Part 3: Component-Based Design: Building with Reusable Parts
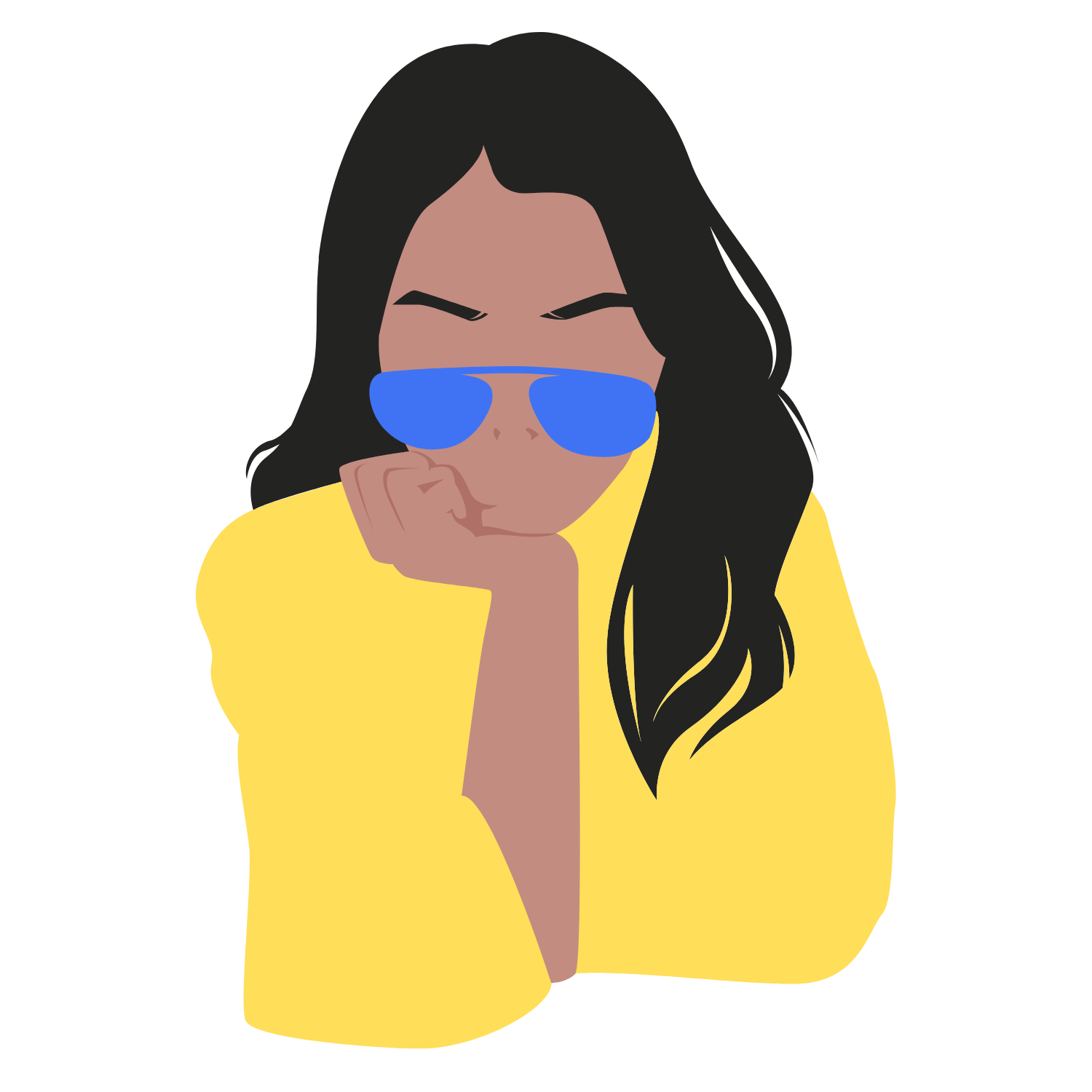
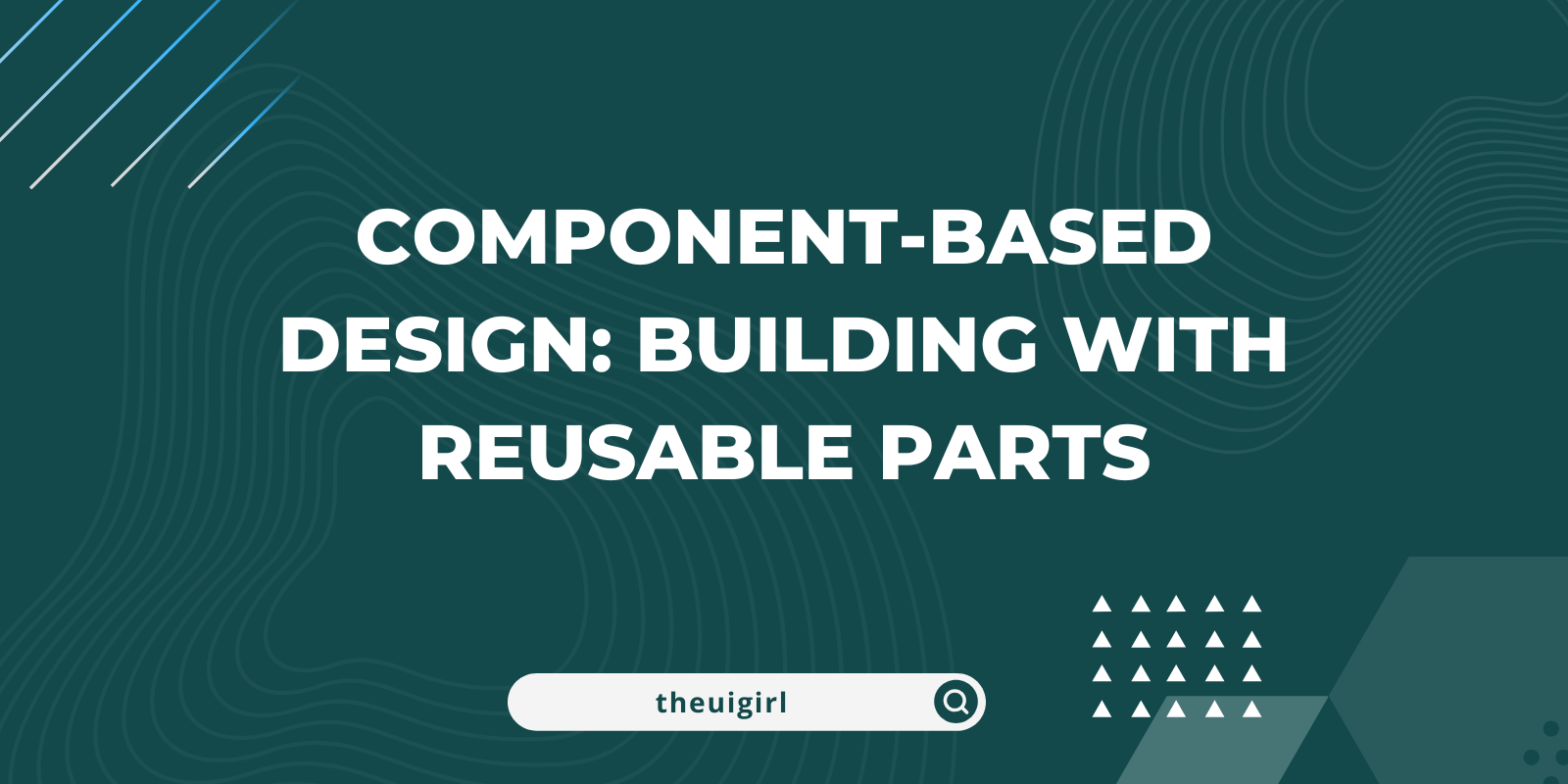
In frontend development, building a scalable and maintainable codebase is essential, especially as projects grow. One of the best approaches to achieve this is by adopting component-based design. Component-based design means breaking down the user interface (UI) into small, reusable parts, or components. Each component has a specific responsibility, making it easier to develop, test, and maintain.
In this article, we’ll cover:
What component-based design is
Key benefits of reusable components
How to build simple, reusable components
Practical examples in React
1. What is Component-Based Design?
Component-based design is a modular approach to building UIs. Instead of creating a monolithic UI, you break it down into individual, self-contained components, each representing a specific part of the interface (like buttons, forms, navigation bars, etc.).
Each component can:
Encapsulate structure and behavior (HTML and JavaScript).
Have its own styling.
Communicate with other components through props or state management.
This approach allows you to build complex interfaces by assembling these small, reusable components.
2. Key Benefits of Reusable Components
a) Scalability
Components can be added, removed, or rearranged independently without affecting the entire application.
b) Maintainability
When you need to make changes, updating a single component can update all instances where it is used.
c) Consistency
Using the same component across multiple parts of an app ensures a consistent design and behavior.
d) Reusability
Once created, components can be reused across different projects or sections of the app, reducing redundant code.
3. How to Build Simple, Reusable Components
When building a component, consider:
Purpose: What does this component do?
Props: What information will it need to display or function?
State: Does it need to manage its own state, or is it purely presentational?
Let’s look at some examples to illustrate this approach.
4. Practical Examples in React
We'll build two simple reusable components in React: Button and Card.
Example 1: A Reusable Button Component
Let's create a simple Button
component that can be customized with different labels, colors, and click behaviors.
import React from 'react';
// Button component definition
const Button = ({ label, onClick, color = 'blue' }) => {
return (
<button
onClick={onClick}
style={{ backgroundColor: color, padding: '10px 20px', color: 'white', border: 'none', borderRadius: '4px' }}
>
{label}
</button>
);
};
export default Button;
Explanation
Props: The
Button
component takes three props:label
: The text displayed on the button.onClick
: The function to execute when the button is clicked.color
: The background color of the button, with a default color of blue.
Usage: Now we can reuse this button in different parts of our application, passing different props as needed.
Using the Button Component
import React from 'react';
import Button from './Button';
const App = () => {
return (
<div>
<Button label="Primary Action" onClick={() => alert('Primary clicked!')} color="blue" />
<Button label="Secondary Action" onClick={() => alert('Secondary clicked!')} color="green" />
</div>
);
};
export default App;
Example 2: A Reusable Card Component
Let’s create a Card
component to display some content in a boxed format.
import React from 'react';
// Card component definition
const Card = ({ title, description, footer }) => {
return (
<div style={{ border: '1px solid #ddd', borderRadius: '8px', padding: '16px', margin: '8px', width: '300px' }}>
<h2>{title}</h2>
<p>{description}</p>
<div style={{ borderTop: '1px solid #ddd', padding: '8px 0 0', marginTop: '8px', textAlign: 'right' }}>
{footer}
</div>
</div>
);
};
export default Card;
Explanation
Props: The
Card
component takes three props:title
: The title text displayed at the top of the card.description
: A short description or content displayed in the body of the card.footer
: An optional footer element (e.g., a button or a small text).
Usage: This
Card
component can display any content and footer, making it a versatile UI building block.
Using the Card Component
import React from 'react';
import Card from './Card';
import Button from './Button';
const App = () => {
return (
<div style={{ display: 'flex', gap: '16px' }}>
<Card
title="Card Title 1"
description="This is a description of the card content. It explains what the card is about."
footer={<Button label="Learn More" onClick={() => alert('Learn more clicked!')} />}
/>
<Card
title="Card Title 2"
description="This is another description, showcasing the reusability of the Card component."
footer={<Button label="Explore" onClick={() => alert('Explore clicked!')} color="green" />}
/>
</div>
);
};
export default App;
Conclusion
Component-based design is a powerful approach for frontend developers, offering modularity, reusability, and maintainability. By creating small, self-contained components like Button
and Card
, we can build complex interfaces from simple parts while ensuring a consistent, scalable codebase.
This article is just the beginning—experimenting with component-based design in your projects will help solidify these concepts. Happy coding!
Subscribe to my newsletter
Read articles from Anisha Swain | The UI Girl directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
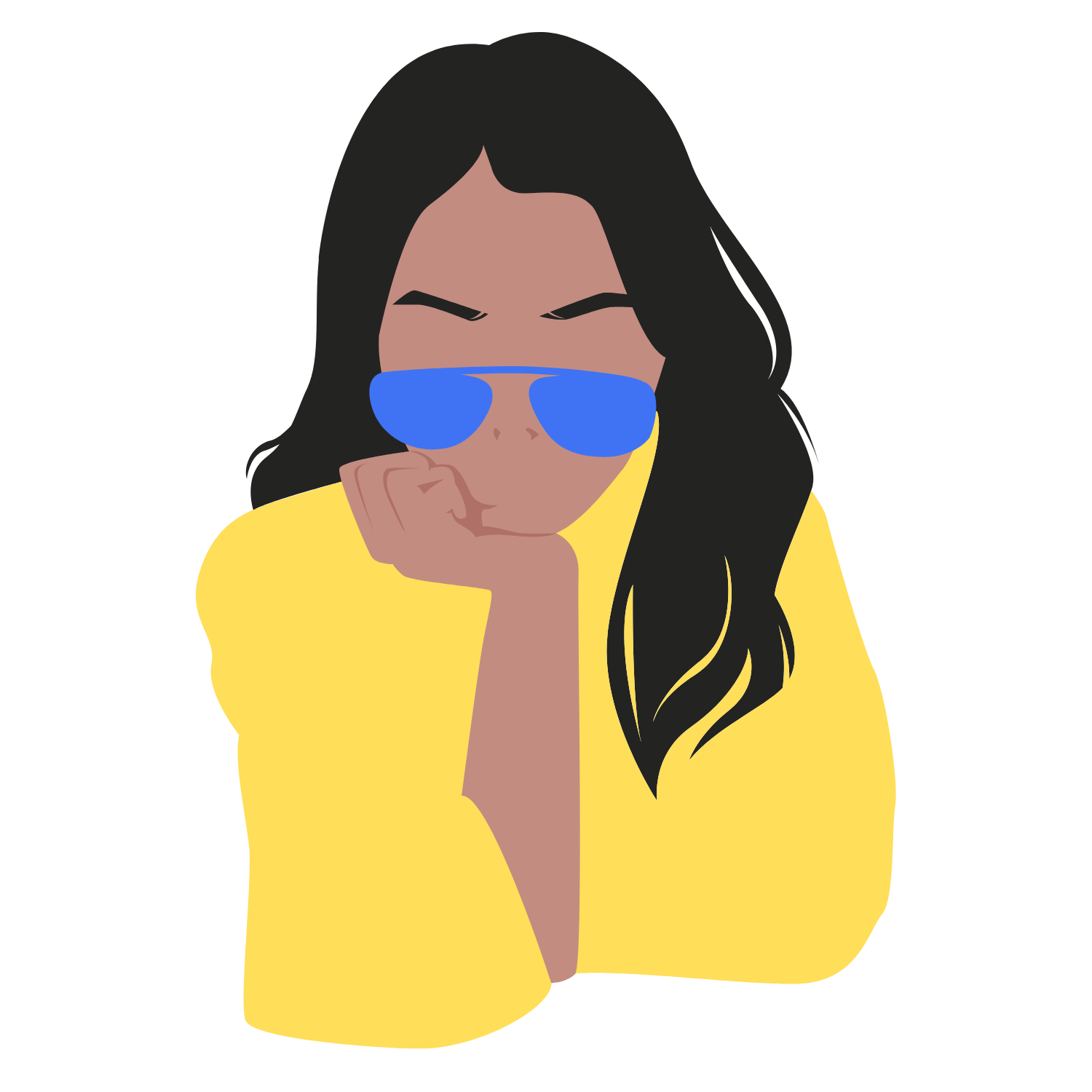
Anisha Swain | The UI Girl
Anisha Swain | The UI Girl
Anisha Swain | The UI Girl Hello world! Anisha this side👋 💪Making @theuigirl 💻 speaker http://t.ly/9D22 🍀 1:1 https://topmate.io/anishaswain 🎙️ podcast host http://t.ly/_MUml ✏️ blog https://medium.com/the-ui-girl 🧳 Travel story http://t.ly/Xa-5v https://bento.me/anishaswain Let's connect 🤝