State in Angular Apps
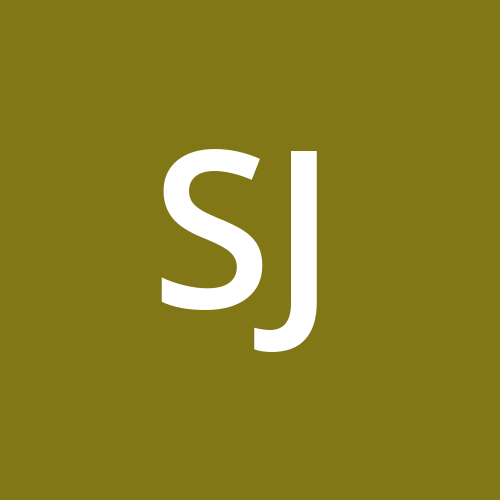
In Angular (and frontend applications in general), state can be categorized into different types based on what the state represents and how it is managed. Two common types are UI state and Model state, but there are a few more types worth mentioning:
1. UI State
Description: This state manages the visual aspects and behavior of the user interface. It includes states like loading indicators, modals visibility, form input values, error messages, active tabs, and selected items.
Examples:
Whether a sidebar is open or closed.
Which tab is currently active.
Whether a loading spinner is visible.
Characteristics: UI state is often ephemeral (short-lived) and is specific to a particular component or page.
2. Model State (Data State)
Description: This state represents the actual data fetched from APIs or databases that the application operates on. It is often stored in services or a state management store (e.g., NgRx, Akita) and includes entities like users, products, orders, etc.
Examples:
A list of users fetched from a server.
The current user profile data.
Data of a specific item to be edited in a form.
Characteristics: Model state is more persistent and typically comes from external data sources like APIs.
3. Global (Application) State
Description: This state is shared across multiple parts of the application. It can include both UI and model state but has a broader scope, influencing the behavior of the entire app.
Examples:
User authentication status (e.g., logged in or not).
The current theme or language settings.
Characteristics: Global state is usually managed with a centralized store like NgRx or NgXS in Angular.
4. Component (Local) State
Description: This is the state that is specific to an individual component and not shared outside of it. It is usually short-lived and tied to the lifecycle of the component.
Examples:
Form control values in a single form component.
The selected item in a dropdown.
Characteristics: Component state is ephemeral and reset when the component is destroyed.
5. Server State
Description: Server state refers to the state managed by the backend that can affect the frontend. It is often synced or fetched from the backend and may include cached responses or results of a user action that affects the database.
Examples:
Search results fetched from a server based on user input.
Notifications fetched from a server in real-time.
Characteristics: It requires communication with the backend, and changes can occur outside the client application (e.g., via a push notification).
6. Router State
Description: This state is related to the current route or navigation state of the application. It includes information about the active route, query parameters, and route parameters.
Examples:
The current page the user is on.
Query parameters for a search result page.
Characteristics: Router state is typically managed using Angular's
Router
andActivatedRoute
services.
Other Common Names
Application State: Refers to the state that spans across the whole application, encompassing global and shared states.
Session State: A subset of the application state that is tied to a particular user session, often stored in session storage or local storage.
Summary Table
Type | Description | Examples |
UI State | Controls visual components and UI behavior | Modal visibility, loading spinners |
Model State | Data fetched from APIs, representing entities | User data, product list |
Global State | Shared across the app, broader scope | Auth status, app theme |
Component State | Local to a specific component | Form values, dropdown selection |
Server State | Synced with backend, can change externally | API responses, real-time notifications |
Router State | Related to the current route/navigation | Active route, query params |
Managing State in Angular
Local Component State: Managed directly in the component class with properties.
Global State: Managed using services or state management libraries like NgRx, NgXS, or Akita.
Router State: Managed using Angular's Router and ActivatedRoute services.
UI State: Can be managed locally in the component or using shared services/state stores if the UI state needs to be shared across multiple components.
Understanding the different types of state helps in making decisions about where and how to store and manage data in an Angular application.
Subscribe to my newsletter
Read articles from Sumaya J. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
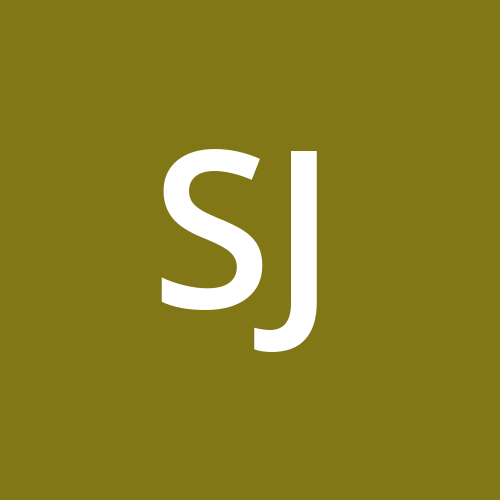