Common Terraform Interview Scenarios: Infrastructure Migration and Drift Detection
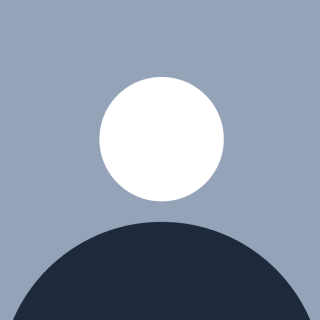
As organizations increasingly adopt Infrastructure as Code (IaC) practices, Terraform has become a crucial skill for DevOps engineers. This article explores two of the most commonly asked Terraform interview scenarios and provides detailed solutions for handling them effectively.
Scenario 1: Infrastructure Migration to Terraform
The Challenge
You join a team that has existing infrastructure created through other means (e.g., CloudFormation, manual configuration) and need to migrate it to Terraform management. How do you handle this transition?
Solution Steps
Create Initial Terraform Configuration
# main.tf provider "aws" { region = "us-east-1" } import { id = "<instance-id>" to = aws_instance.example }
Generate Resource Configuration
terraform init terraform plan -generate-config out=generated_resource.tf
Import Existing Resources
terraform import aws_instance.example <instance-id>
Best Practices
Always start with a small subset of resources to test the migration process
Document all imported resources and their original state
Verify the state file after import to ensure all attributes are correctly captured
Remove unnecessary configuration options that Terraform generates
Scenario 2: Drift Detection
The Challenge
How do you detect and manage manual changes made to Terraform-managed infrastructure outside of Terraform?
Solution Approaches
Using Terraform Refresh
Set up a cron job to run
terraform refresh
periodicallyMonitor state changes and send notifications
Limitations: Command is being deprecated, not real-time
Implementing Strict IAM Policies
Restrict direct AWS console access
Require approval for manual changes
Create super-user accounts for emergency access
Audit Logs and Automation
Monitor CloudWatch or other logging tools
Set up Lambda functions to detect changes
Components:
Resource inventory tracking
Change detection through audit logs
Automated notifications
Identification of change source (Terraform role vs. IAM user)
Implementation Example for Audit Solution
def lambda_handler(event, context):
# List of resources managed by Terraform
terraform_managed_resources = get_terraform_managed_resources()
# Get the change details from CloudWatch
changed_resource = event['detail']['resources']
change_made_by = event['detail']['userIdentity']
# Check if changed resource is managed by Terraform
if resource_is_managed_by_terraform(changed_resource, terraform_managed_resources):
if not change_made_by_terraform_role(change_made_by):
send_notification(
f"Manual change detected on Terraform-managed resource: {changed_resource}"
f"Change made by: {change_made_by}"
)
Interview Tips
For Migration Scenarios:
Emphasize experience with state management
Discuss challenges faced during large-scale migrations
Mention the importance of testing and validation
Explain the process of handling dependencies
For Drift Detection:
Show understanding of multiple approaches
Discuss pros and cons of each solution
Emphasize the importance of automation
Demonstrate knowledge of AWS services and IAM
Conclusion
Understanding these scenarios and their solutions is crucial for Terraform practitioners. While the migration scenario has a straightforward technical solution, drift detection requires a more comprehensive approach combining tools, policies, and automation.
Remember that the best solution often depends on your organization's specific needs, scale, and security requirements. Being able to discuss these trade-offs and demonstrate practical experience with these scenarios will set you apart in Terraform-related interviews.
Subscribe to my newsletter
Read articles from Amulya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by