 2024?
The Modern Developer's Swiss Army Knife
Cloud-Native Development: With the explosion of cloud computing and microservices, Go has become the de facto language for cloud-native applications
AI/ML Infrastructure: Companies like OpenAI use Go for their infrastructure components
DevOps & SRE: Major tools like Kubernetes, Docker, and Terraform are written in Go
Web Services: From high-performance APIs to real-time web applications
Key Statistics (2024)
Over 2.5 million developers worldwide use Go
Consistently ranks in the top 10 most loved languages on Stack Overflow
Average Go developer salary: $140,000+ (US)
76% of developers report improved productivity after switching to Go
The Story Behind Go {#history}
The Perfect Storm at Google
Picture this: It's 2007, and Google's engineers are drowning in a sea of complexity. Build times are measured in coffee breaks, code bases are becoming unmanageable, and multicore processors are becoming the norm, but programming languages haven't caught up.
The Dream Team
Three extraordinary minds came together:
Robert Griesemer: The language design expert who helped create the V8 JavaScript engine
Rob Pike: The Unix veteran and UTF-8 co-creator
Ken Thompson: The legendary Bell Labs researcher who gave us Unix and C
Their mission? Create a language that would:
Compile at the speed of thought
Make concurrent programming easy and safe
Be readable enough that you could understand someone else's code without a manual
Scale from small scripts to massive systems
Why Go Was Created {#why-go}
The Problems Go Solves
The Complexity Crisis
C++ and Java's overwhelming feature sets
Python's performance limitations
Lack of built-in concurrency support in mainstream languages
Modern Computing Challenges
Multicore processors being underutilized
Distributed systems becoming the norm
Build times affecting developer productivity
Dependencies becoming unmanageable
Go's Solutions
Simplicity by Design
// Example of Go's simplicity type User struct { Name string Age int } func (u User) Greet() string { return fmt.Sprintf("Hello, I'm %s!", u.Name) }
Built-in Concurrency
// Example of concurrent programming in Go func processItems(items []string) { for _, item := range items { go func(i string) { // Process each item concurrently fmt.Println("Processing:", i) }(item) } }
Getting Started with Go {#installation}
Modern Installation Methods (2024)
Official Installation
# Windows (using winget) winget install GoLang.Go # macOS (using Homebrew) brew install go # Linux (using apt) sudo apt install golang-go
Using Docker
FROM golang:1.22 WORKDIR /app
Environment Setup
# Create your Go workspace
mkdir -p ~/go/{bin,src,pkg}
# Add to your profile (.bashrc, .zshrc, etc.)
export GOPATH=$HOME/go
export PATH=$PATH:$GOPATH/bin
Your First Go Adventure {#first-program}
The Modern "Hello, World!"
package main
import (
"fmt"
"time"
)
func main() {
name := "Gopher"
currentTime := time.Now().Format("15:04:05")
fmt.Printf("Hello, %s! The time is %s\n", name, currentTime)
}
Understanding the Code
Package Declaration: Every Go file starts with
package main
Imports: Standard library packages are imported using their path
Main Function: The entry point of every Go program
Variable Declaration: Using the
:=
shorthand for declaration and assignmentString Formatting: Using
Printf
for formatted output
Go's Evolution: From Birth to Maturity {#evolution}
Recent Milestones (2022-2024)
Go 1.18: Introduction of Generics
Go 1.19: Performance improvements in the garbage collector
Go 1.20: Enhanced error handling and fuzzing
Go 1.21: Built-in slices package and faster compilation
Go 1.22: Enhanced HTTP routing and improved tooling
Go 1.23: (Expected) Further performance optimizations
Modern Features Showcase
// Generics Example (Go 1.18+)
func Min[T constraints.Ordered](x, y T) T {
if x < y {
return x
}
return y
}
// Error Handling (Modern Approach)
if err := doSomething(); err != nil {
err = fmt.Errorf("failed to do something: %w", err)
return err
}
The Go Developer's Mindset {#mindset}
Core Principles for 2024
Embrace Simplicity
Clear is better than clever
Explicit is better than implicit
Small functions and packages
Think Concurrently
Goroutines are cheap, use them
Channels for communication
Share memory by communicating
Performance Matters
Profile before optimizing
Use benchmarks
Understand the garbage collector
Best Practices
// Good Practice: Use meaningful names
func ProcessUserData(user User) error {
// vs "func process(u User)"
// Good Practice: Error handling
if err != nil {
return fmt.Errorf("failed to process user %s: %w", user.ID, err)
}
// Good Practice: Structured logging
log.WithFields(log.Fields{
"user_id": user.ID,
"action": "process",
}).Info("Processing user data")
Learning Resources and Community {#resources}
Modern Learning Paths
Interactive Learning
Go Playground (Updated 2024)
Tour of Go
Exercism's Go Track
Books & Documentation
"Learning Go" (2024 Edition)
"Go Programming Language" (Classic)
Official Go Blog
Community Resources
Go Discord Server
r/golang
Local Go Meetups
Recommended Project Ideas
Beginner
CLI tool for file management
Simple REST API
Task manager
Intermediate
Chat application using WebSockets
Microservice with gRPC
Custom database driver
Advanced
Kubernetes operator
Distributed cache system
High-performance web server
What's Next in Your Go Journey {#conclusion}
Immediate Next Steps
Set up your Go development environment
Complete the Tour of Go
Build your first command-line tool
Join the Go community on Discord or Reddit
Looking Ahead
The Go ecosystem is continuously evolving, with exciting developments in:
AI/ML tooling
WebAssembly support
Cloud-native development
Performance optimizations
Final Thoughts
Go's journey from a solution to Google's problems to a mainstream programming language is a testament to its design principles. As you begin your Go journey, remember that simplicity is your friend, and the Go community is here to help.
Stay tuned for our next article in the series, where we'll dive deep into Go's type system and explore how to write idiomatic Go code!
Did you find this guide helpful? Share it with your network and let us know your thoughts in the comments below! Don't forget to follow for more Go content.
Subscribe to my newsletter
Read articles from Sundaram Kumar Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sundaram Kumar Jha
Sundaram Kumar Jha
I Like Building Cloud Native Stuff , the microservices, backends, distributed systems and cloud native tools using Golang